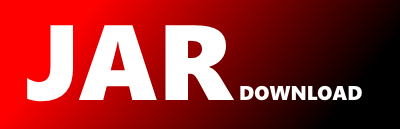
com.datatorrent.lib.logs.ApacheLogParseMapOutputOperator Maven / Gradle / Ivy
/**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package com.datatorrent.lib.logs;
import java.text.ParseException;
import java.util.Arrays;
import java.util.HashMap;
import java.util.Map;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.datatorrent.api.Context.OperatorContext;
import com.datatorrent.api.DefaultInputPort;
import com.datatorrent.api.DefaultOutputPort;
import com.datatorrent.api.annotation.OperatorAnnotation;
import com.datatorrent.api.annotation.Stateless;
import com.datatorrent.common.util.BaseOperator;
/**
* This operator parses apache logs one line at a time (each tuple is a log line), using the given regex.
* A mapping from log line sections to values is created for each log line and emitted.
*
* This is a pass through operator
*
* StateFull : No
* Partitions : Yes, No dependency among input values.
*
* Ports:
* data: expects String
* output: emits Map
*
* Properties:
* logRegex: defines the regex
* groupMap: defines the mapping from the group ids to the names
*
* @displayName Apache Log Parse Map
* @category Tuple Converters
* @tags apache, parse
*
* @since 0.9.4
*/
@Stateless
@OperatorAnnotation(partitionable = true)
public class ApacheLogParseMapOutputOperator extends BaseOperator
{
/**
* The apache log pattern regex
*/
private String logRegex = getDefaultAccessLogRegex();
/**
* This defines the mapping from group Ids to name
*/
private String[] regexGroups = getDefaultRegexGroups();
/**
* This is the list of extractors
*/
private final Map infoExtractors = new HashMap();
private transient Pattern accessLogPattern;
/**
* This is the input port which receives apache log lines.
*/
public final transient DefaultInputPort data = new DefaultInputPort()
{
@Override
public void process(String s)
{
try {
processTuple(s);
} catch (ParseException ex) {
throw new RuntimeException("Could not parse the input string", ex);
}
}
};
/**
* This is the output port which emits one tuple for each Apache log line.
* Each tuple is a Map whose keys represent various sections of a log line,
* and whose values represent the contents of those sections.
*/
public final transient DefaultOutputPort
© 2015 - 2025 Weber Informatics LLC | Privacy Policy