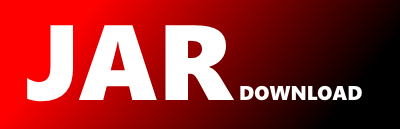
com.datatorrent.lib.join.MapJoinOperator Maven / Gradle / Ivy
/**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package com.datatorrent.lib.join;
import java.util.Calendar;
import java.util.HashMap;
import java.util.Map;
import org.apache.hadoop.classification.InterfaceStability;
/**
* This class takes a HashMap tuple as input from each of the input port. Operator joines the input tuples
* based on join constraint and emit the result.
*
*
* Ports :
* input1 : Input port for stream 1, expects HashMap<String, Object>
* input2 : Input port for stream 2, expects HashMap<String, Object>
* outputPort: Output port emits ArrayList<HashMap<String, Object>>
*
* Example:
* Input tuple from port1 is
* {timestamp = 5000, productId = 3, customerId = 108, regionId = 4, amount = $560 }
*
* Input tuple from port2 is
* { timestamp = 5500, productCategory = 8, productId=3 }
*
* Properties:
* expiryTime: 1000
* includeFieldStr: timestamp, customerId, amount; productCategory, productId
* keyFields: productId, productId
* timeFields: timestamp, timestamp
* bucketSpanInMillis: 500
*
* Output
* { timestamp = 5000, customerId = 108, amount = $560, productCategory = 8, productId=3}
*
* @displayName MapJoin Operator
* @category join
* @tags join
*
* @since 3.4.0
*/
@InterfaceStability.Unstable
public class MapJoinOperator extends AbstractJoinOperator
© 2015 - 2025 Weber Informatics LLC | Privacy Policy