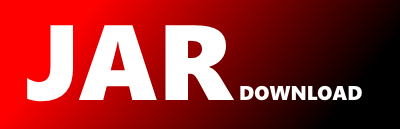
org.apache.camel.component.olingo2.api.Olingo2App Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.camel.component.olingo2.api;
import java.io.InputStream;
import java.util.List;
import java.util.Map;
import org.apache.camel.component.olingo2.api.batch.Olingo2BatchResponse;
import org.apache.olingo.odata2.api.commons.HttpStatusCodes;
import org.apache.olingo.odata2.api.edm.Edm;
import org.apache.olingo.odata2.api.ep.EntityProviderReadProperties;
import org.apache.olingo.odata2.api.ep.EntityProviderWriteProperties;
/**
* Olingo2 Client Api Interface.
*/
public interface Olingo2App {
/**
* Sets Service base URI.
*
* @param serviceUri
*/
void setServiceUri(String serviceUri);
/**
* Returns Service base URI.
*
* @return service base URI.
*/
String getServiceUri();
/**
* Sets custom Http headers to add to every service request.
*
* @param httpHeaders custom Http headers.
*/
void setHttpHeaders(Map httpHeaders);
/**
* Specify custom entity provider read properties.
*
* @param entityProviderReadProperties the custom properties to set.
*/
void setEntityProviderReadProperties(EntityProviderReadProperties entityProviderReadProperties);
/**
* Obtains the custom entity provider read properties.
*
* @return the custom read properties.
*/
EntityProviderReadProperties getEntityProviderReadProperties();
/**
* Specify custom entity provider write properties.
*
* @param entityProviderWriteProperties the custom properties to set.
*/
void setEntityProviderWriteProperties(EntityProviderWriteProperties entityProviderWriteProperties);
/**
* Obtains the custom entity provider write properties.
*
* @return the custom write properties.
*/
EntityProviderWriteProperties getEntityProviderWriteProperties();
/**
* Returns custom Http headers.
*
* @return custom Http headers.
*/
Map getHttpHeaders();
/**
* Returns content type for service calls. Defaults to application/json;charset=utf-8
.
*
* @return content type.
*/
String getContentType();
/**
* Set default service call content type.
*
* @param contentType content type.
*/
void setContentType(String contentType);
/**
* Closes resources.
*/
void close();
/**
* Reads an OData resource and invokes callback with appropriate result.
*
* @param edm Service Edm, read from calling
* read(null, "$metdata", null, responseHandler)
* @param resourcePath OData Resource path
* @param queryParams OData query params from
* http://www.odata.org/documentation/odata-version-2-0/uri-conventions#SystemQueryOptions
* @param endpointHttpHeaders HTTP Headers to add/override the component versions
* @param responseHandler callback handler
*/
void read(
Edm edm, String resourcePath, Map queryParams, Map endpointHttpHeaders,
Olingo2ResponseHandler responseHandler);
/**
* Reads an OData resource and invokes callback with the unparsed input stream.
*
* @param edm Service Edm, read from calling
* read(null, "$metdata", null, responseHandler)
* @param resourcePath OData Resource path
* @param queryParams OData query params from
* http://www.odata.org/documentation/odata-version-2-0/uri-conventions#SystemQueryOptions
* @param endpointHttpHeaders HTTP Headers to add/override the component versions
* @param responseHandler callback handler
*/
void uread(
Edm edm, String resourcePath, Map queryParams, Map endpointHttpHeaders,
Olingo2ResponseHandler responseHandler);
/**
* Deletes an OData resource and invokes callback with {@link org.apache.olingo.odata2.api.commons.HttpStatusCodes}
* on success, or with exception on failure.
*
* @param resourcePath resource path for Entry
* @param endpointHttpHeaders HTTP Headers to add/override the component versions
* @param responseHandler {@link org.apache.olingo.odata2.api.commons.HttpStatusCodes} callback handler
*/
void delete(
String resourcePath, Map endpointHttpHeaders,
Olingo2ResponseHandler responseHandler);
/**
* Creates a new OData resource.
*
* @param edm service Edm
* @param resourcePath resource path to create
* @param endpointHttpHeaders HTTP Headers to add/override the component versions
* @param data request data
* @param responseHandler callback handler
*/
void create(
Edm edm, String resourcePath, Map endpointHttpHeaders, Object data,
Olingo2ResponseHandler responseHandler);
/**
* Updates an OData resource.
*
* @param edm service Edm
* @param resourcePath resource path to update
* @param endpointHttpHeaders HTTP Headers to add/override the component versions
* @param data updated data
* @param responseHandler {@link org.apache.olingo.odata2.api.ep.entry.ODataEntry} callback handler
*/
void update(
Edm edm, String resourcePath, Map endpointHttpHeaders, Object data,
Olingo2ResponseHandler responseHandler);
/**
* Patches/merges an OData resource using HTTP PATCH.
*
* @param edm service Edm
* @param resourcePath resource path to update
* @param endpointHttpHeaders HTTP Headers to add/override the component versions
* @param data patch/merge data
* @param responseHandler {@link org.apache.olingo.odata2.api.ep.entry.ODataEntry} callback handler
*/
void patch(
Edm edm, String resourcePath, Map endpointHttpHeaders, Object data,
Olingo2ResponseHandler responseHandler);
/**
* Patches/merges an OData resource using HTTP MERGE.
*
* @param edm service Edm
* @param resourcePath resource path to update
* @param endpointHttpHeaders HTTP Headers to add/override the component versions
* @param data patch/merge data
* @param responseHandler {@link org.apache.olingo.odata2.api.ep.entry.ODataEntry} callback handler
*/
void merge(
Edm edm, String resourcePath, Map endpointHttpHeaders, Object data,
Olingo2ResponseHandler responseHandler);
/**
* Executes a batch request.
*
* @param edm service Edm
* @param endpointHttpHeaders HTTP Headers to add/override the component versions
* @param data ordered {@link org.apache.camel.component.olingo2.api.batch.Olingo2BatchRequest} list
* @param responseHandler callback handler
*/
void batch(
Edm edm, Map endpointHttpHeaders, Object data,
Olingo2ResponseHandler> responseHandler);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy