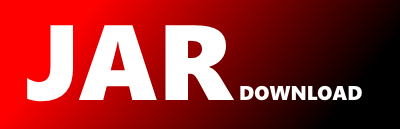
org.apache.brooklyn.util.math.MathPredicates Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of brooklyn-utils-common Show documentation
Show all versions of brooklyn-utils-common Show documentation
Utility classes and methods developed for Brooklyn but not dependendent on Brooklyn or much else
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.brooklyn.util.math;
import javax.annotation.Nullable;
import com.google.common.base.Preconditions;
import com.google.common.base.Predicate;
public class MathPredicates {
/** @deprecated since 0.9.0 kept only to allow conversion of anonymous inner classes */
@SuppressWarnings("unused") @Deprecated
private static Predicate greaterThanOld(final double val) {
// TODO PERSISTENCE WORKAROUND
return new Predicate() {
public boolean apply(@Nullable T input) {
return (input == null) ? false : input.doubleValue() > val;
}
};
}
/** @deprecated since 0.9.0 kept only to allow conversion of anonymous inner classes */
@SuppressWarnings("unused") @Deprecated
private static Predicate greaterThanOrEqualOld(final double val) {
// TODO PERSISTENCE WORKAROUND
return new Predicate() {
public boolean apply(@Nullable T input) {
return (input == null) ? false : input.doubleValue() >= val;
}
};
}
/** @deprecated since 0.9.0 kept only to allow conversion of anonymous inner classes */
@SuppressWarnings("unused") @Deprecated
private static Predicate lessThanOld(final double val) {
// TODO PERSISTENCE WORKAROUND
return new Predicate() {
public boolean apply(@Nullable T input) {
return (input == null) ? false : input.doubleValue() < val;
}
};
}
/** @deprecated since 0.9.0 kept only to allow conversion of anonymous inner classes */
@SuppressWarnings("unused") @Deprecated
private static Predicate lessThanOrEqualOld(final double val) {
// TODO PERSISTENCE WORKAROUND
return new Predicate() {
public boolean apply(@Nullable T input) {
return (input == null) ? false : input.doubleValue() <= val;
}
};
}
/**
* Creates a predicate comparing a given number with {@code val}.
* A number of {@code null} passed to the predicate will always return false.
*/
public static Predicate greaterThan(final double val) {
return new GreaterThan(val);
}
protected static class GreaterThan implements Predicate {
private final double val;
protected GreaterThan(double val) {
this.val = val;
}
public boolean apply(@Nullable T input) {
return (input == null) ? false : input.doubleValue() > val;
}
}
/**
* Creates a predicate comparing a given number with {@code val}.
* A number of {@code null} passed to the predicate will always return false.
*/
public static Predicate greaterThanOrEqual(final double val) {
return new GreaterThanOrEqual(val);
}
protected static class GreaterThanOrEqual implements Predicate {
private final double val;
protected GreaterThanOrEqual(double val) {
this.val = val;
}
public boolean apply(@Nullable T input) {
return (input == null) ? false : input.doubleValue() >= val;
}
}
/**
* Creates a predicate comparing a given number with {@code val}.
* A number of {@code null} passed to the predicate will always return false.
*/
public static Predicate lessThan(final double val) {
return new LessThan(val);
}
protected static class LessThan implements Predicate {
private final double val;
protected LessThan(double val) {
this.val = val;
}
public boolean apply(@Nullable T input) {
return (input == null) ? false : input.doubleValue() < val;
}
}
/**
* Creates a predicate comparing a given number with {@code val}.
* A number of {@code null} passed to the predicate will always return false.
*/
public static Predicate lessThanOrEqual(final double val) {
return new LessThanOrEqual(val);
}
protected static class LessThanOrEqual implements Predicate {
private final double val;
protected LessThanOrEqual(double val) {
this.val = val;
}
public boolean apply(@Nullable T input) {
return (input == null) ? false : input.doubleValue() <= val;
}
}
/**
* Creates a predicate comparing a given number with {@code val}.
* A number of {@code null} passed to the predicate will always return false.
*/
public static Predicate equalsApproximately(final Number val, final double delta) {
return new EqualsApproximately(val, delta);
}
/** Convenience for {@link #equalsApproximately(double,double)} with a delta of 10^{-6}. */
public static Predicate equalsApproximately(final Number val) {
return equalsApproximately(val, 0.0000001);
}
private static final class EqualsApproximately implements Predicate {
private final double val;
private final double delta;
private EqualsApproximately(Number val, double delta) {
this.val = val.doubleValue();
Preconditions.checkArgument(delta>=0, "delta must be non-negative");
this.delta = delta;
}
public boolean apply(@Nullable T input) {
return (input == null) ? false : Math.abs(input.doubleValue() - val) <= delta;
}
@Override
public String toString() {
return "equals-approximately("+val+" +- "+delta+")";
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy