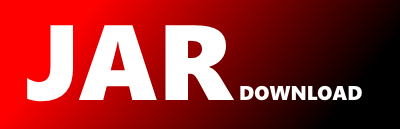
org.apache.camel.component.huaweicloud.frs.FaceRecognitionEndpointConfigurer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of camel-huaweicloud-frs Show documentation
Show all versions of camel-huaweicloud-frs Show documentation
A Camel Huawei Face Recognition Service component
The newest version!
/* Generated by camel build tools - do NOT edit this file! */
package org.apache.camel.component.huaweicloud.frs;
import javax.annotation.processing.Generated;
import java.util.Map;
import org.apache.camel.CamelContext;
import org.apache.camel.spi.ExtendedPropertyConfigurerGetter;
import org.apache.camel.spi.PropertyConfigurerGetter;
import org.apache.camel.spi.ConfigurerStrategy;
import org.apache.camel.spi.GeneratedPropertyConfigurer;
import org.apache.camel.util.CaseInsensitiveMap;
import org.apache.camel.support.component.PropertyConfigurerSupport;
/**
* Generated by camel build tools - do NOT edit this file!
*/
@Generated("org.apache.camel.maven.packaging.EndpointSchemaGeneratorMojo")
@SuppressWarnings("unchecked")
public class FaceRecognitionEndpointConfigurer extends PropertyConfigurerSupport implements GeneratedPropertyConfigurer, PropertyConfigurerGetter {
@Override
public boolean configure(CamelContext camelContext, Object obj, String name, Object value, boolean ignoreCase) {
FaceRecognitionEndpoint target = (FaceRecognitionEndpoint) obj;
switch (ignoreCase ? name.toLowerCase() : name) {
case "accesskey":
case "accessKey": target.setAccessKey(property(camelContext, java.lang.String.class, value)); return true;
case "actiontimes":
case "actionTimes": target.setActionTimes(property(camelContext, java.lang.String.class, value)); return true;
case "actions": target.setActions(property(camelContext, java.lang.String.class, value)); return true;
case "anotherimagebase64":
case "anotherImageBase64": target.setAnotherImageBase64(property(camelContext, java.lang.String.class, value)); return true;
case "anotherimagefilepath":
case "anotherImageFilePath": target.setAnotherImageFilePath(property(camelContext, java.lang.String.class, value)); return true;
case "anotherimageurl":
case "anotherImageUrl": target.setAnotherImageUrl(property(camelContext, java.lang.String.class, value)); return true;
case "endpoint": target.setEndpoint(property(camelContext, java.lang.String.class, value)); return true;
case "ignoresslverification":
case "ignoreSslVerification": target.setIgnoreSslVerification(property(camelContext, boolean.class, value)); return true;
case "imagebase64":
case "imageBase64": target.setImageBase64(property(camelContext, java.lang.String.class, value)); return true;
case "imagefilepath":
case "imageFilePath": target.setImageFilePath(property(camelContext, java.lang.String.class, value)); return true;
case "imageurl":
case "imageUrl": target.setImageUrl(property(camelContext, java.lang.String.class, value)); return true;
case "lazystartproducer":
case "lazyStartProducer": target.setLazyStartProducer(property(camelContext, boolean.class, value)); return true;
case "projectid":
case "projectId": target.setProjectId(property(camelContext, java.lang.String.class, value)); return true;
case "proxyhost":
case "proxyHost": target.setProxyHost(property(camelContext, java.lang.String.class, value)); return true;
case "proxypassword":
case "proxyPassword": target.setProxyPassword(property(camelContext, java.lang.String.class, value)); return true;
case "proxyport":
case "proxyPort": target.setProxyPort(property(camelContext, int.class, value)); return true;
case "proxyuser":
case "proxyUser": target.setProxyUser(property(camelContext, java.lang.String.class, value)); return true;
case "region": target.setRegion(property(camelContext, java.lang.String.class, value)); return true;
case "secretkey":
case "secretKey": target.setSecretKey(property(camelContext, java.lang.String.class, value)); return true;
case "servicekeys":
case "serviceKeys": target.setServiceKeys(property(camelContext, org.apache.camel.component.huaweicloud.common.models.ServiceKeys.class, value)); return true;
case "videobase64":
case "videoBase64": target.setVideoBase64(property(camelContext, java.lang.String.class, value)); return true;
case "videofilepath":
case "videoFilePath": target.setVideoFilePath(property(camelContext, java.lang.String.class, value)); return true;
case "videourl":
case "videoUrl": target.setVideoUrl(property(camelContext, java.lang.String.class, value)); return true;
default: return false;
}
}
@Override
public Class> getOptionType(String name, boolean ignoreCase) {
switch (ignoreCase ? name.toLowerCase() : name) {
case "accesskey":
case "accessKey": return java.lang.String.class;
case "actiontimes":
case "actionTimes": return java.lang.String.class;
case "actions": return java.lang.String.class;
case "anotherimagebase64":
case "anotherImageBase64": return java.lang.String.class;
case "anotherimagefilepath":
case "anotherImageFilePath": return java.lang.String.class;
case "anotherimageurl":
case "anotherImageUrl": return java.lang.String.class;
case "endpoint": return java.lang.String.class;
case "ignoresslverification":
case "ignoreSslVerification": return boolean.class;
case "imagebase64":
case "imageBase64": return java.lang.String.class;
case "imagefilepath":
case "imageFilePath": return java.lang.String.class;
case "imageurl":
case "imageUrl": return java.lang.String.class;
case "lazystartproducer":
case "lazyStartProducer": return boolean.class;
case "projectid":
case "projectId": return java.lang.String.class;
case "proxyhost":
case "proxyHost": return java.lang.String.class;
case "proxypassword":
case "proxyPassword": return java.lang.String.class;
case "proxyport":
case "proxyPort": return int.class;
case "proxyuser":
case "proxyUser": return java.lang.String.class;
case "region": return java.lang.String.class;
case "secretkey":
case "secretKey": return java.lang.String.class;
case "servicekeys":
case "serviceKeys": return org.apache.camel.component.huaweicloud.common.models.ServiceKeys.class;
case "videobase64":
case "videoBase64": return java.lang.String.class;
case "videofilepath":
case "videoFilePath": return java.lang.String.class;
case "videourl":
case "videoUrl": return java.lang.String.class;
default: return null;
}
}
@Override
public Object getOptionValue(Object obj, String name, boolean ignoreCase) {
FaceRecognitionEndpoint target = (FaceRecognitionEndpoint) obj;
switch (ignoreCase ? name.toLowerCase() : name) {
case "accesskey":
case "accessKey": return target.getAccessKey();
case "actiontimes":
case "actionTimes": return target.getActionTimes();
case "actions": return target.getActions();
case "anotherimagebase64":
case "anotherImageBase64": return target.getAnotherImageBase64();
case "anotherimagefilepath":
case "anotherImageFilePath": return target.getAnotherImageFilePath();
case "anotherimageurl":
case "anotherImageUrl": return target.getAnotherImageUrl();
case "endpoint": return target.getEndpoint();
case "ignoresslverification":
case "ignoreSslVerification": return target.isIgnoreSslVerification();
case "imagebase64":
case "imageBase64": return target.getImageBase64();
case "imagefilepath":
case "imageFilePath": return target.getImageFilePath();
case "imageurl":
case "imageUrl": return target.getImageUrl();
case "lazystartproducer":
case "lazyStartProducer": return target.isLazyStartProducer();
case "projectid":
case "projectId": return target.getProjectId();
case "proxyhost":
case "proxyHost": return target.getProxyHost();
case "proxypassword":
case "proxyPassword": return target.getProxyPassword();
case "proxyport":
case "proxyPort": return target.getProxyPort();
case "proxyuser":
case "proxyUser": return target.getProxyUser();
case "region": return target.getRegion();
case "secretkey":
case "secretKey": return target.getSecretKey();
case "servicekeys":
case "serviceKeys": return target.getServiceKeys();
case "videobase64":
case "videoBase64": return target.getVideoBase64();
case "videofilepath":
case "videoFilePath": return target.getVideoFilePath();
case "videourl":
case "videoUrl": return target.getVideoUrl();
default: return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy