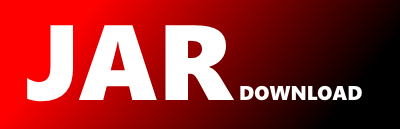
org.apache.camel.component.jooq.db.Keys Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of camel-jooq Show documentation
Show all versions of camel-jooq Show documentation
The jooq component enables you to store and retrieve entities from databases using JOOQ
/*
* This file is generated by jOOQ.
*/
package org.apache.camel.component.jooq.db;
import org.apache.camel.component.jooq.db.tables.Author;
import org.apache.camel.component.jooq.db.tables.Book;
import org.apache.camel.component.jooq.db.tables.BookStore;
import org.apache.camel.component.jooq.db.tables.BookToBookStore;
import org.apache.camel.component.jooq.db.tables.records.AuthorRecord;
import org.apache.camel.component.jooq.db.tables.records.BookRecord;
import org.apache.camel.component.jooq.db.tables.records.BookStoreRecord;
import org.apache.camel.component.jooq.db.tables.records.BookToBookStoreRecord;
import org.jooq.ForeignKey;
import org.jooq.TableField;
import org.jooq.UniqueKey;
import org.jooq.impl.DSL;
import org.jooq.impl.Internal;
/**
* A class modelling foreign key relationships and constraints of tables in
* PUBLIC.
*/
@SuppressWarnings({ "all", "unchecked", "rawtypes", "this-escape" })
public class Keys {
// -------------------------------------------------------------------------
// UNIQUE and PRIMARY KEY definitions
// -------------------------------------------------------------------------
public static final UniqueKey PK_T_AUTHOR = Internal.createUniqueKey(Author.AUTHOR, DSL.name("PK_T_AUTHOR"), new TableField[] { Author.AUTHOR.ID }, true);
public static final UniqueKey PK_T_BOOK = Internal.createUniqueKey(Book.BOOK, DSL.name("PK_T_BOOK"), new TableField[] { Book.BOOK.ID }, true);
public static final UniqueKey UK_T_BOOK_STORE_NAME = Internal.createUniqueKey(BookStore.BOOK_STORE, DSL.name("UK_T_BOOK_STORE_NAME"), new TableField[] { BookStore.BOOK_STORE.NAME }, true);
public static final UniqueKey PK_B2BS = Internal.createUniqueKey(BookToBookStore.BOOK_TO_BOOK_STORE, DSL.name("PK_B2BS"), new TableField[] { BookToBookStore.BOOK_TO_BOOK_STORE.BOOK_STORE_NAME, BookToBookStore.BOOK_TO_BOOK_STORE.BOOK_ID }, true);
// -------------------------------------------------------------------------
// FOREIGN KEY definitions
// -------------------------------------------------------------------------
public static final ForeignKey FK_T_BOOK_AUTHOR_ID = Internal.createForeignKey(Book.BOOK, DSL.name("FK_T_BOOK_AUTHOR_ID"), new TableField[] { Book.BOOK.AUTHOR_ID }, Keys.PK_T_AUTHOR, new TableField[] { Author.AUTHOR.ID }, true);
public static final ForeignKey FK_T_BOOK_CO_AUTHOR_ID = Internal.createForeignKey(Book.BOOK, DSL.name("FK_T_BOOK_CO_AUTHOR_ID"), new TableField[] { Book.BOOK.CO_AUTHOR_ID }, Keys.PK_T_AUTHOR, new TableField[] { Author.AUTHOR.ID }, true);
public static final ForeignKey FK_B2BS_B_ID = Internal.createForeignKey(BookToBookStore.BOOK_TO_BOOK_STORE, DSL.name("FK_B2BS_B_ID"), new TableField[] { BookToBookStore.BOOK_TO_BOOK_STORE.BOOK_ID }, Keys.PK_T_BOOK, new TableField[] { Book.BOOK.ID }, true);
public static final ForeignKey FK_B2BS_BS_NAME = Internal.createForeignKey(BookToBookStore.BOOK_TO_BOOK_STORE, DSL.name("FK_B2BS_BS_NAME"), new TableField[] { BookToBookStore.BOOK_TO_BOOK_STORE.BOOK_STORE_NAME }, Keys.UK_T_BOOK_STORE_NAME, new TableField[] { BookStore.BOOK_STORE.NAME }, true);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy