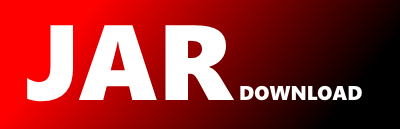
org.apache.aries.component.dsl.OSGi Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.aries.component.dsl;
import org.apache.aries.component.dsl.function.Function10;
import org.apache.aries.component.dsl.function.Function14;
import org.apache.aries.component.dsl.function.Function16;
import org.apache.aries.component.dsl.function.Function19;
import org.apache.aries.component.dsl.function.Function2;
import org.apache.aries.component.dsl.function.Function20;
import org.apache.aries.component.dsl.function.Function25;
import org.apache.aries.component.dsl.function.Function4;
import org.apache.aries.component.dsl.function.Function6;
import org.apache.aries.component.dsl.function.Function8;
import org.apache.aries.component.dsl.function.Function9;
import org.apache.aries.component.dsl.internal.ConfigurationOSGiImpl;
import org.apache.aries.component.dsl.internal.EffectsOSGi;
import org.apache.aries.component.dsl.internal.NothingOSGiImpl;
import org.apache.aries.component.dsl.internal.Pad;
import org.apache.aries.component.dsl.internal.ServiceReferenceOSGi;
import org.apache.aries.component.dsl.internal.ServiceRegistrationOSGiImpl;
import org.apache.aries.component.dsl.function.Function11;
import org.apache.aries.component.dsl.function.Function12;
import org.apache.aries.component.dsl.function.Function13;
import org.apache.aries.component.dsl.function.Function15;
import org.apache.aries.component.dsl.function.Function17;
import org.apache.aries.component.dsl.function.Function18;
import org.apache.aries.component.dsl.function.Function21;
import org.apache.aries.component.dsl.function.Function22;
import org.apache.aries.component.dsl.function.Function23;
import org.apache.aries.component.dsl.function.Function24;
import org.apache.aries.component.dsl.function.Function26;
import org.apache.aries.component.dsl.function.Function3;
import org.apache.aries.component.dsl.function.Function5;
import org.apache.aries.component.dsl.function.Function7;
import org.apache.aries.component.dsl.internal.BundleContextOSGiImpl;
import org.apache.aries.component.dsl.internal.BundleOSGi;
import org.apache.aries.component.dsl.internal.ChangeContextOSGiImpl;
import org.apache.aries.component.dsl.internal.ConcurrentDoublyLinkedList;
import org.apache.aries.component.dsl.internal.ConfigurationsOSGiImpl;
import org.apache.aries.component.dsl.internal.AllOSGi;
import org.apache.aries.component.dsl.internal.IgnoreImpl;
import org.apache.aries.component.dsl.internal.JustOSGiImpl;
import org.apache.aries.component.dsl.internal.OSGiImpl;
import org.apache.aries.component.dsl.internal.OnCloseOSGiImpl;
import org.osgi.framework.Bundle;
import org.osgi.framework.BundleContext;
import org.osgi.framework.ServiceFactory;
import org.osgi.framework.ServiceObjects;
import org.osgi.framework.ServiceRegistration;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.Dictionary;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.concurrent.atomic.AtomicInteger;
import java.util.concurrent.atomic.AtomicReference;
import java.util.function.BiFunction;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.function.Supplier;
import java.util.stream.Collectors;
/**
* @author Carlos Sierra Andrés
*/
public interface OSGi extends OSGiRunnable {
OSGiResult NOOP = () -> {};
@SafeVarargs
static OSGi all(OSGi ... programs) {
return new AllOSGi<>(programs);
}
static OSGi bundleContext() {
return new BundleContextOSGiImpl();
}
static OSGi bundles(int stateMask) {
return new BundleOSGi(stateMask);
}
static OSGi changeContext(
BundleContext bundleContext, OSGi program) {
return new ChangeContextOSGiImpl<>(program, bundleContext);
}
static OSGi combine(Function2 fun, OSGi a, OSGi b) {
return b.applyTo(a.applyTo(just(fun.curried())));
}
static OSGi combine(Function3 fun, OSGi a, OSGi b, OSGi c) {
return c.applyTo(combine((A aa, B bb) -> fun.curried().apply(aa).apply(bb), a, b));
}
static OSGi combine(Function4 fun, OSGi a, OSGi b, OSGi c, OSGi d) {
return d.applyTo(combine((A aa, B bb, C cc) -> fun.curried().apply(aa).apply(bb).apply(cc), a, b, c));
}
static OSGi combine(Function5 fun, OSGi a, OSGi b, OSGi c, OSGi d, OSGi e) {
return e.applyTo(combine((A aa, B bb, C cc, D dd) -> fun.curried().apply(aa).apply(bb).apply(cc).apply(dd), a, b, c, d));
}
static OSGi combine(Function6 fun, OSGi a, OSGi b, OSGi c, OSGi d, OSGi e, OSGi f) {
return f.applyTo(combine((A aa, B bb, C cc, D dd, E ee) -> fun.curried().apply(aa).apply(bb).apply(cc).apply(dd).apply(ee), a, b, c, d, e));
}
static OSGi combine(Function7 fun, OSGi a, OSGi b, OSGi c, OSGi d, OSGi e, OSGi f, OSGi g) {
return g.applyTo(combine((A aa, B bb, C cc, D dd, E ee, F ff) -> fun.curried().apply(aa).apply(bb).apply(cc).apply(dd).apply(ee).apply(ff), a, b, c, d, e, f));
}
static OSGi combine(Function8 fun, OSGi a, OSGi b, OSGi c, OSGi d, OSGi e, OSGi f, OSGi g, OSGi h) {
return h.applyTo(combine((A aa, B bb, C cc, D dd, E ee, F ff, G gg) -> fun.curried().apply(aa).apply(bb).apply(cc).apply(dd).apply(ee).apply(ff).apply(gg), a, b, c, d, e, f, g));
}
static OSGi combine(Function9 fun, OSGi a, OSGi b, OSGi c, OSGi d, OSGi e, OSGi f, OSGi g, OSGi h, OSGi i) {
return i.applyTo(combine((A aa, B bb, C cc, D dd, E ee, F ff, G gg, H hh) -> fun.curried().apply(aa).apply(bb).apply(cc).apply(dd).apply(ee).apply(ff).apply(gg).apply(hh), a, b, c, d, e, f, g, h));
}
static OSGi combine(Function10 fun, OSGi a, OSGi b, OSGi c, OSGi d, OSGi e, OSGi f, OSGi g, OSGi h, OSGi i, OSGi j) {
return j.applyTo(combine((A aa, B bb, C cc, D dd, E ee, F ff, G gg, H hh, I ii) -> fun.curried().apply(aa).apply(bb).apply(cc).apply(dd).apply(ee).apply(ff).apply(gg).apply(hh).apply(ii), a, b, c, d, e, f, g, h, i));
}
static OSGi combine(Function11 fun, OSGi a, OSGi b, OSGi c, OSGi d, OSGi e, OSGi f, OSGi g, OSGi h, OSGi i, OSGi j, OSGi k) {
return k.applyTo(combine((A aa, B bb, C cc, D dd, E ee, F ff, G gg, H hh, I ii, J jj) -> fun.curried().apply(aa).apply(bb).apply(cc).apply(dd).apply(ee).apply(ff).apply(gg).apply(hh).apply(ii).apply(jj), a, b, c, d, e, f, g, h, i, j));
}
static OSGi combine(Function12 fun, OSGi a, OSGi b, OSGi c, OSGi d, OSGi e, OSGi f, OSGi g, OSGi h, OSGi i, OSGi j, OSGi k, OSGi l) {
return l.applyTo(combine((A aa, B bb, C cc, D dd, E ee, F ff, G gg, H hh, I ii, J jj, K kk) -> fun.curried().apply(aa).apply(bb).apply(cc).apply(dd).apply(ee).apply(ff).apply(gg).apply(hh).apply(ii).apply(jj).apply(kk), a, b, c, d, e, f, g, h, i, j, k));
}
static OSGi combine(Function13 fun, OSGi a, OSGi b, OSGi c, OSGi d, OSGi e, OSGi f, OSGi g, OSGi h, OSGi i, OSGi j, OSGi k, OSGi l, OSGi m) {
return m.applyTo(combine((A aa, B bb, C cc, D dd, E ee, F ff, G gg, H hh, I ii, J jj, K kk, L ll) -> fun.curried().apply(aa).apply(bb).apply(cc).apply(dd).apply(ee).apply(ff).apply(gg).apply(hh).apply(ii).apply(jj).apply(kk).apply(ll), a, b, c, d, e, f, g, h, i, j, k, l));
}
static OSGi combine(Function14 fun, OSGi a, OSGi b, OSGi c, OSGi d, OSGi e, OSGi f, OSGi g, OSGi h, OSGi i, OSGi j, OSGi k, OSGi l, OSGi m, OSGi n) {
return n.applyTo(combine((A aa, B bb, C cc, D dd, E ee, F ff, G gg, H hh, I ii, J jj, K kk, L ll, M mm) -> fun.curried().apply(aa).apply(bb).apply(cc).apply(dd).apply(ee).apply(ff).apply(gg).apply(hh).apply(ii).apply(jj).apply(kk).apply(ll).apply(mm), a, b, c, d, e, f, g, h, i, j, k, l, m));
}
static OSGi combine(Function15 fun, OSGi a, OSGi b, OSGi c, OSGi d, OSGi e, OSGi f, OSGi g, OSGi h, OSGi i, OSGi j, OSGi k, OSGi l, OSGi m, OSGi n, OSGi o) {
return o.applyTo(combine((A aa, B bb, C cc, D dd, E ee, F ff, G gg, H hh, I ii, J jj, K kk, L ll, M mm, N nn) -> fun.curried().apply(aa).apply(bb).apply(cc).apply(dd).apply(ee).apply(ff).apply(gg).apply(hh).apply(ii).apply(jj).apply(kk).apply(ll).apply(mm).apply(nn), a, b, c, d, e, f, g, h, i, j, k, l, m, n));
}
static OSGi combine(Function16 fun, OSGi a, OSGi b, OSGi c, OSGi d, OSGi e, OSGi f, OSGi g, OSGi h, OSGi i, OSGi j, OSGi k, OSGi l, OSGi m, OSGi n, OSGi o, OSGi p) {
return p.applyTo(combine((A aa, B bb, C cc, D dd, E ee, F ff, G gg, H hh, I ii, J jj, K kk, L ll, M mm, N nn, O oo) -> fun.curried().apply(aa).apply(bb).apply(cc).apply(dd).apply(ee).apply(ff).apply(gg).apply(hh).apply(ii).apply(jj).apply(kk).apply(ll).apply(mm).apply(nn).apply(oo), a, b, c, d, e, f, g, h, i, j, k, l, m, n, o));
}
static OSGi combine(Function17 fun, OSGi a, OSGi b, OSGi c, OSGi d, OSGi e, OSGi f, OSGi g, OSGi h, OSGi i, OSGi j, OSGi k, OSGi l, OSGi m, OSGi n, OSGi o, OSGi p, OSGi q) {
return q.applyTo(combine((A aa, B bb, C cc, D dd, E ee, F ff, G gg, H hh, I ii, J jj, K kk, L ll, M mm, N nn, O oo, P pp) -> fun.curried().apply(aa).apply(bb).apply(cc).apply(dd).apply(ee).apply(ff).apply(gg).apply(hh).apply(ii).apply(jj).apply(kk).apply(ll).apply(mm).apply(nn).apply(oo).apply(pp), a, b, c, d, e, f, g, h, i, j, k, l, m, n, o, p));
}
static OSGi combine(Function18 fun, OSGi a, OSGi b, OSGi c, OSGi d, OSGi e, OSGi f, OSGi g, OSGi h, OSGi i, OSGi j, OSGi k, OSGi l, OSGi m, OSGi n, OSGi o, OSGi p, OSGi q, OSGi r) {
return r.applyTo(combine((A aa, B bb, C cc, D dd, E ee, F ff, G gg, H hh, I ii, J jj, K kk, L ll, M mm, N nn, O oo, P pp, Q qq) -> fun.curried().apply(aa).apply(bb).apply(cc).apply(dd).apply(ee).apply(ff).apply(gg).apply(hh).apply(ii).apply(jj).apply(kk).apply(ll).apply(mm).apply(nn).apply(oo).apply(pp).apply(qq), a, b, c, d, e, f, g, h, i, j, k, l, m, n, o, p, q));
}
static OSGi combine(Function19 fun, OSGi a, OSGi b, OSGi c, OSGi d, OSGi e, OSGi f, OSGi g, OSGi h, OSGi i, OSGi j, OSGi k, OSGi l, OSGi m, OSGi n, OSGi o, OSGi p, OSGi q, OSGi r, OSGi s) {
return s.applyTo(combine((A aa, B bb, C cc, D dd, E ee, F ff, G gg, H hh, I ii, J jj, K kk, L ll, M mm, N nn, O oo, P pp, Q qq, R rr) -> fun.curried().apply(aa).apply(bb).apply(cc).apply(dd).apply(ee).apply(ff).apply(gg).apply(hh).apply(ii).apply(jj).apply(kk).apply(ll).apply(mm).apply(nn).apply(oo).apply(pp).apply(qq).apply(rr), a, b, c, d, e, f, g, h, i, j, k, l, m, n, o, p, q, r));
}
static OSGi combine(Function20 fun, OSGi a, OSGi b, OSGi c, OSGi d, OSGi e, OSGi f, OSGi g, OSGi h, OSGi i, OSGi j, OSGi k, OSGi l, OSGi m, OSGi