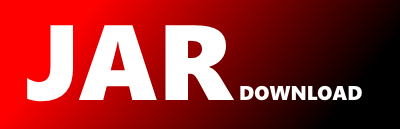
org.apache.camel.xml.LwModelToXMLDumper Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.camel.xml;
import java.io.IOException;
import java.io.StringWriter;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import java.util.function.Consumer;
import org.apache.camel.CamelContext;
import org.apache.camel.CamelContextAware;
import org.apache.camel.Expression;
import org.apache.camel.NamedNode;
import org.apache.camel.model.ExpressionNode;
import org.apache.camel.model.FromDefinition;
import org.apache.camel.model.OptionalIdentifiedDefinition;
import org.apache.camel.model.RouteDefinition;
import org.apache.camel.model.RouteTemplateDefinition;
import org.apache.camel.model.RouteTemplatesDefinition;
import org.apache.camel.model.RoutesDefinition;
import org.apache.camel.model.SendDefinition;
import org.apache.camel.model.ToDynamicDefinition;
import org.apache.camel.model.app.RegistryBeanDefinition;
import org.apache.camel.model.language.ExpressionDefinition;
import org.apache.camel.spi.ModelToXMLDumper;
import org.apache.camel.spi.NamespaceAware;
import org.apache.camel.spi.annotations.JdkService;
import org.apache.camel.util.KeyValueHolder;
import org.apache.camel.xml.out.ModelWriter;
import static org.apache.camel.model.ProcessorDefinitionHelper.filterTypeInOutputs;
/**
* Lightweight {@link ModelToXMLDumper} based on the generated {@link ModelWriter}.
*/
@JdkService(ModelToXMLDumper.FACTORY)
public class LwModelToXMLDumper implements ModelToXMLDumper {
@Override
public String dumpModelAsXml(CamelContext context, NamedNode definition) throws Exception {
return dumpModelAsXml(context, definition, false, true);
}
@Override
public String dumpModelAsXml(
CamelContext context, NamedNode definition, boolean resolvePlaceholders, boolean generatedIds)
throws Exception {
Properties properties = new Properties();
Map namespaces = new LinkedHashMap<>();
Map> locations = new HashMap<>();
Consumer extractor = route -> {
extractNamespaces(route, namespaces);
if (context.isDebugging()) {
extractSourceLocations(route, locations);
}
resolveEndpointDslUris(route);
if (Boolean.TRUE.equals(route.isTemplate())) {
Map parameters = route.getTemplateParameters();
if (parameters != null) {
properties.putAll(parameters);
}
}
};
StringWriter buffer = new StringWriter();
ModelWriter writer = new ModelWriter(buffer, "http://camel.apache.org/schema/spring") {
@Override
protected void doWriteOptionalIdentifiedDefinitionAttributes(OptionalIdentifiedDefinition> def)
throws IOException {
if (generatedIds || Boolean.TRUE.equals(def.getCustomId())) {
// write id
doWriteAttribute("id", def.getId());
}
// write description
if (def.getDescriptionText() != null) {
doWriteAttribute("description", def.getDescriptionText());
}
// write location information
if (context.isDebugging()) {
String loc = (def instanceof RouteDefinition ? ((RouteDefinition) def).getInput() : def).getLocation();
int line = (def instanceof RouteDefinition ? ((RouteDefinition) def).getInput() : def).getLineNumber();
if (line != -1) {
writer.addAttribute("sourceLineNumber", Integer.toString(line));
writer.addAttribute("sourceLocation", loc);
}
}
}
@Override
protected void startElement(String name) throws IOException {
boolean namespaceWritten = this.namespaceWritten;
super.startElement(name);
if (!namespaceWritten) {
for (Map.Entry entry : namespaces.entrySet()) {
// TODO: check duplicate xmlns="xxx" attribute ?
String nsPrefix = entry.getKey();
String prefix = nsPrefix.equals("xmlns") ? nsPrefix : "xmlns:" + nsPrefix;
writer.addAttribute(prefix, entry.getValue());
}
}
}
@Override
protected void doWriteValue(String value) throws IOException {
if (value != null && !value.isEmpty()) {
if (resolvePlaceholders) {
value = resolve(value, properties);
}
super.doWriteValue(value);
}
}
@Override
protected void text(String name, String text) throws IOException {
if (resolvePlaceholders) {
text = resolve(text, properties);
}
super.text(name, text);
}
@Override
protected void attribute(String name, Object value) throws IOException {
if (resolvePlaceholders && value != null) {
value = resolve(value.toString(), properties);
}
super.attribute(name, value);
}
String resolve(String value, Properties properties) {
context.getPropertiesComponent().setLocalProperties(properties);
try {
return context.resolvePropertyPlaceholders(value);
} catch (Exception e) {
return value;
} finally {
// clear local after the route is dumped
context.getPropertiesComponent().setLocalProperties(null);
}
}
};
// gather all namespaces from the routes or route which is stored on the expression nodes
if (definition instanceof RouteTemplatesDefinition templates) {
templates.getRouteTemplates().forEach(template -> extractor.accept(template.getRoute()));
} else if (definition instanceof RouteTemplateDefinition template) {
extractor.accept(template.getRoute());
} else if (definition instanceof RoutesDefinition routes) {
routes.getRoutes().forEach(extractor);
} else if (definition instanceof RouteDefinition route) {
extractor.accept(route);
}
writer.writeOptionalIdentifiedDefinitionRef((OptionalIdentifiedDefinition) definition);
return buffer.toString();
}
@Override
public String dumpBeansAsXml(CamelContext context, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy