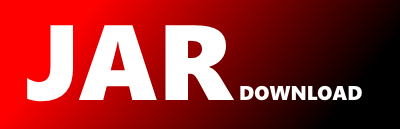
org.apache.camel.component.sip.SipEndpointConfigurer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of camel-sip Show documentation
Show all versions of camel-sip Show documentation
Camel SIP protocol based communication component
/* Generated by camel build tools - do NOT edit this file! */
package org.apache.camel.component.sip;
import java.util.Map;
import org.apache.camel.CamelContext;
import org.apache.camel.spi.GeneratedPropertyConfigurer;
import org.apache.camel.spi.PropertyConfigurerGetter;
import org.apache.camel.util.CaseInsensitiveMap;
import org.apache.camel.support.component.PropertyConfigurerSupport;
/**
* Generated by camel build tools - do NOT edit this file!
*/
@SuppressWarnings("unchecked")
public class SipEndpointConfigurer extends PropertyConfigurerSupport implements GeneratedPropertyConfigurer, PropertyConfigurerGetter {
@Override
public boolean configure(CamelContext camelContext, Object obj, String name, Object value, boolean ignoreCase) {
SipEndpoint target = (SipEndpoint) obj;
switch (ignoreCase ? name.toLowerCase() : name) {
case "addressfactory":
case "addressFactory": target.getConfiguration().setAddressFactory(property(camelContext, javax.sip.address.AddressFactory.class, value)); return true;
case "basicpropertybinding":
case "basicPropertyBinding": target.setBasicPropertyBinding(property(camelContext, boolean.class, value)); return true;
case "bridgeerrorhandler":
case "bridgeErrorHandler": target.setBridgeErrorHandler(property(camelContext, boolean.class, value)); return true;
case "cacheconnections":
case "cacheConnections": target.getConfiguration().setCacheConnections(property(camelContext, boolean.class, value)); return true;
case "callidheader":
case "callIdHeader": target.getConfiguration().setCallIdHeader(property(camelContext, javax.sip.header.CallIdHeader.class, value)); return true;
case "consumer": target.getConfiguration().setConsumer(property(camelContext, boolean.class, value)); return true;
case "contactheader":
case "contactHeader": target.getConfiguration().setContactHeader(property(camelContext, javax.sip.header.ContactHeader.class, value)); return true;
case "contentsubtype":
case "contentSubType": target.getConfiguration().setContentSubType(property(camelContext, java.lang.String.class, value)); return true;
case "contenttype":
case "contentType": target.getConfiguration().setContentType(property(camelContext, java.lang.String.class, value)); return true;
case "contenttypeheader":
case "contentTypeHeader": target.getConfiguration().setContentTypeHeader(property(camelContext, javax.sip.header.ContentTypeHeader.class, value)); return true;
case "eventheader":
case "eventHeader": target.getConfiguration().setEventHeader(property(camelContext, javax.sip.header.EventHeader.class, value)); return true;
case "eventheadername":
case "eventHeaderName": target.getConfiguration().setEventHeaderName(property(camelContext, java.lang.String.class, value)); return true;
case "eventid":
case "eventId": target.getConfiguration().setEventId(property(camelContext, java.lang.String.class, value)); return true;
case "exceptionhandler":
case "exceptionHandler": target.setExceptionHandler(property(camelContext, org.apache.camel.spi.ExceptionHandler.class, value)); return true;
case "exchangepattern":
case "exchangePattern": target.setExchangePattern(property(camelContext, org.apache.camel.ExchangePattern.class, value)); return true;
case "expiresheader":
case "expiresHeader": target.getConfiguration().setExpiresHeader(property(camelContext, javax.sip.header.ExpiresHeader.class, value)); return true;
case "extensionheader":
case "extensionHeader": target.getConfiguration().setExtensionHeader(property(camelContext, javax.sip.header.ExtensionHeader.class, value)); return true;
case "fromheader":
case "fromHeader": target.getConfiguration().setFromHeader(property(camelContext, javax.sip.header.FromHeader.class, value)); return true;
case "fromhost":
case "fromHost": target.getConfiguration().setFromHost(property(camelContext, java.lang.String.class, value)); return true;
case "fromport":
case "fromPort": target.getConfiguration().setFromPort(property(camelContext, int.class, value)); return true;
case "fromuser":
case "fromUser": target.getConfiguration().setFromUser(property(camelContext, java.lang.String.class, value)); return true;
case "headerfactory":
case "headerFactory": target.getConfiguration().setHeaderFactory(property(camelContext, javax.sip.header.HeaderFactory.class, value)); return true;
case "implementationdebuglogfile":
case "implementationDebugLogFile": target.getConfiguration().setImplementationDebugLogFile(property(camelContext, java.lang.String.class, value)); return true;
case "implementationserverlogfile":
case "implementationServerLogFile": target.getConfiguration().setImplementationServerLogFile(property(camelContext, java.lang.String.class, value)); return true;
case "implementationtracelevel":
case "implementationTraceLevel": target.getConfiguration().setImplementationTraceLevel(property(camelContext, java.lang.String.class, value)); return true;
case "lazystartproducer":
case "lazyStartProducer": target.setLazyStartProducer(property(camelContext, boolean.class, value)); return true;
case "listeningpoint":
case "listeningPoint": target.getConfiguration().setListeningPoint(property(camelContext, javax.sip.ListeningPoint.class, value)); return true;
case "maxforwards":
case "maxForwards": target.getConfiguration().setMaxForwards(property(camelContext, int.class, value)); return true;
case "maxforwardsheader":
case "maxForwardsHeader": target.getConfiguration().setMaxForwardsHeader(property(camelContext, javax.sip.header.MaxForwardsHeader.class, value)); return true;
case "maxmessagesize":
case "maxMessageSize": target.getConfiguration().setMaxMessageSize(property(camelContext, int.class, value)); return true;
case "messagefactory":
case "messageFactory": target.getConfiguration().setMessageFactory(property(camelContext, javax.sip.message.MessageFactory.class, value)); return true;
case "msgexpiration":
case "msgExpiration": target.getConfiguration().setMsgExpiration(property(camelContext, int.class, value)); return true;
case "presenceagent":
case "presenceAgent": target.getConfiguration().setPresenceAgent(property(camelContext, boolean.class, value)); return true;
case "receivetimeoutmillis":
case "receiveTimeoutMillis": target.getConfiguration().setReceiveTimeoutMillis(property(camelContext, long.class, value)); return true;
case "sipfactory":
case "sipFactory": target.getConfiguration().setSipFactory(property(camelContext, javax.sip.SipFactory.class, value)); return true;
case "sipstack":
case "sipStack": target.getConfiguration().setSipStack(property(camelContext, javax.sip.SipStack.class, value)); return true;
case "sipuri":
case "sipUri": target.getConfiguration().setSipUri(property(camelContext, javax.sip.address.SipURI.class, value)); return true;
case "stackname":
case "stackName": target.getConfiguration().setStackName(property(camelContext, java.lang.String.class, value)); return true;
case "synchronous": target.setSynchronous(property(camelContext, boolean.class, value)); return true;
case "toheader":
case "toHeader": target.getConfiguration().setToHeader(property(camelContext, javax.sip.header.ToHeader.class, value)); return true;
case "tohost":
case "toHost": target.getConfiguration().setToHost(property(camelContext, java.lang.String.class, value)); return true;
case "toport":
case "toPort": target.getConfiguration().setToPort(property(camelContext, int.class, value)); return true;
case "touser":
case "toUser": target.getConfiguration().setToUser(property(camelContext, java.lang.String.class, value)); return true;
case "transport": target.getConfiguration().setTransport(property(camelContext, java.lang.String.class, value)); return true;
case "userouterforalluris":
case "useRouterForAllUris": target.getConfiguration().setUseRouterForAllUris(property(camelContext, boolean.class, value)); return true;
case "viaheaders":
case "viaHeaders": target.getConfiguration().setViaHeaders(property(camelContext, java.util.List.class, value)); return true;
default: return false;
}
}
@Override
public Map getAllOptions(Object target) {
Map answer = new CaseInsensitiveMap();
answer.put("addressFactory", javax.sip.address.AddressFactory.class);
answer.put("basicPropertyBinding", boolean.class);
answer.put("bridgeErrorHandler", boolean.class);
answer.put("cacheConnections", boolean.class);
answer.put("callIdHeader", javax.sip.header.CallIdHeader.class);
answer.put("consumer", boolean.class);
answer.put("contactHeader", javax.sip.header.ContactHeader.class);
answer.put("contentSubType", java.lang.String.class);
answer.put("contentType", java.lang.String.class);
answer.put("contentTypeHeader", javax.sip.header.ContentTypeHeader.class);
answer.put("eventHeader", javax.sip.header.EventHeader.class);
answer.put("eventHeaderName", java.lang.String.class);
answer.put("eventId", java.lang.String.class);
answer.put("exceptionHandler", org.apache.camel.spi.ExceptionHandler.class);
answer.put("exchangePattern", org.apache.camel.ExchangePattern.class);
answer.put("expiresHeader", javax.sip.header.ExpiresHeader.class);
answer.put("extensionHeader", javax.sip.header.ExtensionHeader.class);
answer.put("fromHeader", javax.sip.header.FromHeader.class);
answer.put("fromHost", java.lang.String.class);
answer.put("fromPort", int.class);
answer.put("fromUser", java.lang.String.class);
answer.put("headerFactory", javax.sip.header.HeaderFactory.class);
answer.put("implementationDebugLogFile", java.lang.String.class);
answer.put("implementationServerLogFile", java.lang.String.class);
answer.put("implementationTraceLevel", java.lang.String.class);
answer.put("lazyStartProducer", boolean.class);
answer.put("listeningPoint", javax.sip.ListeningPoint.class);
answer.put("maxForwards", int.class);
answer.put("maxForwardsHeader", javax.sip.header.MaxForwardsHeader.class);
answer.put("maxMessageSize", int.class);
answer.put("messageFactory", javax.sip.message.MessageFactory.class);
answer.put("msgExpiration", int.class);
answer.put("presenceAgent", boolean.class);
answer.put("receiveTimeoutMillis", long.class);
answer.put("sipFactory", javax.sip.SipFactory.class);
answer.put("sipStack", javax.sip.SipStack.class);
answer.put("sipUri", javax.sip.address.SipURI.class);
answer.put("stackName", java.lang.String.class);
answer.put("synchronous", boolean.class);
answer.put("toHeader", javax.sip.header.ToHeader.class);
answer.put("toHost", java.lang.String.class);
answer.put("toPort", int.class);
answer.put("toUser", java.lang.String.class);
answer.put("transport", java.lang.String.class);
answer.put("useRouterForAllUris", boolean.class);
answer.put("viaHeaders", java.util.List.class);
return answer;
}
@Override
public Object getOptionValue(Object obj, String name, boolean ignoreCase) {
SipEndpoint target = (SipEndpoint) obj;
switch (ignoreCase ? name.toLowerCase() : name) {
case "addressfactory":
case "addressFactory": return target.getConfiguration().getAddressFactory();
case "basicpropertybinding":
case "basicPropertyBinding": return target.isBasicPropertyBinding();
case "bridgeerrorhandler":
case "bridgeErrorHandler": return target.isBridgeErrorHandler();
case "cacheconnections":
case "cacheConnections": return target.getConfiguration().isCacheConnections();
case "callidheader":
case "callIdHeader": return target.getConfiguration().getCallIdHeader();
case "consumer": return target.getConfiguration().isConsumer();
case "contactheader":
case "contactHeader": return target.getConfiguration().getContactHeader();
case "contentsubtype":
case "contentSubType": return target.getConfiguration().getContentSubType();
case "contenttype":
case "contentType": return target.getConfiguration().getContentType();
case "contenttypeheader":
case "contentTypeHeader": return target.getConfiguration().getContentTypeHeader();
case "eventheader":
case "eventHeader": return target.getConfiguration().getEventHeader();
case "eventheadername":
case "eventHeaderName": return target.getConfiguration().getEventHeaderName();
case "eventid":
case "eventId": return target.getConfiguration().getEventId();
case "exceptionhandler":
case "exceptionHandler": return target.getExceptionHandler();
case "exchangepattern":
case "exchangePattern": return target.getExchangePattern();
case "expiresheader":
case "expiresHeader": return target.getConfiguration().getExpiresHeader();
case "extensionheader":
case "extensionHeader": return target.getConfiguration().getExtensionHeader();
case "fromheader":
case "fromHeader": return target.getConfiguration().getFromHeader();
case "fromhost":
case "fromHost": return target.getConfiguration().getFromHost();
case "fromport":
case "fromPort": return target.getConfiguration().getFromPort();
case "fromuser":
case "fromUser": return target.getConfiguration().getFromUser();
case "headerfactory":
case "headerFactory": return target.getConfiguration().getHeaderFactory();
case "implementationdebuglogfile":
case "implementationDebugLogFile": return target.getConfiguration().getImplementationDebugLogFile();
case "implementationserverlogfile":
case "implementationServerLogFile": return target.getConfiguration().getImplementationServerLogFile();
case "implementationtracelevel":
case "implementationTraceLevel": return target.getConfiguration().getImplementationTraceLevel();
case "lazystartproducer":
case "lazyStartProducer": return target.isLazyStartProducer();
case "listeningpoint":
case "listeningPoint": return target.getConfiguration().getListeningPoint();
case "maxforwards":
case "maxForwards": return target.getConfiguration().getMaxForwards();
case "maxforwardsheader":
case "maxForwardsHeader": return target.getConfiguration().getMaxForwardsHeader();
case "maxmessagesize":
case "maxMessageSize": return target.getConfiguration().getMaxMessageSize();
case "messagefactory":
case "messageFactory": return target.getConfiguration().getMessageFactory();
case "msgexpiration":
case "msgExpiration": return target.getConfiguration().getMsgExpiration();
case "presenceagent":
case "presenceAgent": return target.getConfiguration().isPresenceAgent();
case "receivetimeoutmillis":
case "receiveTimeoutMillis": return target.getConfiguration().getReceiveTimeoutMillis();
case "sipfactory":
case "sipFactory": return target.getConfiguration().getSipFactory();
case "sipstack":
case "sipStack": return target.getConfiguration().getSipStack();
case "sipuri":
case "sipUri": return target.getConfiguration().getSipUri();
case "stackname":
case "stackName": return target.getConfiguration().getStackName();
case "synchronous": return target.isSynchronous();
case "toheader":
case "toHeader": return target.getConfiguration().getToHeader();
case "tohost":
case "toHost": return target.getConfiguration().getToHost();
case "toport":
case "toPort": return target.getConfiguration().getToPort();
case "touser":
case "toUser": return target.getConfiguration().getToUser();
case "transport": return target.getConfiguration().getTransport();
case "userouterforalluris":
case "useRouterForAllUris": return target.getConfiguration().isUseRouterForAllUris();
case "viaheaders":
case "viaHeaders": return target.getConfiguration().getViaHeaders();
default: return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy