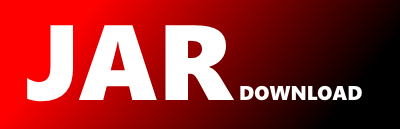
org.apache.brooklyn.location.jclouds.JcloudsLocation Maven / Gradle / Ivy
Show all versions of brooklyn-locations-jclouds Show documentation
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.brooklyn.location.jclouds;
import static com.google.common.base.Preconditions.checkArgument;
import static com.google.common.base.Preconditions.checkNotNull;
import static org.apache.brooklyn.util.JavaGroovyEquivalents.elvis;
import static org.apache.brooklyn.util.JavaGroovyEquivalents.groovyTruth;
import static org.apache.brooklyn.util.ssh.BashCommands.sbinPath;
import static org.jclouds.util.Throwables2.getFirstThrowableOfType;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.IOException;
import java.lang.reflect.InvocationTargetException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.NoSuchElementException;
import java.util.Set;
import java.util.concurrent.Callable;
import java.util.concurrent.Semaphore;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.atomic.AtomicBoolean;
import java.util.concurrent.atomic.AtomicReference;
import javax.annotation.Nullable;
import javax.xml.ws.WebServiceException;
import org.apache.brooklyn.api.entity.Entity;
import org.apache.brooklyn.api.location.LocationSpec;
import org.apache.brooklyn.api.location.MachineLocation;
import org.apache.brooklyn.api.location.MachineLocationCustomizer;
import org.apache.brooklyn.api.location.MachineManagementMixins;
import org.apache.brooklyn.api.location.NoMachinesAvailableException;
import org.apache.brooklyn.api.location.PortRange;
import org.apache.brooklyn.api.mgmt.AccessController;
import org.apache.brooklyn.api.mgmt.Task;
import org.apache.brooklyn.config.ConfigKey;
import org.apache.brooklyn.config.ConfigKey.HasConfigKey;
import org.apache.brooklyn.core.config.ConfigUtils;
import org.apache.brooklyn.core.config.Sanitizer;
import org.apache.brooklyn.core.location.AbstractLocation;
import org.apache.brooklyn.core.location.BasicMachineMetadata;
import org.apache.brooklyn.core.location.LocationConfigKeys;
import org.apache.brooklyn.core.location.LocationConfigUtils;
import org.apache.brooklyn.core.location.LocationConfigUtils.OsCredential;
import org.apache.brooklyn.core.location.PortRanges;
import org.apache.brooklyn.core.location.access.PortForwardManager;
import org.apache.brooklyn.core.location.access.PortForwardManagerLocationResolver;
import org.apache.brooklyn.core.location.access.PortMapping;
import org.apache.brooklyn.core.location.cloud.AbstractCloudMachineProvisioningLocation;
import org.apache.brooklyn.core.location.cloud.AvailabilityZoneExtension;
import org.apache.brooklyn.core.location.cloud.names.AbstractCloudMachineNamer;
import org.apache.brooklyn.core.location.cloud.names.CloudMachineNamer;
import org.apache.brooklyn.core.location.internal.LocationInternal;
import org.apache.brooklyn.core.mgmt.internal.LocalLocationManager;
import org.apache.brooklyn.core.mgmt.persist.LocationWithObjectStore;
import org.apache.brooklyn.core.mgmt.persist.PersistenceObjectStore;
import org.apache.brooklyn.core.mgmt.persist.jclouds.JcloudsBlobStoreBasedObjectStore;
import org.apache.brooklyn.location.jclouds.networking.JcloudsPortForwarderExtension;
import org.apache.brooklyn.location.jclouds.templates.PortableTemplateBuilder;
import org.apache.brooklyn.location.jclouds.templates.customize.TemplateBuilderCustomizer;
import org.apache.brooklyn.location.jclouds.templates.customize.TemplateBuilderCustomizers;
import org.apache.brooklyn.location.jclouds.templates.customize.TemplateOptionCustomizer;
import org.apache.brooklyn.location.jclouds.templates.customize.TemplateOptionCustomizers;
import org.apache.brooklyn.location.jclouds.zone.AwsAvailabilityZoneExtension;
import org.apache.brooklyn.location.ssh.SshMachineLocation;
import org.apache.brooklyn.location.winrm.WinRmMachineLocation;
import org.apache.brooklyn.util.collections.CollectionMerger;
import org.apache.brooklyn.util.collections.MutableList;
import org.apache.brooklyn.util.collections.MutableMap;
import org.apache.brooklyn.util.collections.MutableSet;
import org.apache.brooklyn.util.core.ClassLoaderUtils;
import org.apache.brooklyn.util.core.ResourceUtils;
import org.apache.brooklyn.util.core.config.ConfigBag;
import org.apache.brooklyn.util.core.config.ResolvingConfigBag;
import org.apache.brooklyn.util.core.flags.SetFromFlag;
import org.apache.brooklyn.util.core.internal.ssh.ShellTool;
import org.apache.brooklyn.util.core.internal.ssh.SshTool;
import org.apache.brooklyn.util.core.internal.winrm.WinRmTool;
import org.apache.brooklyn.util.core.internal.winrm.WinRmToolResponse;
import org.apache.brooklyn.util.core.task.DynamicTasks;
import org.apache.brooklyn.util.core.task.DynamicTasks.TaskQueueingResult;
import org.apache.brooklyn.util.core.task.TaskBuilder;
import org.apache.brooklyn.util.core.task.TaskInternal;
import org.apache.brooklyn.util.core.task.Tasks;
import org.apache.brooklyn.util.core.task.ssh.SshTasks;
import org.apache.brooklyn.util.core.text.TemplateProcessor;
import org.apache.brooklyn.util.exceptions.CompoundRuntimeException;
import org.apache.brooklyn.util.exceptions.Exceptions;
import org.apache.brooklyn.util.exceptions.ReferenceWithError;
import org.apache.brooklyn.util.exceptions.UserFacingException;
import org.apache.brooklyn.util.guava.Maybe;
import org.apache.brooklyn.util.javalang.Reflections;
import org.apache.brooklyn.util.net.Cidr;
import org.apache.brooklyn.util.net.Networking;
import org.apache.brooklyn.util.net.Protocol;
import org.apache.brooklyn.util.repeat.Repeater;
import org.apache.brooklyn.util.ssh.BashCommands;
import org.apache.brooklyn.util.ssh.IptablesCommands;
import org.apache.brooklyn.util.ssh.IptablesCommands.Chain;
import org.apache.brooklyn.util.ssh.IptablesCommands.Policy;
import org.apache.brooklyn.util.stream.Streams;
import org.apache.brooklyn.util.text.KeyValueParser;
import org.apache.brooklyn.util.text.StringPredicates;
import org.apache.brooklyn.util.text.Strings;
import org.apache.brooklyn.util.time.Duration;
import org.apache.brooklyn.util.time.Time;
import org.apache.commons.lang3.ArrayUtils;
import org.apache.commons.lang3.tuple.Pair;
import org.jclouds.compute.ComputeService;
import org.jclouds.compute.RunNodesException;
import org.jclouds.compute.config.AdminAccessConfiguration;
import org.jclouds.compute.domain.ComputeMetadata;
import org.jclouds.compute.domain.Hardware;
import org.jclouds.compute.domain.Image;
import org.jclouds.compute.domain.NodeMetadata;
import org.jclouds.compute.domain.NodeMetadata.Status;
import org.jclouds.compute.domain.NodeMetadataBuilder;
import org.jclouds.compute.domain.OperatingSystem;
import org.jclouds.compute.domain.OsFamily;
import org.jclouds.compute.domain.Template;
import org.jclouds.compute.domain.TemplateBuilder;
import org.jclouds.compute.options.TemplateOptions;
import org.jclouds.domain.Credentials;
import org.jclouds.domain.LocationScope;
import org.jclouds.domain.LoginCredentials;
import org.jclouds.rest.AuthorizationException;
import org.jclouds.scriptbuilder.domain.Statement;
import org.jclouds.scriptbuilder.domain.StatementList;
import org.jclouds.scriptbuilder.functions.InitAdminAccess;
import org.jclouds.scriptbuilder.statements.ssh.AuthorizeRSAPublicKeys;
import org.jclouds.softlayer.compute.options.SoftLayerTemplateOptions;
import org.jclouds.util.Predicates2;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.google.common.annotations.VisibleForTesting;
import com.google.common.base.Function;
import com.google.common.base.Joiner;
import com.google.common.base.Objects;
import com.google.common.base.Optional;
import com.google.common.base.Predicate;
import com.google.common.base.Predicates;
import com.google.common.base.Splitter;
import com.google.common.base.Stopwatch;
import com.google.common.base.Supplier;
import com.google.common.base.Suppliers;
import com.google.common.base.Throwables;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.ImmutableSet;
import com.google.common.collect.Iterables;
import com.google.common.collect.Iterators;
import com.google.common.collect.Lists;
import com.google.common.collect.Maps;
import com.google.common.collect.Sets;
import com.google.common.collect.Sets.SetView;
import com.google.common.net.HostAndPort;
/**
* For provisioning and managing VMs in a particular provider/region, using jclouds.
* Configuration flags are defined in {@link JcloudsLocationConfig}.
*/
public class JcloudsLocation extends AbstractCloudMachineProvisioningLocation implements
JcloudsLocationConfig, MachineManagementMixins.RichMachineProvisioningLocation,
LocationWithObjectStore, MachineManagementMixins.SuspendResumeLocation {
// TODO After converting from Groovy to Java, this is now very bad code! It relies entirely on putting
// things into and taking them out of maps; it's not type-safe, and it's thus very error-prone.
// In Groovy, that's considered ok but not in Java.
// TODO test (and fix) ability to set config keys from flags
// TODO we say config is inherited, but it isn't the case for many "deep" / jclouds properties
// e.g. when we pass getRawLocalConfigBag() in and decorate it with additional flags
// (inheritance only works when we call getConfig in this class)
public static final Logger LOG = LoggerFactory.getLogger(JcloudsLocation.class);
public static final String ROOT_USERNAME = "root";
/** these userNames are known to be the preferred/required logins in some common/default images
* where root@ is not allowed to log in */
public static final List ROOT_ALIASES = ImmutableList.of("ubuntu", "centos", "ec2-user");
public static final List COMMON_USER_NAMES_TO_TRY = ImmutableList.builder().add(ROOT_USERNAME).addAll(ROOT_ALIASES).add("admin").build();
private static final int NOTES_MAX_LENGTH = 1000;
@VisibleForTesting
static final String AWS_VPC_HELP_URL = "http://brooklyn.apache.org/v/latest/ops/locations/index.html#ec2-classic-problems-with-vpc-only-hardware-instance-types";
private final AtomicBoolean listedAvailableTemplatesOnNoSuchTemplate = new AtomicBoolean(false);
private final Map> tagMapping = Maps.newLinkedHashMap();
@SetFromFlag // so it's persisted
private final Map vmInstanceIds = Maps.newLinkedHashMap();
static { Networking.init(); }
public JcloudsLocation() {
super();
}
/** typically wants at least ACCESS_IDENTITY and ACCESS_CREDENTIAL */
public JcloudsLocation(Map,?> conf) {
super(conf);
}
@Override
@Deprecated
public JcloudsLocation configure(Map,?> properties) {
super.configure(properties);
if (config().getLocalBag().containsKey("providerLocationId")) {
LOG.warn("Using deprecated 'providerLocationId' key in "+this);
if (!config().getLocalBag().containsKey(CLOUD_REGION_ID))
config().putAll(MutableMap.of(CLOUD_REGION_ID.getName(), (String)config().getLocalBag().getStringKey("providerLocationId")));
}
if (isDisplayNameAutoGenerated() || !groovyTruth(getDisplayName())) {
setDisplayName(elvis(getProvider(), "unknown") +
(groovyTruth(getRegion()) ? ":"+getRegion() : "") +
(groovyTruth(getEndpoint()) ? ":"+getEndpoint() : ""));
}
if (getConfig(MACHINE_CREATION_SEMAPHORE) == null) {
Integer maxConcurrent = getConfig(MAX_CONCURRENT_MACHINE_CREATIONS);
if (maxConcurrent == null || maxConcurrent < 1) {
throw new IllegalStateException(MAX_CONCURRENT_MACHINE_CREATIONS.getName() + " must be >= 1, but was "+maxConcurrent);
}
config().set(MACHINE_CREATION_SEMAPHORE, new Semaphore(maxConcurrent, true));
}
return this;
}
@Override
public void init() {
super.init();
if ("aws-ec2".equals(getProvider())) {
addExtension(AvailabilityZoneExtension.class, new AwsAvailabilityZoneExtension(getManagementContext(), this));
}
}
@Override
public JcloudsLocation newSubLocation(Map,?> newFlags) {
return newSubLocation(getClass(), newFlags);
}
@Override
public JcloudsLocation newSubLocation(Class extends AbstractCloudMachineProvisioningLocation> type, Map,?> newFlags) {
// TODO should be able to use ConfigBag.newInstanceExtending; would require moving stuff around to api etc
return (JcloudsLocation) getManagementContext().getLocationManager().createLocation(LocationSpec.create(type)
.parent(this)
.configure(config().getLocalBag().getAllConfig()) // FIXME Should this just be inherited?
.configure(MACHINE_CREATION_SEMAPHORE, getMachineCreationSemaphore())
.configure(newFlags));
}
@Override
public String toString() {
Object identity = getIdentity();
String configDescription = config().getLocalBag().getDescription();
if (configDescription!=null && configDescription.startsWith(getClass().getSimpleName()))
return configDescription;
return getClass().getSimpleName()+"["+getDisplayName()+":"+(identity != null ? identity : null)+
(configDescription!=null ? "/"+configDescription : "") + "@" + getId() + "]";
}
@Override
public String toVerboseString() {
return Objects.toStringHelper(this).omitNullValues()
.add("id", getId()).add("name", getDisplayName()).add("identity", getIdentity())
.add("description", config().getLocalBag().getDescription()).add("provider", getProvider())
.add("region", getRegion()).add("endpoint", getEndpoint())
.toString();
}
public String getProvider() {
return getConfig(CLOUD_PROVIDER);
}
public String getIdentity() {
return getConfig(ACCESS_IDENTITY);
}
public String getCredential() {
return getConfig(ACCESS_CREDENTIAL);
}
/** returns the location ID used by the provider, if set, e.g. us-west-1 */
public String getRegion() {
return getConfig(CLOUD_REGION_ID);
}
public String getEndpoint() {
return (String) config().getBag().getWithDeprecation(CLOUD_ENDPOINT, JCLOUDS_KEY_ENDPOINT);
}
public String getUser(ConfigBag config) {
return (String) config.getWithDeprecation(USER, JCLOUDS_KEY_USERNAME);
}
public boolean isWindows(Template template, ConfigBag config) {
return isWindows(template.getImage(), config);
}
/**
* Whether VMs provisioned from this image will be Windows. Assume windows if the image
* explicitly says so, or if image does not tell us then fall back to whether the config
* explicitly says windows in {@link JcloudsLocationConfig#OS_FAMILY}.
*
* Will first look at {@link JcloudsLocationConfig#OS_FAMILY_OVERRIDE}, to check if that
* is set. If so, no further checks are done: the value is compared against {@link OsFamily#WINDOWS}.
*
* We believe the config (e.g. from brooklyn.properties) because for some clouds there is
* insufficient meta-data so the Image might not tell us. Thus a user can work around it
* by explicitly supplying configuration.
*/
public boolean isWindows(Image image, ConfigBag config) {
OsFamily override = config.get(OS_FAMILY_OVERRIDE);
if (override != null) return override == OsFamily.WINDOWS;
OsFamily confFamily = config.get(OS_FAMILY);
OperatingSystem os = (image != null) ? image.getOperatingSystem() : null;
return (os != null && os.getFamily() != OsFamily.UNRECOGNIZED)
? (OsFamily.WINDOWS == os.getFamily())
: (OsFamily.WINDOWS == confFamily);
}
/**
* Whether the given VM is Windows.
*
* @see #isWindows(Image, ConfigBag)
*/
public boolean isWindows(NodeMetadata node, ConfigBag config) {
OsFamily override = config.get(OS_FAMILY_OVERRIDE);
if (override != null) return override == OsFamily.WINDOWS;
OsFamily confFamily = config.get(OS_FAMILY);
OperatingSystem os = (node != null) ? node.getOperatingSystem() : null;
return (os != null && os.getFamily() != OsFamily.UNRECOGNIZED)
? (OsFamily.WINDOWS == os.getFamily())
: (OsFamily.WINDOWS == confFamily);
}
public boolean isLocationFirewalldEnabled(SshMachineLocation location) {
int result = location.execCommands("checking if firewalld is active",
ImmutableList.of(IptablesCommands.firewalldServiceIsActive()));
return result == 0;
}
protected Semaphore getMachineCreationSemaphore() {
return checkNotNull(getConfig(MACHINE_CREATION_SEMAPHORE), MACHINE_CREATION_SEMAPHORE.getName());
}
protected CloudMachineNamer getCloudMachineNamer(ConfigBag config) {
String namerClass = config.get(LocationConfigKeys.CLOUD_MACHINE_NAMER_CLASS);
if (Strings.isNonBlank(namerClass)) {
Maybe cloudNamer = Reflections.invokeConstructorFromArgs(getManagementContext().getCatalogClassLoader(), CloudMachineNamer.class, namerClass);
if (cloudNamer.isPresent()) {
return cloudNamer.get();
} else {
throw new IllegalStateException("Failed to create CloudMachineNamer "+namerClass+" for location "+this);
}
} else {
return new JcloudsMachineNamer();
}
}
public Collection getCustomizers(ConfigBag setup) {
@SuppressWarnings("deprecation")
JcloudsLocationCustomizer customizer = setup.get(JCLOUDS_LOCATION_CUSTOMIZER);
Collection customizers = setup.get(JCLOUDS_LOCATION_CUSTOMIZERS);
@SuppressWarnings("deprecation")
String customizerType = setup.get(JCLOUDS_LOCATION_CUSTOMIZER_TYPE);
@SuppressWarnings("deprecation")
String customizersSupplierType = setup.get(JCLOUDS_LOCATION_CUSTOMIZERS_SUPPLIER_TYPE);
ClassLoader catalogClassLoader = getManagementContext().getCatalogClassLoader();
List result = new ArrayList();
if (customizer != null) result.add(customizer);
if (customizers != null) result.addAll(customizers);
if (Strings.isNonBlank(customizerType)) {
Maybe customizerByType = Reflections.invokeConstructorFromArgs(catalogClassLoader, JcloudsLocationCustomizer.class, customizerType, setup);
if (customizerByType.isPresent()) {
result.add(customizerByType.get());
} else {
customizerByType = Reflections.invokeConstructorFromArgs(catalogClassLoader, JcloudsLocationCustomizer.class, customizerType);
if (customizerByType.isPresent()) {
result.add(customizerByType.get());
} else {
throw new IllegalStateException("Failed to create JcloudsLocationCustomizer "+customizersSupplierType+" for location "+this);
}
}
}
if (Strings.isNonBlank(customizersSupplierType)) {
Maybe>> supplier = Reflections.>>invokeConstructorFromArgsUntyped(catalogClassLoader, customizersSupplierType, setup);
if (supplier.isPresent()) {
result.addAll(supplier.get().get());
} else {
supplier = Reflections.>>invokeConstructorFromArgsUntyped(catalogClassLoader, customizersSupplierType);
if (supplier.isPresent()) {
result.addAll(supplier.get().get());
} else {
throw new IllegalStateException("Failed to create JcloudsLocationCustomizer supplier "+customizersSupplierType+" for location "+this);
}
}
}
return result;
}
public ConnectivityResolver getLocationNetworkInfoCustomizer(ConfigBag setup) {
ConnectivityResolver configured = setup.get(CONNECTIVITY_RESOLVER);
return (configured != null) ? configured : new DefaultConnectivityResolver();
}
protected Collection getMachineCustomizers(ConfigBag setup) {
Collection customizers = setup.get(MACHINE_LOCATION_CUSTOMIZERS);
return (customizers == null ? ImmutableList.of() : customizers);
}
public void setDefaultImageId(String val) {
config().set(DEFAULT_IMAGE_ID, val);
}
// TODO remove tagMapping, or promote it
// (i think i favour removing it, letting the config come in from the entity)
public void setTagMapping(Map> val) {
tagMapping.clear();
tagMapping.putAll(val);
}
// TODO Decide on semantics. If I give "TomcatServer" and "Ubuntu", then must I get back an image that matches both?
// Currently, just takes first match that it finds...
@Override
public Map getProvisioningFlags(Collection tags) {
Map result = Maps.newLinkedHashMap();
Collection unmatchedTags = Lists.newArrayList();
for (String it : tags) {
if (groovyTruth(tagMapping.get(it)) && !groovyTruth(result)) {
result.putAll(tagMapping.get(it));
} else {
unmatchedTags.add(it);
}
}
if (unmatchedTags.size() > 0) {
LOG.debug("Location {}, failed to match provisioning tags {}", this, unmatchedTags);
}
return result;
}
public static final Set> getAllSupportedProperties() {
Set configsOnClass = Sets.newLinkedHashSet(
Iterables.transform(ConfigUtils.getStaticKeysOnClass(JcloudsLocation.class),
new Function,String>() {
@Override @Nullable
public String apply(@Nullable HasConfigKey> input) {
return input.getConfigKey().getName();
}
}));
Set> configKeysInList = ImmutableSet.>builder()
.addAll(SUPPORTED_TEMPLATE_BUILDER_PROPERTIES.keySet())
.addAll(SUPPORTED_TEMPLATE_OPTIONS_PROPERTIES.keySet())
.build();
Set configsInList = Sets.newLinkedHashSet(
Iterables.transform(configKeysInList,
new Function,String>() {
@Override @Nullable
public String apply(@Nullable ConfigKey> input) {
return input.getName();
}
}));
SetView extrasInList = Sets.difference(configsInList, configsOnClass);
// notInList is normal
if (!extrasInList.isEmpty())
LOG.warn("JcloudsLocation supported properties differs from config defined on class: " + extrasInList);
return Collections.unmodifiableSet(configKeysInList);
}
public ComputeService getComputeService() {
return getComputeService(MutableMap.of());
}
public ComputeService getComputeService(Map,?> flags) {
ConfigBag conf = (flags==null || flags.isEmpty())
? config().getBag()
: ConfigBag.newInstanceExtending(config().getBag(), flags);
return getComputeService(conf);
}
public ComputeService getComputeService(ConfigBag config) {
ComputeServiceRegistry registry = getConfig(COMPUTE_SERVICE_REGISTRY);
return registry.findComputeService(ResolvingConfigBag.newInstanceExtending(getManagementContext(), config), true);
}
/** @deprecated since 0.7.0 use {@link #listMachines()} */ @Deprecated
public Set extends ComputeMetadata> listNodes() {
return listNodes(MutableMap.of());
}
/** @deprecated since 0.7.0 use {@link #listMachines()}.
* (no support for custom compute service flags; if that is needed, we'll have to introduce a new method,
* but it seems there are no usages) */ @Deprecated
public Set extends ComputeMetadata> listNodes(Map,?> flags) {
return getComputeService(flags).listNodes();
}
@Override
public Map listMachines() {
Set extends ComputeMetadata> nodes =
getRegion()!=null ? getComputeService().listNodesDetailsMatching(JcloudsPredicates.nodeInLocation(getRegion(), true))
: getComputeService().listNodes();
Map result = new LinkedHashMap();
for (ComputeMetadata node: nodes)
result.put(node.getId(), getMachineMetadata(node));
return result;
}
protected MachineManagementMixins.MachineMetadata getMachineMetadata(ComputeMetadata node) {
if (node==null)
return null;
return new BasicMachineMetadata(node.getId(), node.getName(),
((node instanceof NodeMetadata) ? Iterators.tryFind( ((NodeMetadata)node).getPublicAddresses().iterator(), Predicates.alwaysTrue() ).orNull() : null),
((node instanceof NodeMetadata) ? ((NodeMetadata)node).getStatus()==Status.RUNNING : null),
node);
}
@Override
public MachineManagementMixins.MachineMetadata getMachineMetadata(MachineLocation l) {
if (l instanceof JcloudsSshMachineLocation) {
return getMachineMetadata(getComputeService().getNodeMetadata(((JcloudsSshMachineLocation) l).getJcloudsId()));
}
return null;
}
@Override
public void killMachine(String cloudServiceId) {
getComputeService().destroyNode(cloudServiceId);
}
@Override
public void killMachine(MachineLocation l) {
MachineManagementMixins.MachineMetadata m = getMachineMetadata(l);
if (m==null) throw new NoSuchElementException("Machine "+l+" is not known at "+this);
killMachine(m.getId());
}
/** can generate a string describing where something is being created
* (provider, region/location and/or endpoint, callerContext);
* previously set on the config bag, but not any longer (Sept 2016) as config is treated like entities */
protected String getCreationString(ConfigBag config) {
return elvis(config.get(CLOUD_PROVIDER), "unknown")+
(config.containsKey(CLOUD_REGION_ID) ? ":"+config.get(CLOUD_REGION_ID) : "")+
(config.containsKey(CLOUD_ENDPOINT) ? ":"+config.get(CLOUD_ENDPOINT) : "")+
(config.containsKey(CALLER_CONTEXT) ? "@"+config.get(CALLER_CONTEXT) : "");
}
// ----------------- obtaining a new machine ------------------------
public MachineLocation obtain() throws NoMachinesAvailableException {
return obtain(MutableMap.of());
}
public MachineLocation obtain(TemplateBuilder tb) throws NoMachinesAvailableException {
return obtain(MutableMap.of(), tb);
}
public MachineLocation obtain(Map,?> flags, TemplateBuilder tb) throws NoMachinesAvailableException {
return obtain(MutableMap.builder().putAll(flags).put(TEMPLATE_BUILDER, tb).build());
}
/** core method for obtaining a VM using jclouds;
* Map should contain CLOUD_PROVIDER and CLOUD_ENDPOINT or CLOUD_REGION, depending on the cloud,
* as well as ACCESS_IDENTITY and ACCESS_CREDENTIAL,
* plus any further properties to specify e.g. images, hardware profiles, accessing user
* (for initial login, and a user potentially to create for subsequent ie normal access) */
@Override
public MachineLocation obtain(Map,?> flags) throws NoMachinesAvailableException {
ConfigBag setupRaw = ConfigBag.newInstanceExtending(config().getBag(), flags);
ConfigBag setup = ResolvingConfigBag.newInstanceExtending(getManagementContext(), setupRaw);
Map flagTemplateOptions = ConfigBag.newInstance(flags).get(TEMPLATE_OPTIONS);
Map baseTemplateOptions = config().get(TEMPLATE_OPTIONS);
Map templateOptions = (Map) shallowMerge(Maybe.fromNullable(flagTemplateOptions), Maybe.fromNullable(baseTemplateOptions), TEMPLATE_OPTIONS).orNull();
setup.put(TEMPLATE_OPTIONS, templateOptions);
Integer attempts = setup.get(MACHINE_CREATE_ATTEMPTS);
List exceptions = Lists.newArrayList();
if (attempts == null || attempts < 1) attempts = 1;
for (int i = 1; i <= attempts; i++) {
try {
return obtainOnce(setup);
} catch (RuntimeException e) {
LOG.warn("Attempt #{}/{} to obtain machine threw error: {}", new Object[]{i, attempts, e});
exceptions.add(e);
}
}
String msg = String.format("Failed to get VM after %d attempt%s.", attempts, attempts == 1 ? "" : "s");
Exception cause = (exceptions.size() == 1)
? exceptions.get(0)
: new CompoundRuntimeException(msg + " - "
+ "First cause is "+exceptions.get(0)+" (listed in primary trace); "
+ "plus " + (exceptions.size()-1) + " more (e.g. the last is "+exceptions.get(exceptions.size()-1)+")",
exceptions.get(0), exceptions);
if (exceptions.get(exceptions.size()-1) instanceof NoMachinesAvailableException) {
throw new NoMachinesAvailableException(msg, cause);
} else {
throw Exceptions.propagate(cause);
}
}
protected ConnectivityResolverOptions.Builder getConnectivityOptionsBuilder(ConfigBag setup, boolean isWindows) {
boolean waitForSshable = !"false".equalsIgnoreCase(setup.get(JcloudsLocationConfig.WAIT_FOR_SSHABLE));
boolean waitForWinRmable = !"false".equalsIgnoreCase(setup.get(JcloudsLocationConfig.WAIT_FOR_WINRM_AVAILABLE));
boolean waitForConnectable = isWindows ? waitForWinRmable : waitForSshable;
boolean usePortForwarding = setup.get(JcloudsLocationConfig.USE_PORT_FORWARDING);
boolean skipJcloudsSshing = usePortForwarding ||
Boolean.FALSE.equals(setup.get(JcloudsLocationConfig.USE_JCLOUDS_SSH_INIT));
ConnectivityResolverOptions.Builder builder = ConnectivityResolverOptions.builder()
.waitForConnectable(waitForConnectable)
.usePortForwarding(usePortForwarding)
.skipJcloudsSshing(skipJcloudsSshing);
String pollForFirstReachable = setup.get(JcloudsLocationConfig.POLL_FOR_FIRST_REACHABLE_ADDRESS);
boolean pollEnabled = !"false".equalsIgnoreCase(pollForFirstReachable);
if (pollEnabled) {
Predicate super HostAndPort> reachableAddressesPredicate = getReachableAddressesPredicate(setup);
Duration pollTimeout = "true".equals(pollForFirstReachable)
? Duration.FIVE_MINUTES
: Duration.of(pollForFirstReachable);
builder.pollForReachableAddresses(reachableAddressesPredicate, pollTimeout, true);
}
return builder;
}
protected MachineLocation obtainOnce(ConfigBag setup) throws NoMachinesAvailableException {
AccessController.Response access = getManagementContext().getAccessController().canProvisionLocation(this);
if (!access.isAllowed()) {
throw new IllegalStateException("Access controller forbids provisioning in "+this+": "+access.getMsg());
}
Predicate super HostAndPort> reachablePredicate = getReachableAddressesPredicate(setup);
ConnectivityResolverOptions options = getConnectivityOptionsBuilder(setup, false).build();
// FIXME How do we influence the node.getLoginPort, so it is set correctly for Windows?
// Setup port-forwarding, if required
JcloudsPortForwarderExtension portForwarder = setup.get(PORT_FORWARDER);
if (options.usePortForwarding()) checkNotNull(portForwarder, "portForwarder, when use-port-forwarding enabled");
final ComputeService computeService = getComputeService(setup);
CloudMachineNamer cloudMachineNamer = getCloudMachineNamer(setup);
String groupId = elvis(setup.get(GROUP_ID), cloudMachineNamer.generateNewGroupId(setup));
NodeMetadata node = null;
JcloudsMachineLocation machineLocation = null;
Duration semaphoreTimestamp = null;
Duration templateTimestamp = null;
Duration provisionTimestamp = null;
Duration usableTimestamp = null;
Duration customizedTimestamp = null;
Stopwatch provisioningStopwatch = Stopwatch.createStarted();
try {
LOG.info("Creating VM "+getCreationString(setup)+" in "+this);
Semaphore machineCreationSemaphore = getMachineCreationSemaphore();
boolean acquired = machineCreationSemaphore.tryAcquire(0, TimeUnit.SECONDS);
if (!acquired) {
LOG.info("Waiting in {} for machine-creation permit ({} other queuing requests already)", new Object[] {this, machineCreationSemaphore.getQueueLength()});
Stopwatch blockStopwatch = Stopwatch.createStarted();
machineCreationSemaphore.acquire();
LOG.info("Acquired in {} machine-creation permit, after waiting {}", this, Time.makeTimeStringRounded(blockStopwatch));
} else {
LOG.debug("Acquired in {} machine-creation permit immediately", this);
}
semaphoreTimestamp = Duration.of(provisioningStopwatch);
LoginCredentials userCredentials = null;
Set extends NodeMetadata> nodes;
Template template;
Collection customizers = getCustomizers(setup);
Collection machineCustomizers = getMachineCustomizers(setup);
try {
// Setup the template
template = buildTemplate(computeService, setup, customizers);
boolean expectWindows = isWindows(template, setup);
if (!options.skipJcloudsSshing()) {
if (expectWindows) {
// TODO Was this too early to look at template.getImage? e.g. customizeTemplate could subsequently modify it.
LOG.warn("Ignoring invalid configuration for Windows provisioning of "+template.getImage()+": "+USE_JCLOUDS_SSH_INIT.getName()+" should be false");
options = options.toBuilder()
.skipJcloudsSshing(true)
.build();
} else if (options.waitForConnectable()) {
userCredentials = initTemplateForCreateUser(template, setup);
}
}
templateTimestamp = Duration.of(provisioningStopwatch);
// "Name" metadata seems to set the display name; at least in AWS
// TODO it would be nice if this salt comes from the location's ID (but we don't know that yet as the ssh machine location isn't created yet)
// TODO in softlayer we want to control the suffix of the hostname which is 3 random hex digits
template.getOptions().getUserMetadata().put("Name", cloudMachineNamer.generateNewMachineUniqueNameFromGroupId(setup, groupId));
if (setup.get(JcloudsLocationConfig.INCLUDE_BROOKLYN_USER_METADATA)) {
template.getOptions().getUserMetadata().put("brooklyn-user", System.getProperty("user.name"));
Object context = setup.get(CALLER_CONTEXT);
if (context instanceof Entity) {
Entity entity = (Entity)context;
template.getOptions().getUserMetadata().put("brooklyn-app-id", entity.getApplicationId());
template.getOptions().getUserMetadata().put("brooklyn-app-name", entity.getApplication().getDisplayName());
template.getOptions().getUserMetadata().put("brooklyn-entity-id", entity.getId());
template.getOptions().getUserMetadata().put("brooklyn-entity-name", entity.getDisplayName());
template.getOptions().getUserMetadata().put("brooklyn-server-creation-date", Time.makeDateSimpleStampString());
}
}
customizeTemplate(computeService, template, customizers);
LOG.debug("jclouds using template {} / options {} to provision machine in {}",
new Object[] {template, template.getOptions(), getCreationString(setup)});
nodes = computeService.createNodesInGroup(groupId, 1, template);
provisionTimestamp = Duration.of(provisioningStopwatch);
} finally {
machineCreationSemaphore.release();
}
node = Iterables.getOnlyElement(nodes, null);
LOG.debug("jclouds created {} for {}", node, getCreationString(setup));
if (node == null)
throw new IllegalStateException("No nodes returned by jclouds create-nodes in " + getCreationString(setup));
boolean windows = isWindows(node, setup);
if (windows) {
int newLoginPort = node.getLoginPort() == 22
? (getConfig(WinRmMachineLocation.USE_HTTPS_WINRM) ? 5986 : 5985)
: node.getLoginPort();
String newLoginUser = "root".equals(node.getCredentials().getUser())
? "Administrator"
: node.getCredentials().getUser();
LOG.debug("jclouds created Windows VM {}; transforming connection details: loginPort from {} to {}; loginUser from {} to {}",
new Object[] {node, node.getLoginPort(), newLoginPort, node.getCredentials().getUser(), newLoginUser});
node = NodeMetadataBuilder.fromNodeMetadata(node)
.loginPort(newLoginPort)
.credentials(LoginCredentials.builder(node.getCredentials()).user(newLoginUser).build())
.build();
}
Optional portForwardSshOverride;
if (options.usePortForwarding()) {
portForwardSshOverride = Optional.of(portForwarder.openPortForwarding(
node,
node.getLoginPort(),
Optional.absent(),
Protocol.TCP,
Cidr.UNIVERSAL));
} else {
portForwardSshOverride = Optional.absent();
}
options = options.toBuilder()
.isWindows(windows)
.defaultLoginPort(node.getLoginPort())
.portForwardSshOverride(portForwardSshOverride.orNull())
.initialCredentials(node.getCredentials())
.userCredentials(userCredentials)
.build();
ConnectivityResolver networkInfoCustomizer = getLocationNetworkInfoCustomizer(setup);
ManagementAddressResolveResult hostPortCred = networkInfoCustomizer.resolve(this, node, setup, options);
final HostAndPort managementHostAndPort = hostPortCred.hostAndPort();
LoginCredentials creds = hostPortCred.credentials();
LOG.info("Using host-and-port={} and user={} when connecting to {}",
new Object[]{managementHostAndPort, creds.getUser(), node});
if (options.skipJcloudsSshing() && options.waitForConnectable()) {
LoginCredentials createdCredentials = createUser(computeService, node, managementHostAndPort, creds, setup);
if (createdCredentials != null) {
userCredentials = createdCredentials;
}
}
if (userCredentials == null) {
userCredentials = creds;
}
// store the credentials, in case they have changed
putIfPresentButDifferent(setup, JcloudsLocationConfig.PASSWORD, userCredentials.getOptionalPassword().orNull());
putIfPresentButDifferent(setup, JcloudsLocationConfig.PRIVATE_KEY_DATA, userCredentials.getOptionalPrivateKey().orNull());
// Wait for the VM to be reachable over SSH
if (options.waitForConnectable() && !options.isWindows()) {
waitForSshable(computeService, node, managementHostAndPort, ImmutableList.of(userCredentials), setup);
} else {
LOG.debug("Skipping ssh check for {} ({}) due to config waitForConnectable={}, windows={}",
new Object[]{node, getCreationString(setup), options.waitForConnectable(), windows});
}
// Do not store the credentials on the node as this may leak the credentials if they
// are obtained from an external supplier
node = NodeMetadataBuilder.fromNodeMetadata(node).credentials(null).build();
usableTimestamp = Duration.of(provisioningStopwatch);
// Create a JcloudsSshMachineLocation, and register it
if (windows) {
machineLocation = registerWinRmMachineLocation(computeService, node, Optional.fromNullable(template), userCredentials, managementHostAndPort, setup);
} else {
machineLocation = registerJcloudsSshMachineLocation(computeService, node, Optional.fromNullable(template), userCredentials, managementHostAndPort, setup);
}
PortForwardManager portForwardManager = setup.get(PORT_FORWARDING_MANAGER);
if (portForwardManager == null) {
LOG.debug("No PortForwardManager, using default");
portForwardManager = (PortForwardManager) getManagementContext().getLocationRegistry().getLocationManaged(PortForwardManagerLocationResolver.PFM_GLOBAL_SPEC);
}
if (options.usePortForwarding() && portForwardSshOverride.isPresent()) {
// Now that we have the sshMachineLocation, we can associate the port-forwarding address with it.
portForwardManager.associate(node.getId(), portForwardSshOverride.get(), machineLocation, node.getLoginPort());
}
if ("docker".equals(this.getProvider())) {
if (windows) {
throw new UnsupportedOperationException("Docker not supported on Windows");
}
Map portMappings = JcloudsUtil.dockerPortMappingsFor(this, node.getId());
for(Integer containerPort : portMappings.keySet()) {
Integer hostPort = portMappings.get(containerPort);
String dockerHost = ((JcloudsSshMachineLocation)machineLocation).getSshHostAndPort().getHostText();
portForwardManager.associate(node.getId(), HostAndPort.fromParts(dockerHost, hostPort), machineLocation, containerPort);
}
}
List customisationForLogging = new ArrayList();
// Apply same securityGroups rules to iptables, if iptables is running on the node
if (options.waitForConnectable()) {
String setupScript = setup.get(JcloudsLocationConfig.CUSTOM_MACHINE_SETUP_SCRIPT_URL);
List setupScripts = setup.get(JcloudsLocationConfig.CUSTOM_MACHINE_SETUP_SCRIPT_URL_LIST);
Collection allScripts = new MutableList().appendIfNotNull(setupScript).appendAll(setupScripts);
for (String setupScriptItem : allScripts) {
if (Strings.isNonBlank(setupScriptItem)) {
customisationForLogging.add("custom setup script " + setupScriptItem);
String setupVarsString = setup.get(JcloudsLocationConfig.CUSTOM_MACHINE_SETUP_SCRIPT_VARS);
Map substitutions = (setupVarsString != null)
? Splitter.on(",").withKeyValueSeparator(":").split(setupVarsString)
: ImmutableMap.of();
String scriptContent = ResourceUtils.create(this).getResourceAsString(setupScriptItem);
String script = TemplateProcessor.processTemplateContents(scriptContent, getManagementContext(), substitutions);
if (windows) {
WinRmToolResponse resp = ((WinRmMachineLocation)machineLocation).executeCommand(ImmutableList.copyOf((script.replace("\r", "").split("\n"))));
if (resp.getStatusCode() != 0) {
throw new IllegalStateException("Command 'Customizing node " + this + "' failed with exit code " + resp.getStatusCode() + " for location " + machineLocation);
}
} else {
executeCommandThrowingOnError(
(SshMachineLocation)machineLocation,
"Customizing node " + this,
ImmutableList.of(script));
}
}
}
Boolean dontRequireTtyForSudo = setup.get(JcloudsLocationConfig.DONT_REQUIRE_TTY_FOR_SUDO);
if (Boolean.TRUE.equals(dontRequireTtyForSudo) ||
(dontRequireTtyForSudo == null && setup.get(DONT_CREATE_USER))) {
if (windows) {
LOG.warn("Ignoring flag DONT_REQUIRE_TTY_FOR_SUDO on Windows location {}", machineLocation);
} else {
customisationForLogging.add("patch /etc/sudoers to disable requiretty");
queueLocationTask("patch /etc/sudoers to disable requiretty",
SshTasks.dontRequireTtyForSudo((SshMachineLocation)machineLocation, true).newTask().asTask());
}
}
if (setup.get(JcloudsLocationConfig.MAP_DEV_RANDOM_TO_DEV_URANDOM)) {
if (windows) {
LOG.warn("Ignoring flag MAP_DEV_RANDOM_TO_DEV_URANDOM on Windows location {}", machineLocation);
} else {
customisationForLogging.add("point /dev/random to urandom");
executeCommandThrowingOnError(
(SshMachineLocation)machineLocation,
"using urandom instead of random",
Arrays.asList(
BashCommands.sudo("mv /dev/random /dev/random-real"),
BashCommands.sudo("ln -s /dev/urandom /dev/random")));
}
}
if (setup.get(GENERATE_HOSTNAME)) {
if (windows) {
// TODO: Generate Windows Hostname
LOG.warn("Ignoring flag GENERATE_HOSTNAME on Windows location {}", machineLocation);
} else {
customisationForLogging.add("configure hostname");
// also see TODO in SetHostnameCustomizer - ideally we share code between here and there
executeCommandThrowingOnError(
(SshMachineLocation)machineLocation,
"Generate hostname " + node.getName(),
ImmutableList.of(BashCommands.chainGroup(
String.format("echo '127.0.0.1 %s' | ( %s )", node.getName(), BashCommands.sudo("tee -a /etc/hosts")),
"{ " + BashCommands.sudo("sed -i \"s/HOSTNAME=.*/HOSTNAME=" + node.getName() + "/g\" /etc/sysconfig/network") + " || true ; }",
BashCommands.sudo("hostname " + node.getName()))));
}
}
if (setup.get(OPEN_IPTABLES)) {
if (windows) {
LOG.warn("Ignoring DEPRECATED flag OPEN_IPTABLES on Windows location {}", machineLocation);
} else {
LOG.warn("Using DEPRECATED flag OPEN_IPTABLES (will not be supported in future versions) for {} at {}", machineLocation, this);
@SuppressWarnings("unchecked")
Iterable inboundPorts = (Iterable) setup.get(INBOUND_PORTS);
if (inboundPorts == null || Iterables.isEmpty(inboundPorts)) {
LOG.info("No ports to open in iptables (no inbound ports) for {} at {}", machineLocation, this);
} else {
customisationForLogging.add("open iptables");
List iptablesRules = Lists.newArrayList();
if (isLocationFirewalldEnabled((SshMachineLocation)machineLocation)) {
for (Integer port : inboundPorts) {
iptablesRules.add(IptablesCommands.addFirewalldRule(Chain.INPUT, Protocol.TCP, port, Policy.ACCEPT));
}
} else {
iptablesRules = Lists.newArrayList();
for (Integer port : inboundPorts) {
iptablesRules.add(IptablesCommands.insertIptablesRule(Chain.INPUT, Protocol.TCP, port, Policy.ACCEPT));
}
iptablesRules.add(IptablesCommands.saveIptablesRules());
}
List batch = Lists.newArrayList();
// Some entities, such as Riak (erlang based) have a huge range of ports, which leads to a script that
// is too large to run (fails with a broken pipe). Batch the rules into batches of 50
for (String rule : iptablesRules) {
batch.add(rule);
if (batch.size() == 50) {
executeCommandWarningOnError(
(SshMachineLocation)machineLocation,
"Inserting iptables rules, 50 command batch",
batch);
batch.clear();
}
}
if (batch.size() > 0) {
executeCommandWarningOnError(
(SshMachineLocation)machineLocation,
"Inserting iptables rules",
batch);
}
executeCommandWarningOnError(
(SshMachineLocation)machineLocation,
"List iptables rules",
ImmutableList.of(IptablesCommands.listIptablesRule()));
}
}
}
if (setup.get(STOP_IPTABLES)) {
if (windows) {
LOG.warn("Ignoring DEPRECATED flag OPEN_IPTABLES on Windows location {}", machineLocation);
} else {
LOG.warn("Using DEPRECATED flag STOP_IPTABLES (will not be supported in future versions) for {} at {}", machineLocation, this);
customisationForLogging.add("stop iptables");
List cmds = ImmutableList.of();
if (isLocationFirewalldEnabled((SshMachineLocation)machineLocation)) {
cmds = ImmutableList.of(IptablesCommands.firewalldServiceStop(), IptablesCommands.firewalldServiceStatus());
} else {
cmds = ImmutableList.of(IptablesCommands.iptablesServiceStop(), IptablesCommands.iptablesServiceStatus());
}
executeCommandWarningOnError(
(SshMachineLocation)machineLocation,
"Stopping iptables", cmds);
}
}
List extraKeyUrlsToAuth = setup.get(EXTRA_PUBLIC_KEY_URLS_TO_AUTH);
if (extraKeyUrlsToAuth!=null && !extraKeyUrlsToAuth.isEmpty()) {
if (windows) {
LOG.warn("Ignoring flag EXTRA_PUBLIC_KEY_URLS_TO_AUTH on Windows location", machineLocation);
} else {
List extraKeyDataToAuth = MutableList.of();
for (String keyUrl : extraKeyUrlsToAuth) {
extraKeyDataToAuth.add(ResourceUtils.create().getResourceAsString(keyUrl));
}
executeCommandThrowingOnError(
(SshMachineLocation)machineLocation,
"Authorizing ssh keys from URLs",
ImmutableList.of(new AuthorizeRSAPublicKeys(extraKeyDataToAuth).render(org.jclouds.scriptbuilder.domain.OsFamily.UNIX)));
}
}
String extraKeyDataToAuth = setup.get(EXTRA_PUBLIC_KEY_DATA_TO_AUTH);
if (extraKeyDataToAuth!=null && !extraKeyDataToAuth.isEmpty()) {
if (windows) {
LOG.warn("Ignoring flag EXTRA_PUBLIC_KEY_DATA_TO_AUTH on Windows location", machineLocation);
} else {
executeCommandThrowingOnError(
(SshMachineLocation)machineLocation,
"Authorizing ssh keys from data",
ImmutableList.of(new AuthorizeRSAPublicKeys(Collections.singletonList(extraKeyDataToAuth)).render(org.jclouds.scriptbuilder.domain.OsFamily.UNIX)));
}
}
} else {
// Otherwise we have deliberately not waited to be ssh'able, so don't try now to
// ssh to exec these commands!
}
// Apply any optional app-specific customization.
for (JcloudsLocationCustomizer customizer : customizers) {
LOG.debug("Customizing machine {}, using customizer {}", machineLocation, customizer);
customizer.customize(this, computeService, machineLocation);
}
for (MachineLocationCustomizer customizer : machineCustomizers) {
LOG.debug("Customizing machine {}, using customizer {}", machineLocation, customizer);
customizer.customize(machineLocation);
}
customizedTimestamp = Duration.of(provisioningStopwatch);
String logMessage = "Finished VM "+getCreationString(setup)+" creation:"
+ " "+machineLocation.getUser()+"@"+machineLocation.getAddress()+":"+machineLocation.getPort()
+ (Boolean.TRUE.equals(setup.get(LOG_CREDENTIALS))
? "password=" + userCredentials.getOptionalPassword().or("")
+ " && key=" + userCredentials.getOptionalPrivateKey().or("")
: "")
+ " ready after "+Duration.of(provisioningStopwatch).toStringRounded()
+ " ("
+ "semaphore obtained in "+Duration.of(semaphoreTimestamp).toStringRounded()+";"
+ template+" template built in "+Duration.of(templateTimestamp).subtract(semaphoreTimestamp).toStringRounded()+";"
+ " "+node+" provisioned in "+Duration.of(provisionTimestamp).subtract(templateTimestamp).toStringRounded()+";"
+ " "+machineLocation+" connection usable in "+Duration.of(usableTimestamp).subtract(provisionTimestamp).toStringRounded()+";"
+ " and os customized in "+Duration.of(customizedTimestamp).subtract(usableTimestamp).toStringRounded()+" - "+Joiner.on(", ").join(customisationForLogging)+")";
LOG.info(logMessage);
return machineLocation;
} catch (Exception e) {
if (e instanceof RunNodesException && ((RunNodesException)e).getNodeErrors().size() > 0) {
node = Iterables.get(((RunNodesException)e).getNodeErrors().keySet(), 0);
}
// sometimes AWS nodes come up busted (eg ssh not allowed); just throw it back (and maybe try for another one)
boolean destroyNode = (node != null) && Boolean.TRUE.equals(setup.get(DESTROY_ON_FAILURE));
if (e.toString().contains("VPCResourceNotSpecified")) {
String message = "Detected that your EC2 account is a legacy 'EC2 Classic' account, "
+ "but the most appropriate hardware instance type requires 'VPC'. "
+ "One quick fix is to use the 'eu-central-1' region. "
+ "Other remedies are described at "
+ AWS_VPC_HELP_URL;
LOG.error(message);
e = new UserFacingException(message, e);
}
LOG.error("Failed to start VM for "+getCreationString(setup) + (destroyNode ? " (destroying)" : "")
+ (node != null ? "; node "+node : "")
+ " after "+Duration.of(provisioningStopwatch).toStringRounded()
+ (semaphoreTimestamp != null ? " ("
+ "semaphore obtained in "+Duration.of(semaphoreTimestamp).toStringRounded()+";"
+ (templateTimestamp != null && semaphoreTimestamp != null ? " template built in "+Duration.of(templateTimestamp).subtract(semaphoreTimestamp).toStringRounded()+";" : "")
+ (provisionTimestamp != null && templateTimestamp != null ? " node provisioned in "+Duration.of(provisionTimestamp).subtract(templateTimestamp).toStringRounded()+";" : "")
+ (usableTimestamp != null && provisioningStopwatch != null ? " connection usable in "+Duration.of(usableTimestamp).subtract(provisionTimestamp).toStringRounded()+";" : "")
+ (customizedTimestamp != null && usableTimestamp != null ? " and OS customized in "+Duration.of(customizedTimestamp).subtract(usableTimestamp).toStringRounded() : "")
+ ")"
: "")
+ ": "+e.getMessage());
LOG.debug(Throwables.getStackTraceAsString(e));
if (destroyNode) {
Stopwatch destroyingStopwatch = Stopwatch.createStarted();
if (machineLocation != null) {
releaseSafely(machineLocation);
} else {
releaseNodeSafely(node);
}
LOG.info("Destroyed " + (machineLocation != null ? "machine " + machineLocation : "node " + node)
+ " in " + Duration.of(destroyingStopwatch).toStringRounded());
}
throw Exceptions.propagate(e);
}
}
private void executeCommandThrowingOnError(SshMachineLocation loc, String name, List commands) {
executeCommandThrowingOnError(ImmutableMap.of(), loc, name, commands);
}
private void executeCommandThrowingOnError(Map flags, SshMachineLocation loc, String name, List commands) {
Task task = SshTasks.newSshExecTaskFactory(loc, commands)
.summary(name)
.requiringExitCodeZero()
.configure(flags)
.newTask()
.asTask();
queueLocationTask("waiting for '" + name + "' on machine " + loc, task);
}
protected T queueLocationTask(String msg, Task task) {
TaskQueueingResult queueResult = DynamicTasks.queueIfPossible(task);
final String origDetails = Tasks.setBlockingDetails(msg);
try {
if(queueResult.isQueuedOrSubmitted()){
return task.getUnchecked();
} else {
// TODO Should we add an `orExecuteInSameThread()` in `TaskQueueingResult`?
try {
return ((TaskInternal)task).getJob().call();
} catch (Exception e) {
throw Exceptions.propagate(e);
}
}
} finally {
Tasks.setBlockingDetails(origDetails);
}
}
private void executeCommandWarningOnError(SshMachineLocation loc, String name, List commands) {
Task task = SshTasks.newSshExecTaskFactory(loc, commands)
.summary(name)
.allowingNonZeroExitCode()
.newTask()
.asTask();
int ret = queueLocationTask("waiting for '" + name + "' on machine " + loc, task);
if (ret != 0) {
LOG.warn("Command '{}' failed with exit code {} for location {}", new Object[] {name, ret, this});
}
}
// ------------- suspend and resume ------------------------------------
private void putIfPresentButDifferent(ConfigBag setup, ConfigKey key, String expectedValue) {
if (expectedValue==null) return;
String currentValue = setup.get(key);
if (Objects.equal(currentValue, expectedValue)) {
// no need to write -- and good reason not to --
// the currentValue may come from an external supplier,
// so we prefer to keep the secret in that supplier
return;
}
// either current value is null, or
// current value is different (possibly password coming from a one-time source)
// in either case prefer the expected value
setup.put(key, expectedValue);
}
/**
* Suspends the given location.
*
* Note that this method does not call the lifecycle methods of any
* {@link #getCustomizers(ConfigBag) customizers} attached to this location.
*/
@Override
public void suspendMachine(MachineLocation rawLocation) {
String instanceId = vmInstanceIds.remove(rawLocation);
if (instanceId == null) {
LOG.info("Attempt to suspend unknown machine " + rawLocation + " in " + this);
throw new IllegalArgumentException("Unknown machine " + rawLocation);
}
LOG.info("Suspending machine {} in {}, instance id {}", new Object[]{rawLocation, this, instanceId});
Exception toThrow = null;
try {
getComputeService().suspendNode(instanceId);
} catch (Exception e) {
toThrow = e;
LOG.error("Problem suspending machine " + rawLocation + " in " + this + ", instance id " + instanceId, e);
}
removeChild(rawLocation);
if (toThrow != null) {
throw Exceptions.propagate(toThrow);
}
}
/**
* Brings an existing machine with the given details under management.
*
* Note that this method does not call the lifecycle methods of any
* {@link #getCustomizers(ConfigBag) customizers} attached to this location.
*
* @param flags See {@link #registerMachine(ConfigBag)} for a description of required fields.
* @see #registerMachine(ConfigBag)
*/
@Override
public JcloudsMachineLocation resumeMachine(Map, ?> flags) {
ConfigBag setup = ConfigBag.newInstanceExtending(config().getBag(), flags);
LOG.info("{} using resuming node matching properties: {}", this, Sanitizer.sanitize(setup));
ComputeService computeService = getComputeService(setup);
NodeMetadata node = findNodeOrThrow(setup);
LOG.debug("{} resuming {}", this, node);
computeService.resumeNode(node.getId());
// Load the node a second time once it is resumed to get an object with
// hostname and addresses populated.
node = findNodeOrThrow(setup);
LOG.debug("{} resumed {}", this, node);
JcloudsMachineLocation registered = registerMachineLocation(setup, node);
LOG.info("{} resumed and registered {}", this, registered);
return registered;
}
// ------------- constructing the template, etc ------------------------
/** @deprecated since 0.11.0 use {@link TemplateOptionCustomizer} instead */
@Deprecated
public interface CustomizeTemplateOptions extends TemplateOptionCustomizer {
}
/** properties which cause customization of the TemplateBuilder */
public static final Map, ? extends TemplateBuilderCustomizer> SUPPORTED_TEMPLATE_BUILDER_PROPERTIES = ImmutableMap., TemplateBuilderCustomizer>builder()
.put(HARDWARE_ID, TemplateBuilderCustomizers.hardwareId())
.put(IMAGE_DESCRIPTION_REGEX, TemplateBuilderCustomizers.imageDescription())
.put(IMAGE_ID, TemplateBuilderCustomizers.imageId())
.put(IMAGE_NAME_REGEX, TemplateBuilderCustomizers.imageNameRegex())
.put(MIN_CORES, TemplateBuilderCustomizers.minCores())
.put(MIN_DISK, TemplateBuilderCustomizers.minDisk())
.put(MIN_RAM, TemplateBuilderCustomizers.minRam())
.put(OS_64_BIT, TemplateBuilderCustomizers.os64Bit())
.put(OS_FAMILY, TemplateBuilderCustomizers.osFamily())
.put(OS_VERSION_REGEX, TemplateBuilderCustomizers.osVersionRegex())
.put(TEMPLATE_SPEC, TemplateBuilderCustomizers.templateSpec())
/* Both done in the code, but included here so that they are in the map */
.put(DEFAULT_IMAGE_ID, TemplateBuilderCustomizers.noOp())
.put(TEMPLATE_BUILDER, TemplateBuilderCustomizers.noOp())
.build();
/** properties which cause customization of the TemplateOptions */
public static final Map, ? extends TemplateOptionCustomizer>SUPPORTED_TEMPLATE_OPTIONS_PROPERTIES = ImmutableMap., TemplateOptionCustomizer>builder()
.put(AUTO_ASSIGN_FLOATING_IP, TemplateOptionCustomizers.autoAssignFloatingIp())
.put(AUTO_CREATE_FLOATING_IPS, TemplateOptionCustomizers.autoCreateFloatingIps())
.put(AUTO_GENERATE_KEYPAIRS, TemplateOptionCustomizers.autoGenerateKeypairs())
.put(DOMAIN_NAME, TemplateOptionCustomizers.domainName())
.put(EXTRA_PUBLIC_KEY_DATA_TO_AUTH, TemplateOptionCustomizers.extraPublicKeyDataToAuth())
.put(INBOUND_PORTS, TemplateOptionCustomizers.inboundPorts())
.put(KEY_PAIR, TemplateOptionCustomizers.keyPair())
.put(LOGIN_USER, TemplateOptionCustomizers.loginUser())
.put(LOGIN_USER_PASSWORD, TemplateOptionCustomizers.loginUserPassword())
.put(LOGIN_USER_PRIVATE_KEY_DATA, TemplateOptionCustomizers.loginUserPrivateKeyData())
.put(LOGIN_USER_PRIVATE_KEY_FILE, TemplateOptionCustomizers.loginUserPrivateKeyFile())
.put(NETWORK_NAME, TemplateOptionCustomizers.networkName())
.put(RUN_AS_ROOT, TemplateOptionCustomizers.runAsRoot())
.put(SECURITY_GROUPS, TemplateOptionCustomizers.securityGroups())
.put(STRING_TAGS, TemplateOptionCustomizers.stringTags())
.put(TEMPLATE_OPTIONS, TemplateOptionCustomizers.templateOptions())
.put(USER_DATA_UUENCODED, TemplateOptionCustomizers.userDataUuencoded())
.put(USER_METADATA_MAP, TemplateOptionCustomizers.userMetadataMap())
.put(USER_METADATA_STRING, TemplateOptionCustomizers.userMetadataString())
.build();
/** hook whereby template customizations can be made for various clouds */
protected void customizeTemplate(ComputeService computeService, Template template, Collection customizers) {
for (JcloudsLocationCustomizer customizer : customizers) {
customizer.customize(this, computeService, template);
customizer.customize(this, computeService, template.getOptions());
}
// these things are nice on softlayer
if (template.getOptions() instanceof SoftLayerTemplateOptions) {
SoftLayerTemplateOptions slT = ((SoftLayerTemplateOptions)template.getOptions());
if (Strings.isBlank(slT.getDomainName()) || "jclouds.org".equals(slT.getDomainName())) {
// set a quasi-sensible domain name if none was provided (better than the default, jclouds.org)
// NB: things like brooklyn.local are disallowed
slT.domainName("local.brooklyncentral.org");
}
// convert user metadata to tags and notes because user metadata is otherwise ignored
Map md = slT.getUserMetadata();
if (md!=null && !md.isEmpty()) {
Set tags = MutableSet.copyOf(slT.getTags());
for (Map.Entry entry: md.entrySet()) {
tags.add(AbstractCloudMachineNamer.sanitize(entry.getKey())+":"+AbstractCloudMachineNamer.sanitize(entry.getValue()));
}
slT.tags(tags);
if (!md.containsKey("notes")) {
String notes = "User Metadata\n=============\n\n * " + Joiner.on("\n * ").withKeyValueSeparator(": ").join(md);
if (notes.length() > NOTES_MAX_LENGTH) {
String truncatedMsg = "...\n";
notes = notes.substring(0, NOTES_MAX_LENGTH - truncatedMsg.length()) + truncatedMsg;
}
md.put("notes", notes);
}
}
}
}
/**
* If the ImageChooser is a string, then try instantiating a class with that name (in the same
* way as we do for {@link #getCloudMachineNamer(ConfigBag)}, for example). Otherwise, assume
* that convention TypeCoercions will work.
*/
@SuppressWarnings("unchecked")
protected Function, Image> getImageChooser(ComputeService computeService, ConfigBag config) {
Function, Image> chooser;
Object rawVal = config.getStringKey(JcloudsLocationConfig.IMAGE_CHOOSER.getName());
if (rawVal instanceof String && Strings.isNonBlank((String)rawVal)) {
// Configured with a string: it could be a class that we need to instantiate
Class> clazz;
try {
clazz = new ClassLoaderUtils(this.getClass(), getManagementContext()).loadClass((String)rawVal);
} catch (ClassNotFoundException e) {
throw new IllegalStateException("Could not load configured ImageChooser " + rawVal, e);
}
Maybe> instance = Reflections.invokeConstructorFromArgs(clazz);
if (!instance.isPresent()) {
throw new IllegalStateException("Failed to create ImageChooser "+rawVal+" for location "+this);
} else if (!(instance.get() instanceof Function)) {
throw new IllegalStateException("Failed to create ImageChooser "+rawVal+" for location "+this+"; expected type Function but got "+instance.get().getClass());
} else {
chooser = (Function, Image>) instance.get();
}
} else {
chooser = config.get(JcloudsLocationConfig.IMAGE_CHOOSER);
}
return BrooklynImageChooser.cloneFor(chooser, computeService, config);
}
/** returns the jclouds Template which describes the image to be built, for the given config and compute service */
public Template buildTemplate(ComputeService computeService, ConfigBag config, Collection customizers) {
TemplateBuilder templateBuilder = config.get(TEMPLATE_BUILDER);
if (templateBuilder==null) {
templateBuilder = new PortableTemplateBuilder>();
} else {
LOG.debug("jclouds using templateBuilder {} as custom base for provisioning in {} for {}", new Object[] {
templateBuilder, this, getCreationString(config)});
}
if (templateBuilder instanceof PortableTemplateBuilder>) {
if (((PortableTemplateBuilder>)templateBuilder).imageChooser()==null) {
Function, Image> chooser = getImageChooser(computeService, config);
templateBuilder.imageChooser(chooser);
} else {
// an image chooser is already set, so do nothing
}
} else {
// template builder supplied, and we cannot check image chooser status; warn, for now
LOG.warn("Cannot check imageChooser status for {} due to manually supplied black-box TemplateBuilder; "
+ "it is recommended to use a PortableTemplateBuilder if you supply a TemplateBuilder", getCreationString(config));
}
if (!Strings.isEmpty(config.get(CLOUD_REGION_ID))) {
templateBuilder.locationId(config.get(CLOUD_REGION_ID));
}
if (Strings.isNonBlank(config.get(HARDWARE_ID))) {
String oldHardwareId = config.get(HARDWARE_ID);
String newHardwareId = transformHardwareId(oldHardwareId, config);
if (!Objects.equal(oldHardwareId, newHardwareId)) {
LOG.info("Transforming hardwareId from " + oldHardwareId + " to " + newHardwareId + ", in " + toString());
config.put(HARDWARE_ID, newHardwareId);
}
}
// Apply the template builder and options properties
for (Map.Entry, ? extends TemplateBuilderCustomizer> entry : SUPPORTED_TEMPLATE_BUILDER_PROPERTIES.entrySet()) {
ConfigKey> key = entry.getKey();
Object val = config.containsKey(key) ? config.get(key) : key.getDefaultValue();
if (val != null) {
TemplateBuilderCustomizer code = entry.getValue();
code.apply(templateBuilder, config, val);
}
}
if (templateBuilder instanceof PortableTemplateBuilder) {
((PortableTemplateBuilder>)templateBuilder).attachComputeService(computeService);
// do the default last, and only if nothing else specified (guaranteed to be a PTB if nothing else specified)
if (groovyTruth(config.get(DEFAULT_IMAGE_ID))) {
if (((PortableTemplateBuilder>)templateBuilder).isBlank()) {
templateBuilder.imageId(config.get(DEFAULT_IMAGE_ID).toString());
}
}
}
// Then apply any optional app-specific customization.
for (JcloudsLocationCustomizer customizer : customizers) {
customizer.customize(this, computeService, templateBuilder);
}
LOG.debug("jclouds using templateBuilder {} for provisioning in {} for {}", new Object[] {
templateBuilder, this, getCreationString(config)});
// Finally try to build the template
Template template = null;
Image image;
try {
template = templateBuilder.build();
if (template==null) throw new IllegalStateException("No matching template; check image and hardware constraints (e.g. OS, RAM); using "+templateBuilder);
image = template.getImage();
LOG.debug("jclouds found template "+template+" (image "+image+") for provisioning in "+this+" for "+getCreationString(config));
if (image==null) throw new IllegalStateException("No matching image in template at "+toStringNice()+"; check image constraints (OS, providers, ID); using "+templateBuilder);
} catch (AuthorizationException e) {
LOG.warn("Error resolving template -- not authorized (rethrowing: "+e+"); template is: "+template);
throw new IllegalStateException("Not authorized to access cloud "+toStringNice()+"; "+
"check identity, credentials, and endpoint (identity='"+getIdentity()+"', credential length "+getCredential().length()+")", e);
} catch (Exception e) {
try {
IOException ioe = Exceptions.getFirstThrowableOfType(e, IOException.class);
if (ioe != null) {
LOG.warn("IOException found...", ioe);
throw ioe;
}
if (listedAvailableTemplatesOnNoSuchTemplate.compareAndSet(false, true)) {
// delay subsequent log.warns (put in synch block) so the "Loading..." message is obvious
LOG.warn("Unable to match required VM template constraints "+templateBuilder+" when trying to provision VM in "+this+" (rethrowing): "+e);
logAvailableTemplates(config);
}
} catch (Exception e2) {
LOG.warn("Error loading available images to report (following original error matching template which will be rethrown): "+e2, e2);
throw new IllegalStateException("Unable to access cloud "+this+" to resolve "+templateBuilder+": "+e, e);
}
throw new IllegalStateException("Unable to match required VM template constraints "+templateBuilder+" when trying to provision VM in "+this+"; "
+ "see list of images in log. Root cause: "+e, e);
}
TemplateOptions options = template.getOptions();
// For windows, we need a startup-script to be executed that will enable winrm access.
// If there is already conflicting userMetadata, then don't replace it (and just warn).
// TODO this injection is hacky and (currently) cloud specific.
boolean windows = isWindows(template, config);
if (windows) {
String initScript = WinRmMachineLocation.getDefaultUserMetadataString(config());
String provider = getProvider();
if ("google-compute-engine".equals(provider)) {
// see https://cloud.google.com/compute/docs/startupscript:
// Set "sysprep-specialize-script-cmd" in metadata.
String startupScriptKey = "sysprep-specialize-script-cmd";
Object metadataMapRaw = config.get(USER_METADATA_MAP);
if (metadataMapRaw instanceof Map) {
Map,?> metadataMap = (Map, ?>) metadataMapRaw;
if (metadataMap.containsKey(startupScriptKey)) {
LOG.warn("Not adding startup-script for Windows VM on "+provider+", because already has key "+startupScriptKey+" in config "+USER_METADATA_MAP.getName());
} else {
Map