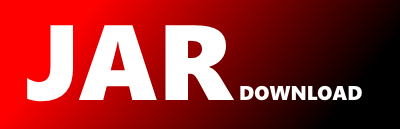
org.apache.camel.maven.packaging.PackageMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of camel-package-maven-plugin Show documentation
Show all versions of camel-package-maven-plugin Show documentation
Maven plugin to help package Camel components and plugins
/**
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.camel.maven.packaging;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.util.Properties;
import org.apache.maven.model.Resource;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.project.MavenProject;
import org.apache.maven.project.MavenProjectHelper;
/**
* Analyses the Camel plugins in a project and generates extra descriptor information for easier auto-discovery in Camel.
*
* @goal generate-components-list
* @execute phase="generate-resources"
*/
public class PackageMojo extends AbstractMojo {
/**
* The maven project.
*
* @parameter property="project"
* @required
* @readonly
*/
protected MavenProject project;
/**
* The output directory for generated components file
*
* @parameter default-value="${project.build.directory}/generated/camel/components"
*/
protected File outDir;
/**
* Maven ProjectHelper.
*
* @component
* @readonly
*/
private MavenProjectHelper projectHelper;
/**
* Execute goal.
*
* @throws MojoExecutionException execution of the main class or one of the
* threads it generated failed.
* @throws MojoFailureException something bad happened...
*/
public void execute() throws MojoExecutionException, MojoFailureException {
File camelMetaDir = new File(outDir, "META-INF/services/org/apache/camel/");
StringBuilder buffer = new StringBuilder();
int count = 0;
for (Resource r : project.getBuild().getResources()) {
File f = new File(r.getDirectory());
if (!f.exists()) {
f = new File(project.getBasedir(), r.getDirectory());
}
f = new File(f, "META-INF/services/org/apache/camel/component");
if (f.exists() && f.isDirectory()) {
File[] files = f.listFiles();
if (files != null) {
for (File file : files) {
String name = file.getName();
if (name.charAt(0) != '.') {
count++;
if (buffer.length() > 0) {
buffer.append(" ");
}
buffer.append(name);
}
}
}
}
}
if (count > 0) {
Properties properties = new Properties();
String names = buffer.toString();
properties.put("components", names);
properties.put("groupId", project.getGroupId());
properties.put("artifactId", project.getArtifactId());
properties.put("version", project.getVersion());
properties.put("projectName", project.getName());
// description is optional
if (project.getDescription() != null) {
properties.put("projectDescription", project.getDescription());
}
camelMetaDir.mkdirs();
File outFile = new File(camelMetaDir, "component.properties");
try {
properties.store(new FileWriter(outFile), "Generated by camel-package-maven-plugin");
getLog().info("Generated " + outFile + " containing the Camel " + (count > 1 ? "components " : "component ") + names);
if (projectHelper != null) {
List includes = new ArrayList();
includes.add("**/component.properties");
projectHelper.addResource(this.project, outDir.getPath(), includes, new ArrayList());
projectHelper.attachArtifact(this.project, "properties", "camelComponent", outFile);
}
} catch (IOException e) {
throw new MojoExecutionException("Failed to write properties to " + outFile + ". Reason: " + e, e);
}
} else {
getLog().debug("No META-INF/services/org/apache/camel/component directory found. Are you sure you have created a Camel component?");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy