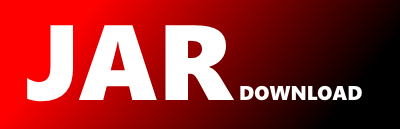
org.apache.axis2.wsdl.template.c.ServiceSkeleton.xsl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of axis2-codegen Show documentation
Show all versions of axis2-codegen Show documentation
Axis2 Code Generation module
/**
* .c
*
* This file was auto-generated from WSDL for " " service
* by the Apache Axis2 version: #axisVersion# #today#
*
*/
#include " .h"
#include <axis2_svc_skeleton.h>
#include <stdio.h>
#include <axis2_svc.h>
#ifdef __cplusplus
extern "C" {
#endif
/**
* creating a custom structure to wrap the axis2_svc_skeleton class
*/
typedef struct {
axis2_svc_skeleton_t svc_skeleton;
/* union to keep all the exception objects */
union {
__fault _fault;
} fault;
} _t;
/**
* functions prototypes
*/
/* On fault, handle the fault */
axiom_node_t* AXIS2_CALL
_on_fault(axis2_svc_skeleton_t *svc_skeleton,
const axutil_env_t *env, axiom_node_t *node);
/* Free the service */
int AXIS2_CALL
_free(axis2_svc_skeleton_t *svc_skeleton,
const axutil_env_t *env);
/* This method invokes the right service method */
axiom_node_t* AXIS2_CALL
_invoke(axis2_svc_skeleton_t *svc_skeleton,
const axutil_env_t *env,
axiom_node_t *node,
axis2_msg_ctx_t *msg_ctx);
/* Initializing the environment */
int AXIS2_CALL
_init(axis2_svc_skeleton_t *svc_skeleton,
const axutil_env_t *env);
/* Create the service */
axis2_svc_skeleton_t* AXIS2_CALL
_create(const axutil_env_t *env);
static const axis2_svc_skeleton_ops_t _svc_skeleton_ops_var = {
_init,
_invoke,
_on_fault,
_free
};
/**
* Following block distinguish the exposed part of the dll.
* create the instance
*/
AXIS2_EXTERN int
axis2_get_instance(struct axis2_svc_skeleton **inst,
const axutil_env_t *env);
AXIS2_EXTERN int
axis2_remove_instance(axis2_svc_skeleton_t *inst,
const axutil_env_t *env);
/**
* function to free any soap input headers
*/
void
axis2_skel_op_ _ _free_input_headers(const axutil_env_t *env, ,
_
);
void
axis2_skel_op_ _ _free_output_headers(const axutil_env_t *env, ,
_
);
/*
* Create the response soap envelope when output headers are to be set..
*/
axiom_soap_envelope_t* AXIS2_CALL
_create_response_envelope(const axutil_env_t *env,
axis2_msg_ctx_t *in_msg_ctx,
axis2_msg_ctx_t *msg_ctx,
axiom_node_t *body_content_node);
#ifdef __cplusplus
}
#endif
/**
* Implementations for the functions
*/
axis2_svc_skeleton_t* AXIS2_CALL
_create(const axutil_env_t *env)
{
_t *svc_skeleton_wrapper = NULL;
axis2_svc_skeleton_t *svc_skeleton = NULL;
/* Allocate memory for the structs */
svc_skeleton_wrapper = ( _t *)AXIS2_MALLOC(env->allocator,
sizeof( _t));
svc_skeleton = (axis2_svc_skeleton_t*)svc_skeleton_wrapper;
svc_skeleton->ops = & _svc_skeleton_ops_var;
return svc_skeleton;
}
int AXIS2_CALL
_init(axis2_svc_skeleton_t *svc_skeleton,
const axutil_env_t *env)
{
/* Nothing special in initialization */
return AXIS2_SUCCESS;
}
int AXIS2_CALL
_free(axis2_svc_skeleton_t *svc_skeleton,
const axutil_env_t *env)
{
/* Free the service skeleton */
if (svc_skeleton)
{
AXIS2_FREE(env->allocator, svc_skeleton);
svc_skeleton = NULL;
}
return AXIS2_SUCCESS;
}
/**
* function to free soap input headers
*/
void
axis2_skel_op_ _ _free_input_headers(const axutil_env_t *env, ,
_
)
{
_
if( )
{
_free( , env);
}
/* we don't have anything to free on */
}
/**
* function to free any soap output headers
*/
void
axis2_skel_op_ _ _free_output_headers(const axutil_env_t *env, ,
_
)
{
_
if( )
{
_free( , env);
}
/* we don't have anything to free on */
}
/*
* Create the response soap envelope when output headers are to be set..
*/
axiom_soap_envelope_t* AXIS2_CALL
_create_response_envelope(const axutil_env_t *env,
axis2_msg_ctx_t *in_msg_ctx,
axis2_msg_ctx_t *msg_ctx,
axiom_node_t *body_content_node)
{
const axis2_char_t *soap_ns = AXIOM_SOAP12_SOAP_ENVELOPE_NAMESPACE_URI;
int soap_version = AXIOM_SOAP12;
axiom_namespace_t *env_ns = NULL;
axiom_soap_envelope_t *default_envelope = NULL;
axiom_soap_header_t *out_header = NULL;
axiom_soap_body_t *out_body = NULL;
axiom_node_t *out_node = NULL;
if (in_msg_ctx && axis2_msg_ctx_get_is_soap_11(in_msg_ctx, env))
{
soap_ns = AXIOM_SOAP11_SOAP_ENVELOPE_NAMESPACE_URI; /* default is 1.2 */
soap_version = AXIOM_SOAP11;
}
/* create the soap envelope here */
env_ns = axiom_namespace_create(env, soap_ns, "soapenv");
if (!env_ns)
{
return NULL;
}
default_envelope = axiom_soap_envelope_create(env, env_ns);
if (!default_envelope)
{
axiom_namespace_free(env_ns, env);
return NULL;
}
out_header = axiom_soap_header_create_with_parent(env, default_envelope);
if (!out_header)
{
axiom_soap_envelope_free(default_envelope, env);
axiom_namespace_free(env_ns, env);
return NULL;
}
out_body = axiom_soap_body_create_with_parent(env, default_envelope);
if (!out_body)
{
axiom_soap_body_free(out_body, env);
axiom_soap_envelope_free(default_envelope, env);
axiom_namespace_free(env_ns, env);
return NULL;
}
out_node = axiom_soap_body_get_base_node(out_body, env);
if (!out_node)
{
axiom_soap_body_free(out_body, env);
axiom_soap_envelope_free(default_envelope, env);
axiom_namespace_free(env_ns, env);
return NULL;
}
if (body_content_node)
{
axiom_node_add_child(out_node, env, body_content_node);
}
if(axis2_msg_ctx_set_soap_envelope(msg_ctx, env, default_envelope) == AXIS2_FAILURE)
{
axiom_soap_body_free(out_body, env);
axiom_soap_envelope_free(default_envelope, env);
axiom_namespace_free(env_ns, env);
if (body_content_node)
{
axiom_node_free_tree(body_content_node, env);
}
return NULL;
}
return default_envelope;
}
/*
* This method invokes the right service method
*/
axiom_node_t* AXIS2_CALL
_invoke(axis2_svc_skeleton_t *svc_skeleton,
const axutil_env_t *env,
axiom_node_t *content_node,
axis2_msg_ctx_t *msg_ctx)
{
/* depending on the function name invoke the
* corresponding method
*/
axis2_op_ctx_t *operation_ctx = NULL;
axis2_op_t *operation = NULL;
axutil_qname_t *op_qname = NULL;
axis2_char_t *op_name = NULL;
axis2_msg_ctx_t *in_msg_ctx = NULL;
axiom_soap_envelope_t *req_soap_env = NULL;
axiom_soap_header_t *req_soap_header = NULL;
axiom_soap_envelope_t *res_soap_env = NULL;
axiom_soap_header_t *res_soap_header = NULL;
axiom_node_t *ret_node = NULL;
axiom_node_t *input_header = NULL;
axiom_node_t *output_header = NULL;
axiom_node_t *header_base_node = NULL;
_t *svc_skeleton_wrapper = NULL;
axis2_status_t
ret_val ;
input_val ;
_ = NULL;
_ = NULL;
svc_skeleton_wrapper = ( _t*)svc_skeleton;
operation_ctx = axis2_msg_ctx_get_op_ctx(msg_ctx, env);
operation = axis2_op_ctx_get_op(operation_ctx, env);
op_qname = (axutil_qname_t *)axis2_op_get_qname(operation, env);
op_name = axutil_qname_get_localpart(op_qname, env);
if (op_name)
{
if(operation_ctx)
{
in_msg_ctx = axis2_op_ctx_get_msg_ctx(operation_ctx, env, AXIS2_WSDL_MESSAGE_LABEL_IN);
}
if(in_msg_ctx)
{
req_soap_env = axis2_msg_ctx_get_soap_envelope(in_msg_ctx, env);
}
if(!req_soap_env)
{
AXIS2_LOG_ERROR( env->log, AXIS2_LOG_SI, "request evelope is NULL");
return NULL;
}
req_soap_header = axiom_soap_envelope_get_header(req_soap_env, env);
if(req_soap_header)
{
header_base_node = axiom_soap_header_get_base_node(req_soap_header, env);
}
if ( axutil_strcmp(op_name, " ") == 0 )
{
input_val =
_create( env);
if( AXIS2_FAILURE == _deserialize(input_val , env, &content_node, NULL, AXIS2_FALSE))
{
_free(input_val , env);
AXIS2_ERROR_SET(env->error, AXIS2_ERROR_DATA_ELEMENT_IS_NULL, AXIS2_FAILURE);
AXIS2_LOG_ERROR( env->log, AXIS2_LOG_SI, "NULL returnted from the _deserialize: "
"This should be due to an invalid XML");
return NULL;
}
content_node;
input_header = axiom_node_get_first_child(header_base_node, env);
while(input_header && axiom_node_get_node_type(input_header, env) != AXIOM_ELEMENT)
{
input_header = axiom_node_get_next_sibling(input_header, env);
}
input_header = axiom_node_get_next_sibling(input_header, env);
while(input_header && axiom_node_get_node_type(input_header, env) != AXIOM_ELEMENT)
{
input_header = axiom_node_get_next_sibling(input_header, env);
}
_
if( NULL == input_header)
{
AXIS2_LOG_ERROR( env->log, AXIS2_LOG_SI, "Response header is NULL");
/* you can't have a response header NULL, just free things and exit */
axis2_skel_op_ _ _free_input_headers(env, ,
_
);
_free(input_val , env);
return NULL;
}
= _create(env);
if( _deserialize( , env, &input_header, NULL, AXIS2_FALSE ) == AXIS2_FAILURE)
{
if( != NULL)
{
_free( , env);
}
AXIS2_LOG_ERROR( env->log, AXIS2_LOG_SI, "NULL returnted from the _deserialize: "
"This should be due to an invalid input header");
axis2_skel_op_ _ _free_input_headers(env, ,
_
);
_free(input_val , env);
return NULL;
}
= input_header;
,
_get_property (
_get_property1(input_val, env)
, env)
,
_get_property (input_val, env)
{
ret_unwrapped = _(env
, msg_ctx
,
&_
,
( __fault* )&(svc_skeleton_wrapper->fault) );
ret_val = _create_with_values(env,
_create_with_values(env, ret_unwrapped));
ret_val = _create_with_values(env, ret_unwrapped);
if(ret_unwrapped)
{
/* we are freeing this for the user */
AXIS2_FREE(env->allocator, ret_unwrapped);
}
}
,
input_val ,
_
ret_val = _(env
, msg_ctx
,
&_
,
( __fault* )&(svc_skeleton_wrapper->fault) );
if ( NULL == ret_val )
{
_free(input_val , env);
axis2_skel_op_ _ _free_input_headers(env, ,
_
);
axis2_skel_op_ _ _free_output_headers(env, ,
_
);
return NULL;
}
ret_node =
_serialize(ret_val , env, NULL, NULL, AXIS2_TRUE, NULL, NULL);
_free(ret_val , env);
_free(input_val , env);
ret_val ;
res_soap_env = axis2_msg_ctx_get_response_soap_envelope(msg_ctx, env);
if(!res_soap_env)
{
res_soap_env = _create_response_envelope(env, in_msg_ctx, msg_ctx, ret_node);
}
if(!res_soap_env)
{
AXIS2_LOG_ERROR( env->log, AXIS2_LOG_SI, "response evelope is NULL");
axis2_skel_op_ _ _free_input_headers(env, ,
_
);
axis2_skel_op_ _ _free_output_headers(env, ,
_
);
return NULL;
}
res_soap_header = axiom_soap_envelope_get_header(res_soap_env, env);
if(res_soap_header)
{
header_base_node = axiom_soap_header_get_base_node(res_soap_header, env);
}
if(!header_base_node)
{
axis2_skel_op_ _ _free_input_headers(env, ,
_
);
axis2_skel_op_ _ _free_output_headers(env, ,
_
);
AXIS2_LOG_ERROR( env->log, AXIS2_LOG_SI, "Required response header is NULL");
return NULL;
}
output_header = _serialize(_ , env, NULL, NULL, AXIS2_TRUE, NULL, NULL);
output_header = _ ;
axiom_node_add_child(header_base_node, env, output_header);
axis2_skel_op_ _ _free_input_headers(env, ,
_
);
axis2_skel_op_ _ _free_output_headers(env, ,
_
);
return ret_node;
if( AXIS2_FAILURE == ret_val )
{
AXIS2_LOG_ERROR(env->log, AXIS2_LOG_SI, "NULL returnted from the business logic from ");
}
_free(input_val , env);
axis2_skel_op_ _ _free_input_headers(env, ,
_
);
return NULL;
/* since this has no output params it just returns NULL */
return NULL;
}
}
AXIS2_LOG_ERROR(env->log, AXIS2_LOG_SI, " service ERROR: invalid OM parameters in request\n");
return NULL;
}
axiom_node_t* AXIS2_CALL
_on_fault(axis2_svc_skeleton_t *svc_skeleton,
const axutil_env_t *env, axiom_node_t *node)
{
axiom_node_t *error_node = NULL;
axiom_element_t *error_ele = NULL;
axutil_error_codes_t error_code;
_t *svc_skeleton_wrapper = NULL;
svc_skeleton_wrapper = ( _t*)svc_skeleton;
error_code = env->error->error_number;
if(error_code <= _ERROR_NONE ||
error_code >= _ERROR_LAST )
{
error_ele = axiom_element_create(env, node, "fault", NULL,
&error_node);
axiom_element_set_text(error_ele, env, " failed",
error_node);
}
_ _FAULT_
else if(error_code == )
{
/* found which error code */
adb_obj = NULL;
adb_obj = ( )svc_skeleton_wrapper->fault. _fault. ;
if(adb_obj)
{
error_node = _serialize(adb_obj, env, NULL, NULL, AXIS2_TRUE, NULL, NULL);
_free(adb_obj, env);
svc_skeleton_wrapper->fault. _fault. = NULL;
}
}
return error_node;
}
/**
* Following block distinguish the exposed part of the dll.
*/
AXIS2_EXTERN int
axis2_get_instance(struct axis2_svc_skeleton **inst,
const axutil_env_t *env)
{
*inst = _create(env);
if(!(*inst))
{
return AXIS2_FAILURE;
}
return AXIS2_SUCCESS;
}
AXIS2_EXTERN int
axis2_remove_instance(axis2_svc_skeleton_t *inst,
const axutil_env_t *env)
{
axis2_status_t status = AXIS2_FAILURE;
if (inst)
{
status = AXIS2_SVC_SKELETON_FREE(inst, env);
}
return status;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy