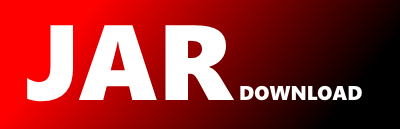
org.apache.camel.support.DefaultMessage Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.camel.support;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
import java.util.function.Supplier;
import org.apache.camel.CamelContext;
import org.apache.camel.Exchange;
import org.apache.camel.spi.HeadersMapFactory;
/**
* The default implementation of {@link org.apache.camel.Message}
*
* This implementation uses a {@link org.apache.camel.util.CaseInsensitiveMap} storing the headers. This allows us to be
* able to lookup headers using case insensitive keys, making it easier for end users as they do not have to be worried
* about using exact keys. See more details at {@link org.apache.camel.util.CaseInsensitiveMap}. The implementation of
* the map can be configured by the {@link HeadersMapFactory} which can be set on the {@link CamelContext}. The default
* implementation uses the {@link org.apache.camel.util.CaseInsensitiveMap CaseInsensitiveMap}.
*/
public class DefaultMessage extends MessageSupport {
private Map headers;
public DefaultMessage(Exchange exchange) {
setExchange(exchange);
if (exchange != null) {
setCamelContext(exchange.getContext());
}
}
public DefaultMessage(CamelContext camelContext) {
this.camelContext = camelContext;
this.typeConverter = camelContext.getTypeConverter();
}
@Override
public void reset() {
super.reset();
if (headers != null) {
headers.clear();
}
}
@Override
public Object getHeader(String name) {
if (headers == null) {
// force creating headers
headers = createHeaders();
}
if (!headers.isEmpty()) {
return headers.get(name);
} else {
return null;
}
}
@Override
public Object getHeader(String name, Object defaultValue) {
Object answer = null;
if (headers == null) {
// force creating headers
headers = createHeaders();
}
if (!headers.isEmpty()) {
answer = headers.get(name);
}
return answer != null ? answer : defaultValue;
}
@Override
public Object getHeader(String name, Supplier
© 2015 - 2025 Weber Informatics LLC | Privacy Policy