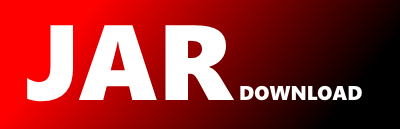
org.apache.camel.component.netty.NettyEndpointConfigurer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of camel-netty Show documentation
Show all versions of camel-netty Show documentation
Camel Netty NIO based socket communication component
The newest version!
/* Generated by camel build tools - do NOT edit this file! */
package org.apache.camel.component.netty;
import javax.annotation.processing.Generated;
import java.util.Map;
import org.apache.camel.CamelContext;
import org.apache.camel.spi.ExtendedPropertyConfigurerGetter;
import org.apache.camel.spi.PropertyConfigurerGetter;
import org.apache.camel.spi.ConfigurerStrategy;
import org.apache.camel.spi.GeneratedPropertyConfigurer;
import org.apache.camel.util.CaseInsensitiveMap;
import org.apache.camel.support.component.PropertyConfigurerSupport;
/**
* Generated by camel build tools - do NOT edit this file!
*/
@Generated("org.apache.camel.maven.packaging.EndpointSchemaGeneratorMojo")
@SuppressWarnings("unchecked")
public class NettyEndpointConfigurer extends PropertyConfigurerSupport implements GeneratedPropertyConfigurer, PropertyConfigurerGetter {
@Override
public boolean configure(CamelContext camelContext, Object obj, String name, Object value, boolean ignoreCase) {
NettyEndpoint target = (NettyEndpoint) obj;
switch (ignoreCase ? name.toLowerCase() : name) {
case "allowdefaultcodec":
case "allowDefaultCodec": target.getConfiguration().setAllowDefaultCodec(property(camelContext, boolean.class, value)); return true;
case "allowserializedheaders":
case "allowSerializedHeaders": target.getConfiguration().setAllowSerializedHeaders(property(camelContext, boolean.class, value)); return true;
case "autoappenddelimiter":
case "autoAppendDelimiter": target.getConfiguration().setAutoAppendDelimiter(property(camelContext, boolean.class, value)); return true;
case "backlog": target.getConfiguration().setBacklog(property(camelContext, int.class, value)); return true;
case "bosscount":
case "bossCount": target.getConfiguration().setBossCount(property(camelContext, int.class, value)); return true;
case "bossgroup":
case "bossGroup": target.getConfiguration().setBossGroup(property(camelContext, io.netty.channel.EventLoopGroup.class, value)); return true;
case "bridgeerrorhandler":
case "bridgeErrorHandler": target.setBridgeErrorHandler(property(camelContext, boolean.class, value)); return true;
case "broadcast": target.getConfiguration().setBroadcast(property(camelContext, boolean.class, value)); return true;
case "channelgroup":
case "channelGroup": target.getConfiguration().setChannelGroup(property(camelContext, io.netty.channel.group.ChannelGroup.class, value)); return true;
case "clientinitializerfactory":
case "clientInitializerFactory": target.getConfiguration().setClientInitializerFactory(property(camelContext, org.apache.camel.component.netty.ClientInitializerFactory.class, value)); return true;
case "clientmode":
case "clientMode": target.getConfiguration().setClientMode(property(camelContext, boolean.class, value)); return true;
case "connecttimeout":
case "connectTimeout": target.getConfiguration().setConnectTimeout(property(camelContext, int.class, value)); return true;
case "correlationmanager":
case "correlationManager": target.getConfiguration().setCorrelationManager(property(camelContext, org.apache.camel.component.netty.NettyCamelStateCorrelationManager.class, value)); return true;
case "decodermaxlinelength":
case "decoderMaxLineLength": target.getConfiguration().setDecoderMaxLineLength(property(camelContext, int.class, value)); return true;
case "decoders": target.getConfiguration().setDecoders(property(camelContext, java.lang.String.class, value)); return true;
case "delimiter": target.getConfiguration().setDelimiter(property(camelContext, org.apache.camel.component.netty.TextLineDelimiter.class, value)); return true;
case "disconnect": target.getConfiguration().setDisconnect(property(camelContext, boolean.class, value)); return true;
case "disconnectonnoreply":
case "disconnectOnNoReply": target.getConfiguration().setDisconnectOnNoReply(property(camelContext, boolean.class, value)); return true;
case "enabledprotocols":
case "enabledProtocols": target.getConfiguration().setEnabledProtocols(property(camelContext, java.lang.String.class, value)); return true;
case "encoders": target.getConfiguration().setEncoders(property(camelContext, java.lang.String.class, value)); return true;
case "encoding": target.getConfiguration().setEncoding(property(camelContext, java.lang.String.class, value)); return true;
case "exceptionhandler":
case "exceptionHandler": target.setExceptionHandler(property(camelContext, org.apache.camel.spi.ExceptionHandler.class, value)); return true;
case "exchangepattern":
case "exchangePattern": target.setExchangePattern(property(camelContext, org.apache.camel.ExchangePattern.class, value)); return true;
case "hostnameverification":
case "hostnameVerification": target.getConfiguration().setHostnameVerification(property(camelContext, boolean.class, value)); return true;
case "keepalive":
case "keepAlive": target.getConfiguration().setKeepAlive(property(camelContext, boolean.class, value)); return true;
case "keystorefile":
case "keyStoreFile": target.getConfiguration().setKeyStoreFile(property(camelContext, java.io.File.class, value)); return true;
case "keystoreformat":
case "keyStoreFormat": target.getConfiguration().setKeyStoreFormat(property(camelContext, java.lang.String.class, value)); return true;
case "keystoreresource":
case "keyStoreResource": target.getConfiguration().setKeyStoreResource(property(camelContext, java.lang.String.class, value)); return true;
case "lazychannelcreation":
case "lazyChannelCreation": target.getConfiguration().setLazyChannelCreation(property(camelContext, boolean.class, value)); return true;
case "lazystartproducer":
case "lazyStartProducer": target.setLazyStartProducer(property(camelContext, boolean.class, value)); return true;
case "nativetransport":
case "nativeTransport": target.getConfiguration().setNativeTransport(property(camelContext, boolean.class, value)); return true;
case "needclientauth":
case "needClientAuth": target.getConfiguration().setNeedClientAuth(property(camelContext, boolean.class, value)); return true;
case "nettyserverbootstrapfactory":
case "nettyServerBootstrapFactory": target.getConfiguration().setNettyServerBootstrapFactory(property(camelContext, org.apache.camel.component.netty.NettyServerBootstrapFactory.class, value)); return true;
case "networkinterface":
case "networkInterface": target.getConfiguration().setNetworkInterface(property(camelContext, java.lang.String.class, value)); return true;
case "noreplyloglevel":
case "noReplyLogLevel": target.getConfiguration().setNoReplyLogLevel(property(camelContext, org.apache.camel.LoggingLevel.class, value)); return true;
case "options": target.getConfiguration().setOptions(property(camelContext, java.util.Map.class, value)); return true;
case "passphrase": target.getConfiguration().setPassphrase(property(camelContext, java.lang.String.class, value)); return true;
case "producerpoolblockwhenexhausted":
case "producerPoolBlockWhenExhausted": target.getConfiguration().setProducerPoolBlockWhenExhausted(property(camelContext, boolean.class, value)); return true;
case "producerpoolenabled":
case "producerPoolEnabled": target.getConfiguration().setProducerPoolEnabled(property(camelContext, boolean.class, value)); return true;
case "producerpoolmaxidle":
case "producerPoolMaxIdle": target.getConfiguration().setProducerPoolMaxIdle(property(camelContext, int.class, value)); return true;
case "producerpoolmaxtotal":
case "producerPoolMaxTotal": target.getConfiguration().setProducerPoolMaxTotal(property(camelContext, int.class, value)); return true;
case "producerpoolmaxwait":
case "producerPoolMaxWait": target.getConfiguration().setProducerPoolMaxWait(property(camelContext, long.class, value)); return true;
case "producerpoolminevictableidle":
case "producerPoolMinEvictableIdle": target.getConfiguration().setProducerPoolMinEvictableIdle(property(camelContext, long.class, value)); return true;
case "producerpoolminidle":
case "producerPoolMinIdle": target.getConfiguration().setProducerPoolMinIdle(property(camelContext, int.class, value)); return true;
case "receivebuffersize":
case "receiveBufferSize": target.getConfiguration().setReceiveBufferSize(property(camelContext, int.class, value)); return true;
case "receivebuffersizepredictor":
case "receiveBufferSizePredictor": target.getConfiguration().setReceiveBufferSizePredictor(property(camelContext, int.class, value)); return true;
case "reconnect": target.getConfiguration().setReconnect(property(camelContext, boolean.class, value)); return true;
case "reconnectinterval":
case "reconnectInterval": target.getConfiguration().setReconnectInterval(property(camelContext, int.class, value)); return true;
case "requesttimeout":
case "requestTimeout": target.getConfiguration().setRequestTimeout(property(camelContext, long.class, value)); return true;
case "reuseaddress":
case "reuseAddress": target.getConfiguration().setReuseAddress(property(camelContext, boolean.class, value)); return true;
case "reusechannel":
case "reuseChannel": target.getConfiguration().setReuseChannel(property(camelContext, boolean.class, value)); return true;
case "securityprovider":
case "securityProvider": target.getConfiguration().setSecurityProvider(property(camelContext, java.lang.String.class, value)); return true;
case "sendbuffersize":
case "sendBufferSize": target.getConfiguration().setSendBufferSize(property(camelContext, int.class, value)); return true;
case "serverclosedchannelexceptioncaughtloglevel":
case "serverClosedChannelExceptionCaughtLogLevel": target.getConfiguration().setServerClosedChannelExceptionCaughtLogLevel(property(camelContext, org.apache.camel.LoggingLevel.class, value)); return true;
case "serverexceptioncaughtloglevel":
case "serverExceptionCaughtLogLevel": target.getConfiguration().setServerExceptionCaughtLogLevel(property(camelContext, org.apache.camel.LoggingLevel.class, value)); return true;
case "serverinitializerfactory":
case "serverInitializerFactory": target.getConfiguration().setServerInitializerFactory(property(camelContext, org.apache.camel.component.netty.ServerInitializerFactory.class, value)); return true;
case "ssl": target.getConfiguration().setSsl(property(camelContext, boolean.class, value)); return true;
case "sslclientcertheaders":
case "sslClientCertHeaders": target.getConfiguration().setSslClientCertHeaders(property(camelContext, boolean.class, value)); return true;
case "sslcontextparameters":
case "sslContextParameters": target.getConfiguration().setSslContextParameters(property(camelContext, org.apache.camel.support.jsse.SSLContextParameters.class, value)); return true;
case "sslhandler":
case "sslHandler": target.getConfiguration().setSslHandler(property(camelContext, io.netty.handler.ssl.SslHandler.class, value)); return true;
case "sync": target.getConfiguration().setSync(property(camelContext, boolean.class, value)); return true;
case "synchronous": target.setSynchronous(property(camelContext, boolean.class, value)); return true;
case "tcpnodelay":
case "tcpNoDelay": target.getConfiguration().setTcpNoDelay(property(camelContext, boolean.class, value)); return true;
case "textline": target.getConfiguration().setTextline(property(camelContext, boolean.class, value)); return true;
case "transferexchange":
case "transferExchange": target.getConfiguration().setTransferExchange(property(camelContext, boolean.class, value)); return true;
case "truststorefile":
case "trustStoreFile": target.getConfiguration().setTrustStoreFile(property(camelContext, java.io.File.class, value)); return true;
case "truststoreresource":
case "trustStoreResource": target.getConfiguration().setTrustStoreResource(property(camelContext, java.lang.String.class, value)); return true;
case "udpbytearraycodec":
case "udpByteArrayCodec": target.getConfiguration().setUdpByteArrayCodec(property(camelContext, boolean.class, value)); return true;
case "udpconnectionlesssending":
case "udpConnectionlessSending": target.getConfiguration().setUdpConnectionlessSending(property(camelContext, boolean.class, value)); return true;
case "unixdomainsocketpath":
case "unixDomainSocketPath": target.getConfiguration().setUnixDomainSocketPath(property(camelContext, java.lang.String.class, value)); return true;
case "usebytebuf":
case "useByteBuf": target.getConfiguration().setUseByteBuf(property(camelContext, boolean.class, value)); return true;
case "usingexecutorservice":
case "usingExecutorService": target.getConfiguration().setUsingExecutorService(property(camelContext, boolean.class, value)); return true;
case "workercount":
case "workerCount": target.getConfiguration().setWorkerCount(property(camelContext, int.class, value)); return true;
case "workergroup":
case "workerGroup": target.getConfiguration().setWorkerGroup(property(camelContext, io.netty.channel.EventLoopGroup.class, value)); return true;
default: return false;
}
}
@Override
public Class> getOptionType(String name, boolean ignoreCase) {
switch (ignoreCase ? name.toLowerCase() : name) {
case "allowdefaultcodec":
case "allowDefaultCodec": return boolean.class;
case "allowserializedheaders":
case "allowSerializedHeaders": return boolean.class;
case "autoappenddelimiter":
case "autoAppendDelimiter": return boolean.class;
case "backlog": return int.class;
case "bosscount":
case "bossCount": return int.class;
case "bossgroup":
case "bossGroup": return io.netty.channel.EventLoopGroup.class;
case "bridgeerrorhandler":
case "bridgeErrorHandler": return boolean.class;
case "broadcast": return boolean.class;
case "channelgroup":
case "channelGroup": return io.netty.channel.group.ChannelGroup.class;
case "clientinitializerfactory":
case "clientInitializerFactory": return org.apache.camel.component.netty.ClientInitializerFactory.class;
case "clientmode":
case "clientMode": return boolean.class;
case "connecttimeout":
case "connectTimeout": return int.class;
case "correlationmanager":
case "correlationManager": return org.apache.camel.component.netty.NettyCamelStateCorrelationManager.class;
case "decodermaxlinelength":
case "decoderMaxLineLength": return int.class;
case "decoders": return java.lang.String.class;
case "delimiter": return org.apache.camel.component.netty.TextLineDelimiter.class;
case "disconnect": return boolean.class;
case "disconnectonnoreply":
case "disconnectOnNoReply": return boolean.class;
case "enabledprotocols":
case "enabledProtocols": return java.lang.String.class;
case "encoders": return java.lang.String.class;
case "encoding": return java.lang.String.class;
case "exceptionhandler":
case "exceptionHandler": return org.apache.camel.spi.ExceptionHandler.class;
case "exchangepattern":
case "exchangePattern": return org.apache.camel.ExchangePattern.class;
case "hostnameverification":
case "hostnameVerification": return boolean.class;
case "keepalive":
case "keepAlive": return boolean.class;
case "keystorefile":
case "keyStoreFile": return java.io.File.class;
case "keystoreformat":
case "keyStoreFormat": return java.lang.String.class;
case "keystoreresource":
case "keyStoreResource": return java.lang.String.class;
case "lazychannelcreation":
case "lazyChannelCreation": return boolean.class;
case "lazystartproducer":
case "lazyStartProducer": return boolean.class;
case "nativetransport":
case "nativeTransport": return boolean.class;
case "needclientauth":
case "needClientAuth": return boolean.class;
case "nettyserverbootstrapfactory":
case "nettyServerBootstrapFactory": return org.apache.camel.component.netty.NettyServerBootstrapFactory.class;
case "networkinterface":
case "networkInterface": return java.lang.String.class;
case "noreplyloglevel":
case "noReplyLogLevel": return org.apache.camel.LoggingLevel.class;
case "options": return java.util.Map.class;
case "passphrase": return java.lang.String.class;
case "producerpoolblockwhenexhausted":
case "producerPoolBlockWhenExhausted": return boolean.class;
case "producerpoolenabled":
case "producerPoolEnabled": return boolean.class;
case "producerpoolmaxidle":
case "producerPoolMaxIdle": return int.class;
case "producerpoolmaxtotal":
case "producerPoolMaxTotal": return int.class;
case "producerpoolmaxwait":
case "producerPoolMaxWait": return long.class;
case "producerpoolminevictableidle":
case "producerPoolMinEvictableIdle": return long.class;
case "producerpoolminidle":
case "producerPoolMinIdle": return int.class;
case "receivebuffersize":
case "receiveBufferSize": return int.class;
case "receivebuffersizepredictor":
case "receiveBufferSizePredictor": return int.class;
case "reconnect": return boolean.class;
case "reconnectinterval":
case "reconnectInterval": return int.class;
case "requesttimeout":
case "requestTimeout": return long.class;
case "reuseaddress":
case "reuseAddress": return boolean.class;
case "reusechannel":
case "reuseChannel": return boolean.class;
case "securityprovider":
case "securityProvider": return java.lang.String.class;
case "sendbuffersize":
case "sendBufferSize": return int.class;
case "serverclosedchannelexceptioncaughtloglevel":
case "serverClosedChannelExceptionCaughtLogLevel": return org.apache.camel.LoggingLevel.class;
case "serverexceptioncaughtloglevel":
case "serverExceptionCaughtLogLevel": return org.apache.camel.LoggingLevel.class;
case "serverinitializerfactory":
case "serverInitializerFactory": return org.apache.camel.component.netty.ServerInitializerFactory.class;
case "ssl": return boolean.class;
case "sslclientcertheaders":
case "sslClientCertHeaders": return boolean.class;
case "sslcontextparameters":
case "sslContextParameters": return org.apache.camel.support.jsse.SSLContextParameters.class;
case "sslhandler":
case "sslHandler": return io.netty.handler.ssl.SslHandler.class;
case "sync": return boolean.class;
case "synchronous": return boolean.class;
case "tcpnodelay":
case "tcpNoDelay": return boolean.class;
case "textline": return boolean.class;
case "transferexchange":
case "transferExchange": return boolean.class;
case "truststorefile":
case "trustStoreFile": return java.io.File.class;
case "truststoreresource":
case "trustStoreResource": return java.lang.String.class;
case "udpbytearraycodec":
case "udpByteArrayCodec": return boolean.class;
case "udpconnectionlesssending":
case "udpConnectionlessSending": return boolean.class;
case "unixdomainsocketpath":
case "unixDomainSocketPath": return java.lang.String.class;
case "usebytebuf":
case "useByteBuf": return boolean.class;
case "usingexecutorservice":
case "usingExecutorService": return boolean.class;
case "workercount":
case "workerCount": return int.class;
case "workergroup":
case "workerGroup": return io.netty.channel.EventLoopGroup.class;
default: return null;
}
}
@Override
public Object getOptionValue(Object obj, String name, boolean ignoreCase) {
NettyEndpoint target = (NettyEndpoint) obj;
switch (ignoreCase ? name.toLowerCase() : name) {
case "allowdefaultcodec":
case "allowDefaultCodec": return target.getConfiguration().isAllowDefaultCodec();
case "allowserializedheaders":
case "allowSerializedHeaders": return target.getConfiguration().isAllowSerializedHeaders();
case "autoappenddelimiter":
case "autoAppendDelimiter": return target.getConfiguration().isAutoAppendDelimiter();
case "backlog": return target.getConfiguration().getBacklog();
case "bosscount":
case "bossCount": return target.getConfiguration().getBossCount();
case "bossgroup":
case "bossGroup": return target.getConfiguration().getBossGroup();
case "bridgeerrorhandler":
case "bridgeErrorHandler": return target.isBridgeErrorHandler();
case "broadcast": return target.getConfiguration().isBroadcast();
case "channelgroup":
case "channelGroup": return target.getConfiguration().getChannelGroup();
case "clientinitializerfactory":
case "clientInitializerFactory": return target.getConfiguration().getClientInitializerFactory();
case "clientmode":
case "clientMode": return target.getConfiguration().isClientMode();
case "connecttimeout":
case "connectTimeout": return target.getConfiguration().getConnectTimeout();
case "correlationmanager":
case "correlationManager": return target.getConfiguration().getCorrelationManager();
case "decodermaxlinelength":
case "decoderMaxLineLength": return target.getConfiguration().getDecoderMaxLineLength();
case "decoders": return target.getConfiguration().getDecoders();
case "delimiter": return target.getConfiguration().getDelimiter();
case "disconnect": return target.getConfiguration().isDisconnect();
case "disconnectonnoreply":
case "disconnectOnNoReply": return target.getConfiguration().isDisconnectOnNoReply();
case "enabledprotocols":
case "enabledProtocols": return target.getConfiguration().getEnabledProtocols();
case "encoders": return target.getConfiguration().getEncoders();
case "encoding": return target.getConfiguration().getEncoding();
case "exceptionhandler":
case "exceptionHandler": return target.getExceptionHandler();
case "exchangepattern":
case "exchangePattern": return target.getExchangePattern();
case "hostnameverification":
case "hostnameVerification": return target.getConfiguration().isHostnameVerification();
case "keepalive":
case "keepAlive": return target.getConfiguration().isKeepAlive();
case "keystorefile":
case "keyStoreFile": return target.getConfiguration().getKeyStoreFile();
case "keystoreformat":
case "keyStoreFormat": return target.getConfiguration().getKeyStoreFormat();
case "keystoreresource":
case "keyStoreResource": return target.getConfiguration().getKeyStoreResource();
case "lazychannelcreation":
case "lazyChannelCreation": return target.getConfiguration().isLazyChannelCreation();
case "lazystartproducer":
case "lazyStartProducer": return target.isLazyStartProducer();
case "nativetransport":
case "nativeTransport": return target.getConfiguration().isNativeTransport();
case "needclientauth":
case "needClientAuth": return target.getConfiguration().isNeedClientAuth();
case "nettyserverbootstrapfactory":
case "nettyServerBootstrapFactory": return target.getConfiguration().getNettyServerBootstrapFactory();
case "networkinterface":
case "networkInterface": return target.getConfiguration().getNetworkInterface();
case "noreplyloglevel":
case "noReplyLogLevel": return target.getConfiguration().getNoReplyLogLevel();
case "options": return target.getConfiguration().getOptions();
case "passphrase": return target.getConfiguration().getPassphrase();
case "producerpoolblockwhenexhausted":
case "producerPoolBlockWhenExhausted": return target.getConfiguration().isProducerPoolBlockWhenExhausted();
case "producerpoolenabled":
case "producerPoolEnabled": return target.getConfiguration().isProducerPoolEnabled();
case "producerpoolmaxidle":
case "producerPoolMaxIdle": return target.getConfiguration().getProducerPoolMaxIdle();
case "producerpoolmaxtotal":
case "producerPoolMaxTotal": return target.getConfiguration().getProducerPoolMaxTotal();
case "producerpoolmaxwait":
case "producerPoolMaxWait": return target.getConfiguration().getProducerPoolMaxWait();
case "producerpoolminevictableidle":
case "producerPoolMinEvictableIdle": return target.getConfiguration().getProducerPoolMinEvictableIdle();
case "producerpoolminidle":
case "producerPoolMinIdle": return target.getConfiguration().getProducerPoolMinIdle();
case "receivebuffersize":
case "receiveBufferSize": return target.getConfiguration().getReceiveBufferSize();
case "receivebuffersizepredictor":
case "receiveBufferSizePredictor": return target.getConfiguration().getReceiveBufferSizePredictor();
case "reconnect": return target.getConfiguration().isReconnect();
case "reconnectinterval":
case "reconnectInterval": return target.getConfiguration().getReconnectInterval();
case "requesttimeout":
case "requestTimeout": return target.getConfiguration().getRequestTimeout();
case "reuseaddress":
case "reuseAddress": return target.getConfiguration().isReuseAddress();
case "reusechannel":
case "reuseChannel": return target.getConfiguration().isReuseChannel();
case "securityprovider":
case "securityProvider": return target.getConfiguration().getSecurityProvider();
case "sendbuffersize":
case "sendBufferSize": return target.getConfiguration().getSendBufferSize();
case "serverclosedchannelexceptioncaughtloglevel":
case "serverClosedChannelExceptionCaughtLogLevel": return target.getConfiguration().getServerClosedChannelExceptionCaughtLogLevel();
case "serverexceptioncaughtloglevel":
case "serverExceptionCaughtLogLevel": return target.getConfiguration().getServerExceptionCaughtLogLevel();
case "serverinitializerfactory":
case "serverInitializerFactory": return target.getConfiguration().getServerInitializerFactory();
case "ssl": return target.getConfiguration().isSsl();
case "sslclientcertheaders":
case "sslClientCertHeaders": return target.getConfiguration().isSslClientCertHeaders();
case "sslcontextparameters":
case "sslContextParameters": return target.getConfiguration().getSslContextParameters();
case "sslhandler":
case "sslHandler": return target.getConfiguration().getSslHandler();
case "sync": return target.getConfiguration().isSync();
case "synchronous": return target.isSynchronous();
case "tcpnodelay":
case "tcpNoDelay": return target.getConfiguration().isTcpNoDelay();
case "textline": return target.getConfiguration().isTextline();
case "transferexchange":
case "transferExchange": return target.getConfiguration().isTransferExchange();
case "truststorefile":
case "trustStoreFile": return target.getConfiguration().getTrustStoreFile();
case "truststoreresource":
case "trustStoreResource": return target.getConfiguration().getTrustStoreResource();
case "udpbytearraycodec":
case "udpByteArrayCodec": return target.getConfiguration().isUdpByteArrayCodec();
case "udpconnectionlesssending":
case "udpConnectionlessSending": return target.getConfiguration().isUdpConnectionlessSending();
case "unixdomainsocketpath":
case "unixDomainSocketPath": return target.getConfiguration().getUnixDomainSocketPath();
case "usebytebuf":
case "useByteBuf": return target.getConfiguration().isUseByteBuf();
case "usingexecutorservice":
case "usingExecutorService": return target.getConfiguration().isUsingExecutorService();
case "workercount":
case "workerCount": return target.getConfiguration().getWorkerCount();
case "workergroup":
case "workerGroup": return target.getConfiguration().getWorkerGroup();
default: return null;
}
}
@Override
public Object getCollectionValueType(Object target, String name, boolean ignoreCase) {
switch (ignoreCase ? name.toLowerCase() : name) {
case "options": return java.lang.Object.class;
default: return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy