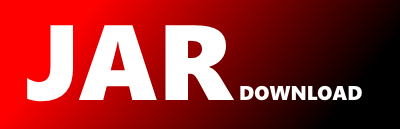
org.apache.baremaps.data.collection.DataConversions Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to you under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.baremaps.data.collection;
import java.util.*;
import java.util.Map.Entry;
/**
* Utility class for converting between collections and data collections.
*/
public class DataConversions {
private DataConversions() {
// Utility class
}
/**
* Converts a {@code DataCollection} to a {@code Collection}.
*
* @param dataCollection
* @return
* @param
*/
public static Collection asCollection(DataCollection dataCollection) {
if (dataCollection instanceof DataCollectionAdapteradapter) {
return adapter.collection;
} else {
return new CollectionAdapter<>(dataCollection);
}
}
/**
* Converts a {@code Collection} to a {@code DataCollection}.
*
* @param collection
* @return
* @param
*/
public static DataCollection asDataCollection(Collection collection) {
if (collection instanceof CollectionAdapteradapter) {
return adapter.collection;
} else {
return new DataCollectionAdapter<>(collection);
}
}
/**
* Converts a {@code DataList} to a {@code List}.
*
* @param dataList the data list
* @return the list
*/
public static List asList(DataList dataList) {
if (dataList instanceof DataListAdapteradapter) {
return adapter.list;
} else {
return new ListAdapter<>(dataList);
}
}
/**
* Converts a {@code List} to a {@code DataList}.
*
* @param list the list
* @return the data list
*/
public static DataList asDataList(List list) {
if (list instanceof ListAdapteradapter) {
return adapter.list;
} else {
return new DataListAdapter<>(list);
}
}
/**
* Converts a {@code DataMap} to a {@code Map}.
*
* @param dataMap the data map
* @return the map
*/
public static Map asMap(DataMap dataMap) {
if (dataMap instanceof DataMapAdapteradapter) {
return adapter.map;
} else {
return new MapAdapter<>(dataMap);
}
}
/**
* Converts a {@code Map} to a {@code DataMap}.
*
* @param map the map
* @return the data map
*/
public static DataMap asDataMap(Map map) {
if (map instanceof MapAdapteradapter) {
return adapter.map;
} else {
return new DataMapAdapter<>(map);
}
}
private static class CollectionAdapter extends AbstractCollection {
private final DataCollection collection;
private final int size;
public CollectionAdapter(DataCollection dataCollection) {
this.collection = dataCollection;
this.size = (int) dataCollection.size();
}
@Override
public int size() {
return size;
}
@Override
public Iterator iterator() {
return collection.iterator();
}
}
private static class DataCollectionAdapter implements DataCollection {
private final Collection collection;
public DataCollectionAdapter(Collection collection) {
this.collection = collection;
}
@Override
public long size() {
return collection.size();
}
@Override
public boolean add(E value) {
return collection.add(value);
}
@Override
public void clear() {
collection.clear();
}
@Override
public Iterator iterator() {
return collection.iterator();
}
}
private static class ListAdapter extends AbstractList {
private final DataList list;
private final int size;
public ListAdapter(DataList dataList) {
this.list = dataList;
this.size = (int) dataList.size();
}
@Override
public boolean add(E value) {
return list.add(value);
}
@Override
public E set(int index, E value) {
var oldValue = list.get(index);
list.set(index, value);
return oldValue;
}
@Override
public E get(int index) {
return list.get(index);
}
@Override
public int size() {
return size;
}
@Override
public boolean equals(Object object) {
return list.equals(object);
}
@Override
public int hashCode() {
return list.hashCode();
}
}
private static class DataListAdapter implements DataList {
private final List list;
public DataListAdapter(List list) {
this.list = list;
}
@Override
public long size() {
return list.size();
}
@Override
public void clear() {
list.clear();
}
@Override
public long addIndexed(E value) {
list.add(value);
return list.size() - 1L;
}
@Override
public void set(long index, E value) {
list.set((int) index, value);
}
@Override
public E get(long index) {
return list.get((int) index);
}
@Override
public boolean equals(Object object) {
return list.equals(object);
}
@Override
public int hashCode() {
return list.hashCode();
}
}
private static class MapAdapter extends AbstractMap {
private final DataMap map;
private final int size;
public MapAdapter(DataMap dataMap) {
this.map = dataMap;
this.size = (int) dataMap.size();
}
@Override
public V put(K key, V value) {
return map.put(key, value);
}
@Override
public V get(Object key) {
return map.get(key);
}
@Override
public Set> entrySet() {
return new AbstractSet<>() {
@Override
public Iterator> iterator() {
return map.entryIterator();
}
@Override
public int size() {
return size;
}
};
}
@Override
public boolean equals(Object object) {
return map.equals(object);
}
@Override
public int hashCode() {
return map.hashCode();
}
}
private static class DataMapAdapter implements DataMap {
private final Map map;
public DataMapAdapter(Map map) {
this.map = map;
}
@Override
public long size() {
return map.size();
}
@Override
public V get(Object key) {
return map.get(key);
}
@Override
public V put(K key, V value) {
return map.put(key, value);
}
@Override
public boolean containsKey(Object key) {
return map.containsKey(key);
}
@Override
public boolean containsValue(V value) {
return map.containsValue(value);
}
@Override
public void clear() {
map.clear();
}
@Override
public Iterator keyIterator() {
return map.keySet().iterator();
}
@Override
public Iterator valueIterator() {
return map.values().iterator();
}
@Override
public Iterator> entryIterator() {
return map.entrySet().iterator();
}
@Override
public boolean equals(Object object) {
return map.equals(object);
}
@Override
public int hashCode() {
return map.hashCode();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy