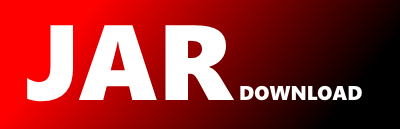
org.apache.camel.component.smooks.SmooksProcessor Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.camel.component.smooks;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.io.Reader;
import java.net.MalformedURLException;
import java.net.URL;
import java.nio.charset.Charset;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import javax.xml.transform.sax.SAXSource;
import org.w3c.dom.Node;
import org.xml.sax.SAXException;
import org.apache.camel.CamelContext;
import org.apache.camel.CamelContextAware;
import org.apache.camel.Exchange;
import org.apache.camel.InvalidPayloadException;
import org.apache.camel.Message;
import org.apache.camel.Processor;
import org.apache.camel.RuntimeCamelException;
import org.apache.camel.WrappedFile;
import org.apache.camel.support.ExchangeHelper;
import org.apache.camel.support.ResourceHelper;
import org.apache.camel.support.builder.OutputStreamBuilder;
import org.apache.camel.support.service.ServiceSupport;
import org.apache.camel.util.IOHelper;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.smooks.Smooks;
import org.smooks.SmooksFactory;
import org.smooks.api.ExecutionContext;
import org.smooks.api.NotAppContextScoped;
import org.smooks.api.SmooksException;
import org.smooks.api.TypedKey;
import org.smooks.api.delivery.VisitorAppender;
import org.smooks.api.io.Sink;
import org.smooks.api.io.Source;
import org.smooks.api.resource.visitor.Visitor;
import org.smooks.engine.lookup.ExportsLookup;
import org.smooks.engine.report.HtmlReportGenerator;
import org.smooks.io.payload.Exports;
import org.smooks.io.sink.StreamSink;
import org.smooks.io.source.ByteSource;
import org.smooks.io.source.DOMSource;
import org.smooks.io.source.ReaderSource;
import org.smooks.io.source.StreamSource;
import org.smooks.io.source.URLSource;
/**
* Smooks {@link Processor} for Camel.
*/
public class SmooksProcessor extends ServiceSupport implements Processor, CamelContextAware {
private static final TypedKey EXCHANGE_TYPED_KEY = TypedKey.of();
private static final Logger LOG = LoggerFactory.getLogger(SmooksProcessor.class);
private SmooksFactory smooksFactory;
private Smooks smooks;
private String configUri;
private String reportPath;
private Boolean allowExecutionContextFromHeader = false;
private final Set visitorAppender = new HashSet<>();
private final Map selectorVisitorMap = new HashMap<>();
private CamelContext camelContext;
public SmooksProcessor(final CamelContext camelContext) {
this.camelContext = camelContext;
}
public SmooksProcessor(final Smooks smooks, final CamelContext camelContext) {
this(camelContext);
this.smooks = smooks;
}
public SmooksProcessor(final String configUri, final CamelContext camelContext) throws IOException, SAXException {
this(camelContext);
this.configUri = configUri;
}
public void setCamelContext(CamelContext camelContext) {
this.camelContext = camelContext;
}
public CamelContext getCamelContext() {
return camelContext;
}
public Boolean getAllowExecutionContextFromHeader() {
return allowExecutionContextFromHeader;
}
public void setAllowExecutionContextFromHeader(Boolean allowExecutionContextFromHeader) {
this.allowExecutionContextFromHeader = allowExecutionContextFromHeader;
}
public void process(final Exchange exchange) {
ExecutionContext executionContext = null;
if (allowExecutionContextFromHeader) {
executionContext
= exchange.getMessage().getHeader(SmooksConstants.SMOOKS_EXECUTION_CONTEXT, ExecutionContext.class);
}
if (executionContext == null) {
executionContext = smooks.createExecutionContext();
Charset charset = ExchangeHelper.getCharset(exchange, false);
if (charset != null) {
// if provided use the same character encoding
executionContext.setContentEncoding(charset.name());
}
}
try {
executionContext.put(EXCHANGE_TYPED_KEY, exchange);
exchange.getIn().setHeader(SmooksConstants.SMOOKS_EXECUTION_CONTEXT, executionContext);
setUpSmooksReporting(executionContext);
final Exports exports = smooks.getApplicationContext().getRegistry().lookup(new ExportsLookup());
if (exports.hasExports()) {
final Sink[] sinks = exports.createSinks();
smooks.filterSource(executionContext, getSource(exchange), sinks);
setResultOnBody(exports, sinks, exchange);
} else {
final OutputStreamBuilder outputStreamBuilder = OutputStreamBuilder.withExchange(exchange);
smooks.filterSource(executionContext, getSource(exchange),
new StreamSink<>(outputStreamBuilder));
exchange.getMessage().setBody(outputStreamBuilder.build());
}
} catch (IOException | InvalidPayloadException e) {
throw new RuntimeCamelException(e);
} finally {
executionContext.remove(EXCHANGE_TYPED_KEY);
}
}
protected void setResultOnBody(final Exports exports, final Sink[] sinks, final Exchange exchange) {
final Message message = exchange.getMessage();
final List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy