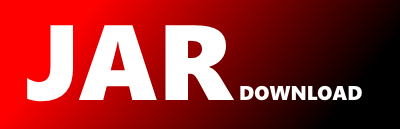
com.datatorrent.lib.math.QuotientMap Maven / Gradle / Ivy
/**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package com.datatorrent.lib.math;
import java.util.HashMap;
import java.util.Map;
import javax.validation.constraints.Min;
import org.apache.commons.lang.mutable.MutableDouble;
import com.datatorrent.api.DefaultInputPort;
import com.datatorrent.api.DefaultOutputPort;
import com.datatorrent.api.annotation.OperatorAnnotation;
import com.datatorrent.lib.util.BaseNumberKeyValueOperator;
/**
* Add all the values for each key on "numerator" and "denominator" and emits quotient at end of window for all keys in the denominator.
*
*
* Application can set multiplication value for quotient(default = 1).
* Operator will calculate quotient of occurrence of key in numerator divided by
* occurrence of key in denominator if countKey flag is true.
* Application can allow or block keys by setting filter key and inverse flag.
*
* StateFull : Yes, numerator/denominator values are summed over
* application window.
* Partitions : No, , will yield wrong results, since values are summed
* over app window.
*
* Ports:
* numerator: expects Map<K,V extends Number>
* denominator: expects Map<K,V extends Number>
* quotient: emits HashMap<K,Double>
*
* Properties:
* inverse : if set to true the key in the filter will block tuple
* filterBy : List of keys to filter on
* countkey : Get quotient of occurrence of keys in numerator and
* denominator.
* mult_by : Set multiply by constant value.
*
* @displayName Quotient Map
* @category Math
* @tags division, sum, map
* @since 0.3.3
*/
@OperatorAnnotation(partitionable = false)
public class QuotientMap extends
BaseNumberKeyValueOperator
{
/**
* Numerator key/sum value map.
*/
protected HashMap numerators = new HashMap();
/**
* Denominator key/sum value map.
*/
protected HashMap denominators = new HashMap();
/**
* Count occurrence of keys if set to true.
*/
boolean countkey = false;
/**
* Quotient multiply by value.
*/
int mult_by = 1;
/**
* Numerator input port.
*/
public final transient DefaultInputPort