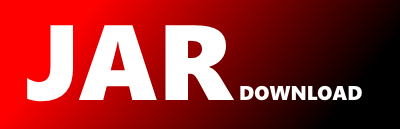
com.datatorrent.lib.math.MinKeyVal Maven / Gradle / Ivy
/**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package com.datatorrent.lib.math;
import java.util.HashMap;
import java.util.Map;
import com.datatorrent.api.DefaultInputPort;
import com.datatorrent.api.DefaultOutputPort;
import com.datatorrent.api.StreamCodec;
import com.datatorrent.lib.util.BaseNumberKeyValueOperator;
import com.datatorrent.lib.util.KeyValPair;
/**
*
* This operator emits minimum of all values sub-classed from Number for each key in KeyValPair at end of window.
*
*
* Ports:
* data: expects KeyValPair<K,V extends Number>
* min: emits KeyValPair<K,V extends Number>, one entry per key
*
* Properties:
* inverse: if set to true the key in the filter will block tuple
* filterBy: List of keys to filter on
*
* @displayName Minimum Key Value
* @category Math
* @tags minimum, numeric, key value
* @since 0.3.2
*/
public class MinKeyVal extends BaseNumberKeyValueOperator
{
/**
* Input port which takes a key vaue pair and updates the value for each key if there is a new min.
*/
public final transient DefaultInputPort> data = new DefaultInputPort>()
{
/**
* For each key, updates the hash if the new value is a new min.
*/
@Override
public void process(KeyValPair tuple)
{
K key = tuple.getKey();
V tval = tuple.getValue();
if (!doprocessKey(key) || (tval == null)) {
return;
}
V val = mins.get(key);
if (val == null) {
mins.put(cloneKey(key), tval);
} else if (val.doubleValue() > tval.doubleValue()) {
mins.put(key, tval);
}
}
/**
* Set StreamCodec used for partitioning.
*/
@Override
public StreamCodec> getStreamCodec()
{
return getKeyValPairStreamCodec();
}
};
/**
* Min value output port.
*/
public final transient DefaultOutputPort> min = new DefaultOutputPort>();
protected HashMap mins = new HashMap();
/**
* Emits all key,min value pairs.
* Clears internal data. Node only works in windowed mode.
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
@Override
public void endWindow()
{
if (!mins.isEmpty()) {
for (Map.Entry e: mins.entrySet()) {
min.emit(new KeyValPair(e.getKey(), e.getValue()));
}
mins.clear();
}
}
}