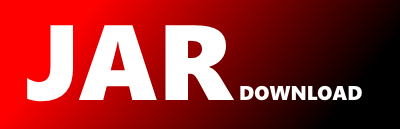
org.apache.camel.component.ironmq.IronMQConsumer Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.camel.component.ironmq;
import java.util.LinkedList;
import java.util.Queue;
import io.iron.ironmq.EmptyQueueException;
import io.iron.ironmq.Message;
import io.iron.ironmq.Messages;
import org.apache.camel.Endpoint;
import org.apache.camel.Exchange;
import org.apache.camel.ExchangePropertyKey;
import org.apache.camel.Processor;
import org.apache.camel.spi.ScheduledPollConsumerScheduler;
import org.apache.camel.spi.Synchronization;
import org.apache.camel.support.DefaultScheduledPollConsumerScheduler;
import org.apache.camel.support.ExchangeHelper;
import org.apache.camel.support.ScheduledBatchPollingConsumer;
import org.apache.camel.util.CastUtils;
import org.apache.camel.util.ObjectHelper;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* The IronMQ consumer.
*/
public class IronMQConsumer extends ScheduledBatchPollingConsumer {
private static final Logger LOG = LoggerFactory.getLogger(IronMQConsumer.class);
private io.iron.ironmq.Queue ironQueue;
public IronMQConsumer(Endpoint endpoint, Processor processor) {
super(endpoint, processor);
}
@Override
protected void afterConfigureScheduler(ScheduledPollConsumerScheduler scheduler, boolean newScheduler) {
if (newScheduler && scheduler instanceof DefaultScheduledPollConsumerScheduler) {
DefaultScheduledPollConsumerScheduler ds = (DefaultScheduledPollConsumerScheduler) scheduler;
ds.setConcurrentConsumers(getEndpoint().getConfiguration().getConcurrentConsumers());
// if using concurrent consumers then resize pool to be at least same size
int ps = Math.max(ds.getPoolSize(), getEndpoint().getConfiguration().getConcurrentConsumers());
ds.setPoolSize(ps);
}
}
@Override
protected void doStart() throws Exception {
super.doStart();
ironQueue = getEndpoint().getClient().queue(getEndpoint().getConfiguration().getQueueName());
}
@Override
protected int poll() throws Exception {
// must reset for each poll
shutdownRunningTask = null;
pendingExchanges = 0;
try {
Messages messages;
LOG.trace("Receiving messages with request [messagePerPoll {}, timeout {}]...", getMaxMessagesPerPoll(),
getEndpoint().getConfiguration().getTimeout());
messages = this.ironQueue.reserve(getMaxMessagesPerPoll(), getEndpoint().getConfiguration().getTimeout(),
getEndpoint().getConfiguration().getWait());
LOG.trace("Received {} messages", messages.getSize());
// okay we have some response from ironmq so lets mark the consumer as ready
forceConsumerAsReady();
Queue exchanges = createExchanges(messages.getMessages());
int noProcessed = processBatch(CastUtils.cast(exchanges));
// delete all processed messages in one batch;
if (getEndpoint().getConfiguration().isBatchDelete()) {
LOG.trace("Batch deleting {} messages", messages.getSize());
this.ironQueue.deleteMessages(messages);
}
return noProcessed;
} catch (EmptyQueueException e) {
return 0;
}
}
protected Queue createExchanges(Message[] messages) {
LOG.trace("Received {} messages in this poll", messages.length);
Queue answer = new LinkedList<>();
for (Message message : messages) {
Exchange exchange = createExchange(message);
answer.add(exchange);
}
return answer;
}
@Override
public int processBatch(Queue
© 2015 - 2025 Weber Informatics LLC | Privacy Policy