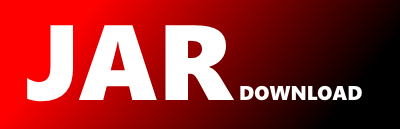
org.apache.camel.component.olingo2.api.Olingo2App Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of camel-olingo2-api Show documentation
Show all versions of camel-olingo2-api Show documentation
Camel API for Apache Olingo2
/**
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.camel.component.olingo2.api;
import java.util.List;
import java.util.Map;
import org.apache.camel.component.olingo2.api.batch.Olingo2BatchResponse;
import org.apache.olingo.odata2.api.commons.HttpStatusCodes;
import org.apache.olingo.odata2.api.edm.Edm;
/**
* Olingo2 Client Api Interface.
*/
public interface Olingo2App {
/**
* Sets Service base URI.
* @param serviceUri
*/
void setServiceUri(String serviceUri);
/**
* Returns Service base URI.
* @return service base URI.
*/
String getServiceUri();
/**
* Sets custom Http headers to add to every service request.
* @param httpHeaders custom Http headers.
*/
void setHttpHeaders(Map httpHeaders);
/**
* Returns custom Http headers.
* @return custom Http headers.
*/
Map getHttpHeaders();
/**
* Returns content type for service calls. Defaults to application/json;charset=utf-8
.
* @return content type.
*/
String getContentType();
/**
* Set default service call content type.
* @param contentType content type.
*/
void setContentType(String contentType);
/**
* Closes resources.
*/
void close();
/**
* Reads an OData resource and invokes callback with appropriate result.
* @param edm Service Edm, read from calling read(null, "$metdata", null, responseHandler)
* @param resourcePath OData Resource path
* @param queryParams OData query params
* from http://www.odata.org/documentation/odata-version-2-0/uri-conventions#SystemQueryOptions
* @param responseHandler callback handler
*/
void read(Edm edm, String resourcePath, Map queryParams,
Olingo2ResponseHandler responseHandler);
/**
* Deletes an OData resource and invokes callback
* with {@link org.apache.olingo.odata2.api.commons.HttpStatusCodes} on success, or with exception on failure.
* @param resourcePath resource path for Entry
* @param responseHandler {@link org.apache.olingo.odata2.api.commons.HttpStatusCodes} callback handler
*/
void delete(String resourcePath, Olingo2ResponseHandler responseHandler);
/**
* Creates a new OData resource.
* @param edm service Edm
* @param resourcePath resource path to create
* @param data request data
* @param responseHandler callback handler
*/
void create(Edm edm, String resourcePath, Object data, Olingo2ResponseHandler responseHandler);
/**
* Updates an OData resource.
* @param edm service Edm
* @param resourcePath resource path to update
* @param data updated data
* @param responseHandler {@link org.apache.olingo.odata2.api.ep.entry.ODataEntry} callback handler
*/
void update(Edm edm, String resourcePath, Object data, Olingo2ResponseHandler responseHandler);
/**
* Patches/merges an OData resource using HTTP PATCH.
* @param edm service Edm
* @param resourcePath resource path to update
* @param data patch/merge data
* @param responseHandler {@link org.apache.olingo.odata2.api.ep.entry.ODataEntry} callback handler
*/
void patch(Edm edm, String resourcePath, Object data, Olingo2ResponseHandler responseHandler);
/**
* Patches/merges an OData resource using HTTP MERGE.
* @param edm service Edm
* @param resourcePath resource path to update
* @param data patch/merge data
* @param responseHandler {@link org.apache.olingo.odata2.api.ep.entry.ODataEntry} callback handler
*/
void merge(Edm edm, String resourcePath, Object data, Olingo2ResponseHandler responseHandler);
/**
* Executes a batch request.
* @param edm service Edm
* @param data ordered {@link org.apache.camel.component.olingo2.api.batch.Olingo2BatchRequest} list
* @param responseHandler callback handler
*/
void batch(Edm edm, Object data, Olingo2ResponseHandler> responseHandler);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy