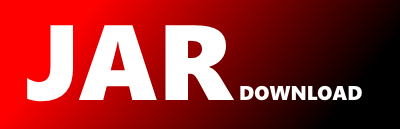
org.apache.camel.component.jbpm.JBPMEndpointConfigurer Maven / Gradle / Ivy
/* Generated by org.apache.camel:apt */
package org.apache.camel.component.jbpm;
import java.util.HashMap;
import java.util.Map;
import org.apache.camel.CamelContext;
import org.apache.camel.spi.GeneratedPropertyConfigurer;
import org.apache.camel.support.component.PropertyConfigurerSupport;
/**
* Source code generated by org.apache.camel:apt
*/
@SuppressWarnings("unchecked")
public class JBPMEndpointConfigurer extends PropertyConfigurerSupport implements GeneratedPropertyConfigurer {
@Override
public boolean configure(CamelContext camelContext, Object endpoint, String name, Object value, boolean ignoreCase) {
if (ignoreCase) {
return doConfigureIgnoreCase(camelContext, endpoint, name, value);
} else {
return doConfigure(camelContext, endpoint, name, value);
}
}
private static boolean doConfigure(CamelContext camelContext, Object endpoint, String name, Object value) {
switch (name) {
case "operation": ((JBPMEndpoint) endpoint).getConfiguration().setOperation(property(camelContext, java.lang.String.class, value)); return true;
case "deploymentId": ((JBPMEndpoint) endpoint).getConfiguration().setDeploymentId(property(camelContext, java.lang.String.class, value)); return true;
case "processInstanceId": ((JBPMEndpoint) endpoint).getConfiguration().setProcessInstanceId(property(camelContext, java.lang.Long.class, value)); return true;
case "value": ((JBPMEndpoint) endpoint).getConfiguration().setValue(property(camelContext, java.lang.Object.class, value)); return true;
case "processId": ((JBPMEndpoint) endpoint).getConfiguration().setProcessId(property(camelContext, java.lang.String.class, value)); return true;
case "eventType": ((JBPMEndpoint) endpoint).getConfiguration().setEventType(property(camelContext, java.lang.String.class, value)); return true;
case "event": ((JBPMEndpoint) endpoint).getConfiguration().setEvent(property(camelContext, java.lang.Object.class, value)); return true;
case "maxNumber": ((JBPMEndpoint) endpoint).getConfiguration().setMaxNumber(property(camelContext, java.lang.Integer.class, value)); return true;
case "identifier": ((JBPMEndpoint) endpoint).getConfiguration().setIdentifier(property(camelContext, java.lang.String.class, value)); return true;
case "workItemId": ((JBPMEndpoint) endpoint).getConfiguration().setWorkItemId(property(camelContext, java.lang.Long.class, value)); return true;
case "taskId": ((JBPMEndpoint) endpoint).getConfiguration().setTaskId(property(camelContext, java.lang.Long.class, value)); return true;
case "userId": ((JBPMEndpoint) endpoint).getConfiguration().setUserId(property(camelContext, java.lang.String.class, value)); return true;
case "page": ((JBPMEndpoint) endpoint).getConfiguration().setPage(property(camelContext, java.lang.Integer.class, value)); return true;
case "pageSize": ((JBPMEndpoint) endpoint).getConfiguration().setPageSize(property(camelContext, java.lang.Integer.class, value)); return true;
case "targetUserId": ((JBPMEndpoint) endpoint).getConfiguration().setTargetUserId(property(camelContext, java.lang.String.class, value)); return true;
case "attachmentId": ((JBPMEndpoint) endpoint).getConfiguration().setAttachmentId(property(camelContext, java.lang.Long.class, value)); return true;
case "contentId": ((JBPMEndpoint) endpoint).getConfiguration().setContentId(property(camelContext, java.lang.Long.class, value)); return true;
case "task": ((JBPMEndpoint) endpoint).getConfiguration().setTask(property(camelContext, org.kie.api.task.model.Task.class, value)); return true;
case "entities": ((JBPMEndpoint) endpoint).getConfiguration().setEntities(property(camelContext, java.util.List.class, value)); return true;
case "statuses": ((JBPMEndpoint) endpoint).getConfiguration().setStatuses(property(camelContext, java.util.List.class, value)); return true;
case "userName": ((JBPMEndpoint) endpoint).getConfiguration().setUserName(property(camelContext, java.lang.String.class, value)); return true;
case "password": ((JBPMEndpoint) endpoint).getConfiguration().setPassword(property(camelContext, java.lang.String.class, value)); return true;
case "timeout": ((JBPMEndpoint) endpoint).getConfiguration().setTimeout(property(camelContext, java.lang.Integer.class, value)); return true;
case "parameters": ((JBPMEndpoint) endpoint).getConfiguration().setParameters(property(camelContext, java.util.Map.class, value)); return true;
case "extraJaxbClasses": ((JBPMEndpoint) endpoint).getConfiguration().setExtraJaxbClasses(property(camelContext, java.lang.Class[].class, value)); return true;
case "emitterSendItems": ((JBPMEndpoint) endpoint).getConfiguration().setEmitterSendItems(property(camelContext, java.lang.Boolean.class, value)); return true;
case "lazyStartProducer": ((JBPMEndpoint) endpoint).setLazyStartProducer(property(camelContext, boolean.class, value)); return true;
case "bridgeErrorHandler": ((JBPMEndpoint) endpoint).setBridgeErrorHandler(property(camelContext, boolean.class, value)); return true;
case "exceptionHandler": ((JBPMEndpoint) endpoint).setExceptionHandler(property(camelContext, org.apache.camel.spi.ExceptionHandler.class, value)); return true;
case "exchangePattern": ((JBPMEndpoint) endpoint).setExchangePattern(property(camelContext, org.apache.camel.ExchangePattern.class, value)); return true;
case "synchronous": ((JBPMEndpoint) endpoint).setSynchronous(property(camelContext, boolean.class, value)); return true;
case "basicPropertyBinding": ((JBPMEndpoint) endpoint).setBasicPropertyBinding(property(camelContext, boolean.class, value)); return true;
default: return false;
}
}
private static boolean doConfigureIgnoreCase(CamelContext camelContext, Object endpoint, String name, Object value) {
switch (name.toLowerCase()) {
case "operation": ((JBPMEndpoint) endpoint).getConfiguration().setOperation(property(camelContext, java.lang.String.class, value)); return true;
case "deploymentid": ((JBPMEndpoint) endpoint).getConfiguration().setDeploymentId(property(camelContext, java.lang.String.class, value)); return true;
case "processinstanceid": ((JBPMEndpoint) endpoint).getConfiguration().setProcessInstanceId(property(camelContext, java.lang.Long.class, value)); return true;
case "value": ((JBPMEndpoint) endpoint).getConfiguration().setValue(property(camelContext, java.lang.Object.class, value)); return true;
case "processid": ((JBPMEndpoint) endpoint).getConfiguration().setProcessId(property(camelContext, java.lang.String.class, value)); return true;
case "eventtype": ((JBPMEndpoint) endpoint).getConfiguration().setEventType(property(camelContext, java.lang.String.class, value)); return true;
case "event": ((JBPMEndpoint) endpoint).getConfiguration().setEvent(property(camelContext, java.lang.Object.class, value)); return true;
case "maxnumber": ((JBPMEndpoint) endpoint).getConfiguration().setMaxNumber(property(camelContext, java.lang.Integer.class, value)); return true;
case "identifier": ((JBPMEndpoint) endpoint).getConfiguration().setIdentifier(property(camelContext, java.lang.String.class, value)); return true;
case "workitemid": ((JBPMEndpoint) endpoint).getConfiguration().setWorkItemId(property(camelContext, java.lang.Long.class, value)); return true;
case "taskid": ((JBPMEndpoint) endpoint).getConfiguration().setTaskId(property(camelContext, java.lang.Long.class, value)); return true;
case "userid": ((JBPMEndpoint) endpoint).getConfiguration().setUserId(property(camelContext, java.lang.String.class, value)); return true;
case "page": ((JBPMEndpoint) endpoint).getConfiguration().setPage(property(camelContext, java.lang.Integer.class, value)); return true;
case "pagesize": ((JBPMEndpoint) endpoint).getConfiguration().setPageSize(property(camelContext, java.lang.Integer.class, value)); return true;
case "targetuserid": ((JBPMEndpoint) endpoint).getConfiguration().setTargetUserId(property(camelContext, java.lang.String.class, value)); return true;
case "attachmentid": ((JBPMEndpoint) endpoint).getConfiguration().setAttachmentId(property(camelContext, java.lang.Long.class, value)); return true;
case "contentid": ((JBPMEndpoint) endpoint).getConfiguration().setContentId(property(camelContext, java.lang.Long.class, value)); return true;
case "task": ((JBPMEndpoint) endpoint).getConfiguration().setTask(property(camelContext, org.kie.api.task.model.Task.class, value)); return true;
case "entities": ((JBPMEndpoint) endpoint).getConfiguration().setEntities(property(camelContext, java.util.List.class, value)); return true;
case "statuses": ((JBPMEndpoint) endpoint).getConfiguration().setStatuses(property(camelContext, java.util.List.class, value)); return true;
case "username": ((JBPMEndpoint) endpoint).getConfiguration().setUserName(property(camelContext, java.lang.String.class, value)); return true;
case "password": ((JBPMEndpoint) endpoint).getConfiguration().setPassword(property(camelContext, java.lang.String.class, value)); return true;
case "timeout": ((JBPMEndpoint) endpoint).getConfiguration().setTimeout(property(camelContext, java.lang.Integer.class, value)); return true;
case "parameters": ((JBPMEndpoint) endpoint).getConfiguration().setParameters(property(camelContext, java.util.Map.class, value)); return true;
case "extrajaxbclasses": ((JBPMEndpoint) endpoint).getConfiguration().setExtraJaxbClasses(property(camelContext, java.lang.Class[].class, value)); return true;
case "emittersenditems": ((JBPMEndpoint) endpoint).getConfiguration().setEmitterSendItems(property(camelContext, java.lang.Boolean.class, value)); return true;
case "lazystartproducer": ((JBPMEndpoint) endpoint).setLazyStartProducer(property(camelContext, boolean.class, value)); return true;
case "bridgeerrorhandler": ((JBPMEndpoint) endpoint).setBridgeErrorHandler(property(camelContext, boolean.class, value)); return true;
case "exceptionhandler": ((JBPMEndpoint) endpoint).setExceptionHandler(property(camelContext, org.apache.camel.spi.ExceptionHandler.class, value)); return true;
case "exchangepattern": ((JBPMEndpoint) endpoint).setExchangePattern(property(camelContext, org.apache.camel.ExchangePattern.class, value)); return true;
case "synchronous": ((JBPMEndpoint) endpoint).setSynchronous(property(camelContext, boolean.class, value)); return true;
case "basicpropertybinding": ((JBPMEndpoint) endpoint).setBasicPropertyBinding(property(camelContext, boolean.class, value)); return true;
default: return false;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy