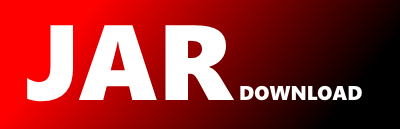
org.apache.brooklyn.location.jclouds.JcloudsLocationConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of brooklyn-locations-jclouds Show documentation
Show all versions of brooklyn-locations-jclouds Show documentation
Support jclouds API for provisioning cloud locations
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.brooklyn.location.jclouds;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.concurrent.Semaphore;
import org.apache.brooklyn.config.ConfigKey;
import org.apache.brooklyn.core.config.BasicConfigKey;
import org.apache.brooklyn.core.config.ConfigKeys;
import org.apache.brooklyn.core.location.LocationConfigKeys;
import org.apache.brooklyn.core.location.access.BrooklynAccessUtils;
import org.apache.brooklyn.core.location.access.PortForwardManager;
import org.apache.brooklyn.core.location.cloud.CloudLocationConfig;
import org.apache.brooklyn.location.jclouds.networking.JcloudsPortForwarderExtension;
import org.jclouds.Constants;
import org.jclouds.compute.domain.Image;
import org.jclouds.compute.domain.OsFamily;
import org.jclouds.compute.domain.TemplateBuilder;
import org.jclouds.domain.LoginCredentials;
import org.apache.brooklyn.util.core.internal.ssh.SshTool;
import com.google.common.annotations.Beta;
import com.google.common.base.Function;
import com.google.common.reflect.TypeToken;
public interface JcloudsLocationConfig extends CloudLocationConfig {
public static final ConfigKey CLOUD_PROVIDER = LocationConfigKeys.CLOUD_PROVIDER;
public static final ConfigKey RUN_AS_ROOT = ConfigKeys.newBooleanConfigKey("runAsRoot",
"Whether to run initial setup as root (default true)", null);
public static final ConfigKey LOGIN_USER = ConfigKeys.newStringConfigKey("loginUser",
"Override the user who logs in initially to perform setup " +
"(otherwise it is detected from the cloud or known defaults in cloud or VM OS)", null);
public static final ConfigKey LOGIN_USER_PASSWORD = ConfigKeys.newStringConfigKey("loginUser.password",
"Custom password for the user who logs in initially", null);
public static final ConfigKey LOGIN_USER_PRIVATE_KEY_DATA = ConfigKeys.newStringConfigKey("loginUser.privateKeyData",
"Custom private key for the user who logs in initially", null);
// not supported in jclouds
// public static final ConfigKey LOGIN_USER_PRIVATE_KEY_PASSPHRASE = ConfigKeys.newStringKey("loginUser.privateKeyPassphrase",
// "Passphrase for the custom private key for the user who logs in initially", null);
public static final ConfigKey LOGIN_USER_PRIVATE_KEY_FILE = ConfigKeys.newStringConfigKey("loginUser.privateKeyFile",
"Custom private key for the user who logs in initially", null);
public static final ConfigKey EXTRA_PUBLIC_KEY_DATA_TO_AUTH = ConfigKeys.newStringConfigKey("extraSshPublicKeyData",
"Additional public key data to add to authorized_keys (multi-line string supported, with one key per line)", null);
@SuppressWarnings("serial")
public static final ConfigKey> EXTRA_PUBLIC_KEY_URLS_TO_AUTH = ConfigKeys.newConfigKey(new TypeToken>() {},
"extraSshPublicKeyUrls", "Additional public keys (files or URLs, in SSH2/RFC4716/id_rsa.pub format) to add to authorized_keys", null);
public static final ConfigKey KEY_PAIR = ConfigKeys.newStringConfigKey("keyPair",
"Custom keypair (name) known at the cloud to be installed on machines for initial login (selected clouds only); "
+ "you may also need to set "+LOGIN_USER_PRIVATE_KEY_FILE.getName(), null);
public static final ConfigKey AUTO_GENERATE_KEYPAIRS = ConfigKeys.newBooleanConfigKey("jclouds.openstack-nova.auto-generate-keypairs",
"Whether to generate keypairs automatically (OpenStack Nova)");
/** @deprecated since 0.9.0 Use {@link #AUTO_ASSIGN_FLOATING_IP} instead (deprecated in 0.7.0 but warning not given until 0.9.0) */
@Deprecated
public static final ConfigKey AUTO_CREATE_FLOATING_IPS = ConfigKeys.newBooleanConfigKey("jclouds.openstack-nova.auto-create-floating-ips",
"Whether to generate floating ips for Nova");
public static final ConfigKey AUTO_ASSIGN_FLOATING_IP = ConfigKeys.newBooleanConfigKey("autoAssignFloatingIp",
"Whether to generate floating ips (in Nova paralance), or elastic IPs (in CloudStack parlance)");
public static final ConfigKey DONT_CREATE_USER = ConfigKeys.newBooleanConfigKey("dontCreateUser",
"Whether to skip creation of 'user' when provisioning machines (default false). " +
"Note that setting this will prevent jclouds from overwriting /etc/sudoers which might be " +
"configured incorrectly by default. See 'dontRequireTtyForSudo' for details.",
false);
public static final ConfigKey GRANT_USER_SUDO = ConfigKeys.newBooleanConfigKey("grantUserSudo",
"Whether to grant the created user sudo privileges. Irrelevant if dontCreateUser is true. Default: true.", true);
public static final ConfigKey DISABLE_ROOT_AND_PASSWORD_SSH = ConfigKeys.newBooleanConfigKey("disableRootAndPasswordSsh",
"Whether to disable direct SSH access for root and disable password-based SSH, "
+ "if creating a user with a key-based login; "
+ "defaults to true (set false to leave root users alone)", true);
public static final ConfigKey CUSTOM_TEMPLATE_OPTIONS_SCRIPT_CONTENTS = ConfigKeys.newStringConfigKey("customTemplateOptionsScriptContents",
"A custom script to pass to jclouds as part of template options, run after AdminAccess, "
+ "for use primarily where a command which must run as root on first login before switching to the admin user, "
+ "e.g. to customize sudoers; may start in an odd location (e.g. /tmp/bootstrap); "
+ "NB: most commands should be run by entities, or if VM-specific but sudo is okay, then via setup.script, not via this");
public static final ConfigKey CUSTOM_CREDENTIALS = new BasicConfigKey(LoginCredentials.class,
"customCredentials", "Custom jclouds LoginCredentials object to be used to connect to the VM", null);
public static final ConfigKey GROUP_ID = ConfigKeys.newStringConfigKey("groupId",
"The Jclouds group provisioned machines should be members of. " +
"Users of this config key are also responsible for configuring security groups.");
// jclouds compatibility
public static final ConfigKey JCLOUDS_KEY_USERNAME = ConfigKeys.newStringConfigKey(
"userName", "Equivalent to 'user'; provided for jclouds compatibility", null);
public static final ConfigKey JCLOUDS_KEY_ENDPOINT = ConfigKeys.newStringConfigKey(
Constants.PROPERTY_ENDPOINT, "Equivalent to 'endpoint'; provided for jclouds compatibility", null);
// note causing problems on centos due to use of `sudo -n`; but required for default RHEL VM
/**
* @deprecated since 0.8.0; instead configure this on the entity. See SoftwareProcess.OPEN_IPTABLES.
*/
@Deprecated
public static final ConfigKey OPEN_IPTABLES = ConfigKeys.newBooleanConfigKey("openIptables",
"[DEPRECATED - use openIptables on SoftwareProcess entity] Whether to open the INBOUND_PORTS via iptables rules; " +
"if true then ssh in to run iptables commands, as part of machine provisioning", true);
/**
* @deprecated since 0.8.0; instead configure this on the entity. See SoftwareProcess.STOP_IPTABLES.
*/
@Deprecated
public static final ConfigKey STOP_IPTABLES = ConfigKeys.newBooleanConfigKey("stopIptables",
"[DEPRECATED - use stopIptables on SoftwareProcess entity] Whether to stop iptables entirely; " +
"if true then ssh in to stop the iptables service, as part of machine provisioning", false);
public static final ConfigKey HARDWARE_ID = ConfigKeys.newStringConfigKey("hardwareId",
"A system-specific identifier for the hardware profile or machine type to be used when creating a VM", null);
public static final ConfigKey IMAGE_ID = ConfigKeys.newStringConfigKey("imageId",
"A system-specific identifier for the VM image to be used when creating a VM", null);
public static final ConfigKey IMAGE_NAME_REGEX = ConfigKeys.newStringConfigKey("imageNameRegex",
"A regular expression to be compared against the 'name' when selecting the VM image to be used when creating a VM", null);
public static final ConfigKey IMAGE_DESCRIPTION_REGEX = ConfigKeys.newStringConfigKey("imageDescriptionRegex",
"A regular expression to be compared against the 'description' when selecting the VM image to be used when creating a VM", null);
public static final ConfigKey TEMPLATE_SPEC = ConfigKeys.newStringConfigKey("templateSpec",
"A jclouds 'spec' string consisting of properties and values to be used when creating a VM " +
"(in most cases the properties can, and should, be specified individually using other Brooklyn location config keys)", null);
public static final ConfigKey DEFAULT_IMAGE_ID = ConfigKeys.newStringConfigKey("defaultImageId",
"A system-specific identifier for the VM image to be used by default when creating a VM " +
"(if no other VM image selection criteria are supplied)", null);
public static final ConfigKey TEMPLATE_BUILDER = ConfigKeys.newConfigKey(TemplateBuilder.class,
"templateBuilder", "A TemplateBuilder instance provided programmatically, to be used when creating a VM");
public static final ConfigKey
© 2015 - 2025 Weber Informatics LLC | Privacy Policy