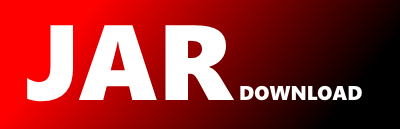
org.apache.camel.component.aws2.kinesis.Kinesis2Consumer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of camel-aws2-kinesis Show documentation
Show all versions of camel-aws2-kinesis Show documentation
A Camel Amazon Kinesis Web Service Component Version 2
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.camel.component.aws2.kinesis;
import java.util.ArrayDeque;
import java.util.List;
import java.util.Queue;
import org.apache.camel.AsyncCallback;
import org.apache.camel.Exchange;
import org.apache.camel.Processor;
import org.apache.camel.support.ScheduledBatchPollingConsumer;
import org.apache.camel.util.CastUtils;
import org.apache.camel.util.ObjectHelper;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.services.kinesis.KinesisClient;
import software.amazon.awssdk.services.kinesis.model.DescribeStreamRequest;
import software.amazon.awssdk.services.kinesis.model.DescribeStreamResponse;
import software.amazon.awssdk.services.kinesis.model.GetRecordsRequest;
import software.amazon.awssdk.services.kinesis.model.GetRecordsResponse;
import software.amazon.awssdk.services.kinesis.model.GetShardIteratorRequest;
import software.amazon.awssdk.services.kinesis.model.GetShardIteratorResponse;
import software.amazon.awssdk.services.kinesis.model.Record;
import software.amazon.awssdk.services.kinesis.model.Shard;
import software.amazon.awssdk.services.kinesis.model.ShardIteratorType;
public class Kinesis2Consumer extends ScheduledBatchPollingConsumer {
private static final Logger LOG = LoggerFactory.getLogger(Kinesis2Consumer.class);
private String currentShardIterator;
private boolean isShardClosed;
public Kinesis2Consumer(Kinesis2Endpoint endpoint, Processor processor) {
super(endpoint, processor);
}
@Override
protected int poll() throws Exception {
String shardIterator = getShardIterator();
if (shardIterator == null) {
// probably closed. Returning 0 as nothing was processed
return 0;
}
GetRecordsRequest req = GetRecordsRequest
.builder()
.shardIterator(shardIterator)
.limit(getEndpoint()
.getConfiguration()
.getMaxResultsPerRequest())
.build();
GetRecordsResponse result = getClient().getRecords(req);
Queue exchanges = createExchanges(result.records());
int processedExchangeCount = processBatch(CastUtils.cast(exchanges));
// May cache the last successful sequence number, and pass it to the
// getRecords request. That way, on the next poll, we start from where
// we left off, however, I don't know what happens to subsequent
// exchanges when an earlier exchange fails.
currentShardIterator = result.nextShardIterator();
if (isShardClosed) {
switch (getEndpoint().getConfiguration().getShardClosed()) {
case ignore:
LOG.warn("The shard {} is in closed state", currentShardIterator);
break;
case silent:
break;
case fail:
LOG.info("Shard Iterator reaches CLOSE status:{} {}", getEndpoint().getConfiguration().getStreamName(),
getEndpoint().getConfiguration().getShardId());
throw new ReachedClosedStatusException(
getEndpoint().getConfiguration().getStreamName(), getEndpoint().getConfiguration().getShardId());
default:
throw new IllegalArgumentException("Unsupported shard closed strategy");
}
}
return processedExchangeCount;
}
@Override
public int processBatch(Queue
© 2015 - 2025 Weber Informatics LLC | Privacy Policy