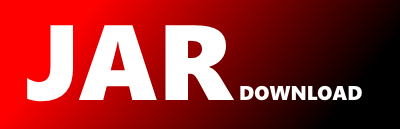
org.apache.camel.component.azure.cosmosdb.CosmosDbConfigurationOptionsProxy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of camel-azure-cosmosdb Show documentation
Show all versions of camel-azure-cosmosdb Show documentation
Camel Azure CosmosDB Component
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.camel.component.azure.cosmosdb;
import java.util.Collections;
import java.util.List;
import java.util.function.Supplier;
import com.azure.cosmos.models.*;
import org.apache.camel.Exchange;
import org.apache.camel.util.ObjectHelper;
/**
* A proxy class for {@link CosmosDbConfiguration} and {@link CosmosDbConstants}. Ideally this is responsible to obtain
* the correct configurations options either from configs or exchange headers
*/
public class CosmosDbConfigurationOptionsProxy {
private final CosmosDbConfiguration configuration;
public CosmosDbConfigurationOptionsProxy(final CosmosDbConfiguration configuration) {
this.configuration = configuration;
}
public String getDatabaseName(final Exchange exchange) {
return getOption(exchange, CosmosDbConstants.DATABASE_NAME, configuration::getDatabaseName, String.class);
}
public ThroughputProperties getThroughputProperties(final Exchange exchange) {
return getOption(exchange, CosmosDbConstants.THROUGHPUT_PROPERTIES, configuration::getThroughputProperties,
ThroughputProperties.class);
}
public CosmosDatabaseRequestOptions getCosmosDatabaseRequestOptions(final Exchange exchange) {
return getOption(exchange, CosmosDbConstants.DATABASE_REQUEST_OPTIONS, nullFallback(),
CosmosDatabaseRequestOptions.class);
}
public CosmosQueryRequestOptions getQueryRequestOptions(final Exchange exchange) {
return getOption(exchange, CosmosDbConstants.QUERY_REQUEST_OPTIONS, configuration::getQueryRequestOptions,
CosmosQueryRequestOptions.class);
}
public boolean isCreateDatabaseIfNotExist(final Exchange exchange) {
return getOption(exchange, CosmosDbConstants.CREATE_DATABASE_IF_NOT_EXIST, configuration::isCreateDatabaseIfNotExists,
boolean.class);
}
public boolean isCreateContainerIfNotExist(final Exchange exchange) {
return getOption(exchange, CosmosDbConstants.CREATE_CONTAINER_IF_NOT_EXIST, configuration::isCreateContainerIfNotExists,
boolean.class);
}
public CosmosDbOperationsDefinition getOperation(final Exchange exchange) {
return getOption(exchange, CosmosDbConstants.OPERATION, configuration::getOperation,
CosmosDbOperationsDefinition.class);
}
public String getQuery(final Exchange exchange) {
return getOption(exchange, CosmosDbConstants.QUERY, configuration::getQuery, String.class);
}
public String getContainerName(final Exchange exchange) {
return getOption(exchange, CosmosDbConstants.CONTAINER_NAME, configuration::getContainerName, String.class);
}
public String getContainerPartitionKeyPath(final Exchange exchange) {
return getOption(exchange, CosmosDbConstants.CONTAINER_PARTITION_KEY_PATH, configuration::getContainerPartitionKeyPath,
String.class);
}
public IndexingPolicy getIndexingPolicy(final Exchange exchange) {
return getOption(exchange, CosmosDbConstants.INDEXING_POLICY, configuration::getIndexingPolicy,
IndexingPolicy.class);
}
public CosmosContainerRequestOptions getContainerRequestOptions(final Exchange exchange) {
return getOption(exchange, CosmosDbConstants.CONTAINER_REQUEST_OPTIONS, nullFallback(),
CosmosContainerRequestOptions.class);
}
public PartitionKey getItemPartitionKey(final Exchange exchange) {
return new PartitionKey(
getOption(exchange, CosmosDbConstants.ITEM_PARTITION_KEY, configuration::getItemPartitionKey,
String.class));
}
public Object getItem(final Exchange exchange) {
return exchange.getIn().getBody();
}
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy