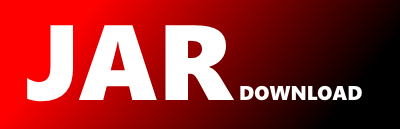
org.apache.asterix.optimizer.rules.am.InvertedIndexJobGenParams Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.asterix.optimizer.rules.am;
import java.util.ArrayList;
import java.util.List;
import org.apache.commons.lang3.mutable.Mutable;
import org.apache.commons.lang3.mutable.MutableObject;
import org.apache.asterix.common.config.DatasetConfig.IndexType;
import org.apache.asterix.om.constants.AsterixConstantValue;
import org.apache.asterix.om.types.ATypeTag;
import org.apache.asterix.optimizer.rules.am.InvertedIndexAccessMethod.SearchModifierType;
import org.apache.hyracks.algebricks.core.algebra.base.ILogicalExpression;
import org.apache.hyracks.algebricks.core.algebra.base.LogicalVariable;
import org.apache.hyracks.algebricks.core.algebra.expressions.ConstantExpression;
import org.apache.hyracks.algebricks.core.algebra.expressions.IAlgebricksConstantValue;
/**
* Helper class for reading and writing job-gen parameters for RTree access methods to
* and from a list of function arguments, typically of an unnest-map.
*/
public class InvertedIndexJobGenParams extends AccessMethodJobGenParams {
protected SearchModifierType searchModifierType;
protected IAlgebricksConstantValue similarityThreshold;
protected ATypeTag searchKeyType;
protected List keyVarList;
protected List nonKeyVarList;
public InvertedIndexJobGenParams() {
}
public InvertedIndexJobGenParams(String indexName, IndexType indexType, String dataverseName, String datasetName,
boolean retainInput, boolean retainNull, boolean requiresBroadcast) {
super(indexName, indexType, dataverseName, datasetName, retainInput, retainNull, requiresBroadcast);
}
public void setSearchModifierType(SearchModifierType searchModifierType) {
this.searchModifierType = searchModifierType;
}
public void setSimilarityThreshold(IAlgebricksConstantValue similarityThreshold) {
this.similarityThreshold = similarityThreshold;
}
public void setSearchKeyType(ATypeTag searchKeyType) {
this.searchKeyType = searchKeyType;
}
public void setKeyVarList(List keyVarList) {
this.keyVarList = keyVarList;
}
public void writeToFuncArgs(List> funcArgs) {
super.writeToFuncArgs(funcArgs);
// Write search modifier type.
funcArgs.add(new MutableObject(AccessMethodUtils.createInt32Constant(searchModifierType
.ordinal())));
// Write similarity threshold.
funcArgs.add(new MutableObject(new ConstantExpression(similarityThreshold)));
// Write search key type.
funcArgs.add(new MutableObject(AccessMethodUtils.createInt32Constant(searchKeyType
.ordinal())));
// Write key var list.
writeVarList(keyVarList, funcArgs);
// Write non-key var list.
if (nonKeyVarList != null) {
writeVarList(nonKeyVarList, funcArgs);
}
}
public void readFromFuncArgs(List> funcArgs) {
super.readFromFuncArgs(funcArgs);
int index = super.getNumParams();
// Read search modifier type.
int searchModifierOrdinal = AccessMethodUtils.getInt32Constant(funcArgs.get(index));
searchModifierType = SearchModifierType.values()[searchModifierOrdinal];
// Read similarity threshold. Concrete type depends on search modifier.
similarityThreshold = ((AsterixConstantValue) ((ConstantExpression) funcArgs.get(index + 1).getValue())
.getValue());
// Read type of search key.
int typeTagOrdinal = AccessMethodUtils.getInt32Constant(funcArgs.get(index + 2));
searchKeyType = ATypeTag.values()[typeTagOrdinal];
// Read key var list.
keyVarList = new ArrayList();
readVarList(funcArgs, index + 3, keyVarList);
// TODO: We could possibly simplify things if we did read the non-key var list here.
// We don't need to read the non-key var list.
nonKeyVarList = null;
}
public SearchModifierType getSearchModifierType() {
return searchModifierType;
}
public IAlgebricksConstantValue getSimilarityThreshold() {
return similarityThreshold;
}
public ATypeTag getSearchKeyType() {
return searchKeyType;
}
public List getKeyVarList() {
return keyVarList;
}
public List getNonKeyVarList() {
return nonKeyVarList;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy