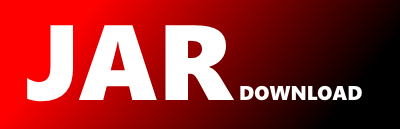
org.apache.camel.language.simple.SimpleLanguage Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.camel.language.simple;
import java.util.Map;
import org.apache.camel.Expression;
import org.apache.camel.Predicate;
import org.apache.camel.StaticService;
import org.apache.camel.spi.annotations.Language;
import org.apache.camel.support.CamelContextHelper;
import org.apache.camel.support.LRUCache;
import org.apache.camel.support.LRUCacheFactory;
import org.apache.camel.support.LanguageSupport;
import org.apache.camel.support.PredicateToExpressionAdapter;
import org.apache.camel.support.builder.ExpressionBuilder;
import org.apache.camel.util.ObjectHelper;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* A simple language
* which maps simple property style notations to access headers and bodies.
* Examples of supported expressions are:
*
* - exchangeId to access the exchange id
* - id to access the inbound message id
* - in.body or body to access the inbound body
* - in.body.OGNL or body.OGNL to access the inbound body using an OGNL expression
* - mandatoryBodyAs(<classname>) to convert the in body to the given type, will throw exception if not possible to convert
* - bodyAs(<classname>) to convert the in body to the given type, will return null if not possible to convert
* - headerAs(<key>, <classname>) to convert the in header to the given type, will return null if not possible to convert
* - out.body to access the inbound body
* - in.header.foo or header.foo to access an inbound header called 'foo'
* - in.header.foo[bar] or header.foo[bar] to access an inbound header called 'foo' as a Map and lookup the map with 'bar' as key
* - in.header.foo.OGNL or header.OGNL to access an inbound header called 'foo' using an OGNL expression
* - out.header.foo to access an outbound header called 'foo'
* - property.foo to access the exchange property called 'foo'
* - property.foo.OGNL to access the exchange property called 'foo' using an OGNL expression
* - sys.foo to access the system property called 'foo'
* - sysenv.foo to access the system environment called 'foo'
* - exception.messsage to access the exception message
* - threadName to access the current thread name
* - date:<command> evaluates to a Date object
* Supported commands are: now for current timestamp,
* in.header.xxx or header.xxx to use the Date object in the in header.
* out.header.xxx to use the Date object in the out header.
* property.xxx to use the Date object in the exchange property.
* file for the last modified timestamp of the file (available with a File consumer).
* Command accepts offsets such as: now-24h or in.header.xxx+1h or even now+1h30m-100.
*
* - date:<command>:<pattern> for date formatting using {@link java.text.SimpleDateFormat} patterns
* - date-with-timezone:<command>:<timezone>:<pattern> for date formatting using {@link java.text.SimpleDateFormat} timezones and patterns
* - bean:<bean expression> to invoke a bean using the
* {@link org.apache.camel.language.bean.BeanLanguage BeanLanguage}
* - properties:<[locations]>:<key> for using property placeholders using the
* {@link org.apache.camel.component.properties.PropertiesComponent}.
* The locations parameter is optional and you can enter multiple locations separated with comma.
*
*
*
* The simple language supports OGNL notation when accessing either body or header.
*
* The simple language now also includes file language out of the box which means the following expression is also
* supported:
*
* - file:name to access the file name (is relative, see note below))
* - file:name.noext to access the file name with no extension
* - file:name.ext to access the file extension
* - file:ext to access the file extension
* - file:onlyname to access the file name (no paths)
* - file:onlyname.noext to access the file name (no paths) with no extension
* - file:parent to access the parent file name
* - file:path to access the file path name
* - file:absolute is the file regarded as absolute or relative
* - file:absolute.path to access the absolute file path name
* - file:length to access the file length as a Long type
* - file:size to access the file length as a Long type
* - file:modified to access the file last modified as a Date type
*
* The relative file is the filename with the starting directory clipped, as opposed to path that will
* return the full path including the starting directory.
*
* The only file is the filename only with all paths clipped.
*/
@Language("simple")
public class SimpleLanguage extends LanguageSupport implements StaticService {
private static final Logger LOG = LoggerFactory.getLogger(SimpleLanguage.class);
// singleton for expressions without a result type
private static final SimpleLanguage SIMPLE = new SimpleLanguage();
boolean allowEscape = true;
// use caches to avoid re-parsing the same expressions over and over again
private Map cacheExpression;
private Map cachePredicate;
/**
* Default constructor.
*/
public SimpleLanguage() {
}
@Override
public void init() {
// setup cache which requires CamelContext to be set first
if (cacheExpression == null && cachePredicate == null && getCamelContext() != null) {
int maxSize = CamelContextHelper.getMaximumSimpleCacheSize(getCamelContext());
if (maxSize > 0) {
cacheExpression = LRUCacheFactory.newLRUCache(16, maxSize, false);
cachePredicate = LRUCacheFactory.newLRUCache(16, maxSize, false);
LOG.debug("Simple language predicate/expression cache size: {}", maxSize);
} else {
LOG.debug("Simple language disabled predicate/expression cache");
}
}
}
@Override
public void start() {
// noop
}
@Override
public void stop() {
if (cachePredicate instanceof LRUCache) {
if (LOG.isDebugEnabled()) {
LRUCache cache = (LRUCache) cachePredicate;
LOG.debug("Clearing simple language predicate cache[size={}, hits={}, misses={}, evicted={}]",
cache.size(), cache.getHits(), cache.getMisses(), cache.getEvicted());
}
}
if (cacheExpression instanceof LRUCache) {
if (LOG.isDebugEnabled()) {
LRUCache cache = (LRUCache) cacheExpression;
LOG.debug("Clearing simple language expression cache[size={}, hits={}, misses={}, evicted={}]",
cache.size(), cache.getHits(), cache.getMisses(), cache.getEvicted());
}
}
}
@Override
public Predicate createPredicate(String expression) {
ObjectHelper.notNull(expression, "expression");
Predicate answer = cachePredicate != null ? cachePredicate.get(expression) : null;
if (answer == null) {
expression = loadResource(expression);
SimplePredicateParser parser = new SimplePredicateParser(expression, allowEscape, cacheExpression);
answer = parser.parsePredicate();
if (cachePredicate != null && answer != null) {
cachePredicate.put(expression, answer);
}
}
return answer;
}
@Override
public Expression createExpression(String expression) {
ObjectHelper.notNull(expression, "expression");
Expression answer = cacheExpression != null ? cacheExpression.get(expression) : null;
if (answer == null) {
expression = loadResource(expression);
SimpleExpressionParser parser = new SimpleExpressionParser(expression, allowEscape, cacheExpression);
answer = parser.parseExpression();
if (cacheExpression != null && answer != null) {
cacheExpression.put(expression, answer);
}
}
return answer;
}
/**
* Creates a new {@link Expression}.
*
* Important: If you need to use a predicate (function to return true|false) then use
* {@link #predicate(String)} instead.
*/
public static Expression simple(String expression) {
return expression(expression);
}
/**
* Creates a new {@link Expression} (or {@link Predicate}
* if the resultType is a Boolean, or boolean type).
*/
public static Expression simple(String expression, Class> resultType) {
return new SimpleLanguage().createExpression(expression, resultType);
}
public Expression createExpression(String expression, Class> resultType) {
if (resultType == Boolean.class || resultType == boolean.class) {
// if its a boolean as result then its a predicate
Predicate predicate = createPredicate(expression);
return PredicateToExpressionAdapter.toExpression(predicate);
} else {
Expression exp = createExpression(expression);
if (resultType != null) {
exp = ExpressionBuilder.convertToExpression(exp, resultType);
}
return exp;
}
}
/**
* Creates a new {@link Expression}.
*
* Important: If you need to use a predicate (function to return true|false) then use
* {@link #predicate(String)} instead.
*/
public static Expression expression(String expression) {
return SIMPLE.createExpression(expression);
}
/**
* Creates a new {@link Predicate}.
*/
public static Predicate predicate(String predicate) {
return SIMPLE.createPredicate(predicate);
}
/**
* Does the expression include a simple function.
*
* @param expression the expression
* @return true if one or more simple function is included in the expression
*/
public static boolean hasSimpleFunction(String expression) {
return SimpleTokenizer.hasFunctionStartToken(expression);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy