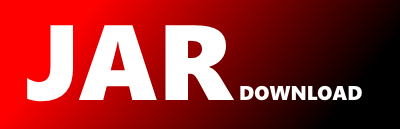
org.apache.asterix.event.schema.cluster.Cluster Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.7
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2016.02.10 at 03:27:57 PM PST
//
package org.apache.asterix.event.schema.cluster;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{cluster}instance_name"/>
* <element ref="{cluster}cluster_name"/>
* <element ref="{cluster}username"/>
* <element ref="{cluster}env" minOccurs="0"/>
* <element ref="{cluster}java_home" minOccurs="0"/>
* <element ref="{cluster}log_dir" minOccurs="0"/>
* <element ref="{cluster}txn_log_dir" minOccurs="0"/>
* <element ref="{cluster}store" minOccurs="0"/>
* <element ref="{cluster}iodevices" minOccurs="0"/>
* <element ref="{cluster}working_dir"/>
* <element ref="{cluster}metadata_node"/>
* <element ref="{cluster}master_node"/>
* <element ref="{cluster}node" maxOccurs="unbounded"/>
* <element ref="{cluster}substitute_nodes"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"instanceName",
"clusterName",
"username",
"env",
"javaHome",
"logDir",
"txnLogDir",
"store",
"iodevices",
"workingDir",
"metadataNode",
"masterNode",
"node",
"substituteNodes"
})
@XmlRootElement(name = "cluster")
public class Cluster implements Serializable
{
private final static long serialVersionUID = 1L;
@XmlElement(name = "instance_name", required = true)
protected String instanceName;
@XmlElement(name = "cluster_name", required = true)
protected String clusterName;
@XmlElement(required = true)
protected String username;
protected Env env;
@XmlElement(name = "java_home")
protected String javaHome;
@XmlElement(name = "log_dir")
protected String logDir;
@XmlElement(name = "txn_log_dir")
protected String txnLogDir;
protected String store;
protected String iodevices;
@XmlElement(name = "working_dir", required = true)
protected WorkingDir workingDir;
@XmlElement(name = "metadata_node", required = true)
protected String metadataNode;
@XmlElement(name = "master_node", required = true)
protected MasterNode masterNode;
@XmlElement(required = true)
protected List node;
@XmlElement(name = "substitute_nodes", required = true)
protected SubstituteNodes substituteNodes;
/**
* Default no-arg constructor
*
*/
public Cluster() {
super();
}
/**
* Fully-initialising value constructor
*
*/
public Cluster(final String instanceName, final String clusterName, final String username, final Env env, final String javaHome, final String logDir, final String txnLogDir, final String store, final String iodevices, final WorkingDir workingDir, final String metadataNode, final MasterNode masterNode, final List node, final SubstituteNodes substituteNodes) {
this.instanceName = instanceName;
this.clusterName = clusterName;
this.username = username;
this.env = env;
this.javaHome = javaHome;
this.logDir = logDir;
this.txnLogDir = txnLogDir;
this.store = store;
this.iodevices = iodevices;
this.workingDir = workingDir;
this.metadataNode = metadataNode;
this.masterNode = masterNode;
this.node = node;
this.substituteNodes = substituteNodes;
}
/**
* Gets the value of the instanceName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getInstanceName() {
return instanceName;
}
/**
* Sets the value of the instanceName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setInstanceName(String value) {
this.instanceName = value;
}
/**
* Gets the value of the clusterName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getClusterName() {
return clusterName;
}
/**
* Sets the value of the clusterName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setClusterName(String value) {
this.clusterName = value;
}
/**
* Gets the value of the username property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getUsername() {
return username;
}
/**
* Sets the value of the username property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setUsername(String value) {
this.username = value;
}
/**
* Gets the value of the env property.
*
* @return
* possible object is
* {@link Env }
*
*/
public Env getEnv() {
return env;
}
/**
* Sets the value of the env property.
*
* @param value
* allowed object is
* {@link Env }
*
*/
public void setEnv(Env value) {
this.env = value;
}
/**
* Gets the value of the javaHome property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getJavaHome() {
return javaHome;
}
/**
* Sets the value of the javaHome property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setJavaHome(String value) {
this.javaHome = value;
}
/**
* Gets the value of the logDir property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLogDir() {
return logDir;
}
/**
* Sets the value of the logDir property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLogDir(String value) {
this.logDir = value;
}
/**
* Gets the value of the txnLogDir property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTxnLogDir() {
return txnLogDir;
}
/**
* Sets the value of the txnLogDir property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTxnLogDir(String value) {
this.txnLogDir = value;
}
/**
* Gets the value of the store property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getStore() {
return store;
}
/**
* Sets the value of the store property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setStore(String value) {
this.store = value;
}
/**
* Gets the value of the iodevices property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getIodevices() {
return iodevices;
}
/**
* Sets the value of the iodevices property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setIodevices(String value) {
this.iodevices = value;
}
/**
* Gets the value of the workingDir property.
*
* @return
* possible object is
* {@link WorkingDir }
*
*/
public WorkingDir getWorkingDir() {
return workingDir;
}
/**
* Sets the value of the workingDir property.
*
* @param value
* allowed object is
* {@link WorkingDir }
*
*/
public void setWorkingDir(WorkingDir value) {
this.workingDir = value;
}
/**
* Gets the value of the metadataNode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMetadataNode() {
return metadataNode;
}
/**
* Sets the value of the metadataNode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMetadataNode(String value) {
this.metadataNode = value;
}
/**
* Gets the value of the masterNode property.
*
* @return
* possible object is
* {@link MasterNode }
*
*/
public MasterNode getMasterNode() {
return masterNode;
}
/**
* Sets the value of the masterNode property.
*
* @param value
* allowed object is
* {@link MasterNode }
*
*/
public void setMasterNode(MasterNode value) {
this.masterNode = value;
}
/**
* Gets the value of the node property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the node property.
*
*
* For example, to add a new item, do as follows:
*
* getNode().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Node }
*
*
*/
public List getNode() {
if (node == null) {
node = new ArrayList();
}
return this.node;
}
/**
* Gets the value of the substituteNodes property.
*
* @return
* possible object is
* {@link SubstituteNodes }
*
*/
public SubstituteNodes getSubstituteNodes() {
return substituteNodes;
}
/**
* Sets the value of the substituteNodes property.
*
* @param value
* allowed object is
* {@link SubstituteNodes }
*
*/
public void setSubstituteNodes(SubstituteNodes value) {
this.substituteNodes = value;
}
public void setNode(List value) {
this.node = null;
List draftl = this.getNode();
draftl.addAll(value);
}
}