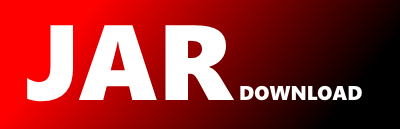
org.apache.camel.component.rabbitmq.RabbitMQEndpointConfigurer Maven / Gradle / Ivy
The newest version!
/* Generated by camel build tools - do NOT edit this file! */
package org.apache.camel.component.rabbitmq;
import java.util.Map;
import org.apache.camel.CamelContext;
import org.apache.camel.spi.ExtendedPropertyConfigurerGetter;
import org.apache.camel.spi.PropertyConfigurerGetter;
import org.apache.camel.spi.ConfigurerStrategy;
import org.apache.camel.spi.GeneratedPropertyConfigurer;
import org.apache.camel.util.CaseInsensitiveMap;
import org.apache.camel.support.component.PropertyConfigurerSupport;
/**
* Generated by camel build tools - do NOT edit this file!
*/
@SuppressWarnings("unchecked")
public class RabbitMQEndpointConfigurer extends PropertyConfigurerSupport implements GeneratedPropertyConfigurer, PropertyConfigurerGetter {
@Override
public boolean configure(CamelContext camelContext, Object obj, String name, Object value, boolean ignoreCase) {
RabbitMQEndpoint target = (RabbitMQEndpoint) obj;
switch (ignoreCase ? name.toLowerCase() : name) {
case "additionalheaders":
case "additionalHeaders": target.setAdditionalHeaders(property(camelContext, java.util.Map.class, value)); return true;
case "additionalproperties":
case "additionalProperties": target.setAdditionalProperties(property(camelContext, java.util.Map.class, value)); return true;
case "addresses": target.setAddresses(property(camelContext, java.lang.String.class, value)); return true;
case "allowcustomheaders":
case "allowCustomHeaders": target.setAllowCustomHeaders(property(camelContext, boolean.class, value)); return true;
case "allowmessagebodyserialization":
case "allowMessageBodySerialization": target.setAllowMessageBodySerialization(property(camelContext, boolean.class, value)); return true;
case "allownullheaders":
case "allowNullHeaders": target.setAllowNullHeaders(property(camelContext, boolean.class, value)); return true;
case "args": target.setArgs(property(camelContext, java.util.Map.class, value)); return true;
case "autoack":
case "autoAck": target.setAutoAck(property(camelContext, boolean.class, value)); return true;
case "autodelete":
case "autoDelete": target.setAutoDelete(property(camelContext, boolean.class, value)); return true;
case "automaticrecoveryenabled":
case "automaticRecoveryEnabled": target.setAutomaticRecoveryEnabled(property(camelContext, java.lang.Boolean.class, value)); return true;
case "bridgeendpoint":
case "bridgeEndpoint": target.setBridgeEndpoint(property(camelContext, boolean.class, value)); return true;
case "bridgeerrorhandler":
case "bridgeErrorHandler": target.setBridgeErrorHandler(property(camelContext, boolean.class, value)); return true;
case "channelpoolmaxsize":
case "channelPoolMaxSize": target.setChannelPoolMaxSize(property(camelContext, int.class, value)); return true;
case "channelpoolmaxwait":
case "channelPoolMaxWait": target.setChannelPoolMaxWait(property(camelContext, long.class, value)); return true;
case "clientproperties":
case "clientProperties": target.setClientProperties(property(camelContext, java.util.Map.class, value)); return true;
case "concurrentconsumers":
case "concurrentConsumers": target.setConcurrentConsumers(property(camelContext, int.class, value)); return true;
case "connectionfactory":
case "connectionFactory": target.setConnectionFactory(property(camelContext, com.rabbitmq.client.ConnectionFactory.class, value)); return true;
case "connectionfactoryexceptionhandler":
case "connectionFactoryExceptionHandler": target.setConnectionFactoryExceptionHandler(property(camelContext, com.rabbitmq.client.ExceptionHandler.class, value)); return true;
case "connectiontimeout":
case "connectionTimeout": target.setConnectionTimeout(property(camelContext, int.class, value)); return true;
case "consumertag":
case "consumerTag": target.setConsumerTag(property(camelContext, java.lang.String.class, value)); return true;
case "deadletterexchange":
case "deadLetterExchange": target.setDeadLetterExchange(property(camelContext, java.lang.String.class, value)); return true;
case "deadletterexchangetype":
case "deadLetterExchangeType": target.setDeadLetterExchangeType(property(camelContext, java.lang.String.class, value)); return true;
case "deadletterqueue":
case "deadLetterQueue": target.setDeadLetterQueue(property(camelContext, java.lang.String.class, value)); return true;
case "deadletterroutingkey":
case "deadLetterRoutingKey": target.setDeadLetterRoutingKey(property(camelContext, java.lang.String.class, value)); return true;
case "declare": target.setDeclare(property(camelContext, boolean.class, value)); return true;
case "durable": target.setDurable(property(camelContext, boolean.class, value)); return true;
case "exceptionhandler":
case "exceptionHandler": target.setExceptionHandler(property(camelContext, org.apache.camel.spi.ExceptionHandler.class, value)); return true;
case "exchangepattern":
case "exchangePattern": target.setExchangePattern(property(camelContext, org.apache.camel.ExchangePattern.class, value)); return true;
case "exchangetype":
case "exchangeType": target.setExchangeType(property(camelContext, java.lang.String.class, value)); return true;
case "exclusive": target.setExclusive(property(camelContext, boolean.class, value)); return true;
case "exclusiveconsumer":
case "exclusiveConsumer": target.setExclusiveConsumer(property(camelContext, boolean.class, value)); return true;
case "guaranteeddeliveries":
case "guaranteedDeliveries": target.setGuaranteedDeliveries(property(camelContext, boolean.class, value)); return true;
case "hostname": target.setHostname(property(camelContext, java.lang.String.class, value)); return true;
case "immediate": target.setImmediate(property(camelContext, boolean.class, value)); return true;
case "lazystartproducer":
case "lazyStartProducer": target.setLazyStartProducer(property(camelContext, boolean.class, value)); return true;
case "mandatory": target.setMandatory(property(camelContext, boolean.class, value)); return true;
case "networkrecoveryinterval":
case "networkRecoveryInterval": target.setNetworkRecoveryInterval(property(camelContext, java.lang.Integer.class, value)); return true;
case "passive": target.setPassive(property(camelContext, boolean.class, value)); return true;
case "password": target.setPassword(property(camelContext, java.lang.String.class, value)); return true;
case "portnumber":
case "portNumber": target.setPortNumber(property(camelContext, int.class, value)); return true;
case "prefetchcount":
case "prefetchCount": target.setPrefetchCount(property(camelContext, int.class, value)); return true;
case "prefetchenabled":
case "prefetchEnabled": target.setPrefetchEnabled(property(camelContext, boolean.class, value)); return true;
case "prefetchglobal":
case "prefetchGlobal": target.setPrefetchGlobal(property(camelContext, boolean.class, value)); return true;
case "prefetchsize":
case "prefetchSize": target.setPrefetchSize(property(camelContext, int.class, value)); return true;
case "publisheracknowledgements":
case "publisherAcknowledgements": target.setPublisherAcknowledgements(property(camelContext, boolean.class, value)); return true;
case "publisheracknowledgementstimeout":
case "publisherAcknowledgementsTimeout": target.setPublisherAcknowledgementsTimeout(property(camelContext, long.class, value)); return true;
case "queue": target.setQueue(property(camelContext, java.lang.String.class, value)); return true;
case "requeue":
case "reQueue": target.setReQueue(property(camelContext, boolean.class, value)); return true;
case "recoverfromdeclareexception":
case "recoverFromDeclareException": target.setRecoverFromDeclareException(property(camelContext, boolean.class, value)); return true;
case "requesttimeout":
case "requestTimeout": target.setRequestTimeout(property(camelContext, long.class, value)); return true;
case "requesttimeoutcheckerinterval":
case "requestTimeoutCheckerInterval": target.setRequestTimeoutCheckerInterval(property(camelContext, long.class, value)); return true;
case "requestedchannelmax":
case "requestedChannelMax": target.setRequestedChannelMax(property(camelContext, int.class, value)); return true;
case "requestedframemax":
case "requestedFrameMax": target.setRequestedFrameMax(property(camelContext, int.class, value)); return true;
case "requestedheartbeat":
case "requestedHeartbeat": target.setRequestedHeartbeat(property(camelContext, int.class, value)); return true;
case "routingkey":
case "routingKey": target.setRoutingKey(property(camelContext, java.lang.String.class, value)); return true;
case "skipdlqdeclare":
case "skipDlqDeclare": target.setSkipDlqDeclare(property(camelContext, boolean.class, value)); return true;
case "skipexchangedeclare":
case "skipExchangeDeclare": target.setSkipExchangeDeclare(property(camelContext, boolean.class, value)); return true;
case "skipqueuebind":
case "skipQueueBind": target.setSkipQueueBind(property(camelContext, boolean.class, value)); return true;
case "skipqueuedeclare":
case "skipQueueDeclare": target.setSkipQueueDeclare(property(camelContext, boolean.class, value)); return true;
case "sslprotocol":
case "sslProtocol": target.setSslProtocol(property(camelContext, java.lang.String.class, value)); return true;
case "threadpoolsize":
case "threadPoolSize": target.setThreadPoolSize(property(camelContext, int.class, value)); return true;
case "topologyrecoveryenabled":
case "topologyRecoveryEnabled": target.setTopologyRecoveryEnabled(property(camelContext, java.lang.Boolean.class, value)); return true;
case "transferexception":
case "transferException": target.setTransferException(property(camelContext, boolean.class, value)); return true;
case "trustmanager":
case "trustManager": target.setTrustManager(property(camelContext, javax.net.ssl.TrustManager.class, value)); return true;
case "username": target.setUsername(property(camelContext, java.lang.String.class, value)); return true;
case "vhost": target.setVhost(property(camelContext, java.lang.String.class, value)); return true;
default: return false;
}
}
@Override
public Class> getOptionType(String name, boolean ignoreCase) {
switch (ignoreCase ? name.toLowerCase() : name) {
case "additionalheaders":
case "additionalHeaders": return java.util.Map.class;
case "additionalproperties":
case "additionalProperties": return java.util.Map.class;
case "addresses": return java.lang.String.class;
case "allowcustomheaders":
case "allowCustomHeaders": return boolean.class;
case "allowmessagebodyserialization":
case "allowMessageBodySerialization": return boolean.class;
case "allownullheaders":
case "allowNullHeaders": return boolean.class;
case "args": return java.util.Map.class;
case "autoack":
case "autoAck": return boolean.class;
case "autodelete":
case "autoDelete": return boolean.class;
case "automaticrecoveryenabled":
case "automaticRecoveryEnabled": return java.lang.Boolean.class;
case "bridgeendpoint":
case "bridgeEndpoint": return boolean.class;
case "bridgeerrorhandler":
case "bridgeErrorHandler": return boolean.class;
case "channelpoolmaxsize":
case "channelPoolMaxSize": return int.class;
case "channelpoolmaxwait":
case "channelPoolMaxWait": return long.class;
case "clientproperties":
case "clientProperties": return java.util.Map.class;
case "concurrentconsumers":
case "concurrentConsumers": return int.class;
case "connectionfactory":
case "connectionFactory": return com.rabbitmq.client.ConnectionFactory.class;
case "connectionfactoryexceptionhandler":
case "connectionFactoryExceptionHandler": return com.rabbitmq.client.ExceptionHandler.class;
case "connectiontimeout":
case "connectionTimeout": return int.class;
case "consumertag":
case "consumerTag": return java.lang.String.class;
case "deadletterexchange":
case "deadLetterExchange": return java.lang.String.class;
case "deadletterexchangetype":
case "deadLetterExchangeType": return java.lang.String.class;
case "deadletterqueue":
case "deadLetterQueue": return java.lang.String.class;
case "deadletterroutingkey":
case "deadLetterRoutingKey": return java.lang.String.class;
case "declare": return boolean.class;
case "durable": return boolean.class;
case "exceptionhandler":
case "exceptionHandler": return org.apache.camel.spi.ExceptionHandler.class;
case "exchangepattern":
case "exchangePattern": return org.apache.camel.ExchangePattern.class;
case "exchangetype":
case "exchangeType": return java.lang.String.class;
case "exclusive": return boolean.class;
case "exclusiveconsumer":
case "exclusiveConsumer": return boolean.class;
case "guaranteeddeliveries":
case "guaranteedDeliveries": return boolean.class;
case "hostname": return java.lang.String.class;
case "immediate": return boolean.class;
case "lazystartproducer":
case "lazyStartProducer": return boolean.class;
case "mandatory": return boolean.class;
case "networkrecoveryinterval":
case "networkRecoveryInterval": return java.lang.Integer.class;
case "passive": return boolean.class;
case "password": return java.lang.String.class;
case "portnumber":
case "portNumber": return int.class;
case "prefetchcount":
case "prefetchCount": return int.class;
case "prefetchenabled":
case "prefetchEnabled": return boolean.class;
case "prefetchglobal":
case "prefetchGlobal": return boolean.class;
case "prefetchsize":
case "prefetchSize": return int.class;
case "publisheracknowledgements":
case "publisherAcknowledgements": return boolean.class;
case "publisheracknowledgementstimeout":
case "publisherAcknowledgementsTimeout": return long.class;
case "queue": return java.lang.String.class;
case "requeue":
case "reQueue": return boolean.class;
case "recoverfromdeclareexception":
case "recoverFromDeclareException": return boolean.class;
case "requesttimeout":
case "requestTimeout": return long.class;
case "requesttimeoutcheckerinterval":
case "requestTimeoutCheckerInterval": return long.class;
case "requestedchannelmax":
case "requestedChannelMax": return int.class;
case "requestedframemax":
case "requestedFrameMax": return int.class;
case "requestedheartbeat":
case "requestedHeartbeat": return int.class;
case "routingkey":
case "routingKey": return java.lang.String.class;
case "skipdlqdeclare":
case "skipDlqDeclare": return boolean.class;
case "skipexchangedeclare":
case "skipExchangeDeclare": return boolean.class;
case "skipqueuebind":
case "skipQueueBind": return boolean.class;
case "skipqueuedeclare":
case "skipQueueDeclare": return boolean.class;
case "sslprotocol":
case "sslProtocol": return java.lang.String.class;
case "threadpoolsize":
case "threadPoolSize": return int.class;
case "topologyrecoveryenabled":
case "topologyRecoveryEnabled": return java.lang.Boolean.class;
case "transferexception":
case "transferException": return boolean.class;
case "trustmanager":
case "trustManager": return javax.net.ssl.TrustManager.class;
case "username": return java.lang.String.class;
case "vhost": return java.lang.String.class;
default: return null;
}
}
@Override
public Object getOptionValue(Object obj, String name, boolean ignoreCase) {
RabbitMQEndpoint target = (RabbitMQEndpoint) obj;
switch (ignoreCase ? name.toLowerCase() : name) {
case "additionalheaders":
case "additionalHeaders": return target.getAdditionalHeaders();
case "additionalproperties":
case "additionalProperties": return target.getAdditionalProperties();
case "addresses": return target.getAddresses();
case "allowcustomheaders":
case "allowCustomHeaders": return target.isAllowCustomHeaders();
case "allowmessagebodyserialization":
case "allowMessageBodySerialization": return target.isAllowMessageBodySerialization();
case "allownullheaders":
case "allowNullHeaders": return target.isAllowNullHeaders();
case "args": return target.getArgs();
case "autoack":
case "autoAck": return target.isAutoAck();
case "autodelete":
case "autoDelete": return target.isAutoDelete();
case "automaticrecoveryenabled":
case "automaticRecoveryEnabled": return target.getAutomaticRecoveryEnabled();
case "bridgeendpoint":
case "bridgeEndpoint": return target.isBridgeEndpoint();
case "bridgeerrorhandler":
case "bridgeErrorHandler": return target.isBridgeErrorHandler();
case "channelpoolmaxsize":
case "channelPoolMaxSize": return target.getChannelPoolMaxSize();
case "channelpoolmaxwait":
case "channelPoolMaxWait": return target.getChannelPoolMaxWait();
case "clientproperties":
case "clientProperties": return target.getClientProperties();
case "concurrentconsumers":
case "concurrentConsumers": return target.getConcurrentConsumers();
case "connectionfactory":
case "connectionFactory": return target.getConnectionFactory();
case "connectionfactoryexceptionhandler":
case "connectionFactoryExceptionHandler": return target.getConnectionFactoryExceptionHandler();
case "connectiontimeout":
case "connectionTimeout": return target.getConnectionTimeout();
case "consumertag":
case "consumerTag": return target.getConsumerTag();
case "deadletterexchange":
case "deadLetterExchange": return target.getDeadLetterExchange();
case "deadletterexchangetype":
case "deadLetterExchangeType": return target.getDeadLetterExchangeType();
case "deadletterqueue":
case "deadLetterQueue": return target.getDeadLetterQueue();
case "deadletterroutingkey":
case "deadLetterRoutingKey": return target.getDeadLetterRoutingKey();
case "declare": return target.isDeclare();
case "durable": return target.isDurable();
case "exceptionhandler":
case "exceptionHandler": return target.getExceptionHandler();
case "exchangepattern":
case "exchangePattern": return target.getExchangePattern();
case "exchangetype":
case "exchangeType": return target.getExchangeType();
case "exclusive": return target.isExclusive();
case "exclusiveconsumer":
case "exclusiveConsumer": return target.isExclusiveConsumer();
case "guaranteeddeliveries":
case "guaranteedDeliveries": return target.isGuaranteedDeliveries();
case "hostname": return target.getHostname();
case "immediate": return target.isImmediate();
case "lazystartproducer":
case "lazyStartProducer": return target.isLazyStartProducer();
case "mandatory": return target.isMandatory();
case "networkrecoveryinterval":
case "networkRecoveryInterval": return target.getNetworkRecoveryInterval();
case "passive": return target.isPassive();
case "password": return target.getPassword();
case "portnumber":
case "portNumber": return target.getPortNumber();
case "prefetchcount":
case "prefetchCount": return target.getPrefetchCount();
case "prefetchenabled":
case "prefetchEnabled": return target.isPrefetchEnabled();
case "prefetchglobal":
case "prefetchGlobal": return target.isPrefetchGlobal();
case "prefetchsize":
case "prefetchSize": return target.getPrefetchSize();
case "publisheracknowledgements":
case "publisherAcknowledgements": return target.isPublisherAcknowledgements();
case "publisheracknowledgementstimeout":
case "publisherAcknowledgementsTimeout": return target.getPublisherAcknowledgementsTimeout();
case "queue": return target.getQueue();
case "requeue":
case "reQueue": return target.isReQueue();
case "recoverfromdeclareexception":
case "recoverFromDeclareException": return target.isRecoverFromDeclareException();
case "requesttimeout":
case "requestTimeout": return target.getRequestTimeout();
case "requesttimeoutcheckerinterval":
case "requestTimeoutCheckerInterval": return target.getRequestTimeoutCheckerInterval();
case "requestedchannelmax":
case "requestedChannelMax": return target.getRequestedChannelMax();
case "requestedframemax":
case "requestedFrameMax": return target.getRequestedFrameMax();
case "requestedheartbeat":
case "requestedHeartbeat": return target.getRequestedHeartbeat();
case "routingkey":
case "routingKey": return target.getRoutingKey();
case "skipdlqdeclare":
case "skipDlqDeclare": return target.isSkipDlqDeclare();
case "skipexchangedeclare":
case "skipExchangeDeclare": return target.isSkipExchangeDeclare();
case "skipqueuebind":
case "skipQueueBind": return target.isSkipQueueBind();
case "skipqueuedeclare":
case "skipQueueDeclare": return target.isSkipQueueDeclare();
case "sslprotocol":
case "sslProtocol": return target.getSslProtocol();
case "threadpoolsize":
case "threadPoolSize": return target.getThreadPoolSize();
case "topologyrecoveryenabled":
case "topologyRecoveryEnabled": return target.getTopologyRecoveryEnabled();
case "transferexception":
case "transferException": return target.isTransferException();
case "trustmanager":
case "trustManager": return target.getTrustManager();
case "username": return target.getUsername();
case "vhost": return target.getVhost();
default: return null;
}
}
@Override
public Object getCollectionValueType(Object target, String name, boolean ignoreCase) {
switch (ignoreCase ? name.toLowerCase() : name) {
case "additionalheaders":
case "additionalHeaders": return java.lang.Object.class;
case "additionalproperties":
case "additionalProperties": return java.lang.Object.class;
case "args": return java.lang.Object.class;
case "clientproperties":
case "clientProperties": return java.lang.Object.class;
default: return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy