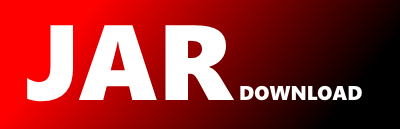
org.apache.camel.RouteTemplateContext Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.camel;
import java.util.Map;
import java.util.function.Consumer;
import java.util.function.Supplier;
import org.apache.camel.spi.BeanRepository;
import org.apache.camel.spi.HasCamelContext;
/**
* The context used during creating a {@link Route} from a route template.
*/
public interface RouteTemplateContext extends HasCamelContext {
/**
* Used for template beans to supply the local bean the route template should use when creating route(s).
*/
@FunctionalInterface
interface BeanSupplier {
T get(RouteTemplateContext rtc);
}
/**
* Binds the bean to the repository (if possible).
*
* If the bean is {@link CamelContextAware} then the registry will automatically inject the context if possible.
*
* @param id the id of the bean
* @param bean the bean
*/
default void bind(String id, Object bean) {
bind(id, bean.getClass(), bean);
}
/**
* Binds the bean to the repository (if possible).
*
* Binding by id and type allows to bind multiple entries with the same id but with different type.
*
* If the bean is {@link CamelContextAware} then the registry will automatically inject the context if possible.
*
* @param id the id of the bean
* @param type the type of the bean to associate the binding
* @param bean the bean
*/
void bind(String id, Class> type, Object bean);
/**
* Binds the bean (via a supplier) to the repository (if possible).
*
* Camel will cache the result from the supplier from first lookup (singleton scope). If you do not need cached then
* use {@link #bindAsPrototype(String, Class, Supplier)} instead.
*
* Binding by id and type allows to bind multiple entries with the same id but with different type.
*
* If the bean is {@link CamelContextAware} then the registry will automatically inject the context if possible.
*
* @param id the id of the bean
* @param type the type of the bean to associate the binding
* @param bean a supplier for the bean
*/
void bind(String id, Class> type, Supplier
© 2015 - 2025 Weber Informatics LLC | Privacy Policy