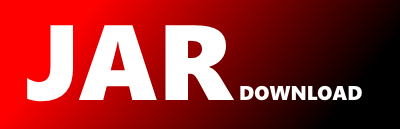
org.apache.brooklyn.util.guava.IfFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of brooklyn-utils-common Show documentation
Show all versions of brooklyn-utils-common Show documentation
Utility classes and methods developed for Brooklyn but not dependendent on Brooklyn or much else
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.brooklyn.util.guava;
import java.util.LinkedHashMap;
import java.util.Map;
import com.google.common.annotations.Beta;
import com.google.common.base.Function;
import com.google.common.base.Functions;
import com.google.common.base.Predicate;
import com.google.common.base.Predicates;
import com.google.common.base.Supplier;
/** Utilities for building {@link Function} instances which return specific values
* (or {@link Supplier} or {@link Function} instances) when certain predicates are satisfied,
* tested in order and returning the first matching,
* with support for an "else" default value if none are satisfied (null by default). */
public class IfFunctions {
public static IfFunctionBuilder newInstance(Class testType, Class returnType) {
return new IfFunctionBuilder();
}
public static IfFunctionBuilderApplyingFirst ifPredicate(Predicate super I> test) {
return new IfFunctionBuilderApplyingFirst(test);
}
public static IfFunctionBuilderApplyingFirst ifEquals(I test) {
return ifPredicate(Predicates.equalTo(test));
}
public static IfFunctionBuilderApplyingFirst ifNotEquals(I test) {
return ifPredicate(Predicates.not(Predicates.equalTo(test)));
}
@Beta
public static class IfFunction implements Function {
protected final Map,Function super I,? extends O>> tests = new LinkedHashMap,Function super I,? extends O>>();
protected Function super I,? extends O> defaultFunction = null;
protected IfFunction(IfFunction input) {
this.tests.putAll(input.tests);
this.defaultFunction = input.defaultFunction;
}
protected IfFunction() {
}
@Override
public O apply(I input) {
for (Map.Entry,Function super I,? extends O>> test: tests.entrySet()) {
if (test.getKey().apply(input))
return test.getValue().apply(input);
}
return defaultFunction==null ? null : defaultFunction.apply(input);
}
@Override
public String toString() {
return "if["+tests+"]"+(defaultFunction!=null ? "-else["+defaultFunction+"]" : "");
}
}
@Beta
public static class IfFunctionBuilder extends IfFunction {
protected IfFunctionBuilder() { super(); }
protected IfFunctionBuilder(IfFunction input) { super(input); }
public IfFunction build() {
return new IfFunction(this);
}
public IfFunctionBuilderApplying ifPredicate(Predicate test) {
return new IfFunctionBuilderApplying(this, (Predicate)test);
}
public IfFunctionBuilderApplying ifEquals(I test) {
return ifPredicate(Predicates.equalTo(test));
}
public IfFunctionBuilderApplying ifNotEquals(I test) {
return ifPredicate(Predicates.not(Predicates.equalTo(test)));
}
public IfFunctionBuilder defaultValue(O defaultValue) {
return defaultApply(new Functionals.ConstantFunction(defaultValue, defaultValue));
}
@SuppressWarnings("unchecked")
public IfFunctionBuilder defaultGet(Supplier extends O> defaultSupplier) {
return defaultApply((Function)Functions.forSupplier(defaultSupplier));
}
public IfFunctionBuilder defaultApply(Function super I,? extends O> defaultFunction) {
IfFunctionBuilder result = new IfFunctionBuilder(this);
result.defaultFunction = defaultFunction;
return result;
}
}
@Beta
public static class IfFunctionBuilderApplying {
private IfFunction input;
private Predicate super I> test;
private IfFunctionBuilderApplying(IfFunction input, Predicate super I> test) {
this.input = input;
this.test = test;
}
public IfFunctionBuilder value(O value) {
return apply(new Functionals.ConstantFunction(value, value));
}
@SuppressWarnings("unchecked")
public IfFunctionBuilder get(Supplier extends O> supplier) {
return apply((Function)Functions.forSupplier(supplier));
}
public IfFunctionBuilder apply(Function super I,? extends O> function) {
IfFunctionBuilder result = new IfFunctionBuilder(input);
result.tests.put(test, function);
return result;
}
}
@Beta
public static class IfFunctionBuilderApplyingFirst {
private Predicate super I> test;
private IfFunctionBuilderApplyingFirst(Predicate super I> test) {
this.test = test;
}
public IfFunctionBuilder value(O value) {
return apply(new Functionals.ConstantFunction(value, value));
}
@SuppressWarnings("unchecked")
public IfFunctionBuilder get(Supplier extends O> supplier) {
return apply((Function)Functions.forSupplier(supplier));
}
public IfFunctionBuilder apply(Function super I,? extends O> function) {
IfFunctionBuilder result = new IfFunctionBuilder();
result.tests.put(test, function);
return result;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy