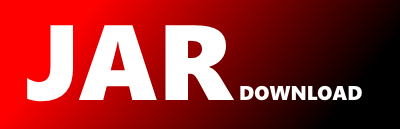
org.apache.camel.spi.ShutdownStrategy Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.camel.spi;
import java.util.List;
import java.util.concurrent.TimeUnit;
import org.apache.camel.CamelContext;
import org.apache.camel.LoggingLevel;
import org.apache.camel.StaticService;
/**
* Pluggable shutdown strategy executed during shutdown of Camel and the active routes.
*
* Shutting down routes in a reliable and graceful manner is not a trivial task. Therefore, Camel provides a pluggable
* strategy allowing 3rd party to use their own strategy if needed.
*
* The shutdown strategy is not intended for Camel end users to use for stopping routes. Instead, use
* {@link RouteController} via {@link CamelContext}.
*
* The key problem is to stop the input consumers for the routes such that no new messages is coming into Camel. But at
* the same time still keep the routes running so the existing in flight exchanges can still be run to completion. On
* top of that there are some in memory components (such as SEDA) which may have pending messages on its in memory queue
* which we want to run to completion as well, otherwise they will get lost.
*
* Camel provides a default strategy which supports all that that can be used as inspiration for your own strategy.
*
* @see org.apache.camel.spi.ShutdownAware
* @see RouteController
*/
public interface ShutdownStrategy extends StaticService {
/**
* Shutdown the routes, forcing shutdown being more aggressive, if timeout occurred.
*
* This operation is used when {@link CamelContext} is shutting down, to ensure Camel will shutdown if messages
* seems to be stuck.
*
* @param context the camel context
* @param routes the routes, ordered by the order they were started
* @throws Exception is thrown if error shutting down the consumers, however its preferred to avoid this
*/
void shutdownForced(CamelContext context, List routes) throws Exception;
/**
* Shutdown the routes
*
* @param context the camel context
* @param routes the routes, ordered by the order they were started
* @throws Exception is thrown if error shutting down the consumers, however its preferred to avoid this
*/
void shutdown(CamelContext context, List routes) throws Exception;
/**
* Suspends the routes
*
* @param context the camel context
* @param routes the routes, ordered by the order they are started
* @throws Exception is thrown if error suspending the consumers, however its preferred to avoid this
*/
void suspend(CamelContext context, List routes) throws Exception;
/**
* Shutdown the routes using a specified timeout instead of the default timeout values
*
* @param context the camel context
* @param routes the routes, ordered by the order they are started
* @param timeout timeout
* @param timeUnit the unit to use
* @throws Exception is thrown if error shutting down the consumers, however its preferred to avoid this
*/
void shutdown(CamelContext context, List routes, long timeout, TimeUnit timeUnit) throws Exception;
/**
* Shutdown the route using a specified timeout instead of the default timeout values and supports abortAfterTimeout
* mode
*
* @param context the camel context
* @param route the route
* @param timeout timeout
* @param timeUnit the unit to use
* @param abortAfterTimeout should abort shutdown after timeout
* @return true if the route is stopped before the timeout
* @throws Exception is thrown if error shutting down the consumer, however its preferred to avoid this
*/
boolean shutdown(CamelContext context, RouteStartupOrder route, long timeout, TimeUnit timeUnit, boolean abortAfterTimeout)
throws Exception;
/**
* Suspends the routes using a specified timeout instead of the default timeout values
*
* @param context the camel context
* @param routes the routes, ordered by the order they were started
* @param timeout timeout
* @param timeUnit the unit to use
* @throws Exception is thrown if error suspending the consumers, however its preferred to avoid this
*/
void suspend(CamelContext context, List routes, long timeout, TimeUnit timeUnit) throws Exception;
/**
* Set a timeout to wait for the shutdown to complete.
*
* You must set a positive value. If you want to wait (forever) then use a very high value such as
* {@link Long#MAX_VALUE}
*
* The default timeout unit is SECONDS
*
* @throws IllegalArgumentException if the timeout value is 0 or negative
* @param timeout timeout
*/
void setTimeout(long timeout);
/**
* Gets the timeout.
*
* The default timeout unit is SECONDS
*
* @return the timeout
*/
long getTimeout();
/**
* Set the time unit to use
*
* @param timeUnit the unit to use
*/
void setTimeUnit(TimeUnit timeUnit);
/**
* Gets the time unit used
*
* @return the time unit
*/
TimeUnit getTimeUnit();
/**
* Whether Camel should try to suppress logging during shutdown and timeout was triggered, meaning forced shutdown
* is happening. And during forced shutdown we want to avoid logging errors/warnings et al. in the logs as a side
* effect of the forced timeout.
*
* By default this is false
*
* Notice the suppression is a best effort as there may still be some logs coming from 3rd party libraries
* and whatnot, which Camel cannot control.
*
* @param suppressLoggingOnTimeout true to suppress logging, false to log as usual.
*/
void setSuppressLoggingOnTimeout(boolean suppressLoggingOnTimeout);
/**
* Whether Camel should try to suppress logging during shutdown and timeout was triggered, meaning forced shutdown
* is happening. And during forced shutdown we want to avoid logging errors/warnings et al. in the logs as a side
* effect of the forced timeout.
*
* By default this is false
*
* Notice the suppression is a best effort as there may still be some logs coming from 3rd party libraries
* and whatnot, which Camel cannot control.
*/
boolean isSuppressLoggingOnTimeout();
/**
* Sets whether to force shutdown of all consumers when a timeout occurred and thus not all consumers was shutdown
* within that period.
*
* You should have good reasons to set this option to false as it means that the routes keep running and is
* halted abruptly when {@link CamelContext} has been shutdown.
*
* @param shutdownNowOnTimeout true to force shutdown, false to leave them running
*/
void setShutdownNowOnTimeout(boolean shutdownNowOnTimeout);
/**
* Whether to force shutdown of all consumers when a timeout occurred.
*
* @return force shutdown or not
*/
boolean isShutdownNowOnTimeout();
/**
* Sets whether routes should be shutdown in reverse or the same order as they were started.
*
* @param shutdownRoutesInReverseOrder true to shutdown in reverse order
*/
void setShutdownRoutesInReverseOrder(boolean shutdownRoutesInReverseOrder);
/**
* Whether to shutdown routes in reverse order than they were started.
*
* This option is by default set to true.
*
* @return true if routes should be shutdown in reverse order.
*/
boolean isShutdownRoutesInReverseOrder();
/**
* Sets whether to log information about the inflight {@link org.apache.camel.Exchange}s which are still running
* during a shutdown which didn't complete without the given timeout.
*
* @param logInflightExchangesOnTimeout true to log information about the inflight exchanges,
* false to not log
*/
void setLogInflightExchangesOnTimeout(boolean logInflightExchangesOnTimeout);
/**
* Whether to log information about the inflight {@link org.apache.camel.Exchange}s which are still running during a
* shutdown which didn't complete without the given timeout.
*/
boolean isLogInflightExchangesOnTimeout();
/**
* Whether the shutdown strategy is forcing to shutdown
*/
boolean isForceShutdown();
/**
* Whether a timeout has occurred during a shutdown.
*/
boolean hasTimeoutOccurred();
/**
* Gets the logging level used for logging shutdown activity (such as starting and stopping routes). The default
* logging level is DEBUG.
*/
LoggingLevel getLoggingLevel();
/**
* Sets the logging level used for logging shutdown activity (such as starting and stopping routes). The default
* logging level is DEBUG.
*/
void setLoggingLevel(LoggingLevel loggingLevel);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy