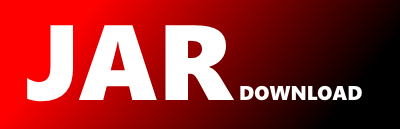
org.apache.camel.component.http.HttpComponentConfigurer Maven / Gradle / Ivy
The newest version!
/* Generated by camel build tools - do NOT edit this file! */
package org.apache.camel.component.http;
import javax.annotation.processing.Generated;
import java.util.Map;
import org.apache.camel.CamelContext;
import org.apache.camel.spi.ExtendedPropertyConfigurerGetter;
import org.apache.camel.spi.PropertyConfigurerGetter;
import org.apache.camel.spi.ConfigurerStrategy;
import org.apache.camel.spi.GeneratedPropertyConfigurer;
import org.apache.camel.util.CaseInsensitiveMap;
import org.apache.camel.support.component.PropertyConfigurerSupport;
/**
* Generated by camel build tools - do NOT edit this file!
*/
@Generated("org.apache.camel.maven.packaging.EndpointSchemaGeneratorMojo")
@SuppressWarnings("unchecked")
public class HttpComponentConfigurer extends PropertyConfigurerSupport implements GeneratedPropertyConfigurer, PropertyConfigurerGetter {
@Override
public boolean configure(CamelContext camelContext, Object obj, String name, Object value, boolean ignoreCase) {
HttpComponent target = (HttpComponent) obj;
switch (ignoreCase ? name.toLowerCase() : name) {
case "allowjavaserializedobject":
case "allowJavaSerializedObject": target.setAllowJavaSerializedObject(property(camelContext, boolean.class, value)); return true;
case "authcachingdisabled":
case "authCachingDisabled": target.setAuthCachingDisabled(property(camelContext, boolean.class, value)); return true;
case "automaticretriesdisabled":
case "automaticRetriesDisabled": target.setAutomaticRetriesDisabled(property(camelContext, boolean.class, value)); return true;
case "autowiredenabled":
case "autowiredEnabled": target.setAutowiredEnabled(property(camelContext, boolean.class, value)); return true;
case "clientconnectionmanager":
case "clientConnectionManager": target.setClientConnectionManager(property(camelContext, org.apache.hc.client5.http.io.HttpClientConnectionManager.class, value)); return true;
case "connecttimeout":
case "connectTimeout": target.setConnectTimeout(property(camelContext, org.apache.hc.core5.util.Timeout.class, value)); return true;
case "connectionrequesttimeout":
case "connectionRequestTimeout": target.setConnectionRequestTimeout(property(camelContext, org.apache.hc.core5.util.Timeout.class, value)); return true;
case "connectionstatedisabled":
case "connectionStateDisabled": target.setConnectionStateDisabled(property(camelContext, boolean.class, value)); return true;
case "connectiontimetolive":
case "connectionTimeToLive": target.setConnectionTimeToLive(property(camelContext, long.class, value)); return true;
case "connectionsperroute":
case "connectionsPerRoute": target.setConnectionsPerRoute(property(camelContext, int.class, value)); return true;
case "contentcompressiondisabled":
case "contentCompressionDisabled": target.setContentCompressionDisabled(property(camelContext, boolean.class, value)); return true;
case "cookiemanagementdisabled":
case "cookieManagementDisabled": target.setCookieManagementDisabled(property(camelContext, boolean.class, value)); return true;
case "cookiestore":
case "cookieStore": target.setCookieStore(property(camelContext, org.apache.hc.client5.http.cookie.CookieStore.class, value)); return true;
case "copyheaders":
case "copyHeaders": target.setCopyHeaders(property(camelContext, boolean.class, value)); return true;
case "defaultuseragentdisabled":
case "defaultUserAgentDisabled": target.setDefaultUserAgentDisabled(property(camelContext, boolean.class, value)); return true;
case "followredirects":
case "followRedirects": target.setFollowRedirects(property(camelContext, boolean.class, value)); return true;
case "headerfilterstrategy":
case "headerFilterStrategy": target.setHeaderFilterStrategy(property(camelContext, org.apache.camel.spi.HeaderFilterStrategy.class, value)); return true;
case "httpbinding":
case "httpBinding": target.setHttpBinding(property(camelContext, org.apache.camel.http.common.HttpBinding.class, value)); return true;
case "httpclientconfigurer":
case "httpClientConfigurer": target.setHttpClientConfigurer(property(camelContext, org.apache.camel.component.http.HttpClientConfigurer.class, value)); return true;
case "httpconfiguration":
case "httpConfiguration": target.setHttpConfiguration(property(camelContext, org.apache.camel.http.common.HttpConfiguration.class, value)); return true;
case "httpcontext":
case "httpContext": target.setHttpContext(property(camelContext, org.apache.hc.core5.http.protocol.HttpContext.class, value)); return true;
case "lazystartproducer":
case "lazyStartProducer": target.setLazyStartProducer(property(camelContext, boolean.class, value)); return true;
case "maxtotalconnections":
case "maxTotalConnections": target.setMaxTotalConnections(property(camelContext, int.class, value)); return true;
case "proxyauthdomain":
case "proxyAuthDomain": target.setProxyAuthDomain(property(camelContext, java.lang.String.class, value)); return true;
case "proxyauthhost":
case "proxyAuthHost": target.setProxyAuthHost(property(camelContext, java.lang.String.class, value)); return true;
case "proxyauthmethod":
case "proxyAuthMethod": target.setProxyAuthMethod(property(camelContext, java.lang.String.class, value)); return true;
case "proxyauthnthost":
case "proxyAuthNtHost": target.setProxyAuthNtHost(property(camelContext, java.lang.String.class, value)); return true;
case "proxyauthpassword":
case "proxyAuthPassword": target.setProxyAuthPassword(property(camelContext, java.lang.String.class, value)); return true;
case "proxyauthport":
case "proxyAuthPort": target.setProxyAuthPort(property(camelContext, java.lang.Integer.class, value)); return true;
case "proxyauthscheme":
case "proxyAuthScheme": target.setProxyAuthScheme(property(camelContext, java.lang.String.class, value)); return true;
case "proxyauthusername":
case "proxyAuthUsername": target.setProxyAuthUsername(property(camelContext, java.lang.String.class, value)); return true;
case "redirecthandlingdisabled":
case "redirectHandlingDisabled": target.setRedirectHandlingDisabled(property(camelContext, boolean.class, value)); return true;
case "responsepayloadstreamingthreshold":
case "responsePayloadStreamingThreshold": target.setResponsePayloadStreamingThreshold(property(camelContext, int.class, value)); return true;
case "responsetimeout":
case "responseTimeout": target.setResponseTimeout(property(camelContext, org.apache.hc.core5.util.Timeout.class, value)); return true;
case "skiprequestheaders":
case "skipRequestHeaders": target.setSkipRequestHeaders(property(camelContext, boolean.class, value)); return true;
case "skipresponseheaders":
case "skipResponseHeaders": target.setSkipResponseHeaders(property(camelContext, boolean.class, value)); return true;
case "sotimeout":
case "soTimeout": target.setSoTimeout(property(camelContext, org.apache.hc.core5.util.Timeout.class, value)); return true;
case "sslcontextparameters":
case "sslContextParameters": target.setSslContextParameters(property(camelContext, org.apache.camel.support.jsse.SSLContextParameters.class, value)); return true;
case "useglobalsslcontextparameters":
case "useGlobalSslContextParameters": target.setUseGlobalSslContextParameters(property(camelContext, boolean.class, value)); return true;
case "x509hostnameverifier":
case "x509HostnameVerifier": target.setX509HostnameVerifier(property(camelContext, javax.net.ssl.HostnameVerifier.class, value)); return true;
default: return false;
}
}
@Override
public Class> getOptionType(String name, boolean ignoreCase) {
switch (ignoreCase ? name.toLowerCase() : name) {
case "allowjavaserializedobject":
case "allowJavaSerializedObject": return boolean.class;
case "authcachingdisabled":
case "authCachingDisabled": return boolean.class;
case "automaticretriesdisabled":
case "automaticRetriesDisabled": return boolean.class;
case "autowiredenabled":
case "autowiredEnabled": return boolean.class;
case "clientconnectionmanager":
case "clientConnectionManager": return org.apache.hc.client5.http.io.HttpClientConnectionManager.class;
case "connecttimeout":
case "connectTimeout": return org.apache.hc.core5.util.Timeout.class;
case "connectionrequesttimeout":
case "connectionRequestTimeout": return org.apache.hc.core5.util.Timeout.class;
case "connectionstatedisabled":
case "connectionStateDisabled": return boolean.class;
case "connectiontimetolive":
case "connectionTimeToLive": return long.class;
case "connectionsperroute":
case "connectionsPerRoute": return int.class;
case "contentcompressiondisabled":
case "contentCompressionDisabled": return boolean.class;
case "cookiemanagementdisabled":
case "cookieManagementDisabled": return boolean.class;
case "cookiestore":
case "cookieStore": return org.apache.hc.client5.http.cookie.CookieStore.class;
case "copyheaders":
case "copyHeaders": return boolean.class;
case "defaultuseragentdisabled":
case "defaultUserAgentDisabled": return boolean.class;
case "followredirects":
case "followRedirects": return boolean.class;
case "headerfilterstrategy":
case "headerFilterStrategy": return org.apache.camel.spi.HeaderFilterStrategy.class;
case "httpbinding":
case "httpBinding": return org.apache.camel.http.common.HttpBinding.class;
case "httpclientconfigurer":
case "httpClientConfigurer": return org.apache.camel.component.http.HttpClientConfigurer.class;
case "httpconfiguration":
case "httpConfiguration": return org.apache.camel.http.common.HttpConfiguration.class;
case "httpcontext":
case "httpContext": return org.apache.hc.core5.http.protocol.HttpContext.class;
case "lazystartproducer":
case "lazyStartProducer": return boolean.class;
case "maxtotalconnections":
case "maxTotalConnections": return int.class;
case "proxyauthdomain":
case "proxyAuthDomain": return java.lang.String.class;
case "proxyauthhost":
case "proxyAuthHost": return java.lang.String.class;
case "proxyauthmethod":
case "proxyAuthMethod": return java.lang.String.class;
case "proxyauthnthost":
case "proxyAuthNtHost": return java.lang.String.class;
case "proxyauthpassword":
case "proxyAuthPassword": return java.lang.String.class;
case "proxyauthport":
case "proxyAuthPort": return java.lang.Integer.class;
case "proxyauthscheme":
case "proxyAuthScheme": return java.lang.String.class;
case "proxyauthusername":
case "proxyAuthUsername": return java.lang.String.class;
case "redirecthandlingdisabled":
case "redirectHandlingDisabled": return boolean.class;
case "responsepayloadstreamingthreshold":
case "responsePayloadStreamingThreshold": return int.class;
case "responsetimeout":
case "responseTimeout": return org.apache.hc.core5.util.Timeout.class;
case "skiprequestheaders":
case "skipRequestHeaders": return boolean.class;
case "skipresponseheaders":
case "skipResponseHeaders": return boolean.class;
case "sotimeout":
case "soTimeout": return org.apache.hc.core5.util.Timeout.class;
case "sslcontextparameters":
case "sslContextParameters": return org.apache.camel.support.jsse.SSLContextParameters.class;
case "useglobalsslcontextparameters":
case "useGlobalSslContextParameters": return boolean.class;
case "x509hostnameverifier":
case "x509HostnameVerifier": return javax.net.ssl.HostnameVerifier.class;
default: return null;
}
}
@Override
public Object getOptionValue(Object obj, String name, boolean ignoreCase) {
HttpComponent target = (HttpComponent) obj;
switch (ignoreCase ? name.toLowerCase() : name) {
case "allowjavaserializedobject":
case "allowJavaSerializedObject": return target.isAllowJavaSerializedObject();
case "authcachingdisabled":
case "authCachingDisabled": return target.isAuthCachingDisabled();
case "automaticretriesdisabled":
case "automaticRetriesDisabled": return target.isAutomaticRetriesDisabled();
case "autowiredenabled":
case "autowiredEnabled": return target.isAutowiredEnabled();
case "clientconnectionmanager":
case "clientConnectionManager": return target.getClientConnectionManager();
case "connecttimeout":
case "connectTimeout": return target.getConnectTimeout();
case "connectionrequesttimeout":
case "connectionRequestTimeout": return target.getConnectionRequestTimeout();
case "connectionstatedisabled":
case "connectionStateDisabled": return target.isConnectionStateDisabled();
case "connectiontimetolive":
case "connectionTimeToLive": return target.getConnectionTimeToLive();
case "connectionsperroute":
case "connectionsPerRoute": return target.getConnectionsPerRoute();
case "contentcompressiondisabled":
case "contentCompressionDisabled": return target.isContentCompressionDisabled();
case "cookiemanagementdisabled":
case "cookieManagementDisabled": return target.isCookieManagementDisabled();
case "cookiestore":
case "cookieStore": return target.getCookieStore();
case "copyheaders":
case "copyHeaders": return target.isCopyHeaders();
case "defaultuseragentdisabled":
case "defaultUserAgentDisabled": return target.isDefaultUserAgentDisabled();
case "followredirects":
case "followRedirects": return target.isFollowRedirects();
case "headerfilterstrategy":
case "headerFilterStrategy": return target.getHeaderFilterStrategy();
case "httpbinding":
case "httpBinding": return target.getHttpBinding();
case "httpclientconfigurer":
case "httpClientConfigurer": return target.getHttpClientConfigurer();
case "httpconfiguration":
case "httpConfiguration": return target.getHttpConfiguration();
case "httpcontext":
case "httpContext": return target.getHttpContext();
case "lazystartproducer":
case "lazyStartProducer": return target.isLazyStartProducer();
case "maxtotalconnections":
case "maxTotalConnections": return target.getMaxTotalConnections();
case "proxyauthdomain":
case "proxyAuthDomain": return target.getProxyAuthDomain();
case "proxyauthhost":
case "proxyAuthHost": return target.getProxyAuthHost();
case "proxyauthmethod":
case "proxyAuthMethod": return target.getProxyAuthMethod();
case "proxyauthnthost":
case "proxyAuthNtHost": return target.getProxyAuthNtHost();
case "proxyauthpassword":
case "proxyAuthPassword": return target.getProxyAuthPassword();
case "proxyauthport":
case "proxyAuthPort": return target.getProxyAuthPort();
case "proxyauthscheme":
case "proxyAuthScheme": return target.getProxyAuthScheme();
case "proxyauthusername":
case "proxyAuthUsername": return target.getProxyAuthUsername();
case "redirecthandlingdisabled":
case "redirectHandlingDisabled": return target.isRedirectHandlingDisabled();
case "responsepayloadstreamingthreshold":
case "responsePayloadStreamingThreshold": return target.getResponsePayloadStreamingThreshold();
case "responsetimeout":
case "responseTimeout": return target.getResponseTimeout();
case "skiprequestheaders":
case "skipRequestHeaders": return target.isSkipRequestHeaders();
case "skipresponseheaders":
case "skipResponseHeaders": return target.isSkipResponseHeaders();
case "sotimeout":
case "soTimeout": return target.getSoTimeout();
case "sslcontextparameters":
case "sslContextParameters": return target.getSslContextParameters();
case "useglobalsslcontextparameters":
case "useGlobalSslContextParameters": return target.isUseGlobalSslContextParameters();
case "x509hostnameverifier":
case "x509HostnameVerifier": return target.getX509HostnameVerifier();
default: return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy