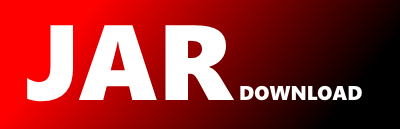
org.apache.camel.component.smpp.SmppEndpointConfigurer Maven / Gradle / Ivy
/* Generated by org.apache.camel:apt */
package org.apache.camel.component.smpp;
import java.util.HashMap;
import java.util.Map;
import org.apache.camel.CamelContext;
import org.apache.camel.spi.GeneratedPropertyConfigurer;
import org.apache.camel.support.component.PropertyConfigurerSupport;
/**
* Source code generated by org.apache.camel:apt
*/
@SuppressWarnings("unchecked")
public class SmppEndpointConfigurer extends PropertyConfigurerSupport implements GeneratedPropertyConfigurer {
@Override
public boolean configure(CamelContext camelContext, Object endpoint, String name, Object value, boolean ignoreCase) {
if (ignoreCase) {
return doConfigureIgnoreCase(camelContext, endpoint, name, value);
} else {
return doConfigure(camelContext, endpoint, name, value);
}
}
private static boolean doConfigure(CamelContext camelContext, Object endpoint, String name, Object value) {
switch (name) {
case "systemId": ((SmppEndpoint) endpoint).getConfiguration().setSystemId(property(camelContext, java.lang.String.class, value)); return true;
case "password": ((SmppEndpoint) endpoint).getConfiguration().setPassword(property(camelContext, java.lang.String.class, value)); return true;
case "systemType": ((SmppEndpoint) endpoint).getConfiguration().setSystemType(property(camelContext, java.lang.String.class, value)); return true;
case "dataCoding": ((SmppEndpoint) endpoint).getConfiguration().setDataCoding(property(camelContext, byte.class, value)); return true;
case "alphabet": ((SmppEndpoint) endpoint).getConfiguration().setAlphabet(property(camelContext, byte.class, value)); return true;
case "encoding": ((SmppEndpoint) endpoint).getConfiguration().setEncoding(property(camelContext, java.lang.String.class, value)); return true;
case "enquireLinkTimer": ((SmppEndpoint) endpoint).getConfiguration().setEnquireLinkTimer(property(camelContext, java.lang.Integer.class, value)); return true;
case "transactionTimer": ((SmppEndpoint) endpoint).getConfiguration().setTransactionTimer(property(camelContext, java.lang.Integer.class, value)); return true;
case "registeredDelivery": ((SmppEndpoint) endpoint).getConfiguration().setRegisteredDelivery(property(camelContext, byte.class, value)); return true;
case "serviceType": ((SmppEndpoint) endpoint).getConfiguration().setServiceType(property(camelContext, java.lang.String.class, value)); return true;
case "sourceAddr": ((SmppEndpoint) endpoint).getConfiguration().setSourceAddr(property(camelContext, java.lang.String.class, value)); return true;
case "destAddr": ((SmppEndpoint) endpoint).getConfiguration().setDestAddr(property(camelContext, java.lang.String.class, value)); return true;
case "sourceAddrTon": ((SmppEndpoint) endpoint).getConfiguration().setSourceAddrTon(property(camelContext, byte.class, value)); return true;
case "destAddrTon": ((SmppEndpoint) endpoint).getConfiguration().setDestAddrTon(property(camelContext, byte.class, value)); return true;
case "sourceAddrNpi": ((SmppEndpoint) endpoint).getConfiguration().setSourceAddrNpi(property(camelContext, byte.class, value)); return true;
case "destAddrNpi": ((SmppEndpoint) endpoint).getConfiguration().setDestAddrNpi(property(camelContext, byte.class, value)); return true;
case "addressRange": ((SmppEndpoint) endpoint).getConfiguration().setAddressRange(property(camelContext, java.lang.String.class, value)); return true;
case "protocolId": ((SmppEndpoint) endpoint).getConfiguration().setProtocolId(property(camelContext, byte.class, value)); return true;
case "priorityFlag": ((SmppEndpoint) endpoint).getConfiguration().setPriorityFlag(property(camelContext, byte.class, value)); return true;
case "replaceIfPresentFlag": ((SmppEndpoint) endpoint).getConfiguration().setReplaceIfPresentFlag(property(camelContext, byte.class, value)); return true;
case "typeOfNumber": ((SmppEndpoint) endpoint).getConfiguration().setTypeOfNumber(property(camelContext, byte.class, value)); return true;
case "numberingPlanIndicator": ((SmppEndpoint) endpoint).getConfiguration().setNumberingPlanIndicator(property(camelContext, byte.class, value)); return true;
case "usingSSL": ((SmppEndpoint) endpoint).getConfiguration().setUsingSSL(property(camelContext, boolean.class, value)); return true;
case "initialReconnectDelay": ((SmppEndpoint) endpoint).getConfiguration().setInitialReconnectDelay(property(camelContext, long.class, value)); return true;
case "reconnectDelay": ((SmppEndpoint) endpoint).getConfiguration().setReconnectDelay(property(camelContext, long.class, value)); return true;
case "maxReconnect": ((SmppEndpoint) endpoint).getConfiguration().setMaxReconnect(property(camelContext, int.class, value)); return true;
case "lazySessionCreation": ((SmppEndpoint) endpoint).getConfiguration().setLazySessionCreation(property(camelContext, boolean.class, value)); return true;
case "httpProxyHost": ((SmppEndpoint) endpoint).getConfiguration().setHttpProxyHost(property(camelContext, java.lang.String.class, value)); return true;
case "httpProxyPort": ((SmppEndpoint) endpoint).getConfiguration().setHttpProxyPort(property(camelContext, java.lang.Integer.class, value)); return true;
case "httpProxyUsername": ((SmppEndpoint) endpoint).getConfiguration().setHttpProxyUsername(property(camelContext, java.lang.String.class, value)); return true;
case "httpProxyPassword": ((SmppEndpoint) endpoint).getConfiguration().setHttpProxyPassword(property(camelContext, java.lang.String.class, value)); return true;
case "proxyHeaders": ((SmppEndpoint) endpoint).getConfiguration().setProxyHeaders(property(camelContext, java.util.Map.class, value)); return true;
case "sessionStateListener": ((SmppEndpoint) endpoint).getConfiguration().setSessionStateListener(property(camelContext, org.jsmpp.session.SessionStateListener.class, value)); return true;
case "splittingPolicy": ((SmppEndpoint) endpoint).getConfiguration().setSplittingPolicy(property(camelContext, org.apache.camel.component.smpp.SmppSplittingPolicy.class, value)); return true;
case "lazyStartProducer": ((SmppEndpoint) endpoint).setLazyStartProducer(property(camelContext, boolean.class, value)); return true;
case "bridgeErrorHandler": ((SmppEndpoint) endpoint).setBridgeErrorHandler(property(camelContext, boolean.class, value)); return true;
case "exceptionHandler": ((SmppEndpoint) endpoint).setExceptionHandler(property(camelContext, org.apache.camel.spi.ExceptionHandler.class, value)); return true;
case "exchangePattern": ((SmppEndpoint) endpoint).setExchangePattern(property(camelContext, org.apache.camel.ExchangePattern.class, value)); return true;
case "synchronous": ((SmppEndpoint) endpoint).setSynchronous(property(camelContext, boolean.class, value)); return true;
case "basicPropertyBinding": ((SmppEndpoint) endpoint).setBasicPropertyBinding(property(camelContext, boolean.class, value)); return true;
default: return false;
}
}
private static boolean doConfigureIgnoreCase(CamelContext camelContext, Object endpoint, String name, Object value) {
switch (name.toLowerCase()) {
case "systemid": ((SmppEndpoint) endpoint).getConfiguration().setSystemId(property(camelContext, java.lang.String.class, value)); return true;
case "password": ((SmppEndpoint) endpoint).getConfiguration().setPassword(property(camelContext, java.lang.String.class, value)); return true;
case "systemtype": ((SmppEndpoint) endpoint).getConfiguration().setSystemType(property(camelContext, java.lang.String.class, value)); return true;
case "datacoding": ((SmppEndpoint) endpoint).getConfiguration().setDataCoding(property(camelContext, byte.class, value)); return true;
case "alphabet": ((SmppEndpoint) endpoint).getConfiguration().setAlphabet(property(camelContext, byte.class, value)); return true;
case "encoding": ((SmppEndpoint) endpoint).getConfiguration().setEncoding(property(camelContext, java.lang.String.class, value)); return true;
case "enquirelinktimer": ((SmppEndpoint) endpoint).getConfiguration().setEnquireLinkTimer(property(camelContext, java.lang.Integer.class, value)); return true;
case "transactiontimer": ((SmppEndpoint) endpoint).getConfiguration().setTransactionTimer(property(camelContext, java.lang.Integer.class, value)); return true;
case "registereddelivery": ((SmppEndpoint) endpoint).getConfiguration().setRegisteredDelivery(property(camelContext, byte.class, value)); return true;
case "servicetype": ((SmppEndpoint) endpoint).getConfiguration().setServiceType(property(camelContext, java.lang.String.class, value)); return true;
case "sourceaddr": ((SmppEndpoint) endpoint).getConfiguration().setSourceAddr(property(camelContext, java.lang.String.class, value)); return true;
case "destaddr": ((SmppEndpoint) endpoint).getConfiguration().setDestAddr(property(camelContext, java.lang.String.class, value)); return true;
case "sourceaddrton": ((SmppEndpoint) endpoint).getConfiguration().setSourceAddrTon(property(camelContext, byte.class, value)); return true;
case "destaddrton": ((SmppEndpoint) endpoint).getConfiguration().setDestAddrTon(property(camelContext, byte.class, value)); return true;
case "sourceaddrnpi": ((SmppEndpoint) endpoint).getConfiguration().setSourceAddrNpi(property(camelContext, byte.class, value)); return true;
case "destaddrnpi": ((SmppEndpoint) endpoint).getConfiguration().setDestAddrNpi(property(camelContext, byte.class, value)); return true;
case "addressrange": ((SmppEndpoint) endpoint).getConfiguration().setAddressRange(property(camelContext, java.lang.String.class, value)); return true;
case "protocolid": ((SmppEndpoint) endpoint).getConfiguration().setProtocolId(property(camelContext, byte.class, value)); return true;
case "priorityflag": ((SmppEndpoint) endpoint).getConfiguration().setPriorityFlag(property(camelContext, byte.class, value)); return true;
case "replaceifpresentflag": ((SmppEndpoint) endpoint).getConfiguration().setReplaceIfPresentFlag(property(camelContext, byte.class, value)); return true;
case "typeofnumber": ((SmppEndpoint) endpoint).getConfiguration().setTypeOfNumber(property(camelContext, byte.class, value)); return true;
case "numberingplanindicator": ((SmppEndpoint) endpoint).getConfiguration().setNumberingPlanIndicator(property(camelContext, byte.class, value)); return true;
case "usingssl": ((SmppEndpoint) endpoint).getConfiguration().setUsingSSL(property(camelContext, boolean.class, value)); return true;
case "initialreconnectdelay": ((SmppEndpoint) endpoint).getConfiguration().setInitialReconnectDelay(property(camelContext, long.class, value)); return true;
case "reconnectdelay": ((SmppEndpoint) endpoint).getConfiguration().setReconnectDelay(property(camelContext, long.class, value)); return true;
case "maxreconnect": ((SmppEndpoint) endpoint).getConfiguration().setMaxReconnect(property(camelContext, int.class, value)); return true;
case "lazysessioncreation": ((SmppEndpoint) endpoint).getConfiguration().setLazySessionCreation(property(camelContext, boolean.class, value)); return true;
case "httpproxyhost": ((SmppEndpoint) endpoint).getConfiguration().setHttpProxyHost(property(camelContext, java.lang.String.class, value)); return true;
case "httpproxyport": ((SmppEndpoint) endpoint).getConfiguration().setHttpProxyPort(property(camelContext, java.lang.Integer.class, value)); return true;
case "httpproxyusername": ((SmppEndpoint) endpoint).getConfiguration().setHttpProxyUsername(property(camelContext, java.lang.String.class, value)); return true;
case "httpproxypassword": ((SmppEndpoint) endpoint).getConfiguration().setHttpProxyPassword(property(camelContext, java.lang.String.class, value)); return true;
case "proxyheaders": ((SmppEndpoint) endpoint).getConfiguration().setProxyHeaders(property(camelContext, java.util.Map.class, value)); return true;
case "sessionstatelistener": ((SmppEndpoint) endpoint).getConfiguration().setSessionStateListener(property(camelContext, org.jsmpp.session.SessionStateListener.class, value)); return true;
case "splittingpolicy": ((SmppEndpoint) endpoint).getConfiguration().setSplittingPolicy(property(camelContext, org.apache.camel.component.smpp.SmppSplittingPolicy.class, value)); return true;
case "lazystartproducer": ((SmppEndpoint) endpoint).setLazyStartProducer(property(camelContext, boolean.class, value)); return true;
case "bridgeerrorhandler": ((SmppEndpoint) endpoint).setBridgeErrorHandler(property(camelContext, boolean.class, value)); return true;
case "exceptionhandler": ((SmppEndpoint) endpoint).setExceptionHandler(property(camelContext, org.apache.camel.spi.ExceptionHandler.class, value)); return true;
case "exchangepattern": ((SmppEndpoint) endpoint).setExchangePattern(property(camelContext, org.apache.camel.ExchangePattern.class, value)); return true;
case "synchronous": ((SmppEndpoint) endpoint).setSynchronous(property(camelContext, boolean.class, value)); return true;
case "basicpropertybinding": ((SmppEndpoint) endpoint).setBasicPropertyBinding(property(camelContext, boolean.class, value)); return true;
default: return false;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy