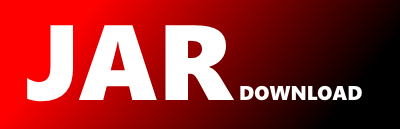
com.datatorrent.lib.math.Sum Maven / Gradle / Ivy
/**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package com.datatorrent.lib.math;
import java.util.HashMap;
import java.util.Map;
import com.datatorrent.api.DefaultInputPort;
import com.datatorrent.api.DefaultOutputPort;
import com.datatorrent.api.Operator.Unifier;
import com.datatorrent.api.annotation.OutputPortFieldAnnotation;
import com.datatorrent.lib.util.BaseNumberValueOperator;
import com.datatorrent.lib.util.UnifierSumNumber;
/**
* This operator implements Unifier interface and emits the sum of values at the end of window.
*
* This is an end of window operator. Application can turn this into accumulated
* sum operator by setting cumulative flag to true.
* StateFull : Yes, sum is computed over application window >= 1.
* Partitions : Yes, sum is unified at output port.
*
* Ports:
* data: expects V extends Number
* sum: emits V extends Number
* sumDouble: emits Double
* sumFloat: emits Float
* sumInteger: emits Integer
* sumLong: emits Long
* sumShort: emits Short
*
* Properties:
* cumulative Sum has to be cumulative.
*
* @displayName Sum
* @category Math
* @tags numeric, sum
* @param
* Generic number type parameter.
* @since 0.3.3
*/
public class Sum extends BaseNumberValueOperator implements
Unifier
{
/**
* Sum value.
*/
protected double sums = 0;
/**
* Input tuple processed flag.
*/
protected boolean tupleAvailable = false;
/**
* Accumulate sum flag.
*/
protected boolean cumulative = false;
/**
* Input port to receive data. It computes sum and count for each tuple.
*/
public final transient DefaultInputPort data = new DefaultInputPort()
{
/**
* Computes sum and count with each tuple
*/
@Override
public void process(V tuple)
{
Sum.this.process(tuple);
tupleAvailable = true;
}
};
/**
* Unifier process override.
*/
@Override
public void process(V tuple)
{
sums += tuple.doubleValue();
tupleAvailable = true; // also need to set here for Unifier
}
/**
* Output sum port.
*/
@OutputPortFieldAnnotation(optional = true)
public final transient DefaultOutputPort sum = new DefaultOutputPort()
{
@Override
public Unifier getUnifier()
{
UnifierSumNumber ret = new UnifierSumNumber();
ret.setVType(getType());
return ret;
}
};
/**
* Output double sum port.
*/
@OutputPortFieldAnnotation(optional = true)
public final transient DefaultOutputPort sumDouble = new DefaultOutputPort()
{
@Override
public Unifier getUnifier()
{
UnifierSumNumber ret = new UnifierSumNumber();
ret.setType(Double.class);
return ret;
}
};
/**
* Output integer sum port.
*/
@OutputPortFieldAnnotation(optional = true)
public final transient DefaultOutputPort sumInteger = new DefaultOutputPort()
{
@Override
public Unifier getUnifier()
{
UnifierSumNumber ret = new UnifierSumNumber();
ret.setType(Integer.class);
return ret;
}
};
/**
* Output Long sum port.
*/
@OutputPortFieldAnnotation(optional = true)
public final transient DefaultOutputPort sumLong = new DefaultOutputPort()
{
@Override
public Unifier getUnifier()
{
UnifierSumNumber ret = new UnifierSumNumber();
ret.setType(Long.class);
return ret;
}
};
/**
* Output short sum port.
*/
@OutputPortFieldAnnotation(optional = true)
public final transient DefaultOutputPort sumShort = new DefaultOutputPort()
{
@Override
public Unifier getUnifier()
{
UnifierSumNumber ret = new UnifierSumNumber();
ret.setType(Short.class);
return ret;
}
};
/**
* Output float sum port.
*/
@OutputPortFieldAnnotation(optional = true)
public final transient DefaultOutputPort sumFloat = new DefaultOutputPort()
{
@Override
public Unifier getUnifier()
{
UnifierSumNumber ret = new UnifierSumNumber();
ret.setType(Float.class);
return ret;
}
};
/**
* Redis server output port.
*/
@OutputPortFieldAnnotation(optional = true)
public final transient DefaultOutputPort