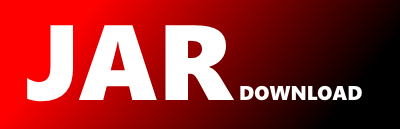
org.apache.axis2.jaxws.description.impl.ParameterDescriptionImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of axis2-metadata Show documentation
Show all versions of axis2-metadata Show documentation
JSR-181 and JSR-224 Annotations Processing
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.axis2.jaxws.description.impl;
import org.apache.axis2.jaxws.description.AttachmentDescription;
import org.apache.axis2.jaxws.description.EndpointDescriptionJava;
import org.apache.axis2.jaxws.description.OperationDescription;
import org.apache.axis2.jaxws.description.ParameterDescription;
import org.apache.axis2.jaxws.description.ParameterDescriptionJava;
import org.apache.axis2.jaxws.description.ParameterDescriptionWSDL;
import org.apache.axis2.jaxws.description.builder.ParameterDescriptionComposite;
import org.apache.axis2.jaxws.description.builder.converter.ConverterUtils;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import javax.jws.WebParam;
import javax.jws.soap.SOAPBinding;
import javax.xml.ws.Holder;
import java.lang.annotation.Annotation;
import java.lang.reflect.Array;
import java.lang.reflect.GenericArrayType;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
/** @see ../ParameterDescription */
class ParameterDescriptionImpl
implements ParameterDescription, ParameterDescriptionJava, ParameterDescriptionWSDL {
private static final Log log = LogFactory.getLog(ParameterDescriptionImpl.class);
private OperationDescription parentOperationDescription;
// The Class representing the parameter. Note that for a Generic, including the JAX-WS Holder Generic,
// this represents the raw type of the Generic (e.g. List for List or Holder for Holder).
private Class parameterType;
// For the JAX-WS Generic Holder (e.g. Holder), this will be the actual type argument (e.g. Foo). For
// any other parameter (including other Generics), this will be null.
// Note that since JAX-WS Holder only supports a single actual type T (not multiple types such as )
private Class parameterHolderActualType;
// 0-based number of the parameter in the argument list
private int parameterNumber = -1;
// The Parameter Description Composite used to build the ParameterDescription
private ParameterDescriptionComposite paramDescComposite;
// ANNOTATION: @WebMethod
private WebParam webParamAnnotation;
private String webParamName;
private String webParamPartName;
public static final String WebParam_TargetNamespace_DEFAULT = "";
private String webParamTargetNamespace;
private WebParam.Mode webParamMode;
public static final Boolean WebParam_Header_DEFAULT = new Boolean(false);
private Boolean webParamHeader;
// Attachment Description information
private boolean _setAttachmentDesc = false;
private AttachmentDescription attachmentDesc = null;
// This boolean indicates whether or not there was an @XMLList on the parameter
private boolean isListType = false;
ParameterDescriptionImpl(int parameterNumber, Class parameterType, Type parameterGenericType,
Annotation[] parameterAnnotations, OperationDescription parent) {
this.parameterNumber = parameterNumber;
this.parentOperationDescription = parent;
this.parameterType = parameterType;
// The Type argument could be a Type (if the parameter is a Paramaterized Generic) or
// just a Class (if it is not). If it JAX-WS Holder parameterized type, then get the
// actual parameter type and hang on to that, too.
if (ParameterizedType.class.isInstance(parameterGenericType)) {
this.parameterHolderActualType =
getGenericParameterActualType((ParameterizedType)parameterGenericType);
}
findWebParamAnnotation(parameterAnnotations);
this.isListType = ConverterUtils.hasXmlListAnnotation(parameterAnnotations);
}
ParameterDescriptionImpl(int parameterNumber, ParameterDescriptionComposite pdc,
OperationDescription parent) {
this.paramDescComposite = pdc;
this.parameterNumber = parameterNumber;
this.parentOperationDescription = parent;
webParamAnnotation = pdc.getWebParamAnnot();
this.isListType = pdc.isListType();
//TODO: Need to build the schema map. Need to add logic to add this parameter
// to the schema map.
}
/*
* This grabs the WebParam annotation from the list of annotations for this parameter
* This should be DEPRECATED once DBC processing is complete.
*/
private void findWebParamAnnotation(Annotation[] annotations) {
for (Annotation checkAnnotation : annotations) {
// REVIEW: This may not work with the MDQInput. From the java.lang.annotation.Annotation interface
// javadoc: "Note that an interface that manually extends this one does not define an annotation type."
if (checkAnnotation.annotationType() == WebParam.class) {
webParamAnnotation = (WebParam)checkAnnotation;
}
}
}
public OperationDescription getOperationDescription() {
return parentOperationDescription;
}
/**
* Returns the class associated with the parameter. Note that for the JAX-WS Holder type,
* you can use getParameterActualType() to get the class associated with T.
*/
public Class getParameterType() {
if (parameterType == null && paramDescComposite != null) {
parameterType = paramDescComposite.getParameterTypeClass();
}
return parameterType;
}
/**
* For a non-Holder type, returns the parameter class. For a Holder type, returns the class
* of T.
*
* @return
*/
public Class getParameterActualType() {
if (parameterHolderActualType == null && paramDescComposite != null &&
paramDescComposite.isHolderType()) {
parameterHolderActualType = paramDescComposite.getHolderActualTypeClass();
return parameterHolderActualType;
} else if (parameterHolderActualType != null) {
return parameterHolderActualType;
} else {
if (paramDescComposite != null && parameterType == null) {
parameterType = paramDescComposite.getParameterTypeClass();
}
return parameterType;
}
}
/**
* TEMPORARY METHOD! For a JAX-WS Holder this returns the class associated with . For a
* Holder>, it returns the class associated with Generic. If the type is not a
* JAX-WS Holder, return a null.
*
* This method SHOULD BE REMOVED when the description layer is refactored to use only DBC and
* not Java reflection directly.
*
* @param parameterGenericType
* @return
*/
// TODO: Remove this method when code refactored to only use DBC.
private Class getGenericParameterActualType(ParameterizedType parameterGenericType) {
Class returnClass = null;
// If this is a JAX-WS Holder type, then get the actual type. Note that we can't use the
// isHolderType method yet because the class variable it is going to check (parameterHolderActualType)
// hasn't been initialized yet.
if (parameterGenericType != null &&
parameterGenericType.getRawType() == javax.xml.ws.Holder.class) {
// NOTE
// If you change this code, please remember to change
// OperationDesc.getResultActualType
Type type = parameterGenericType.getActualTypeArguments()[0];
if (type != null && ParameterizedType.class.isInstance(type)) {
// For types of Holder>, return class associated with Generic
returnClass = (Class)((ParameterizedType)type).getRawType();
} else if (type != null && GenericArrayType.class.isInstance(type)) {
Type componentType = ((GenericArrayType)type).getGenericComponentType();
Class arrayClass = null;
if (ParameterizedType.class.isInstance(componentType)) {
// For types of Holder[]>, return class associated with Generic[]
arrayClass = (Class)((ParameterizedType)componentType).getRawType();
} else {
// For types of Holder
© 2015 - 2024 Weber Informatics LLC | Privacy Policy