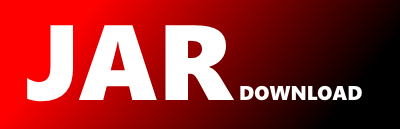
org.apache.camel.component.sip.SipEndpointConfigurer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of camel-sip Show documentation
Show all versions of camel-sip Show documentation
Camel SIP protocol based communication component
/* Generated by org.apache.camel:apt */
package org.apache.camel.component.sip;
import java.util.HashMap;
import java.util.Map;
import org.apache.camel.CamelContext;
import org.apache.camel.spi.GeneratedPropertyConfigurer;
import org.apache.camel.support.component.PropertyConfigurerSupport;
/**
* Source code generated by org.apache.camel:apt
*/
@SuppressWarnings("unchecked")
public class SipEndpointConfigurer extends PropertyConfigurerSupport implements GeneratedPropertyConfigurer {
@Override
public boolean configure(CamelContext camelContext, Object endpoint, String name, Object value, boolean ignoreCase) {
if (ignoreCase) {
return doConfigureIgnoreCase(camelContext, endpoint, name, value);
} else {
return doConfigure(camelContext, endpoint, name, value);
}
}
private static boolean doConfigure(CamelContext camelContext, Object endpoint, String name, Object value) {
switch (name) {
case "addressFactory": ((SipEndpoint) endpoint).getConfiguration().setAddressFactory(property(camelContext, javax.sip.address.AddressFactory.class, value)); return true;
case "messageFactory": ((SipEndpoint) endpoint).getConfiguration().setMessageFactory(property(camelContext, javax.sip.message.MessageFactory.class, value)); return true;
case "headerFactory": ((SipEndpoint) endpoint).getConfiguration().setHeaderFactory(property(camelContext, javax.sip.header.HeaderFactory.class, value)); return true;
case "sipStack": ((SipEndpoint) endpoint).getConfiguration().setSipStack(property(camelContext, javax.sip.SipStack.class, value)); return true;
case "listeningPoint": ((SipEndpoint) endpoint).getConfiguration().setListeningPoint(property(camelContext, javax.sip.ListeningPoint.class, value)); return true;
case "sipUri": ((SipEndpoint) endpoint).getConfiguration().setSipUri(property(camelContext, javax.sip.address.SipURI.class, value)); return true;
case "stackName": ((SipEndpoint) endpoint).getConfiguration().setStackName(property(camelContext, java.lang.String.class, value)); return true;
case "transport": ((SipEndpoint) endpoint).getConfiguration().setTransport(property(camelContext, java.lang.String.class, value)); return true;
case "maxForwards": ((SipEndpoint) endpoint).getConfiguration().setMaxForwards(property(camelContext, int.class, value)); return true;
case "consumer": ((SipEndpoint) endpoint).getConfiguration().setConsumer(property(camelContext, boolean.class, value)); return true;
case "eventHeaderName": ((SipEndpoint) endpoint).getConfiguration().setEventHeaderName(property(camelContext, java.lang.String.class, value)); return true;
case "eventId": ((SipEndpoint) endpoint).getConfiguration().setEventId(property(camelContext, java.lang.String.class, value)); return true;
case "msgExpiration": ((SipEndpoint) endpoint).getConfiguration().setMsgExpiration(property(camelContext, int.class, value)); return true;
case "useRouterForAllUris": ((SipEndpoint) endpoint).getConfiguration().setUseRouterForAllUris(property(camelContext, boolean.class, value)); return true;
case "receiveTimeoutMillis": ((SipEndpoint) endpoint).getConfiguration().setReceiveTimeoutMillis(property(camelContext, long.class, value)); return true;
case "maxMessageSize": ((SipEndpoint) endpoint).getConfiguration().setMaxMessageSize(property(camelContext, int.class, value)); return true;
case "cacheConnections": ((SipEndpoint) endpoint).getConfiguration().setCacheConnections(property(camelContext, boolean.class, value)); return true;
case "contentType": ((SipEndpoint) endpoint).getConfiguration().setContentType(property(camelContext, java.lang.String.class, value)); return true;
case "contentSubType": ((SipEndpoint) endpoint).getConfiguration().setContentSubType(property(camelContext, java.lang.String.class, value)); return true;
case "implementationServerLogFile": ((SipEndpoint) endpoint).getConfiguration().setImplementationServerLogFile(property(camelContext, java.lang.String.class, value)); return true;
case "implementationDebugLogFile": ((SipEndpoint) endpoint).getConfiguration().setImplementationDebugLogFile(property(camelContext, java.lang.String.class, value)); return true;
case "implementationTraceLevel": ((SipEndpoint) endpoint).getConfiguration().setImplementationTraceLevel(property(camelContext, java.lang.String.class, value)); return true;
case "sipFactory": ((SipEndpoint) endpoint).getConfiguration().setSipFactory(property(camelContext, javax.sip.SipFactory.class, value)); return true;
case "fromUser": ((SipEndpoint) endpoint).getConfiguration().setFromUser(property(camelContext, java.lang.String.class, value)); return true;
case "fromHost": ((SipEndpoint) endpoint).getConfiguration().setFromHost(property(camelContext, java.lang.String.class, value)); return true;
case "fromPort": ((SipEndpoint) endpoint).getConfiguration().setFromPort(property(camelContext, int.class, value)); return true;
case "toUser": ((SipEndpoint) endpoint).getConfiguration().setToUser(property(camelContext, java.lang.String.class, value)); return true;
case "toHost": ((SipEndpoint) endpoint).getConfiguration().setToHost(property(camelContext, java.lang.String.class, value)); return true;
case "toPort": ((SipEndpoint) endpoint).getConfiguration().setToPort(property(camelContext, int.class, value)); return true;
case "presenceAgent": ((SipEndpoint) endpoint).getConfiguration().setPresenceAgent(property(camelContext, boolean.class, value)); return true;
case "fromHeader": ((SipEndpoint) endpoint).getConfiguration().setFromHeader(property(camelContext, javax.sip.header.FromHeader.class, value)); return true;
case "toHeader": ((SipEndpoint) endpoint).getConfiguration().setToHeader(property(camelContext, javax.sip.header.ToHeader.class, value)); return true;
case "viaHeaders": ((SipEndpoint) endpoint).getConfiguration().setViaHeaders(property(camelContext, java.util.List.class, value)); return true;
case "contentTypeHeader": ((SipEndpoint) endpoint).getConfiguration().setContentTypeHeader(property(camelContext, javax.sip.header.ContentTypeHeader.class, value)); return true;
case "callIdHeader": ((SipEndpoint) endpoint).getConfiguration().setCallIdHeader(property(camelContext, javax.sip.header.CallIdHeader.class, value)); return true;
case "maxForwardsHeader": ((SipEndpoint) endpoint).getConfiguration().setMaxForwardsHeader(property(camelContext, javax.sip.header.MaxForwardsHeader.class, value)); return true;
case "contactHeader": ((SipEndpoint) endpoint).getConfiguration().setContactHeader(property(camelContext, javax.sip.header.ContactHeader.class, value)); return true;
case "eventHeader": ((SipEndpoint) endpoint).getConfiguration().setEventHeader(property(camelContext, javax.sip.header.EventHeader.class, value)); return true;
case "extensionHeader": ((SipEndpoint) endpoint).getConfiguration().setExtensionHeader(property(camelContext, javax.sip.header.ExtensionHeader.class, value)); return true;
case "expiresHeader": ((SipEndpoint) endpoint).getConfiguration().setExpiresHeader(property(camelContext, javax.sip.header.ExpiresHeader.class, value)); return true;
case "lazyStartProducer": ((SipEndpoint) endpoint).setLazyStartProducer(property(camelContext, boolean.class, value)); return true;
case "bridgeErrorHandler": ((SipEndpoint) endpoint).setBridgeErrorHandler(property(camelContext, boolean.class, value)); return true;
case "exceptionHandler": ((SipEndpoint) endpoint).setExceptionHandler(property(camelContext, org.apache.camel.spi.ExceptionHandler.class, value)); return true;
case "exchangePattern": ((SipEndpoint) endpoint).setExchangePattern(property(camelContext, org.apache.camel.ExchangePattern.class, value)); return true;
case "synchronous": ((SipEndpoint) endpoint).setSynchronous(property(camelContext, boolean.class, value)); return true;
case "basicPropertyBinding": ((SipEndpoint) endpoint).setBasicPropertyBinding(property(camelContext, boolean.class, value)); return true;
default: return false;
}
}
private static boolean doConfigureIgnoreCase(CamelContext camelContext, Object endpoint, String name, Object value) {
switch (name.toLowerCase()) {
case "addressfactory": ((SipEndpoint) endpoint).getConfiguration().setAddressFactory(property(camelContext, javax.sip.address.AddressFactory.class, value)); return true;
case "messagefactory": ((SipEndpoint) endpoint).getConfiguration().setMessageFactory(property(camelContext, javax.sip.message.MessageFactory.class, value)); return true;
case "headerfactory": ((SipEndpoint) endpoint).getConfiguration().setHeaderFactory(property(camelContext, javax.sip.header.HeaderFactory.class, value)); return true;
case "sipstack": ((SipEndpoint) endpoint).getConfiguration().setSipStack(property(camelContext, javax.sip.SipStack.class, value)); return true;
case "listeningpoint": ((SipEndpoint) endpoint).getConfiguration().setListeningPoint(property(camelContext, javax.sip.ListeningPoint.class, value)); return true;
case "sipuri": ((SipEndpoint) endpoint).getConfiguration().setSipUri(property(camelContext, javax.sip.address.SipURI.class, value)); return true;
case "stackname": ((SipEndpoint) endpoint).getConfiguration().setStackName(property(camelContext, java.lang.String.class, value)); return true;
case "transport": ((SipEndpoint) endpoint).getConfiguration().setTransport(property(camelContext, java.lang.String.class, value)); return true;
case "maxforwards": ((SipEndpoint) endpoint).getConfiguration().setMaxForwards(property(camelContext, int.class, value)); return true;
case "consumer": ((SipEndpoint) endpoint).getConfiguration().setConsumer(property(camelContext, boolean.class, value)); return true;
case "eventheadername": ((SipEndpoint) endpoint).getConfiguration().setEventHeaderName(property(camelContext, java.lang.String.class, value)); return true;
case "eventid": ((SipEndpoint) endpoint).getConfiguration().setEventId(property(camelContext, java.lang.String.class, value)); return true;
case "msgexpiration": ((SipEndpoint) endpoint).getConfiguration().setMsgExpiration(property(camelContext, int.class, value)); return true;
case "userouterforalluris": ((SipEndpoint) endpoint).getConfiguration().setUseRouterForAllUris(property(camelContext, boolean.class, value)); return true;
case "receivetimeoutmillis": ((SipEndpoint) endpoint).getConfiguration().setReceiveTimeoutMillis(property(camelContext, long.class, value)); return true;
case "maxmessagesize": ((SipEndpoint) endpoint).getConfiguration().setMaxMessageSize(property(camelContext, int.class, value)); return true;
case "cacheconnections": ((SipEndpoint) endpoint).getConfiguration().setCacheConnections(property(camelContext, boolean.class, value)); return true;
case "contenttype": ((SipEndpoint) endpoint).getConfiguration().setContentType(property(camelContext, java.lang.String.class, value)); return true;
case "contentsubtype": ((SipEndpoint) endpoint).getConfiguration().setContentSubType(property(camelContext, java.lang.String.class, value)); return true;
case "implementationserverlogfile": ((SipEndpoint) endpoint).getConfiguration().setImplementationServerLogFile(property(camelContext, java.lang.String.class, value)); return true;
case "implementationdebuglogfile": ((SipEndpoint) endpoint).getConfiguration().setImplementationDebugLogFile(property(camelContext, java.lang.String.class, value)); return true;
case "implementationtracelevel": ((SipEndpoint) endpoint).getConfiguration().setImplementationTraceLevel(property(camelContext, java.lang.String.class, value)); return true;
case "sipfactory": ((SipEndpoint) endpoint).getConfiguration().setSipFactory(property(camelContext, javax.sip.SipFactory.class, value)); return true;
case "fromuser": ((SipEndpoint) endpoint).getConfiguration().setFromUser(property(camelContext, java.lang.String.class, value)); return true;
case "fromhost": ((SipEndpoint) endpoint).getConfiguration().setFromHost(property(camelContext, java.lang.String.class, value)); return true;
case "fromport": ((SipEndpoint) endpoint).getConfiguration().setFromPort(property(camelContext, int.class, value)); return true;
case "touser": ((SipEndpoint) endpoint).getConfiguration().setToUser(property(camelContext, java.lang.String.class, value)); return true;
case "tohost": ((SipEndpoint) endpoint).getConfiguration().setToHost(property(camelContext, java.lang.String.class, value)); return true;
case "toport": ((SipEndpoint) endpoint).getConfiguration().setToPort(property(camelContext, int.class, value)); return true;
case "presenceagent": ((SipEndpoint) endpoint).getConfiguration().setPresenceAgent(property(camelContext, boolean.class, value)); return true;
case "fromheader": ((SipEndpoint) endpoint).getConfiguration().setFromHeader(property(camelContext, javax.sip.header.FromHeader.class, value)); return true;
case "toheader": ((SipEndpoint) endpoint).getConfiguration().setToHeader(property(camelContext, javax.sip.header.ToHeader.class, value)); return true;
case "viaheaders": ((SipEndpoint) endpoint).getConfiguration().setViaHeaders(property(camelContext, java.util.List.class, value)); return true;
case "contenttypeheader": ((SipEndpoint) endpoint).getConfiguration().setContentTypeHeader(property(camelContext, javax.sip.header.ContentTypeHeader.class, value)); return true;
case "callidheader": ((SipEndpoint) endpoint).getConfiguration().setCallIdHeader(property(camelContext, javax.sip.header.CallIdHeader.class, value)); return true;
case "maxforwardsheader": ((SipEndpoint) endpoint).getConfiguration().setMaxForwardsHeader(property(camelContext, javax.sip.header.MaxForwardsHeader.class, value)); return true;
case "contactheader": ((SipEndpoint) endpoint).getConfiguration().setContactHeader(property(camelContext, javax.sip.header.ContactHeader.class, value)); return true;
case "eventheader": ((SipEndpoint) endpoint).getConfiguration().setEventHeader(property(camelContext, javax.sip.header.EventHeader.class, value)); return true;
case "extensionheader": ((SipEndpoint) endpoint).getConfiguration().setExtensionHeader(property(camelContext, javax.sip.header.ExtensionHeader.class, value)); return true;
case "expiresheader": ((SipEndpoint) endpoint).getConfiguration().setExpiresHeader(property(camelContext, javax.sip.header.ExpiresHeader.class, value)); return true;
case "lazystartproducer": ((SipEndpoint) endpoint).setLazyStartProducer(property(camelContext, boolean.class, value)); return true;
case "bridgeerrorhandler": ((SipEndpoint) endpoint).setBridgeErrorHandler(property(camelContext, boolean.class, value)); return true;
case "exceptionhandler": ((SipEndpoint) endpoint).setExceptionHandler(property(camelContext, org.apache.camel.spi.ExceptionHandler.class, value)); return true;
case "exchangepattern": ((SipEndpoint) endpoint).setExchangePattern(property(camelContext, org.apache.camel.ExchangePattern.class, value)); return true;
case "synchronous": ((SipEndpoint) endpoint).setSynchronous(property(camelContext, boolean.class, value)); return true;
case "basicpropertybinding": ((SipEndpoint) endpoint).setBasicPropertyBinding(property(camelContext, boolean.class, value)); return true;
default: return false;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy