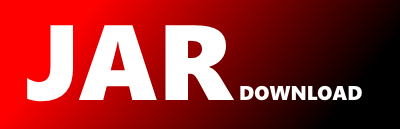
org.apache.camel.component.fhir.FhirReadEndpointConfiguration Maven / Gradle / Ivy
The newest version!
/*
* Camel EndpointConfiguration generated by camel-api-component-maven-plugin
*/
package org.apache.camel.component.fhir;
import org.apache.camel.spi.ApiMethod;
import org.apache.camel.spi.ApiParam;
import org.apache.camel.spi.ApiParams;
import org.apache.camel.spi.Configurer;
import org.apache.camel.spi.UriParam;
import org.apache.camel.spi.UriParams;
/**
* Camel endpoint configuration for {@link org.apache.camel.component.fhir.api.FhirRead}.
*/
@ApiParams(apiName = "read",
description = "API method for read operations",
apiMethods = {@ApiMethod(methodName = "resourceById", description="Reads a IBaseResource on the server by id", signatures={"org.hl7.fhir.instance.model.api.IBaseResource resourceById(Class resource, org.hl7.fhir.instance.model.api.IIdType id, String ifVersionMatches, Boolean returnNull, org.hl7.fhir.instance.model.api.IBaseResource returnResource, Boolean throwError, java.util.Map extraParameters)", "org.hl7.fhir.instance.model.api.IBaseResource resourceById(Class resource, Long longId, String ifVersionMatches, Boolean returnNull, org.hl7.fhir.instance.model.api.IBaseResource returnResource, Boolean throwError, java.util.Map extraParameters)", "org.hl7.fhir.instance.model.api.IBaseResource resourceById(String resourceClass, org.hl7.fhir.instance.model.api.IIdType id, String ifVersionMatches, Boolean returnNull, org.hl7.fhir.instance.model.api.IBaseResource returnResource, Boolean throwError, java.util.Map extraParameters)", "org.hl7.fhir.instance.model.api.IBaseResource resourceById(String resourceClass, Long longId, String ifVersionMatches, Boolean returnNull, org.hl7.fhir.instance.model.api.IBaseResource returnResource, Boolean throwError, java.util.Map extraParameters)", "org.hl7.fhir.instance.model.api.IBaseResource resourceById(Class resource, String stringId, String version, String ifVersionMatches, Boolean returnNull, org.hl7.fhir.instance.model.api.IBaseResource returnResource, Boolean throwError, java.util.Map extraParameters)", "org.hl7.fhir.instance.model.api.IBaseResource resourceById(String resourceClass, String stringId, String ifVersionMatches, String version, Boolean returnNull, org.hl7.fhir.instance.model.api.IBaseResource returnResource, Boolean throwError, java.util.Map extraParameters)"}), @ApiMethod(methodName = "resourceByUrl", description="Reads a IBaseResource on the server by url", signatures={"org.hl7.fhir.instance.model.api.IBaseResource resourceByUrl(Class resource, org.hl7.fhir.instance.model.api.IIdType iUrl, String ifVersionMatches, Boolean returnNull, org.hl7.fhir.instance.model.api.IBaseResource returnResource, Boolean throwError, java.util.Map extraParameters)", "org.hl7.fhir.instance.model.api.IBaseResource resourceByUrl(Class resource, String url, String ifVersionMatches, Boolean returnNull, org.hl7.fhir.instance.model.api.IBaseResource returnResource, Boolean throwError, java.util.Map extraParameters)", "org.hl7.fhir.instance.model.api.IBaseResource resourceByUrl(String resourceClass, org.hl7.fhir.instance.model.api.IIdType iUrl, String ifVersionMatches, Boolean returnNull, org.hl7.fhir.instance.model.api.IBaseResource returnResource, Boolean throwError, java.util.Map extraParameters)", "org.hl7.fhir.instance.model.api.IBaseResource resourceByUrl(String resourceClass, String url, String ifVersionMatches, Boolean returnNull, org.hl7.fhir.instance.model.api.IBaseResource returnResource, Boolean throwError, java.util.Map extraParameters)"}), }, aliases = {})
@UriParams
@Configurer(extended = true)
public final class FhirReadEndpointConfiguration extends FhirConfiguration {
@UriParam
@ApiParam(optional = true, apiMethods = {@ApiMethod(methodName = "resourceById", description="See ExtraParameters for a full list of parameters that can be passed, may be NULL"), @ApiMethod(methodName = "resourceById", description="See ExtraParameters for a full list of parameters that can be passed, may be NULL"), @ApiMethod(methodName = "resourceById", description="See ExtraParameters for a full list of parameters that can be passed, may be NULL"), @ApiMethod(methodName = "resourceById", description="See ExtraParameters for a full list of parameters that can be passed, may be NULL"), @ApiMethod(methodName = "resourceById", description="See ExtraParameters for a full list of parameters that can be passed, may be NULL"), @ApiMethod(methodName = "resourceById", description="See ExtraParameters for a full list of parameters that can be passed, may be NULL"), @ApiMethod(methodName = "resourceByUrl", description="See ExtraParameters for a full list of parameters that can be passed, may be NULL"), @ApiMethod(methodName = "resourceByUrl", description="See ExtraParameters for a full list of parameters that can be passed, may be NULL"), @ApiMethod(methodName = "resourceByUrl", description="See ExtraParameters for a full list of parameters that can be passed, may be NULL"), @ApiMethod(methodName = "resourceByUrl", description="See ExtraParameters for a full list of parameters that can be passed, may be NULL")})
private java.util.Map extraParameters;
@UriParam
@ApiParam(optional = false, apiMethods = {@ApiMethod(methodName = "resourceByUrl", description="The IIdType referencing the resource by absolute url")})
private org.hl7.fhir.instance.model.api.IIdType iUrl;
@UriParam
@ApiParam(optional = false, apiMethods = {@ApiMethod(methodName = "resourceById", description="The IIdType referencing the resource")})
private org.hl7.fhir.instance.model.api.IIdType id;
@UriParam
@ApiParam(optional = true, apiMethods = {@ApiMethod(methodName = "resourceById", description="A version to match against the newest version on the server"), @ApiMethod(methodName = "resourceById", description="A version to match against the newest version on the server"), @ApiMethod(methodName = "resourceById", description="A version to match against the newest version on the server"), @ApiMethod(methodName = "resourceById", description="A version to match against the newest version on the server"), @ApiMethod(methodName = "resourceById", description="A version to match against the newest version on the server"), @ApiMethod(methodName = "resourceById", description="A version to match against the newest version on the server"), @ApiMethod(methodName = "resourceByUrl", description="A version to match against the newest version on the server"), @ApiMethod(methodName = "resourceByUrl", description="A version to match against the newest version on the server"), @ApiMethod(methodName = "resourceByUrl", description="A version to match against the newest version on the server"), @ApiMethod(methodName = "resourceByUrl", description="A version to match against the newest version on the server")})
private String ifVersionMatches;
@UriParam
@ApiParam(optional = false, apiMethods = {@ApiMethod(methodName = "resourceById", description="The resource ID")})
private Long longId;
@UriParam
@ApiParam(optional = false, apiMethods = {@ApiMethod(methodName = "resourceById", description="The resource to read (e.g. Patient)"), @ApiMethod(methodName = "resourceByUrl", description="The resource to read (e.g. Patient)")})
private Class resource;
@UriParam
@ApiParam(optional = false, apiMethods = {@ApiMethod(methodName = "resourceById", description="The resource to read (e.g. Patient)"), @ApiMethod(methodName = "resourceByUrl", description="The resource to read (e.g. Patient.class)")})
private String resourceClass;
@UriParam
@ApiParam(optional = true, apiMethods = {@ApiMethod(methodName = "resourceById", description="Return null if version matches"), @ApiMethod(methodName = "resourceById", description="Return null if version matches"), @ApiMethod(methodName = "resourceById", description="Return null if version matches"), @ApiMethod(methodName = "resourceById", description="Return null if version matches"), @ApiMethod(methodName = "resourceById", description="Return null if version matches"), @ApiMethod(methodName = "resourceById", description="Return null if version matches"), @ApiMethod(methodName = "resourceByUrl", description="Return null if version matches"), @ApiMethod(methodName = "resourceByUrl", description="Return null if version matches"), @ApiMethod(methodName = "resourceByUrl", description="Return null if version matches"), @ApiMethod(methodName = "resourceByUrl", description="Return null if version matches")})
private Boolean returnNull;
@UriParam
@ApiParam(optional = true, apiMethods = {@ApiMethod(methodName = "resourceById", description="Return the resource if version matches"), @ApiMethod(methodName = "resourceById", description="Return the resource if version matches"), @ApiMethod(methodName = "resourceById", description="Return the resource if version matches"), @ApiMethod(methodName = "resourceById", description="Return the resource if version matches"), @ApiMethod(methodName = "resourceById", description="Return the resource if version matches"), @ApiMethod(methodName = "resourceById", description="Return the resource if version matches"), @ApiMethod(methodName = "resourceByUrl", description="Return the resource if version matches"), @ApiMethod(methodName = "resourceByUrl", description="Return the resource if version matches"), @ApiMethod(methodName = "resourceByUrl", description="Return the resource if version matches"), @ApiMethod(methodName = "resourceByUrl", description="Return the resource if version matches")})
private org.hl7.fhir.instance.model.api.IBaseResource returnResource;
@UriParam
@ApiParam(optional = false, apiMethods = {@ApiMethod(methodName = "resourceById", description="The resource ID")})
private String stringId;
@UriParam
@ApiParam(optional = true, apiMethods = {@ApiMethod(methodName = "resourceById", description="Throw error if the version matches"), @ApiMethod(methodName = "resourceById", description="Throw error if the version matches"), @ApiMethod(methodName = "resourceById", description="Throw error if the version matches"), @ApiMethod(methodName = "resourceById", description="Throw error if the version matches"), @ApiMethod(methodName = "resourceById", description="Throw error if the version matches"), @ApiMethod(methodName = "resourceById", description="Throw error if the version matches"), @ApiMethod(methodName = "resourceByUrl", description="Throw error if the version matches"), @ApiMethod(methodName = "resourceByUrl", description="Throw error if the version matches"), @ApiMethod(methodName = "resourceByUrl", description="Throw error if the version matches"), @ApiMethod(methodName = "resourceByUrl", description="Throw error if the version matches")})
private Boolean throwError;
@UriParam
@ApiParam(optional = false, apiMethods = {@ApiMethod(methodName = "resourceByUrl", description="Referencing the resource by absolute url")})
private String url;
@UriParam
@ApiParam(optional = true, apiMethods = {@ApiMethod(methodName = "resourceById", description="The resource version")})
private String version;
public java.util.Map getExtraParameters() {
return extraParameters;
}
public void setExtraParameters(java.util.Map extraParameters) {
this.extraParameters = extraParameters;
}
public org.hl7.fhir.instance.model.api.IIdType getIUrl() {
return iUrl;
}
public void setIUrl(org.hl7.fhir.instance.model.api.IIdType iUrl) {
this.iUrl = iUrl;
}
public org.hl7.fhir.instance.model.api.IIdType getId() {
return id;
}
public void setId(org.hl7.fhir.instance.model.api.IIdType id) {
this.id = id;
}
public String getIfVersionMatches() {
return ifVersionMatches;
}
public void setIfVersionMatches(String ifVersionMatches) {
this.ifVersionMatches = ifVersionMatches;
}
public Long getLongId() {
return longId;
}
public void setLongId(Long longId) {
this.longId = longId;
}
public Class getResource() {
return resource;
}
public void setResource(Class resource) {
this.resource = resource;
}
public String getResourceClass() {
return resourceClass;
}
public void setResourceClass(String resourceClass) {
this.resourceClass = resourceClass;
}
public Boolean getReturnNull() {
return returnNull;
}
public void setReturnNull(Boolean returnNull) {
this.returnNull = returnNull;
}
public org.hl7.fhir.instance.model.api.IBaseResource getReturnResource() {
return returnResource;
}
public void setReturnResource(org.hl7.fhir.instance.model.api.IBaseResource returnResource) {
this.returnResource = returnResource;
}
public String getStringId() {
return stringId;
}
public void setStringId(String stringId) {
this.stringId = stringId;
}
public Boolean getThrowError() {
return throwError;
}
public void setThrowError(Boolean throwError) {
this.throwError = throwError;
}
public String getUrl() {
return url;
}
public void setUrl(String url) {
this.url = url;
}
public String getVersion() {
return version;
}
public void setVersion(String version) {
this.version = version;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy