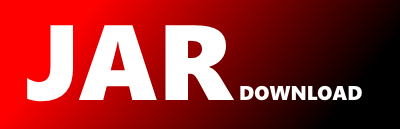
org.apache.camel.component.resteasy.ResteasyEndpointConfigurer Maven / Gradle / Ivy
/* Generated by camel build tools - do NOT edit this file! */
package org.apache.camel.component.resteasy;
import java.util.Map;
import org.apache.camel.CamelContext;
import org.apache.camel.spi.ExtendedPropertyConfigurerGetter;
import org.apache.camel.spi.PropertyConfigurerGetter;
import org.apache.camel.spi.ConfigurerStrategy;
import org.apache.camel.spi.GeneratedPropertyConfigurer;
import org.apache.camel.util.CaseInsensitiveMap;
import org.apache.camel.component.http.HttpEndpointConfigurer;
/**
* Generated by camel build tools - do NOT edit this file!
*/
@SuppressWarnings("unchecked")
public class ResteasyEndpointConfigurer extends HttpEndpointConfigurer implements GeneratedPropertyConfigurer, PropertyConfigurerGetter {
@Override
public boolean configure(CamelContext camelContext, Object obj, String name, Object value, boolean ignoreCase) {
ResteasyEndpoint target = (ResteasyEndpoint) obj;
switch (ignoreCase ? name.toLowerCase() : name) {
case "async": target.setAsync(property(camelContext, boolean.class, value)); return true;
case "bridgeerrorhandler":
case "bridgeErrorHandler": target.setBridgeErrorHandler(property(camelContext, boolean.class, value)); return true;
case "eagercheckcontentavailable":
case "eagerCheckContentAvailable": target.setEagerCheckContentAvailable(property(camelContext, boolean.class, value)); return true;
case "exceptionhandler":
case "exceptionHandler": target.setExceptionHandler(property(camelContext, org.apache.camel.spi.ExceptionHandler.class, value)); return true;
case "exchangepattern":
case "exchangePattern": target.setExchangePattern(property(camelContext, org.apache.camel.ExchangePattern.class, value)); return true;
case "httpmethodrestrict":
case "httpMethodRestrict": target.setHttpMethodRestrict(property(camelContext, java.lang.String.class, value)); return true;
case "maphttpmessagebody":
case "mapHttpMessageBody": target.setMapHttpMessageBody(property(camelContext, boolean.class, value)); return true;
case "maphttpmessageformurlencodedbody":
case "mapHttpMessageFormUrlEncodedBody": target.setMapHttpMessageFormUrlEncodedBody(property(camelContext, boolean.class, value)); return true;
case "maphttpmessageheaders":
case "mapHttpMessageHeaders": target.setMapHttpMessageHeaders(property(camelContext, boolean.class, value)); return true;
case "muteexception":
case "muteException": target.setMuteException(property(camelContext, boolean.class, value)); return true;
case "optionsenabled":
case "optionsEnabled": target.setOptionsEnabled(property(camelContext, boolean.class, value)); return true;
case "password": target.setPassword(property(camelContext, java.lang.String.class, value)); return true;
case "proxyclientclass":
case "proxyClientClass": target.setProxyClientClass(property(camelContext, java.lang.String.class, value)); return true;
case "responsebuffersize":
case "responseBufferSize": target.setResponseBufferSize(property(camelContext, java.lang.Integer.class, value)); return true;
case "resteasymethod":
case "resteasyMethod": target.setResteasyMethod(property(camelContext, java.lang.String.class, value)); return true;
case "servletname":
case "servletName": target.setServletName(property(camelContext, java.lang.String.class, value)); return true;
case "sethttpresponseduringprocessing":
case "setHttpResponseDuringProcessing": target.setSetHttpResponseDuringProcessing(property(camelContext, java.lang.Boolean.class, value)); return true;
case "skipservletprocessing":
case "skipServletProcessing": target.setSkipServletProcessing(property(camelContext, java.lang.Boolean.class, value)); return true;
case "traceenabled":
case "traceEnabled": target.setTraceEnabled(property(camelContext, boolean.class, value)); return true;
case "username": target.setUsername(property(camelContext, java.lang.String.class, value)); return true;
default: return super.configure(camelContext, obj, name, value, ignoreCase);
}
}
@Override
public Class> getOptionType(String name, boolean ignoreCase) {
switch (ignoreCase ? name.toLowerCase() : name) {
case "async": return boolean.class;
case "bridgeerrorhandler":
case "bridgeErrorHandler": return boolean.class;
case "eagercheckcontentavailable":
case "eagerCheckContentAvailable": return boolean.class;
case "exceptionhandler":
case "exceptionHandler": return org.apache.camel.spi.ExceptionHandler.class;
case "exchangepattern":
case "exchangePattern": return org.apache.camel.ExchangePattern.class;
case "httpmethodrestrict":
case "httpMethodRestrict": return java.lang.String.class;
case "maphttpmessagebody":
case "mapHttpMessageBody": return boolean.class;
case "maphttpmessageformurlencodedbody":
case "mapHttpMessageFormUrlEncodedBody": return boolean.class;
case "maphttpmessageheaders":
case "mapHttpMessageHeaders": return boolean.class;
case "muteexception":
case "muteException": return boolean.class;
case "optionsenabled":
case "optionsEnabled": return boolean.class;
case "password": return java.lang.String.class;
case "proxyclientclass":
case "proxyClientClass": return java.lang.String.class;
case "responsebuffersize":
case "responseBufferSize": return java.lang.Integer.class;
case "resteasymethod":
case "resteasyMethod": return java.lang.String.class;
case "servletname":
case "servletName": return java.lang.String.class;
case "sethttpresponseduringprocessing":
case "setHttpResponseDuringProcessing": return java.lang.Boolean.class;
case "skipservletprocessing":
case "skipServletProcessing": return java.lang.Boolean.class;
case "traceenabled":
case "traceEnabled": return boolean.class;
case "username": return java.lang.String.class;
default: return super.getOptionType(name, ignoreCase);
}
}
@Override
public Object getOptionValue(Object obj, String name, boolean ignoreCase) {
ResteasyEndpoint target = (ResteasyEndpoint) obj;
switch (ignoreCase ? name.toLowerCase() : name) {
case "async": return target.isAsync();
case "bridgeerrorhandler":
case "bridgeErrorHandler": return target.isBridgeErrorHandler();
case "eagercheckcontentavailable":
case "eagerCheckContentAvailable": return target.isEagerCheckContentAvailable();
case "exceptionhandler":
case "exceptionHandler": return target.getExceptionHandler();
case "exchangepattern":
case "exchangePattern": return target.getExchangePattern();
case "httpmethodrestrict":
case "httpMethodRestrict": return target.getHttpMethodRestrict();
case "maphttpmessagebody":
case "mapHttpMessageBody": return target.isMapHttpMessageBody();
case "maphttpmessageformurlencodedbody":
case "mapHttpMessageFormUrlEncodedBody": return target.isMapHttpMessageFormUrlEncodedBody();
case "maphttpmessageheaders":
case "mapHttpMessageHeaders": return target.isMapHttpMessageHeaders();
case "muteexception":
case "muteException": return target.isMuteException();
case "optionsenabled":
case "optionsEnabled": return target.isOptionsEnabled();
case "password": return target.getPassword();
case "proxyclientclass":
case "proxyClientClass": return target.getProxyClientClass();
case "responsebuffersize":
case "responseBufferSize": return target.getResponseBufferSize();
case "resteasymethod":
case "resteasyMethod": return target.getResteasyMethod();
case "servletname":
case "servletName": return target.getServletName();
case "sethttpresponseduringprocessing":
case "setHttpResponseDuringProcessing": return target.getSetHttpResponseDuringProcessing();
case "skipservletprocessing":
case "skipServletProcessing": return target.getSkipServletProcessing();
case "traceenabled":
case "traceEnabled": return target.isTraceEnabled();
case "username": return target.getUsername();
default: return super.getOptionValue(obj, name, ignoreCase);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy