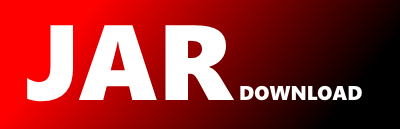
org.apache.camel.component.ironmq.IronMQEndpointConfigurer Maven / Gradle / Ivy
/* Generated by org.apache.camel:apt */
package org.apache.camel.component.ironmq;
import java.util.HashMap;
import java.util.Map;
import org.apache.camel.CamelContext;
import org.apache.camel.spi.GeneratedPropertyConfigurer;
import org.apache.camel.support.component.PropertyConfigurerSupport;
/**
* Source code generated by org.apache.camel:apt
*/
@SuppressWarnings("unchecked")
public class IronMQEndpointConfigurer extends PropertyConfigurerSupport implements GeneratedPropertyConfigurer {
@Override
public boolean configure(CamelContext camelContext, Object endpoint, String name, Object value, boolean ignoreCase) {
if (ignoreCase) {
return doConfigureIgnoreCase(camelContext, endpoint, name, value);
} else {
return doConfigure(camelContext, endpoint, name, value);
}
}
private static boolean doConfigure(CamelContext camelContext, Object endpoint, String name, Object value) {
switch (name) {
case "projectId": ((IronMQEndpoint) endpoint).getConfiguration().setProjectId(property(camelContext, java.lang.String.class, value)); return true;
case "token": ((IronMQEndpoint) endpoint).getConfiguration().setToken(property(camelContext, java.lang.String.class, value)); return true;
case "ironMQCloud": ((IronMQEndpoint) endpoint).getConfiguration().setIronMQCloud(property(camelContext, java.lang.String.class, value)); return true;
case "preserveHeaders": ((IronMQEndpoint) endpoint).getConfiguration().setPreserveHeaders(property(camelContext, boolean.class, value)); return true;
case "client": ((IronMQEndpoint) endpoint).getConfiguration().setClient(property(camelContext, io.iron.ironmq.Client.class, value)); return true;
case "visibilityDelay": ((IronMQEndpoint) endpoint).getConfiguration().setVisibilityDelay(property(camelContext, int.class, value)); return true;
case "concurrentConsumers": ((IronMQEndpoint) endpoint).getConfiguration().setConcurrentConsumers(property(camelContext, int.class, value)); return true;
case "batchDelete": ((IronMQEndpoint) endpoint).getConfiguration().setBatchDelete(property(camelContext, boolean.class, value)); return true;
case "maxMessagesPerPoll": ((IronMQEndpoint) endpoint).getConfiguration().setMaxMessagesPerPoll(property(camelContext, int.class, value)); return true;
case "timeout": ((IronMQEndpoint) endpoint).getConfiguration().setTimeout(property(camelContext, int.class, value)); return true;
case "wait": ((IronMQEndpoint) endpoint).getConfiguration().setWait(property(camelContext, int.class, value)); return true;
case "startScheduler": ((IronMQEndpoint) endpoint).setStartScheduler(property(camelContext, boolean.class, value)); return true;
case "initialDelay": ((IronMQEndpoint) endpoint).setInitialDelay(property(camelContext, long.class, value)); return true;
case "delay": ((IronMQEndpoint) endpoint).setDelay(property(camelContext, long.class, value)); return true;
case "timeUnit": ((IronMQEndpoint) endpoint).setTimeUnit(property(camelContext, java.util.concurrent.TimeUnit.class, value)); return true;
case "useFixedDelay": ((IronMQEndpoint) endpoint).setUseFixedDelay(property(camelContext, boolean.class, value)); return true;
case "pollStrategy": ((IronMQEndpoint) endpoint).setPollStrategy(property(camelContext, org.apache.camel.spi.PollingConsumerPollStrategy.class, value)); return true;
case "runLoggingLevel": ((IronMQEndpoint) endpoint).setRunLoggingLevel(property(camelContext, org.apache.camel.LoggingLevel.class, value)); return true;
case "sendEmptyMessageWhenIdle": ((IronMQEndpoint) endpoint).setSendEmptyMessageWhenIdle(property(camelContext, boolean.class, value)); return true;
case "greedy": ((IronMQEndpoint) endpoint).setGreedy(property(camelContext, boolean.class, value)); return true;
case "scheduler": ((IronMQEndpoint) endpoint).setScheduler(property(camelContext, java.lang.String.class, value)); return true;
case "schedulerProperties": ((IronMQEndpoint) endpoint).setSchedulerProperties(property(camelContext, java.util.Map.class, value)); return true;
case "scheduledExecutorService": ((IronMQEndpoint) endpoint).setScheduledExecutorService(property(camelContext, java.util.concurrent.ScheduledExecutorService.class, value)); return true;
case "backoffMultiplier": ((IronMQEndpoint) endpoint).setBackoffMultiplier(property(camelContext, int.class, value)); return true;
case "backoffIdleThreshold": ((IronMQEndpoint) endpoint).setBackoffIdleThreshold(property(camelContext, int.class, value)); return true;
case "backoffErrorThreshold": ((IronMQEndpoint) endpoint).setBackoffErrorThreshold(property(camelContext, int.class, value)); return true;
case "repeatCount": ((IronMQEndpoint) endpoint).setRepeatCount(property(camelContext, long.class, value)); return true;
case "lazyStartProducer": ((IronMQEndpoint) endpoint).setLazyStartProducer(property(camelContext, boolean.class, value)); return true;
case "bridgeErrorHandler": ((IronMQEndpoint) endpoint).setBridgeErrorHandler(property(camelContext, boolean.class, value)); return true;
case "exceptionHandler": ((IronMQEndpoint) endpoint).setExceptionHandler(property(camelContext, org.apache.camel.spi.ExceptionHandler.class, value)); return true;
case "exchangePattern": ((IronMQEndpoint) endpoint).setExchangePattern(property(camelContext, org.apache.camel.ExchangePattern.class, value)); return true;
case "synchronous": ((IronMQEndpoint) endpoint).setSynchronous(property(camelContext, boolean.class, value)); return true;
case "basicPropertyBinding": ((IronMQEndpoint) endpoint).setBasicPropertyBinding(property(camelContext, boolean.class, value)); return true;
default: return false;
}
}
private static boolean doConfigureIgnoreCase(CamelContext camelContext, Object endpoint, String name, Object value) {
switch (name.toLowerCase()) {
case "projectid": ((IronMQEndpoint) endpoint).getConfiguration().setProjectId(property(camelContext, java.lang.String.class, value)); return true;
case "token": ((IronMQEndpoint) endpoint).getConfiguration().setToken(property(camelContext, java.lang.String.class, value)); return true;
case "ironmqcloud": ((IronMQEndpoint) endpoint).getConfiguration().setIronMQCloud(property(camelContext, java.lang.String.class, value)); return true;
case "preserveheaders": ((IronMQEndpoint) endpoint).getConfiguration().setPreserveHeaders(property(camelContext, boolean.class, value)); return true;
case "client": ((IronMQEndpoint) endpoint).getConfiguration().setClient(property(camelContext, io.iron.ironmq.Client.class, value)); return true;
case "visibilitydelay": ((IronMQEndpoint) endpoint).getConfiguration().setVisibilityDelay(property(camelContext, int.class, value)); return true;
case "concurrentconsumers": ((IronMQEndpoint) endpoint).getConfiguration().setConcurrentConsumers(property(camelContext, int.class, value)); return true;
case "batchdelete": ((IronMQEndpoint) endpoint).getConfiguration().setBatchDelete(property(camelContext, boolean.class, value)); return true;
case "maxmessagesperpoll": ((IronMQEndpoint) endpoint).getConfiguration().setMaxMessagesPerPoll(property(camelContext, int.class, value)); return true;
case "timeout": ((IronMQEndpoint) endpoint).getConfiguration().setTimeout(property(camelContext, int.class, value)); return true;
case "wait": ((IronMQEndpoint) endpoint).getConfiguration().setWait(property(camelContext, int.class, value)); return true;
case "startscheduler": ((IronMQEndpoint) endpoint).setStartScheduler(property(camelContext, boolean.class, value)); return true;
case "initialdelay": ((IronMQEndpoint) endpoint).setInitialDelay(property(camelContext, long.class, value)); return true;
case "delay": ((IronMQEndpoint) endpoint).setDelay(property(camelContext, long.class, value)); return true;
case "timeunit": ((IronMQEndpoint) endpoint).setTimeUnit(property(camelContext, java.util.concurrent.TimeUnit.class, value)); return true;
case "usefixeddelay": ((IronMQEndpoint) endpoint).setUseFixedDelay(property(camelContext, boolean.class, value)); return true;
case "pollstrategy": ((IronMQEndpoint) endpoint).setPollStrategy(property(camelContext, org.apache.camel.spi.PollingConsumerPollStrategy.class, value)); return true;
case "runlogginglevel": ((IronMQEndpoint) endpoint).setRunLoggingLevel(property(camelContext, org.apache.camel.LoggingLevel.class, value)); return true;
case "sendemptymessagewhenidle": ((IronMQEndpoint) endpoint).setSendEmptyMessageWhenIdle(property(camelContext, boolean.class, value)); return true;
case "greedy": ((IronMQEndpoint) endpoint).setGreedy(property(camelContext, boolean.class, value)); return true;
case "scheduler": ((IronMQEndpoint) endpoint).setScheduler(property(camelContext, java.lang.String.class, value)); return true;
case "schedulerproperties": ((IronMQEndpoint) endpoint).setSchedulerProperties(property(camelContext, java.util.Map.class, value)); return true;
case "scheduledexecutorservice": ((IronMQEndpoint) endpoint).setScheduledExecutorService(property(camelContext, java.util.concurrent.ScheduledExecutorService.class, value)); return true;
case "backoffmultiplier": ((IronMQEndpoint) endpoint).setBackoffMultiplier(property(camelContext, int.class, value)); return true;
case "backoffidlethreshold": ((IronMQEndpoint) endpoint).setBackoffIdleThreshold(property(camelContext, int.class, value)); return true;
case "backofferrorthreshold": ((IronMQEndpoint) endpoint).setBackoffErrorThreshold(property(camelContext, int.class, value)); return true;
case "repeatcount": ((IronMQEndpoint) endpoint).setRepeatCount(property(camelContext, long.class, value)); return true;
case "lazystartproducer": ((IronMQEndpoint) endpoint).setLazyStartProducer(property(camelContext, boolean.class, value)); return true;
case "bridgeerrorhandler": ((IronMQEndpoint) endpoint).setBridgeErrorHandler(property(camelContext, boolean.class, value)); return true;
case "exceptionhandler": ((IronMQEndpoint) endpoint).setExceptionHandler(property(camelContext, org.apache.camel.spi.ExceptionHandler.class, value)); return true;
case "exchangepattern": ((IronMQEndpoint) endpoint).setExchangePattern(property(camelContext, org.apache.camel.ExchangePattern.class, value)); return true;
case "synchronous": ((IronMQEndpoint) endpoint).setSynchronous(property(camelContext, boolean.class, value)); return true;
case "basicpropertybinding": ((IronMQEndpoint) endpoint).setBasicPropertyBinding(property(camelContext, boolean.class, value)); return true;
default: return false;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy