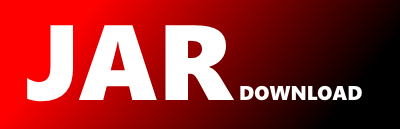
org.apache.camel.component.http.springboot.HttpComponentConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of camel-http-starter Show documentation
Show all versions of camel-http-starter Show documentation
Spring-Boot Starter for Camel HTTP support
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.camel.component.http.springboot;
import javax.annotation.Generated;
import org.apache.camel.spring.boot.ComponentConfigurationPropertiesCommon;
import org.springframework.boot.context.properties.ConfigurationProperties;
/**
* For calling out to external HTTP servers using Apache HTTP Client 4.x.
*
* Generated by camel-package-maven-plugin - do not edit this file!
*/
@Generated("org.apache.camel.maven.packaging.SpringBootAutoConfigurationMojo")
@ConfigurationProperties(prefix = "camel.component.http")
public class HttpComponentConfiguration
extends
ComponentConfigurationPropertiesCommon {
/**
* Whether to enable auto configuration of the http component. This is
* enabled by default.
*/
private Boolean enabled;
/**
* To use the custom HttpClientConfigurer to perform configuration of the
* HttpClient that will be used. The option is a
* org.apache.camel.component.http.HttpClientConfigurer type.
*/
private String httpClientConfigurer;
/**
* To use a custom and shared HttpClientConnectionManager to manage
* connections. If this has been configured then this is always used for all
* endpoints created by this component. The option is a
* org.apache.http.conn.HttpClientConnectionManager type.
*/
private String clientConnectionManager;
/**
* To use a custom org.apache.http.protocol.HttpContext when executing
* requests. The option is a org.apache.http.protocol.HttpContext type.
*/
private String httpContext;
/**
* To configure security using SSLContextParameters. Important: Only one
* instance of org.apache.camel.support.jsse.SSLContextParameters is
* supported per HttpComponent. If you need to use 2 or more different
* instances, you need to define a new HttpComponent per instance you need.
* The option is a org.apache.camel.support.jsse.SSLContextParameters type.
*/
private String sslContextParameters;
/**
* Enable usage of global SSL context parameters.
*/
private Boolean useGlobalSslContextParameters = false;
/**
* To use a custom X509HostnameVerifier such as DefaultHostnameVerifier or
* NoopHostnameVerifier. The option is a javax.net.ssl.HostnameVerifier
* type.
*/
private String x509HostnameVerifier;
/**
* The maximum number of connections.
*/
private Integer maxTotalConnections = 200;
/**
* The maximum number of connections per route.
*/
private Integer connectionsPerRoute = 20;
/**
* The time for connection to live, the time unit is millisecond, the
* default value is always keep alive.
*/
private Long connectionTimeToLive;
/**
* To use a custom org.apache.http.client.CookieStore. By default the
* org.apache.http.impl.client.BasicCookieStore is used which is an
* in-memory only cookie store. Notice if bridgeEndpoint=true then the
* cookie store is forced to be a noop cookie store as cookie shouldn't be
* stored as we are just bridging (eg acting as a proxy). The option is a
* org.apache.http.client.CookieStore type.
*/
private String cookieStore;
/**
* The timeout in milliseconds used when requesting a connection from the
* connection manager. A timeout value of zero is interpreted as an infinite
* timeout. A timeout value of zero is interpreted as an infinite timeout. A
* negative value is interpreted as undefined (system default). Default: -1
*/
private Integer connectionRequestTimeout = -1;
/**
* Determines the timeout in milliseconds until a connection is established.
* A timeout value of zero is interpreted as an infinite timeout. A timeout
* value of zero is interpreted as an infinite timeout. A negative value is
* interpreted as undefined (system default). Default: -1
*/
private Integer connectTimeout = -1;
/**
* Defines the socket timeout (SO_TIMEOUT) in milliseconds, which is the
* timeout for waiting for data or, put differently, a maximum period
* inactivity between two consecutive data packets). A timeout value of zero
* is interpreted as an infinite timeout. A negative value is interpreted as
* undefined (system default). Default: -1
*/
private Integer socketTimeout = -1;
/**
* To use a custom HttpBinding to control the mapping between Camel message
* and HttpClient. The option is a org.apache.camel.http.common.HttpBinding
* type.
*/
private String httpBinding;
/**
* To use the shared HttpConfiguration as base configuration. The option is
* a org.apache.camel.http.common.HttpConfiguration type.
*/
private String httpConfiguration;
/**
* Whether to allow java serialization when a request uses
* context-type=application/x-java-serialized-object. This is by default
* turned off. If you enable this then be aware that Java will deserialize
* the incoming data from the request to Java and that can be a potential
* security risk.
*/
private Boolean allowJavaSerializedObject = false;
/**
* To use a custom org.apache.camel.spi.HeaderFilterStrategy to filter
* header to and from Camel message. The option is a
* org.apache.camel.spi.HeaderFilterStrategy type.
*/
private String headerFilterStrategy;
/**
* Whether the component should use basic property binding (Camel 2.x) or
* the newer property binding with additional capabilities
*/
private Boolean basicPropertyBinding = false;
public String getHttpClientConfigurer() {
return httpClientConfigurer;
}
public void setHttpClientConfigurer(String httpClientConfigurer) {
this.httpClientConfigurer = httpClientConfigurer;
}
public String getClientConnectionManager() {
return clientConnectionManager;
}
public void setClientConnectionManager(String clientConnectionManager) {
this.clientConnectionManager = clientConnectionManager;
}
public String getHttpContext() {
return httpContext;
}
public void setHttpContext(String httpContext) {
this.httpContext = httpContext;
}
public String getSslContextParameters() {
return sslContextParameters;
}
public void setSslContextParameters(String sslContextParameters) {
this.sslContextParameters = sslContextParameters;
}
public Boolean getUseGlobalSslContextParameters() {
return useGlobalSslContextParameters;
}
public void setUseGlobalSslContextParameters(
Boolean useGlobalSslContextParameters) {
this.useGlobalSslContextParameters = useGlobalSslContextParameters;
}
public String getX509HostnameVerifier() {
return x509HostnameVerifier;
}
public void setX509HostnameVerifier(String x509HostnameVerifier) {
this.x509HostnameVerifier = x509HostnameVerifier;
}
public Integer getMaxTotalConnections() {
return maxTotalConnections;
}
public void setMaxTotalConnections(Integer maxTotalConnections) {
this.maxTotalConnections = maxTotalConnections;
}
public Integer getConnectionsPerRoute() {
return connectionsPerRoute;
}
public void setConnectionsPerRoute(Integer connectionsPerRoute) {
this.connectionsPerRoute = connectionsPerRoute;
}
public Long getConnectionTimeToLive() {
return connectionTimeToLive;
}
public void setConnectionTimeToLive(Long connectionTimeToLive) {
this.connectionTimeToLive = connectionTimeToLive;
}
public String getCookieStore() {
return cookieStore;
}
public void setCookieStore(String cookieStore) {
this.cookieStore = cookieStore;
}
public Integer getConnectionRequestTimeout() {
return connectionRequestTimeout;
}
public void setConnectionRequestTimeout(Integer connectionRequestTimeout) {
this.connectionRequestTimeout = connectionRequestTimeout;
}
public Integer getConnectTimeout() {
return connectTimeout;
}
public void setConnectTimeout(Integer connectTimeout) {
this.connectTimeout = connectTimeout;
}
public Integer getSocketTimeout() {
return socketTimeout;
}
public void setSocketTimeout(Integer socketTimeout) {
this.socketTimeout = socketTimeout;
}
public String getHttpBinding() {
return httpBinding;
}
public void setHttpBinding(String httpBinding) {
this.httpBinding = httpBinding;
}
public String getHttpConfiguration() {
return httpConfiguration;
}
public void setHttpConfiguration(String httpConfiguration) {
this.httpConfiguration = httpConfiguration;
}
public Boolean getAllowJavaSerializedObject() {
return allowJavaSerializedObject;
}
public void setAllowJavaSerializedObject(Boolean allowJavaSerializedObject) {
this.allowJavaSerializedObject = allowJavaSerializedObject;
}
public String getHeaderFilterStrategy() {
return headerFilterStrategy;
}
public void setHeaderFilterStrategy(String headerFilterStrategy) {
this.headerFilterStrategy = headerFilterStrategy;
}
public Boolean getBasicPropertyBinding() {
return basicPropertyBinding;
}
public void setBasicPropertyBinding(Boolean basicPropertyBinding) {
this.basicPropertyBinding = basicPropertyBinding;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy