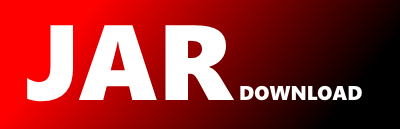
com.salesforce.eventbus.protobuf.PubSubGrpc Maven / Gradle / Ivy
package com.salesforce.eventbus.protobuf;
import static io.grpc.MethodDescriptor.generateFullMethodName;
/**
*
* The Pub/Sub API provides a single interface for publishing and subscribing to platform events, including real-time
* event monitoring events, and change data capture events. The Pub/Sub API is a gRPC API that is based on HTTP/2.
* A session token is needed to authenticate. Any of the Salesforce supported
* OAuth flows can be used to obtain a session token:
* https://help.salesforce.com/articleView?id=sf.remoteaccess_oauth_flows.htm&type=5
* For each RPC, a client needs to pass authentication information
* as metadata headers (https://www.grpc.io/docs/guides/concepts/#metadata) with their method call.
* For Salesforce session token authentication, use:
* accesstoken : access token
* instanceurl : Salesforce instance URL
* tenantid : tenant/org id of the client
* StatusException is thrown in case of response failure for any request.
*
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.57.1)",
comments = "Source: pubsub_api.proto")
@io.grpc.stub.annotations.GrpcGenerated
public final class PubSubGrpc {
private PubSubGrpc() {}
public static final java.lang.String SERVICE_NAME = "eventbus.v1.PubSub";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getSubscribeMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Subscribe",
requestType = com.salesforce.eventbus.protobuf.FetchRequest.class,
responseType = com.salesforce.eventbus.protobuf.FetchResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.BIDI_STREAMING)
public static io.grpc.MethodDescriptor getSubscribeMethod() {
io.grpc.MethodDescriptor getSubscribeMethod;
if ((getSubscribeMethod = PubSubGrpc.getSubscribeMethod) == null) {
synchronized (PubSubGrpc.class) {
if ((getSubscribeMethod = PubSubGrpc.getSubscribeMethod) == null) {
PubSubGrpc.getSubscribeMethod = getSubscribeMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.BIDI_STREAMING)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Subscribe"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.salesforce.eventbus.protobuf.FetchRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.salesforce.eventbus.protobuf.FetchResponse.getDefaultInstance()))
.setSchemaDescriptor(new PubSubMethodDescriptorSupplier("Subscribe"))
.build();
}
}
}
return getSubscribeMethod;
}
private static volatile io.grpc.MethodDescriptor getGetSchemaMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetSchema",
requestType = com.salesforce.eventbus.protobuf.SchemaRequest.class,
responseType = com.salesforce.eventbus.protobuf.SchemaInfo.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetSchemaMethod() {
io.grpc.MethodDescriptor getGetSchemaMethod;
if ((getGetSchemaMethod = PubSubGrpc.getGetSchemaMethod) == null) {
synchronized (PubSubGrpc.class) {
if ((getGetSchemaMethod = PubSubGrpc.getGetSchemaMethod) == null) {
PubSubGrpc.getGetSchemaMethod = getGetSchemaMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetSchema"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.salesforce.eventbus.protobuf.SchemaRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.salesforce.eventbus.protobuf.SchemaInfo.getDefaultInstance()))
.setSchemaDescriptor(new PubSubMethodDescriptorSupplier("GetSchema"))
.build();
}
}
}
return getGetSchemaMethod;
}
private static volatile io.grpc.MethodDescriptor getGetTopicMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetTopic",
requestType = com.salesforce.eventbus.protobuf.TopicRequest.class,
responseType = com.salesforce.eventbus.protobuf.TopicInfo.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetTopicMethod() {
io.grpc.MethodDescriptor getGetTopicMethod;
if ((getGetTopicMethod = PubSubGrpc.getGetTopicMethod) == null) {
synchronized (PubSubGrpc.class) {
if ((getGetTopicMethod = PubSubGrpc.getGetTopicMethod) == null) {
PubSubGrpc.getGetTopicMethod = getGetTopicMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetTopic"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.salesforce.eventbus.protobuf.TopicRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.salesforce.eventbus.protobuf.TopicInfo.getDefaultInstance()))
.setSchemaDescriptor(new PubSubMethodDescriptorSupplier("GetTopic"))
.build();
}
}
}
return getGetTopicMethod;
}
private static volatile io.grpc.MethodDescriptor getPublishMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "Publish",
requestType = com.salesforce.eventbus.protobuf.PublishRequest.class,
responseType = com.salesforce.eventbus.protobuf.PublishResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getPublishMethod() {
io.grpc.MethodDescriptor getPublishMethod;
if ((getPublishMethod = PubSubGrpc.getPublishMethod) == null) {
synchronized (PubSubGrpc.class) {
if ((getPublishMethod = PubSubGrpc.getPublishMethod) == null) {
PubSubGrpc.getPublishMethod = getPublishMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "Publish"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.salesforce.eventbus.protobuf.PublishRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.salesforce.eventbus.protobuf.PublishResponse.getDefaultInstance()))
.setSchemaDescriptor(new PubSubMethodDescriptorSupplier("Publish"))
.build();
}
}
}
return getPublishMethod;
}
private static volatile io.grpc.MethodDescriptor getPublishStreamMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "PublishStream",
requestType = com.salesforce.eventbus.protobuf.PublishRequest.class,
responseType = com.salesforce.eventbus.protobuf.PublishResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.BIDI_STREAMING)
public static io.grpc.MethodDescriptor getPublishStreamMethod() {
io.grpc.MethodDescriptor getPublishStreamMethod;
if ((getPublishStreamMethod = PubSubGrpc.getPublishStreamMethod) == null) {
synchronized (PubSubGrpc.class) {
if ((getPublishStreamMethod = PubSubGrpc.getPublishStreamMethod) == null) {
PubSubGrpc.getPublishStreamMethod = getPublishStreamMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.BIDI_STREAMING)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "PublishStream"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.salesforce.eventbus.protobuf.PublishRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.salesforce.eventbus.protobuf.PublishResponse.getDefaultInstance()))
.setSchemaDescriptor(new PubSubMethodDescriptorSupplier("PublishStream"))
.build();
}
}
}
return getPublishStreamMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static PubSubStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public PubSubStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new PubSubStub(channel, callOptions);
}
};
return PubSubStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static PubSubBlockingStub newBlockingStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public PubSubBlockingStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new PubSubBlockingStub(channel, callOptions);
}
};
return PubSubBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static PubSubFutureStub newFutureStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public PubSubFutureStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new PubSubFutureStub(channel, callOptions);
}
};
return PubSubFutureStub.newStub(factory, channel);
}
/**
*
* The Pub/Sub API provides a single interface for publishing and subscribing to platform events, including real-time
* event monitoring events, and change data capture events. The Pub/Sub API is a gRPC API that is based on HTTP/2.
* A session token is needed to authenticate. Any of the Salesforce supported
* OAuth flows can be used to obtain a session token:
* https://help.salesforce.com/articleView?id=sf.remoteaccess_oauth_flows.htm&type=5
* For each RPC, a client needs to pass authentication information
* as metadata headers (https://www.grpc.io/docs/guides/concepts/#metadata) with their method call.
* For Salesforce session token authentication, use:
* accesstoken : access token
* instanceurl : Salesforce instance URL
* tenantid : tenant/org id of the client
* StatusException is thrown in case of response failure for any request.
*
*/
public interface AsyncService {
/**
*
* Bidirectional streaming RPC to subscribe to a Topic. The subscription is pull-based. A client can request
* for more events as it consumes events. This enables a client to handle flow control based on the client's processing speed.
* Typical flow:
* 1. Client requests for X number of events via FetchRequest.
* 2. Server receives request and delivers events until X events are delivered to the client via one or more FetchResponse messages.
* 3. Client consumes the FetchResponse messages as they come.
* 4. Client issues new FetchRequest for Y more number of events. This request can
* come before the server has delivered the earlier requested X number of events
* so the client gets a continuous stream of events if any.
* If a client requests more events before the server finishes the last
* requested amount, the server appends the new amount to the current amount of
* events it still needs to fetch and deliver.
* A client can subscribe at any point in the stream by providing a replay option in the first FetchRequest.
* The replay option is honored for the first FetchRequest received from a client. Any subsequent FetchRequests with a
* new replay option are ignored. A client needs to call the Subscribe RPC again to restart the subscription
* at a new point in the stream.
* The first FetchRequest of the stream identifies the topic to subscribe to.
* If any subsequent FetchRequest provides topic_name, it must match what
* was provided in the first FetchRequest; otherwise, the RPC returns an error
* with INVALID_ARGUMENT status.
*
*/
default io.grpc.stub.StreamObserver subscribe(
io.grpc.stub.StreamObserver responseObserver) {
return io.grpc.stub.ServerCalls.asyncUnimplementedStreamingCall(getSubscribeMethod(), responseObserver);
}
/**
*
* Get the event schema for a topic based on a schema ID.
*
*/
default void getSchema(com.salesforce.eventbus.protobuf.SchemaRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetSchemaMethod(), responseObserver);
}
/**
*
* Get the topic Information related to the specified topic.
*
*/
default void getTopic(com.salesforce.eventbus.protobuf.TopicRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetTopicMethod(), responseObserver);
}
/**
*
* Send a publish request to synchronously publish events to a topic.
*
*/
default void publish(com.salesforce.eventbus.protobuf.PublishRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getPublishMethod(), responseObserver);
}
/**
*
* Bidirectional Streaming RPC to publish events to the event bus.
* PublishRequest contains the batch of events to publish.
* The first PublishRequest of the stream identifies the topic to publish on.
* If any subsequent PublishRequest provides topic_name, it must match what
* was provided in the first PublishRequest; otherwise, the RPC returns an error
* with INVALID_ARGUMENT status.
* The server returns a PublishResponse for each PublishRequest when publish is
* complete for the batch. A client does not have to wait for a PublishResponse
* before sending a new PublishRequest, i.e. multiple publish batches can be queued
* up, which allows for higher publish rate as a client can asynchronously
* publish more events while publishes are still in flight on the server side.
* PublishResponse holds a PublishResult for each event published that indicates success
* or failure of the publish. A client can then retry the publish as needed before sending
* more PublishRequests for new events to publish.
* A client must send a valid publish request with one or more events every 70 seconds to hold on to the stream.
* Otherwise, the server closes the stream and notifies the client. Once the client is notified of the stream closure,
* it must make a new PublishStream call to resume publishing.
*
*/
default io.grpc.stub.StreamObserver publishStream(
io.grpc.stub.StreamObserver responseObserver) {
return io.grpc.stub.ServerCalls.asyncUnimplementedStreamingCall(getPublishStreamMethod(), responseObserver);
}
}
/**
* Base class for the server implementation of the service PubSub.
*
* The Pub/Sub API provides a single interface for publishing and subscribing to platform events, including real-time
* event monitoring events, and change data capture events. The Pub/Sub API is a gRPC API that is based on HTTP/2.
* A session token is needed to authenticate. Any of the Salesforce supported
* OAuth flows can be used to obtain a session token:
* https://help.salesforce.com/articleView?id=sf.remoteaccess_oauth_flows.htm&type=5
* For each RPC, a client needs to pass authentication information
* as metadata headers (https://www.grpc.io/docs/guides/concepts/#metadata) with their method call.
* For Salesforce session token authentication, use:
* accesstoken : access token
* instanceurl : Salesforce instance URL
* tenantid : tenant/org id of the client
* StatusException is thrown in case of response failure for any request.
*
*/
public static abstract class PubSubImplBase
implements io.grpc.BindableService, AsyncService {
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return PubSubGrpc.bindService(this);
}
}
/**
* A stub to allow clients to do asynchronous rpc calls to service PubSub.
*
* The Pub/Sub API provides a single interface for publishing and subscribing to platform events, including real-time
* event monitoring events, and change data capture events. The Pub/Sub API is a gRPC API that is based on HTTP/2.
* A session token is needed to authenticate. Any of the Salesforce supported
* OAuth flows can be used to obtain a session token:
* https://help.salesforce.com/articleView?id=sf.remoteaccess_oauth_flows.htm&type=5
* For each RPC, a client needs to pass authentication information
* as metadata headers (https://www.grpc.io/docs/guides/concepts/#metadata) with their method call.
* For Salesforce session token authentication, use:
* accesstoken : access token
* instanceurl : Salesforce instance URL
* tenantid : tenant/org id of the client
* StatusException is thrown in case of response failure for any request.
*
*/
public static final class PubSubStub
extends io.grpc.stub.AbstractAsyncStub {
private PubSubStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected PubSubStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new PubSubStub(channel, callOptions);
}
/**
*
* Bidirectional streaming RPC to subscribe to a Topic. The subscription is pull-based. A client can request
* for more events as it consumes events. This enables a client to handle flow control based on the client's processing speed.
* Typical flow:
* 1. Client requests for X number of events via FetchRequest.
* 2. Server receives request and delivers events until X events are delivered to the client via one or more FetchResponse messages.
* 3. Client consumes the FetchResponse messages as they come.
* 4. Client issues new FetchRequest for Y more number of events. This request can
* come before the server has delivered the earlier requested X number of events
* so the client gets a continuous stream of events if any.
* If a client requests more events before the server finishes the last
* requested amount, the server appends the new amount to the current amount of
* events it still needs to fetch and deliver.
* A client can subscribe at any point in the stream by providing a replay option in the first FetchRequest.
* The replay option is honored for the first FetchRequest received from a client. Any subsequent FetchRequests with a
* new replay option are ignored. A client needs to call the Subscribe RPC again to restart the subscription
* at a new point in the stream.
* The first FetchRequest of the stream identifies the topic to subscribe to.
* If any subsequent FetchRequest provides topic_name, it must match what
* was provided in the first FetchRequest; otherwise, the RPC returns an error
* with INVALID_ARGUMENT status.
*
*/
public io.grpc.stub.StreamObserver subscribe(
io.grpc.stub.StreamObserver responseObserver) {
return io.grpc.stub.ClientCalls.asyncBidiStreamingCall(
getChannel().newCall(getSubscribeMethod(), getCallOptions()), responseObserver);
}
/**
*
* Get the event schema for a topic based on a schema ID.
*
*/
public void getSchema(com.salesforce.eventbus.protobuf.SchemaRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetSchemaMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Get the topic Information related to the specified topic.
*
*/
public void getTopic(com.salesforce.eventbus.protobuf.TopicRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetTopicMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Send a publish request to synchronously publish events to a topic.
*
*/
public void publish(com.salesforce.eventbus.protobuf.PublishRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getPublishMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Bidirectional Streaming RPC to publish events to the event bus.
* PublishRequest contains the batch of events to publish.
* The first PublishRequest of the stream identifies the topic to publish on.
* If any subsequent PublishRequest provides topic_name, it must match what
* was provided in the first PublishRequest; otherwise, the RPC returns an error
* with INVALID_ARGUMENT status.
* The server returns a PublishResponse for each PublishRequest when publish is
* complete for the batch. A client does not have to wait for a PublishResponse
* before sending a new PublishRequest, i.e. multiple publish batches can be queued
* up, which allows for higher publish rate as a client can asynchronously
* publish more events while publishes are still in flight on the server side.
* PublishResponse holds a PublishResult for each event published that indicates success
* or failure of the publish. A client can then retry the publish as needed before sending
* more PublishRequests for new events to publish.
* A client must send a valid publish request with one or more events every 70 seconds to hold on to the stream.
* Otherwise, the server closes the stream and notifies the client. Once the client is notified of the stream closure,
* it must make a new PublishStream call to resume publishing.
*
*/
public io.grpc.stub.StreamObserver publishStream(
io.grpc.stub.StreamObserver responseObserver) {
return io.grpc.stub.ClientCalls.asyncBidiStreamingCall(
getChannel().newCall(getPublishStreamMethod(), getCallOptions()), responseObserver);
}
}
/**
* A stub to allow clients to do synchronous rpc calls to service PubSub.
*
* The Pub/Sub API provides a single interface for publishing and subscribing to platform events, including real-time
* event monitoring events, and change data capture events. The Pub/Sub API is a gRPC API that is based on HTTP/2.
* A session token is needed to authenticate. Any of the Salesforce supported
* OAuth flows can be used to obtain a session token:
* https://help.salesforce.com/articleView?id=sf.remoteaccess_oauth_flows.htm&type=5
* For each RPC, a client needs to pass authentication information
* as metadata headers (https://www.grpc.io/docs/guides/concepts/#metadata) with their method call.
* For Salesforce session token authentication, use:
* accesstoken : access token
* instanceurl : Salesforce instance URL
* tenantid : tenant/org id of the client
* StatusException is thrown in case of response failure for any request.
*
*/
public static final class PubSubBlockingStub
extends io.grpc.stub.AbstractBlockingStub {
private PubSubBlockingStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected PubSubBlockingStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new PubSubBlockingStub(channel, callOptions);
}
/**
*
* Get the event schema for a topic based on a schema ID.
*
*/
public com.salesforce.eventbus.protobuf.SchemaInfo getSchema(com.salesforce.eventbus.protobuf.SchemaRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetSchemaMethod(), getCallOptions(), request);
}
/**
*
* Get the topic Information related to the specified topic.
*
*/
public com.salesforce.eventbus.protobuf.TopicInfo getTopic(com.salesforce.eventbus.protobuf.TopicRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetTopicMethod(), getCallOptions(), request);
}
/**
*
* Send a publish request to synchronously publish events to a topic.
*
*/
public com.salesforce.eventbus.protobuf.PublishResponse publish(com.salesforce.eventbus.protobuf.PublishRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getPublishMethod(), getCallOptions(), request);
}
}
/**
* A stub to allow clients to do ListenableFuture-style rpc calls to service PubSub.
*
* The Pub/Sub API provides a single interface for publishing and subscribing to platform events, including real-time
* event monitoring events, and change data capture events. The Pub/Sub API is a gRPC API that is based on HTTP/2.
* A session token is needed to authenticate. Any of the Salesforce supported
* OAuth flows can be used to obtain a session token:
* https://help.salesforce.com/articleView?id=sf.remoteaccess_oauth_flows.htm&type=5
* For each RPC, a client needs to pass authentication information
* as metadata headers (https://www.grpc.io/docs/guides/concepts/#metadata) with their method call.
* For Salesforce session token authentication, use:
* accesstoken : access token
* instanceurl : Salesforce instance URL
* tenantid : tenant/org id of the client
* StatusException is thrown in case of response failure for any request.
*
*/
public static final class PubSubFutureStub
extends io.grpc.stub.AbstractFutureStub {
private PubSubFutureStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected PubSubFutureStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new PubSubFutureStub(channel, callOptions);
}
/**
*
* Get the event schema for a topic based on a schema ID.
*
*/
public com.google.common.util.concurrent.ListenableFuture getSchema(
com.salesforce.eventbus.protobuf.SchemaRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGetSchemaMethod(), getCallOptions()), request);
}
/**
*
* Get the topic Information related to the specified topic.
*
*/
public com.google.common.util.concurrent.ListenableFuture getTopic(
com.salesforce.eventbus.protobuf.TopicRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGetTopicMethod(), getCallOptions()), request);
}
/**
*
* Send a publish request to synchronously publish events to a topic.
*
*/
public com.google.common.util.concurrent.ListenableFuture publish(
com.salesforce.eventbus.protobuf.PublishRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getPublishMethod(), getCallOptions()), request);
}
}
private static final int METHODID_GET_SCHEMA = 0;
private static final int METHODID_GET_TOPIC = 1;
private static final int METHODID_PUBLISH = 2;
private static final int METHODID_SUBSCRIBE = 3;
private static final int METHODID_PUBLISH_STREAM = 4;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final AsyncService serviceImpl;
private final int methodId;
MethodHandlers(AsyncService serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_GET_SCHEMA:
serviceImpl.getSchema((com.salesforce.eventbus.protobuf.SchemaRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_TOPIC:
serviceImpl.getTopic((com.salesforce.eventbus.protobuf.TopicRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_PUBLISH:
serviceImpl.publish((com.salesforce.eventbus.protobuf.PublishRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_SUBSCRIBE:
return (io.grpc.stub.StreamObserver) serviceImpl.subscribe(
(io.grpc.stub.StreamObserver) responseObserver);
case METHODID_PUBLISH_STREAM:
return (io.grpc.stub.StreamObserver) serviceImpl.publishStream(
(io.grpc.stub.StreamObserver) responseObserver);
default:
throw new AssertionError();
}
}
}
public static final io.grpc.ServerServiceDefinition bindService(AsyncService service) {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getSubscribeMethod(),
io.grpc.stub.ServerCalls.asyncBidiStreamingCall(
new MethodHandlers<
com.salesforce.eventbus.protobuf.FetchRequest,
com.salesforce.eventbus.protobuf.FetchResponse>(
service, METHODID_SUBSCRIBE)))
.addMethod(
getGetSchemaMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.salesforce.eventbus.protobuf.SchemaRequest,
com.salesforce.eventbus.protobuf.SchemaInfo>(
service, METHODID_GET_SCHEMA)))
.addMethod(
getGetTopicMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.salesforce.eventbus.protobuf.TopicRequest,
com.salesforce.eventbus.protobuf.TopicInfo>(
service, METHODID_GET_TOPIC)))
.addMethod(
getPublishMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.salesforce.eventbus.protobuf.PublishRequest,
com.salesforce.eventbus.protobuf.PublishResponse>(
service, METHODID_PUBLISH)))
.addMethod(
getPublishStreamMethod(),
io.grpc.stub.ServerCalls.asyncBidiStreamingCall(
new MethodHandlers<
com.salesforce.eventbus.protobuf.PublishRequest,
com.salesforce.eventbus.protobuf.PublishResponse>(
service, METHODID_PUBLISH_STREAM)))
.build();
}
private static abstract class PubSubBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
PubSubBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return com.salesforce.eventbus.protobuf.PubSubProto.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("PubSub");
}
}
private static final class PubSubFileDescriptorSupplier
extends PubSubBaseDescriptorSupplier {
PubSubFileDescriptorSupplier() {}
}
private static final class PubSubMethodDescriptorSupplier
extends PubSubBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final java.lang.String methodName;
PubSubMethodDescriptorSupplier(java.lang.String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (PubSubGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new PubSubFileDescriptorSupplier())
.addMethod(getSubscribeMethod())
.addMethod(getGetSchemaMethod())
.addMethod(getGetTopicMethod())
.addMethod(getPublishMethod())
.addMethod(getPublishStreamMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy