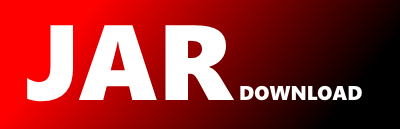
org.apache.camel.support.service.ServiceHelper Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.camel.support.service;
import java.util.Arrays;
import java.util.Collection;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Set;
import org.apache.camel.Channel;
import org.apache.camel.Navigate;
import org.apache.camel.Processor;
import org.apache.camel.Service;
import org.apache.camel.ShutdownableService;
import org.apache.camel.StatefulService;
import org.apache.camel.Suspendable;
import org.apache.camel.SuspendableService;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* A collection of helper methods for working with {@link Service} objects.
*/
public final class ServiceHelper {
private static final Logger LOG = LoggerFactory.getLogger(ServiceHelper.class);
/**
* Utility classes should not have a public constructor.
*/
private ServiceHelper() {
}
/**
* Builds the given {@code value} if it's a {@link Service} or a collection of it.
*
* Calling this method has no effect if {@code value} is {@code null}.
*/
public static void buildService(Object value) {
if (value instanceof Service service) {
service.build();
} else if (value instanceof Iterable iterable) {
for (Object o : iterable) {
buildService(o);
}
}
}
/**
* Builds each element of the given {@code services} if {@code services} itself is not {@code null}, otherwise this
* method would return immediately.
*
* @see #buildService(Object)
*/
public static void buildService(Object... services) {
if (services != null) {
for (Object o : services) {
buildService(o);
}
}
}
/**
* Initializes the given {@code value} if it's a {@link Service} or a collection of it.
*
* Calling this method has no effect if {@code value} is {@code null}.
*/
public static void initService(Object value) {
if (value instanceof Service service) {
service.init();
} else if (value instanceof Iterable iterable) {
for (Object o : iterable) {
initService(o);
}
}
}
/**
* Initializes each element of the given {@code services} if {@code services} itself is not {@code null}, otherwise
* this method would return immediately.
*
* @see #initService(Object)
*/
public static void initService(Object... services) {
if (services != null) {
for (Object o : services) {
initService(o);
}
}
}
/**
* Starts the given {@code value} if it's a {@link Service} or a collection of it.
*
* Calling this method has no effect if {@code value} is {@code null}.
*/
public static void startService(Object value) {
if (value instanceof Service service) {
startService(service);
} else if (value instanceof Iterable iterable) {
startService(iterable);
}
}
/**
* Starts the given {@code value} if it's a {@link Service} or a collection of it.
*
* Calling this method has no effect if {@code value} is {@code null}.
*/
public static void startService(Service service) {
if (service != null) {
service.start();
}
}
/**
* Starts the given {@code value} if it's a {@link Service} or a collection of it.
*
* Calling this method has no effect if {@code value} is {@code null}.
*/
public static void startService(Iterable> value) {
if (value != null) {
for (Object o : value) {
startService(o);
}
}
}
/**
* Starts each element of the given {@code services} if {@code services} itself is not {@code null}, otherwise this
* method would return immediately.
*
* @see #startService(Object)
*/
public static void startService(Object... services) {
if (services != null) {
for (Object o : services) {
startService(o);
}
}
}
/**
* Stops each element of the given {@code services} if {@code services} itself is not {@code null}, otherwise this
* method would return immediately.
*
* If there's any exception being thrown while stopping the elements one after the other this method would rethrow
* the first such exception being thrown.
*
* @see #stopService(Collection)
*/
public static void stopService(Object... services) {
if (services != null) {
for (Object o : services) {
stopService(o);
}
}
}
/**
* Stops the given {@code value}, rethrowing the first exception caught.
*
* Calling this method has no effect if {@code value} is {@code null}.
*
* @see Service#stop()
* @see #stopService(Collection)
*/
public static void stopService(Object value) {
if (value instanceof Service service) {
stopService(service);
} else if (value instanceof Iterable iterable) {
stopService(iterable);
}
}
/**
* Stops the given {@code value}, rethrowing the first exception caught.
*
* Calling this method has no effect if {@code value} is {@code null}.
*
* @see Service#stop()
* @see #stopService(Collection)
*/
public static void stopService(Service service) {
if (service != null) {
service.stop();
}
}
/**
* Stops the given {@code value}, rethrowing the first exception caught.
*
* Calling this method has no effect if {@code value} is {@code null}.
*
* @see Service#stop()
* @see #stopService(Collection)
*/
public static void stopService(Iterable> value) {
if (value != null) {
for (Object o : value) {
stopService(o);
}
}
}
/**
* Stops each element of the given {@code services} if {@code services} itself is not {@code null}, otherwise this
* method would return immediately.
*
* If there's any exception being thrown while stopping the elements one after the other this method would rethrow
* the first such exception being thrown.
*
* @see #stopService(Object)
*/
public static void stopService(Collection> services) {
if (services == null) {
return;
}
RuntimeException firstException = null;
for (Object value : services) {
try {
stopService(value);
} catch (RuntimeException e) {
if (LOG.isDebugEnabled()) {
LOG.debug("Caught exception stopping service: {}", value, e);
}
if (firstException == null) {
firstException = e;
}
}
}
if (firstException != null) {
throw firstException;
}
}
/**
* Stops and shutdowns each element of the given {@code services} if {@code services} itself is not {@code null},
* otherwise this method would return immediately.
*
* If there's any exception being thrown while stopping/shutting down the elements one after the other this method
* would rethrow the first such exception being thrown.
*
* @see #stopAndShutdownServices(Collection)
*/
public static void stopAndShutdownServices(Object... services) {
if (services == null) {
return;
}
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy