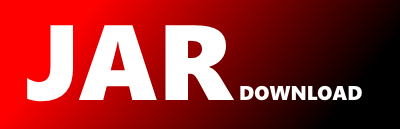
org.apache.camel.component.azure.cosmosdb.CosmosDbComponentConfigurer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of camel-azure-cosmosdb Show documentation
Show all versions of camel-azure-cosmosdb Show documentation
Camel Azure CosmosDB Component
/* Generated by camel build tools - do NOT edit this file! */
package org.apache.camel.component.azure.cosmosdb;
import java.util.Map;
import org.apache.camel.CamelContext;
import org.apache.camel.spi.ExtendedPropertyConfigurerGetter;
import org.apache.camel.spi.PropertyConfigurerGetter;
import org.apache.camel.spi.ConfigurerStrategy;
import org.apache.camel.spi.GeneratedPropertyConfigurer;
import org.apache.camel.util.CaseInsensitiveMap;
import org.apache.camel.support.component.PropertyConfigurerSupport;
/**
* Generated by camel build tools - do NOT edit this file!
*/
@SuppressWarnings("unchecked")
public class CosmosDbComponentConfigurer extends PropertyConfigurerSupport implements GeneratedPropertyConfigurer, PropertyConfigurerGetter {
private org.apache.camel.component.azure.cosmosdb.CosmosDbConfiguration getOrCreateConfiguration(CosmosDbComponent target) {
if (target.getConfiguration() == null) {
target.setConfiguration(new org.apache.camel.component.azure.cosmosdb.CosmosDbConfiguration());
}
return target.getConfiguration();
}
@Override
public boolean configure(CamelContext camelContext, Object obj, String name, Object value, boolean ignoreCase) {
CosmosDbComponent target = (CosmosDbComponent) obj;
switch (ignoreCase ? name.toLowerCase() : name) {
case "accountkey":
case "accountKey": getOrCreateConfiguration(target).setAccountKey(property(camelContext, java.lang.String.class, value)); return true;
case "autowiredenabled":
case "autowiredEnabled": target.setAutowiredEnabled(property(camelContext, boolean.class, value)); return true;
case "bridgeerrorhandler":
case "bridgeErrorHandler": target.setBridgeErrorHandler(property(camelContext, boolean.class, value)); return true;
case "changefeedprocessoroptions":
case "changeFeedProcessorOptions": getOrCreateConfiguration(target).setChangeFeedProcessorOptions(property(camelContext, com.azure.cosmos.models.ChangeFeedProcessorOptions.class, value)); return true;
case "clienttelemetryenabled":
case "clientTelemetryEnabled": getOrCreateConfiguration(target).setClientTelemetryEnabled(property(camelContext, boolean.class, value)); return true;
case "configuration": target.setConfiguration(property(camelContext, org.apache.camel.component.azure.cosmosdb.CosmosDbConfiguration.class, value)); return true;
case "connectionsharingacrossclientsenabled":
case "connectionSharingAcrossClientsEnabled": getOrCreateConfiguration(target).setConnectionSharingAcrossClientsEnabled(property(camelContext, boolean.class, value)); return true;
case "consistencylevel":
case "consistencyLevel": getOrCreateConfiguration(target).setConsistencyLevel(property(camelContext, com.azure.cosmos.ConsistencyLevel.class, value)); return true;
case "containerpartitionkeypath":
case "containerPartitionKeyPath": getOrCreateConfiguration(target).setContainerPartitionKeyPath(property(camelContext, java.lang.String.class, value)); return true;
case "contentresponseonwriteenabled":
case "contentResponseOnWriteEnabled": getOrCreateConfiguration(target).setContentResponseOnWriteEnabled(property(camelContext, boolean.class, value)); return true;
case "cosmosasyncclient":
case "cosmosAsyncClient": getOrCreateConfiguration(target).setCosmosAsyncClient(property(camelContext, com.azure.cosmos.CosmosAsyncClient.class, value)); return true;
case "createcontainerifnotexists":
case "createContainerIfNotExists": getOrCreateConfiguration(target).setCreateContainerIfNotExists(property(camelContext, boolean.class, value)); return true;
case "createdatabaseifnotexists":
case "createDatabaseIfNotExists": getOrCreateConfiguration(target).setCreateDatabaseIfNotExists(property(camelContext, boolean.class, value)); return true;
case "createleasecontainerifnotexists":
case "createLeaseContainerIfNotExists": getOrCreateConfiguration(target).setCreateLeaseContainerIfNotExists(property(camelContext, boolean.class, value)); return true;
case "createleasedatabaseifnotexists":
case "createLeaseDatabaseIfNotExists": getOrCreateConfiguration(target).setCreateLeaseDatabaseIfNotExists(property(camelContext, boolean.class, value)); return true;
case "credentialtype":
case "credentialType": getOrCreateConfiguration(target).setCredentialType(property(camelContext, org.apache.camel.component.azure.cosmosdb.CredentialType.class, value)); return true;
case "databaseendpoint":
case "databaseEndpoint": getOrCreateConfiguration(target).setDatabaseEndpoint(property(camelContext, java.lang.String.class, value)); return true;
case "hostname":
case "hostName": getOrCreateConfiguration(target).setHostName(property(camelContext, java.lang.String.class, value)); return true;
case "indexingpolicy":
case "indexingPolicy": getOrCreateConfiguration(target).setIndexingPolicy(property(camelContext, com.azure.cosmos.models.IndexingPolicy.class, value)); return true;
case "itemid":
case "itemId": getOrCreateConfiguration(target).setItemId(property(camelContext, java.lang.String.class, value)); return true;
case "itempartitionkey":
case "itemPartitionKey": getOrCreateConfiguration(target).setItemPartitionKey(property(camelContext, java.lang.String.class, value)); return true;
case "lazystartproducer":
case "lazyStartProducer": target.setLazyStartProducer(property(camelContext, boolean.class, value)); return true;
case "leasecontainername":
case "leaseContainerName": getOrCreateConfiguration(target).setLeaseContainerName(property(camelContext, java.lang.String.class, value)); return true;
case "leasedatabasename":
case "leaseDatabaseName": getOrCreateConfiguration(target).setLeaseDatabaseName(property(camelContext, java.lang.String.class, value)); return true;
case "multiplewriteregionsenabled":
case "multipleWriteRegionsEnabled": getOrCreateConfiguration(target).setMultipleWriteRegionsEnabled(property(camelContext, boolean.class, value)); return true;
case "operation": getOrCreateConfiguration(target).setOperation(property(camelContext, org.apache.camel.component.azure.cosmosdb.CosmosDbOperationsDefinition.class, value)); return true;
case "preferredregions":
case "preferredRegions": getOrCreateConfiguration(target).setPreferredRegions(property(camelContext, java.lang.String.class, value)); return true;
case "query": getOrCreateConfiguration(target).setQuery(property(camelContext, java.lang.String.class, value)); return true;
case "queryrequestoptions":
case "queryRequestOptions": getOrCreateConfiguration(target).setQueryRequestOptions(property(camelContext, com.azure.cosmos.models.CosmosQueryRequestOptions.class, value)); return true;
case "readrequestsfallbackenabled":
case "readRequestsFallbackEnabled": getOrCreateConfiguration(target).setReadRequestsFallbackEnabled(property(camelContext, boolean.class, value)); return true;
case "throughputproperties":
case "throughputProperties": getOrCreateConfiguration(target).setThroughputProperties(property(camelContext, com.azure.cosmos.models.ThroughputProperties.class, value)); return true;
default: return false;
}
}
@Override
public String[] getAutowiredNames() {
return new String[]{"cosmosAsyncClient"};
}
@Override
public Class> getOptionType(String name, boolean ignoreCase) {
switch (ignoreCase ? name.toLowerCase() : name) {
case "accountkey":
case "accountKey": return java.lang.String.class;
case "autowiredenabled":
case "autowiredEnabled": return boolean.class;
case "bridgeerrorhandler":
case "bridgeErrorHandler": return boolean.class;
case "changefeedprocessoroptions":
case "changeFeedProcessorOptions": return com.azure.cosmos.models.ChangeFeedProcessorOptions.class;
case "clienttelemetryenabled":
case "clientTelemetryEnabled": return boolean.class;
case "configuration": return org.apache.camel.component.azure.cosmosdb.CosmosDbConfiguration.class;
case "connectionsharingacrossclientsenabled":
case "connectionSharingAcrossClientsEnabled": return boolean.class;
case "consistencylevel":
case "consistencyLevel": return com.azure.cosmos.ConsistencyLevel.class;
case "containerpartitionkeypath":
case "containerPartitionKeyPath": return java.lang.String.class;
case "contentresponseonwriteenabled":
case "contentResponseOnWriteEnabled": return boolean.class;
case "cosmosasyncclient":
case "cosmosAsyncClient": return com.azure.cosmos.CosmosAsyncClient.class;
case "createcontainerifnotexists":
case "createContainerIfNotExists": return boolean.class;
case "createdatabaseifnotexists":
case "createDatabaseIfNotExists": return boolean.class;
case "createleasecontainerifnotexists":
case "createLeaseContainerIfNotExists": return boolean.class;
case "createleasedatabaseifnotexists":
case "createLeaseDatabaseIfNotExists": return boolean.class;
case "credentialtype":
case "credentialType": return org.apache.camel.component.azure.cosmosdb.CredentialType.class;
case "databaseendpoint":
case "databaseEndpoint": return java.lang.String.class;
case "hostname":
case "hostName": return java.lang.String.class;
case "indexingpolicy":
case "indexingPolicy": return com.azure.cosmos.models.IndexingPolicy.class;
case "itemid":
case "itemId": return java.lang.String.class;
case "itempartitionkey":
case "itemPartitionKey": return java.lang.String.class;
case "lazystartproducer":
case "lazyStartProducer": return boolean.class;
case "leasecontainername":
case "leaseContainerName": return java.lang.String.class;
case "leasedatabasename":
case "leaseDatabaseName": return java.lang.String.class;
case "multiplewriteregionsenabled":
case "multipleWriteRegionsEnabled": return boolean.class;
case "operation": return org.apache.camel.component.azure.cosmosdb.CosmosDbOperationsDefinition.class;
case "preferredregions":
case "preferredRegions": return java.lang.String.class;
case "query": return java.lang.String.class;
case "queryrequestoptions":
case "queryRequestOptions": return com.azure.cosmos.models.CosmosQueryRequestOptions.class;
case "readrequestsfallbackenabled":
case "readRequestsFallbackEnabled": return boolean.class;
case "throughputproperties":
case "throughputProperties": return com.azure.cosmos.models.ThroughputProperties.class;
default: return null;
}
}
@Override
public Object getOptionValue(Object obj, String name, boolean ignoreCase) {
CosmosDbComponent target = (CosmosDbComponent) obj;
switch (ignoreCase ? name.toLowerCase() : name) {
case "accountkey":
case "accountKey": return getOrCreateConfiguration(target).getAccountKey();
case "autowiredenabled":
case "autowiredEnabled": return target.isAutowiredEnabled();
case "bridgeerrorhandler":
case "bridgeErrorHandler": return target.isBridgeErrorHandler();
case "changefeedprocessoroptions":
case "changeFeedProcessorOptions": return getOrCreateConfiguration(target).getChangeFeedProcessorOptions();
case "clienttelemetryenabled":
case "clientTelemetryEnabled": return getOrCreateConfiguration(target).isClientTelemetryEnabled();
case "configuration": return target.getConfiguration();
case "connectionsharingacrossclientsenabled":
case "connectionSharingAcrossClientsEnabled": return getOrCreateConfiguration(target).isConnectionSharingAcrossClientsEnabled();
case "consistencylevel":
case "consistencyLevel": return getOrCreateConfiguration(target).getConsistencyLevel();
case "containerpartitionkeypath":
case "containerPartitionKeyPath": return getOrCreateConfiguration(target).getContainerPartitionKeyPath();
case "contentresponseonwriteenabled":
case "contentResponseOnWriteEnabled": return getOrCreateConfiguration(target).isContentResponseOnWriteEnabled();
case "cosmosasyncclient":
case "cosmosAsyncClient": return getOrCreateConfiguration(target).getCosmosAsyncClient();
case "createcontainerifnotexists":
case "createContainerIfNotExists": return getOrCreateConfiguration(target).isCreateContainerIfNotExists();
case "createdatabaseifnotexists":
case "createDatabaseIfNotExists": return getOrCreateConfiguration(target).isCreateDatabaseIfNotExists();
case "createleasecontainerifnotexists":
case "createLeaseContainerIfNotExists": return getOrCreateConfiguration(target).isCreateLeaseContainerIfNotExists();
case "createleasedatabaseifnotexists":
case "createLeaseDatabaseIfNotExists": return getOrCreateConfiguration(target).isCreateLeaseDatabaseIfNotExists();
case "credentialtype":
case "credentialType": return getOrCreateConfiguration(target).getCredentialType();
case "databaseendpoint":
case "databaseEndpoint": return getOrCreateConfiguration(target).getDatabaseEndpoint();
case "hostname":
case "hostName": return getOrCreateConfiguration(target).getHostName();
case "indexingpolicy":
case "indexingPolicy": return getOrCreateConfiguration(target).getIndexingPolicy();
case "itemid":
case "itemId": return getOrCreateConfiguration(target).getItemId();
case "itempartitionkey":
case "itemPartitionKey": return getOrCreateConfiguration(target).getItemPartitionKey();
case "lazystartproducer":
case "lazyStartProducer": return target.isLazyStartProducer();
case "leasecontainername":
case "leaseContainerName": return getOrCreateConfiguration(target).getLeaseContainerName();
case "leasedatabasename":
case "leaseDatabaseName": return getOrCreateConfiguration(target).getLeaseDatabaseName();
case "multiplewriteregionsenabled":
case "multipleWriteRegionsEnabled": return getOrCreateConfiguration(target).isMultipleWriteRegionsEnabled();
case "operation": return getOrCreateConfiguration(target).getOperation();
case "preferredregions":
case "preferredRegions": return getOrCreateConfiguration(target).getPreferredRegions();
case "query": return getOrCreateConfiguration(target).getQuery();
case "queryrequestoptions":
case "queryRequestOptions": return getOrCreateConfiguration(target).getQueryRequestOptions();
case "readrequestsfallbackenabled":
case "readRequestsFallbackEnabled": return getOrCreateConfiguration(target).isReadRequestsFallbackEnabled();
case "throughputproperties":
case "throughputProperties": return getOrCreateConfiguration(target).getThroughputProperties();
default: return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy