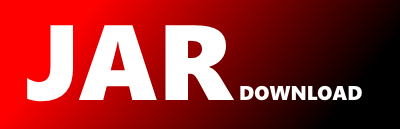
org.apache.camel.xml.out.ModelWriter Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
/**
* Generated by Camel build tools - do NOT edit this file!
*/
package org.apache.camel.xml.out;
import java.io.IOException;
import java.io.Writer;
import java.util.ArrayList;
import java.util.Base64;
import java.util.List;
import org.apache.camel.Expression;
import org.apache.camel.model.*;
import org.apache.camel.model.app.*;
import org.apache.camel.model.cloud.*;
import org.apache.camel.model.config.BatchResequencerConfig;
import org.apache.camel.model.config.ResequencerConfig;
import org.apache.camel.model.config.StreamResequencerConfig;
import org.apache.camel.model.dataformat.*;
import org.apache.camel.model.errorhandler.*;
import org.apache.camel.model.language.*;
import org.apache.camel.model.loadbalancer.*;
import org.apache.camel.model.rest.*;
import org.apache.camel.model.transformer.*;
import org.apache.camel.model.validator.*;
@SuppressWarnings("all")
public class ModelWriter extends BaseWriter {
public ModelWriter(Writer writer, String namespace) throws IOException {
super(writer, namespace);
}
public ModelWriter(Writer writer) throws IOException {
super(writer, null);
}
public void writeAggregateDefinition(
AggregateDefinition def)
throws IOException {
doWriteAggregateDefinition("aggregate", def);
}
public void writeBeanDefinition(BeanDefinition def) throws IOException {
doWriteBeanDefinition("bean", def);
}
public void writeCatchDefinition(CatchDefinition def) throws IOException {
doWriteCatchDefinition("doCatch", def);
}
public void writeChoiceDefinition(ChoiceDefinition def) throws IOException {
doWriteChoiceDefinition("choice", def);
}
public void writeCircuitBreakerDefinition(
CircuitBreakerDefinition def)
throws IOException {
doWriteCircuitBreakerDefinition("circuitBreaker", def);
}
public void writeClaimCheckDefinition(
ClaimCheckDefinition def)
throws IOException {
doWriteClaimCheckDefinition("claimCheck", def);
}
public void writeContextScanDefinition(
ContextScanDefinition def)
throws IOException {
doWriteContextScanDefinition("contextScan", def);
}
public void writeConvertBodyDefinition(
ConvertBodyDefinition def)
throws IOException {
doWriteConvertBodyDefinition("convertBodyTo", def);
}
public void writeConvertHeaderDefinition(
ConvertHeaderDefinition def)
throws IOException {
doWriteConvertHeaderDefinition("convertHeaderTo", def);
}
public void writeConvertVariableDefinition(
ConvertVariableDefinition def)
throws IOException {
doWriteConvertVariableDefinition("convertVariableTo", def);
}
public void writeDelayDefinition(DelayDefinition def) throws IOException {
doWriteDelayDefinition("delay", def);
}
public void writeDynamicRouterDefinition(
DynamicRouterDefinition def)
throws IOException {
doWriteDynamicRouterDefinition("dynamicRouter", def);
}
public void writeEnrichDefinition(EnrichDefinition def) throws IOException {
doWriteEnrichDefinition("enrich", def);
}
public void writeErrorHandlerDefinition(
ErrorHandlerDefinition def)
throws IOException {
doWriteErrorHandlerDefinition("errorHandler", def);
}
public void writeExpressionSubElementDefinition(
ExpressionSubElementDefinition def)
throws IOException {
doWriteExpressionSubElementDefinition("expression", def);
}
public void writeFaultToleranceConfigurationDefinition(
FaultToleranceConfigurationDefinition def)
throws IOException {
doWriteFaultToleranceConfigurationDefinition("faultToleranceConfiguration", def);
}
public void writeFilterDefinition(FilterDefinition def) throws IOException {
doWriteFilterDefinition("filter", def);
}
public void writeFinallyDefinition(FinallyDefinition def) throws IOException {
doWriteFinallyDefinition("doFinally", def);
}
public void writeFromDefinition(FromDefinition def) throws IOException {
doWriteFromDefinition("from", def);
}
public void writeGlobalOptionDefinition(
GlobalOptionDefinition def)
throws IOException {
doWriteGlobalOptionDefinition("globalOption", def);
}
public void writeGlobalOptionsDefinition(
GlobalOptionsDefinition def)
throws IOException {
doWriteGlobalOptionsDefinition("globalOptions", def);
}
public void writeIdempotentConsumerDefinition(
IdempotentConsumerDefinition def)
throws IOException {
doWriteIdempotentConsumerDefinition("idempotentConsumer", def);
}
public void writeInputTypeDefinition(
InputTypeDefinition def)
throws IOException {
doWriteInputTypeDefinition("inputType", def);
}
public void writeInterceptDefinition(
InterceptDefinition def)
throws IOException {
doWriteInterceptDefinition("intercept", def);
}
public void writeInterceptFromDefinition(
InterceptFromDefinition def)
throws IOException {
doWriteInterceptFromDefinition("interceptFrom", def);
}
public void writeInterceptSendToEndpointDefinition(
InterceptSendToEndpointDefinition def)
throws IOException {
doWriteInterceptSendToEndpointDefinition("interceptSendToEndpoint", def);
}
public void writeKameletDefinition(KameletDefinition def) throws IOException {
doWriteKameletDefinition("kamelet", def);
}
public void writeLoadBalanceDefinition(
LoadBalanceDefinition def)
throws IOException {
doWriteLoadBalanceDefinition("loadBalance", def);
}
public void writeLogDefinition(LogDefinition def) throws IOException {
doWriteLogDefinition("log", def);
}
public void writeLoopDefinition(LoopDefinition def) throws IOException {
doWriteLoopDefinition("loop", def);
}
public void writeMarshalDefinition(MarshalDefinition def) throws IOException {
doWriteMarshalDefinition("marshal", def);
}
public void writeMulticastDefinition(
MulticastDefinition def)
throws IOException {
doWriteMulticastDefinition("multicast", def);
}
public void writeOnCompletionDefinition(
OnCompletionDefinition def)
throws IOException {
doWriteOnCompletionDefinition("onCompletion", def);
}
public void writeOnExceptionDefinition(
OnExceptionDefinition def)
throws IOException {
doWriteOnExceptionDefinition("onException", def);
}
public void writeOnFallbackDefinition(
OnFallbackDefinition def)
throws IOException {
doWriteOnFallbackDefinition("onFallback", def);
}
public void writeOptimisticLockRetryPolicyDefinition(
OptimisticLockRetryPolicyDefinition def)
throws IOException {
doWriteOptimisticLockRetryPolicyDefinition("optimisticLockRetryPolicy", def);
}
public void writeOtherwiseDefinition(
OtherwiseDefinition def)
throws IOException {
doWriteOtherwiseDefinition("otherwise", def);
}
public void writeOutputTypeDefinition(
OutputTypeDefinition def)
throws IOException {
doWriteOutputTypeDefinition("outputType", def);
}
public void writePackageScanDefinition(
PackageScanDefinition def)
throws IOException {
doWritePackageScanDefinition("packageScan", def);
}
public void writePausableDefinition(
PausableDefinition def)
throws IOException {
doWritePausableDefinition("pausable", def);
}
public void writePipelineDefinition(
PipelineDefinition def)
throws IOException {
doWritePipelineDefinition("pipeline", def);
}
public void writePolicyDefinition(PolicyDefinition def) throws IOException {
doWritePolicyDefinition("policy", def);
}
public void writePollEnrichDefinition(
PollEnrichDefinition def)
throws IOException {
doWritePollEnrichDefinition("pollEnrich", def);
}
public void writeProcessDefinition(ProcessDefinition def) throws IOException {
doWriteProcessDefinition("process", def);
}
public void writePropertyDefinition(
PropertyDefinition def)
throws IOException {
doWritePropertyDefinition("property", def);
}
public void writePropertyExpressionDefinition(
PropertyExpressionDefinition def)
throws IOException {
doWritePropertyExpressionDefinition("propertyExpression", def);
}
public void writeRecipientListDefinition(
RecipientListDefinition def)
throws IOException {
doWriteRecipientListDefinition("recipientList", def);
}
public void writeRedeliveryPolicyDefinition(
RedeliveryPolicyDefinition def)
throws IOException {
doWriteRedeliveryPolicyDefinition("redeliveryPolicy", def);
}
public void writeRemoveHeaderDefinition(
RemoveHeaderDefinition def)
throws IOException {
doWriteRemoveHeaderDefinition("removeHeader", def);
}
public void writeRemoveHeadersDefinition(
RemoveHeadersDefinition def)
throws IOException {
doWriteRemoveHeadersDefinition("removeHeaders", def);
}
public void writeRemovePropertiesDefinition(
RemovePropertiesDefinition def)
throws IOException {
doWriteRemovePropertiesDefinition("removeProperties", def);
}
public void writeRemovePropertyDefinition(
RemovePropertyDefinition def)
throws IOException {
doWriteRemovePropertyDefinition("removeProperty", def);
}
public void writeRemoveVariableDefinition(
RemoveVariableDefinition def)
throws IOException {
doWriteRemoveVariableDefinition("removeVariable", def);
}
public void writeResequenceDefinition(
ResequenceDefinition def)
throws IOException {
doWriteResequenceDefinition("resequence", def);
}
public void writeResilience4jConfigurationDefinition(
Resilience4jConfigurationDefinition def)
throws IOException {
doWriteResilience4jConfigurationDefinition("resilience4jConfiguration", def);
}
public void writeRestContextRefDefinition(
RestContextRefDefinition def)
throws IOException {
doWriteRestContextRefDefinition("restContextRef", def);
}
public void writeResumableDefinition(
ResumableDefinition def)
throws IOException {
doWriteResumableDefinition("resumable", def);
}
public void writeRollbackDefinition(
RollbackDefinition def)
throws IOException {
doWriteRollbackDefinition("rollback", def);
}
public void writeRouteBuilderDefinition(
RouteBuilderDefinition def)
throws IOException {
doWriteRouteBuilderDefinition("routeBuilder", def);
}
public void writeRouteConfigurationContextRefDefinition(
RouteConfigurationContextRefDefinition def)
throws IOException {
doWriteRouteConfigurationContextRefDefinition("routeConfigurationContextRef", def);
}
public void writeRouteConfigurationDefinition(
RouteConfigurationDefinition def)
throws IOException {
doWriteRouteConfigurationDefinition("routeConfiguration", def);
}
public void writeRouteConfigurationsDefinition(
RouteConfigurationsDefinition def)
throws IOException {
doWriteRouteConfigurationsDefinition("routeConfigurations", def);
}
public void writeRouteContextRefDefinition(
RouteContextRefDefinition def)
throws IOException {
doWriteRouteContextRefDefinition("routeContextRef", def);
}
public void writeRouteDefinition(RouteDefinition def) throws IOException {
doWriteRouteDefinition("route", def);
}
public void writeRouteTemplateBeanDefinition(
RouteTemplateBeanDefinition def)
throws IOException {
doWriteRouteTemplateBeanDefinition("templateBean", def);
}
public void writeRouteTemplateContextRefDefinition(
RouteTemplateContextRefDefinition def)
throws IOException {
doWriteRouteTemplateContextRefDefinition("routeTemplateContextRef", def);
}
public void writeRouteTemplateDefinition(
RouteTemplateDefinition def)
throws IOException {
doWriteRouteTemplateDefinition("routeTemplate", def);
}
public void writeRouteTemplateParameterDefinition(
RouteTemplateParameterDefinition def)
throws IOException {
doWriteRouteTemplateParameterDefinition("templateParameter", def);
}
public void writeRouteTemplatesDefinition(
RouteTemplatesDefinition def)
throws IOException {
doWriteRouteTemplatesDefinition("routeTemplates", def);
}
public void writeRoutesDefinition(RoutesDefinition def) throws IOException {
doWriteRoutesDefinition("routes", def);
}
public void writeRoutingSlipDefinition(
RoutingSlipDefinition def)
throws IOException {
doWriteRoutingSlipDefinition("routingSlip", def);
}
public void writeSagaDefinition(SagaDefinition def) throws IOException {
doWriteSagaDefinition("saga", def);
}
public void writeSamplingDefinition(
SamplingDefinition def)
throws IOException {
doWriteSamplingDefinition("sample", def);
}
public void writeScriptDefinition(ScriptDefinition def) throws IOException {
doWriteScriptDefinition("script", def);
}
public void writeSetBodyDefinition(SetBodyDefinition def) throws IOException {
doWriteSetBodyDefinition("setBody", def);
}
public void writeSetExchangePatternDefinition(
SetExchangePatternDefinition def)
throws IOException {
doWriteSetExchangePatternDefinition("setExchangePattern", def);
}
public void writeSetHeaderDefinition(
SetHeaderDefinition def)
throws IOException {
doWriteSetHeaderDefinition("setHeader", def);
}
public void writeSetHeadersDefinition(
SetHeadersDefinition def)
throws IOException {
doWriteSetHeadersDefinition("setHeaders", def);
}
public void writeSetPropertyDefinition(
SetPropertyDefinition def)
throws IOException {
doWriteSetPropertyDefinition("setProperty", def);
}
public void writeSetVariableDefinition(
SetVariableDefinition def)
throws IOException {
doWriteSetVariableDefinition("setVariable", def);
}
public void writeSortDefinition(SortDefinition def) throws IOException {
doWriteSortDefinition("sort", def);
}
public void writeSplitDefinition(SplitDefinition def) throws IOException {
doWriteSplitDefinition("split", def);
}
public void writeStepDefinition(StepDefinition def) throws IOException {
doWriteStepDefinition("step", def);
}
public void writeStopDefinition(StopDefinition def) throws IOException {
doWriteStopDefinition("stop", def);
}
public void writeTemplatedRouteBeanDefinition(
TemplatedRouteBeanDefinition def)
throws IOException {
doWriteTemplatedRouteBeanDefinition("templatedRouteBean", def);
}
public void writeTemplatedRouteDefinition(
TemplatedRouteDefinition def)
throws IOException {
doWriteTemplatedRouteDefinition("templatedRoute", def);
}
public void writeTemplatedRouteParameterDefinition(
TemplatedRouteParameterDefinition def)
throws IOException {
doWriteTemplatedRouteParameterDefinition("templatedRouteParameter", def);
}
public void writeTemplatedRoutesDefinition(
TemplatedRoutesDefinition def)
throws IOException {
doWriteTemplatedRoutesDefinition("templatedRoutes", def);
}
public void writeThreadPoolProfileDefinition(
ThreadPoolProfileDefinition def)
throws IOException {
doWriteThreadPoolProfileDefinition("threadPoolProfile", def);
}
public void writeThreadsDefinition(ThreadsDefinition def) throws IOException {
doWriteThreadsDefinition("threads", def);
}
public void writeThrottleDefinition(
ThrottleDefinition def)
throws IOException {
doWriteThrottleDefinition("throttle", def);
}
public void writeThrowExceptionDefinition(
ThrowExceptionDefinition def)
throws IOException {
doWriteThrowExceptionDefinition("throwException", def);
}
public void writeToDefinition(ToDefinition def) throws IOException {
doWriteToDefinition("to", def);
}
public void writeToDynamicDefinition(
ToDynamicDefinition def)
throws IOException {
doWriteToDynamicDefinition("toD", def);
}
public void writeTransactedDefinition(
TransactedDefinition def)
throws IOException {
doWriteTransactedDefinition("transacted", def);
}
public void writeTransformDefinition(
TransformDefinition def)
throws IOException {
doWriteTransformDefinition("transform", def);
}
public void writeTryDefinition(TryDefinition def) throws IOException {
doWriteTryDefinition("doTry", def);
}
public void writeUnmarshalDefinition(
UnmarshalDefinition def)
throws IOException {
doWriteUnmarshalDefinition("unmarshal", def);
}
public void writeValidateDefinition(
ValidateDefinition def)
throws IOException {
doWriteValidateDefinition("validate", def);
}
public void writeValueDefinition(ValueDefinition def) throws IOException {
doWriteValueDefinition("value", def);
}
public void writeWhenDefinition(WhenDefinition def) throws IOException {
doWriteWhenDefinition("when", def);
}
public void writeWireTapDefinition(WireTapDefinition def) throws IOException {
doWriteWireTapDefinition("wireTap", def);
}
public void writeApplicationDefinition(
ApplicationDefinition def)
throws IOException {
doWriteApplicationDefinition("camel", def);
}
public void writeBeansDefinition(BeansDefinition def) throws IOException {
doWriteBeansDefinition("beans", def);
}
public void writeBlacklistServiceCallServiceFilterConfiguration(
BlacklistServiceCallServiceFilterConfiguration def)
throws IOException {
doWriteBlacklistServiceCallServiceFilterConfiguration("blacklistServiceFilter", def);
}
public void writeCachingServiceCallServiceDiscoveryConfiguration(
CachingServiceCallServiceDiscoveryConfiguration def)
throws IOException {
doWriteCachingServiceCallServiceDiscoveryConfiguration("cachingServiceDiscovery", def);
}
public void writeCombinedServiceCallServiceDiscoveryConfiguration(
CombinedServiceCallServiceDiscoveryConfiguration def)
throws IOException {
doWriteCombinedServiceCallServiceDiscoveryConfiguration("combinedServiceDiscovery", def);
}
public void writeCombinedServiceCallServiceFilterConfiguration(
CombinedServiceCallServiceFilterConfiguration def)
throws IOException {
doWriteCombinedServiceCallServiceFilterConfiguration("combinedServiceFilter", def);
}
public void writeConsulServiceCallServiceDiscoveryConfiguration(
ConsulServiceCallServiceDiscoveryConfiguration def)
throws IOException {
doWriteConsulServiceCallServiceDiscoveryConfiguration("consulServiceDiscovery", def);
}
public void writeCustomServiceCallServiceFilterConfiguration(
CustomServiceCallServiceFilterConfiguration def)
throws IOException {
doWriteCustomServiceCallServiceFilterConfiguration("customServiceFilter", def);
}
public void writeDefaultServiceCallServiceLoadBalancerConfiguration(
DefaultServiceCallServiceLoadBalancerConfiguration def)
throws IOException {
doWriteDefaultServiceCallServiceLoadBalancerConfiguration("defaultLoadBalancer", def);
}
public void writeDnsServiceCallServiceDiscoveryConfiguration(
DnsServiceCallServiceDiscoveryConfiguration def)
throws IOException {
doWriteDnsServiceCallServiceDiscoveryConfiguration("dnsServiceDiscovery", def);
}
public void writeHealthyServiceCallServiceFilterConfiguration(
HealthyServiceCallServiceFilterConfiguration def)
throws IOException {
doWriteHealthyServiceCallServiceFilterConfiguration("healthyServiceFilter", def);
}
public void writeKubernetesServiceCallServiceDiscoveryConfiguration(
KubernetesServiceCallServiceDiscoveryConfiguration def)
throws IOException {
doWriteKubernetesServiceCallServiceDiscoveryConfiguration("kubernetesServiceDiscovery", def);
}
public void writePassThroughServiceCallServiceFilterConfiguration(
PassThroughServiceCallServiceFilterConfiguration def)
throws IOException {
doWritePassThroughServiceCallServiceFilterConfiguration("passThroughServiceFilter", def);
}
public void writeServiceCallConfigurationDefinition(
ServiceCallConfigurationDefinition def)
throws IOException {
doWriteServiceCallConfigurationDefinition("serviceCallConfiguration", def);
}
public void writeServiceCallDefinition(
ServiceCallDefinition def)
throws IOException {
doWriteServiceCallDefinition("serviceCall", def);
}
public void writeServiceCallExpressionConfiguration(
ServiceCallExpressionConfiguration def)
throws IOException {
doWriteServiceCallExpressionConfiguration("serviceExpression", def);
}
public void writeServiceCallServiceChooserConfiguration(
ServiceCallServiceChooserConfiguration def)
throws IOException {
doWriteServiceCallServiceChooserConfiguration("serviceChooserConfiguration", def);
}
public void writeServiceCallServiceDiscoveryConfiguration(
ServiceCallServiceDiscoveryConfiguration def)
throws IOException {
doWriteServiceCallServiceDiscoveryConfiguration("serviceDiscoveryConfiguration", def);
}
public void writeServiceCallServiceFilterConfiguration(
ServiceCallServiceFilterConfiguration def)
throws IOException {
doWriteServiceCallServiceFilterConfiguration("serviceFilterConfiguration", def);
}
public void writeServiceCallServiceLoadBalancerConfiguration(
ServiceCallServiceLoadBalancerConfiguration def)
throws IOException {
doWriteServiceCallServiceLoadBalancerConfiguration("loadBalancerConfiguration", def);
}
public void writeStaticServiceCallServiceDiscoveryConfiguration(
StaticServiceCallServiceDiscoveryConfiguration def)
throws IOException {
doWriteStaticServiceCallServiceDiscoveryConfiguration("staticServiceDiscovery", def);
}
public void writeZooKeeperServiceCallServiceDiscoveryConfiguration(
ZooKeeperServiceCallServiceDiscoveryConfiguration def)
throws IOException {
doWriteZooKeeperServiceCallServiceDiscoveryConfiguration("zookeeperServiceDiscovery", def);
}
public void writeBatchResequencerConfig(
BatchResequencerConfig def)
throws IOException {
doWriteBatchResequencerConfig("batchConfig", def);
}
public void writeStreamResequencerConfig(
StreamResequencerConfig def)
throws IOException {
doWriteStreamResequencerConfig("streamConfig", def);
}
public void writeASN1DataFormat(ASN1DataFormat def) throws IOException {
doWriteASN1DataFormat("asn1", def);
}
public void writeAvroDataFormat(AvroDataFormat def) throws IOException {
doWriteAvroDataFormat("avro", def);
}
public void writeBarcodeDataFormat(BarcodeDataFormat def) throws IOException {
doWriteBarcodeDataFormat("barcode", def);
}
public void writeBase64DataFormat(Base64DataFormat def) throws IOException {
doWriteBase64DataFormat("base64", def);
}
public void writeBeanioDataFormat(BeanioDataFormat def) throws IOException {
doWriteBeanioDataFormat("beanio", def);
}
public void writeBindyDataFormat(BindyDataFormat def) throws IOException {
doWriteBindyDataFormat("bindy", def);
}
public void writeCBORDataFormat(CBORDataFormat def) throws IOException {
doWriteCBORDataFormat("cbor", def);
}
public void writeCryptoDataFormat(CryptoDataFormat def) throws IOException {
doWriteCryptoDataFormat("crypto", def);
}
public void writeCsvDataFormat(CsvDataFormat def) throws IOException {
doWriteCsvDataFormat("csv", def);
}
public void writeCustomDataFormat(CustomDataFormat def) throws IOException {
doWriteCustomDataFormat("custom", def);
}
public void writeDataFormatsDefinition(
DataFormatsDefinition def)
throws IOException {
doWriteDataFormatsDefinition("dataFormats", def);
}
public void writeFhirJsonDataFormat(
FhirJsonDataFormat def)
throws IOException {
doWriteFhirJsonDataFormat("fhirJson", def);
}
public void writeFhirXmlDataFormat(FhirXmlDataFormat def) throws IOException {
doWriteFhirXmlDataFormat("fhirXml", def);
}
public void writeFlatpackDataFormat(
FlatpackDataFormat def)
throws IOException {
doWriteFlatpackDataFormat("flatpack", def);
}
public void writeGrokDataFormat(GrokDataFormat def) throws IOException {
doWriteGrokDataFormat("grok", def);
}
public void writeGzipDeflaterDataFormat(
GzipDeflaterDataFormat def)
throws IOException {
doWriteGzipDeflaterDataFormat("gzipDeflater", def);
}
public void writeHL7DataFormat(HL7DataFormat def) throws IOException {
doWriteHL7DataFormat("hl7", def);
}
public void writeIcalDataFormat(IcalDataFormat def) throws IOException {
doWriteIcalDataFormat("ical", def);
}
public void writeJacksonXMLDataFormat(
JacksonXMLDataFormat def)
throws IOException {
doWriteJacksonXMLDataFormat("jacksonXml", def);
}
public void writeJaxbDataFormat(JaxbDataFormat def) throws IOException {
doWriteJaxbDataFormat("jaxb", def);
}
public void writeJsonApiDataFormat(JsonApiDataFormat def) throws IOException {
doWriteJsonApiDataFormat("jsonApi", def);
}
public void writeJsonDataFormat(JsonDataFormat def) throws IOException {
doWriteJsonDataFormat("json", def);
}
public void writeLZFDataFormat(LZFDataFormat def) throws IOException {
doWriteLZFDataFormat("lzf", def);
}
public void writeMimeMultipartDataFormat(
MimeMultipartDataFormat def)
throws IOException {
doWriteMimeMultipartDataFormat("mimeMultipart", def);
}
public void writePGPDataFormat(PGPDataFormat def) throws IOException {
doWritePGPDataFormat("pgp", def);
}
public void writeParquetAvroDataFormat(
ParquetAvroDataFormat def)
throws IOException {
doWriteParquetAvroDataFormat("parquetAvro", def);
}
public void writeProtobufDataFormat(
ProtobufDataFormat def)
throws IOException {
doWriteProtobufDataFormat("protobuf", def);
}
public void writeRssDataFormat(RssDataFormat def) throws IOException {
doWriteRssDataFormat("rss", def);
}
public void writeSoapDataFormat(SoapDataFormat def) throws IOException {
doWriteSoapDataFormat("soap", def);
}
public void writeSwiftMtDataFormat(SwiftMtDataFormat def) throws IOException {
doWriteSwiftMtDataFormat("swiftMt", def);
}
public void writeSwiftMxDataFormat(SwiftMxDataFormat def) throws IOException {
doWriteSwiftMxDataFormat("swiftMx", def);
}
public void writeSyslogDataFormat(SyslogDataFormat def) throws IOException {
doWriteSyslogDataFormat("syslog", def);
}
public void writeTarFileDataFormat(TarFileDataFormat def) throws IOException {
doWriteTarFileDataFormat("tarFile", def);
}
public void writeThriftDataFormat(ThriftDataFormat def) throws IOException {
doWriteThriftDataFormat("thrift", def);
}
public void writeTidyMarkupDataFormat(
TidyMarkupDataFormat def)
throws IOException {
doWriteTidyMarkupDataFormat("tidyMarkup", def);
}
public void writeUniVocityCsvDataFormat(
UniVocityCsvDataFormat def)
throws IOException {
doWriteUniVocityCsvDataFormat("univocityCsv", def);
}
public void writeUniVocityFixedDataFormat(
UniVocityFixedDataFormat def)
throws IOException {
doWriteUniVocityFixedDataFormat("univocityFixed", def);
}
public void writeUniVocityHeader(UniVocityHeader def) throws IOException {
doWriteUniVocityHeader("univocityHeader", def);
}
public void writeUniVocityTsvDataFormat(
UniVocityTsvDataFormat def)
throws IOException {
doWriteUniVocityTsvDataFormat("univocityTsv", def);
}
public void writeXMLSecurityDataFormat(
XMLSecurityDataFormat def)
throws IOException {
doWriteXMLSecurityDataFormat("xmlSecurity", def);
}
public void writeYAMLDataFormat(YAMLDataFormat def) throws IOException {
doWriteYAMLDataFormat("yaml", def);
}
public void writeYAMLTypeFilterDefinition(
YAMLTypeFilterDefinition def)
throws IOException {
doWriteYAMLTypeFilterDefinition("typeFilter", def);
}
public void writeZipDeflaterDataFormat(
ZipDeflaterDataFormat def)
throws IOException {
doWriteZipDeflaterDataFormat("zipDeflater", def);
}
public void writeZipFileDataFormat(ZipFileDataFormat def) throws IOException {
doWriteZipFileDataFormat("zipFile", def);
}
public void writeDeadLetterChannelDefinition(
DeadLetterChannelDefinition def)
throws IOException {
doWriteDeadLetterChannelDefinition("deadLetterChannel", def);
}
public void writeDefaultErrorHandlerDefinition(
DefaultErrorHandlerDefinition def)
throws IOException {
doWriteDefaultErrorHandlerDefinition("defaultErrorHandler", def);
}
public void writeJtaTransactionErrorHandlerDefinition(
JtaTransactionErrorHandlerDefinition def)
throws IOException {
doWriteJtaTransactionErrorHandlerDefinition("jtaTransactionErrorHandler", def);
}
public void writeNoErrorHandlerDefinition(
NoErrorHandlerDefinition def)
throws IOException {
doWriteNoErrorHandlerDefinition("noErrorHandler", def);
}
public void writeRefErrorHandlerDefinition(
RefErrorHandlerDefinition def)
throws IOException {
doWriteRefErrorHandlerDefinition("refErrorHandler", def);
}
public void writeSpringTransactionErrorHandlerDefinition(
SpringTransactionErrorHandlerDefinition def)
throws IOException {
doWriteSpringTransactionErrorHandlerDefinition("springTransactionErrorHandler", def);
}
public void writeCSimpleExpression(CSimpleExpression def) throws IOException {
doWriteCSimpleExpression("csimple", def);
}
public void writeConstantExpression(
ConstantExpression def)
throws IOException {
doWriteConstantExpression("constant", def);
}
public void writeDatasonnetExpression(
DatasonnetExpression def)
throws IOException {
doWriteDatasonnetExpression("datasonnet", def);
}
public void writeExchangePropertyExpression(
ExchangePropertyExpression def)
throws IOException {
doWriteExchangePropertyExpression("exchangeProperty", def);
}
public void writeExpressionDefinition(
ExpressionDefinition def)
throws IOException {
doWriteExpressionDefinition("##default", def);
}
public void writeGroovyExpression(GroovyExpression def) throws IOException {
doWriteGroovyExpression("groovy", def);
}
public void writeHeaderExpression(HeaderExpression def) throws IOException {
doWriteHeaderExpression("header", def);
}
public void writeHl7TerserExpression(
Hl7TerserExpression def)
throws IOException {
doWriteHl7TerserExpression("hl7terser", def);
}
public void writeJavaExpression(JavaExpression def) throws IOException {
doWriteJavaExpression("java", def);
}
public void writeJavaScriptExpression(
JavaScriptExpression def)
throws IOException {
doWriteJavaScriptExpression("js", def);
}
public void writeJoorExpression(JoorExpression def) throws IOException {
doWriteJoorExpression("joor", def);
}
public void writeJqExpression(JqExpression def) throws IOException {
doWriteJqExpression("jq", def);
}
public void writeJsonPathExpression(
JsonPathExpression def)
throws IOException {
doWriteJsonPathExpression("jsonpath", def);
}
public void writeLanguageExpression(
LanguageExpression def)
throws IOException {
doWriteLanguageExpression("language", def);
}
public void writeMethodCallExpression(
MethodCallExpression def)
throws IOException {
doWriteMethodCallExpression("method", def);
}
public void writeMvelExpression(MvelExpression def) throws IOException {
doWriteMvelExpression("mvel", def);
}
public void writeOgnlExpression(OgnlExpression def) throws IOException {
doWriteOgnlExpression("ognl", def);
}
public void writePythonExpression(PythonExpression def) throws IOException {
doWritePythonExpression("python", def);
}
public void writeRefExpression(RefExpression def) throws IOException {
doWriteRefExpression("ref", def);
}
public void writeSimpleExpression(SimpleExpression def) throws IOException {
doWriteSimpleExpression("simple", def);
}
public void writeSpELExpression(SpELExpression def) throws IOException {
doWriteSpELExpression("spel", def);
}
public void writeTokenizerExpression(
TokenizerExpression def)
throws IOException {
doWriteTokenizerExpression("tokenize", def);
}
public void writeVariableExpression(
VariableExpression def)
throws IOException {
doWriteVariableExpression("variable", def);
}
public void writeWasmExpression(WasmExpression def) throws IOException {
doWriteWasmExpression("wasm", def);
}
public void writeXMLTokenizerExpression(
XMLTokenizerExpression def)
throws IOException {
doWriteXMLTokenizerExpression("xtokenize", def);
}
public void writeXPathExpression(XPathExpression def) throws IOException {
doWriteXPathExpression("xpath", def);
}
public void writeXQueryExpression(XQueryExpression def) throws IOException {
doWriteXQueryExpression("xquery", def);
}
public void writeCustomLoadBalancerDefinition(
CustomLoadBalancerDefinition def)
throws IOException {
doWriteCustomLoadBalancerDefinition("customLoadBalancer", def);
}
public void writeFailoverLoadBalancerDefinition(
FailoverLoadBalancerDefinition def)
throws IOException {
doWriteFailoverLoadBalancerDefinition("failover", def);
}
public void writeRandomLoadBalancerDefinition(
RandomLoadBalancerDefinition def)
throws IOException {
doWriteRandomLoadBalancerDefinition("random", def);
}
public void writeRoundRobinLoadBalancerDefinition(
RoundRobinLoadBalancerDefinition def)
throws IOException {
doWriteRoundRobinLoadBalancerDefinition("roundRobin", def);
}
public void writeStickyLoadBalancerDefinition(
StickyLoadBalancerDefinition def)
throws IOException {
doWriteStickyLoadBalancerDefinition("sticky", def);
}
public void writeTopicLoadBalancerDefinition(
TopicLoadBalancerDefinition def)
throws IOException {
doWriteTopicLoadBalancerDefinition("topic", def);
}
public void writeWeightedLoadBalancerDefinition(
WeightedLoadBalancerDefinition def)
throws IOException {
doWriteWeightedLoadBalancerDefinition("weighted", def);
}
public void writeApiKeyDefinition(ApiKeyDefinition def) throws IOException {
doWriteApiKeyDefinition("apiKey", def);
}
public void writeBasicAuthDefinition(
BasicAuthDefinition def)
throws IOException {
doWriteBasicAuthDefinition("basicAuth", def);
}
public void writeBearerTokenDefinition(
BearerTokenDefinition def)
throws IOException {
doWriteBearerTokenDefinition("bearerToken", def);
}
public void writeDeleteDefinition(DeleteDefinition def) throws IOException {
doWriteDeleteDefinition("delete", def);
}
public void writeGetDefinition(GetDefinition def) throws IOException {
doWriteGetDefinition("get", def);
}
public void writeHeadDefinition(HeadDefinition def) throws IOException {
doWriteHeadDefinition("head", def);
}
public void writeMutualTLSDefinition(
MutualTLSDefinition def)
throws IOException {
doWriteMutualTLSDefinition("mutualTLS", def);
}
public void writeOAuth2Definition(OAuth2Definition def) throws IOException {
doWriteOAuth2Definition("oauth2", def);
}
public void writeOpenIdConnectDefinition(
OpenIdConnectDefinition def)
throws IOException {
doWriteOpenIdConnectDefinition("openIdConnect", def);
}
public void writeParamDefinition(ParamDefinition def) throws IOException {
doWriteParamDefinition("param", def);
}
public void writePatchDefinition(PatchDefinition def) throws IOException {
doWritePatchDefinition("patch", def);
}
public void writePostDefinition(PostDefinition def) throws IOException {
doWritePostDefinition("post", def);
}
public void writePutDefinition(PutDefinition def) throws IOException {
doWritePutDefinition("put", def);
}
public void writeResponseHeaderDefinition(
ResponseHeaderDefinition def)
throws IOException {
doWriteResponseHeaderDefinition("responseHeader", def);
}
public void writeResponseMessageDefinition(
ResponseMessageDefinition def)
throws IOException {
doWriteResponseMessageDefinition("responseMessage", def);
}
public void writeRestBindingDefinition(
RestBindingDefinition def)
throws IOException {
doWriteRestBindingDefinition("restBinding", def);
}
public void writeRestConfigurationDefinition(
RestConfigurationDefinition def)
throws IOException {
doWriteRestConfigurationDefinition("restConfiguration", def);
}
public void writeRestDefinition(RestDefinition def) throws IOException {
doWriteRestDefinition("rest", def);
}
public void writeRestPropertyDefinition(
RestPropertyDefinition def)
throws IOException {
doWriteRestPropertyDefinition("restProperty", def);
}
public void writeRestSecuritiesDefinition(
RestSecuritiesDefinition def)
throws IOException {
doWriteRestSecuritiesDefinition("securityDefinitions", def);
}
public void writeRestsDefinition(RestsDefinition def) throws IOException {
doWriteRestsDefinition("rests", def);
}
public void writeSecurityDefinition(
SecurityDefinition def)
throws IOException {
doWriteSecurityDefinition("security", def);
}
public void writeTransformersDefinition(
TransformersDefinition def)
throws IOException {
doWriteTransformersDefinition("transformers", def);
}
public void writeValidatorsDefinition(
ValidatorsDefinition def)
throws IOException {
doWriteValidatorsDefinition("validators", def);
}
public void writeOptionalIdentifiedDefinitionRef(OptionalIdentifiedDefinition def) throws IOException {
doWriteOptionalIdentifiedDefinitionRef(null, def);
}
protected void doWriteAggregateDefinition(
String name,
AggregateDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("aggregationStrategy", def.getAggregationStrategy());
doWriteAttribute("aggregationRepository", def.getAggregationRepository());
doWriteAttribute("executorService", def.getExecutorService());
doWriteAttribute("closeCorrelationKeyOnCompletion", def.getCloseCorrelationKeyOnCompletion());
doWriteAttribute("discardOnAggregationFailure", def.getDiscardOnAggregationFailure());
doWriteAttribute("eagerCheckCompletion", def.getEagerCheckCompletion());
doWriteAttribute("timeoutCheckerExecutorService", def.getTimeoutCheckerExecutorService());
doWriteAttribute("completionOnNewCorrelationGroup", def.getCompletionOnNewCorrelationGroup());
doWriteAttribute("completionInterval", def.getCompletionInterval());
doWriteAttribute("parallelProcessing", def.getParallelProcessing());
doWriteAttribute("forceCompletionOnStop", def.getForceCompletionOnStop());
doWriteAttribute("aggregationStrategyMethodAllowNull", def.getAggregationStrategyMethodAllowNull());
doWriteAttribute("completionFromBatchConsumer", def.getCompletionFromBatchConsumer());
doWriteAttribute("completeAllOnStop", def.getCompleteAllOnStop());
doWriteAttribute("completionSize", def.getCompletionSize());
doWriteAttribute("aggregationStrategyMethodName", def.getAggregationStrategyMethodName());
doWriteAttribute("aggregateController", def.getAggregateController());
doWriteAttribute("completionTimeout", def.getCompletionTimeout());
doWriteAttribute("ignoreInvalidCorrelationKeys", def.getIgnoreInvalidCorrelationKeys());
doWriteAttribute("discardOnCompletionTimeout", def.getDiscardOnCompletionTimeout());
doWriteAttribute("completionTimeoutCheckerInterval", def.getCompletionTimeoutCheckerInterval());
doWriteAttribute("optimisticLocking", def.getOptimisticLocking());
doWriteElement("optimisticLockRetryPolicy", def.getOptimisticLockRetryPolicyDefinition(), this::doWriteOptimisticLockRetryPolicyDefinition);
doWriteElement("correlationExpression", def.getCorrelationExpression(), this::doWriteExpressionSubElementDefinition);
doWriteElement("completionPredicate", def.getCompletionPredicate(), this::doWriteExpressionSubElementDefinition);
doWriteElement("completionSizeExpression", def.getCompletionSizeExpression(), this::doWriteExpressionSubElementDefinition);
doWriteElement("completionTimeoutExpression", def.getCompletionTimeoutExpression(), this::doWriteExpressionSubElementDefinition);
doWriteList(null, null, def.getOutputs(), this::doWriteProcessorDefinitionRef);
endElement(name);
}
protected void doWriteBeanDefinition(
String name,
BeanDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("ref", def.getRef());
doWriteAttribute("method", def.getMethod());
doWriteAttribute("scope", def.getScope());
doWriteAttribute("beanType", def.getBeanType());
endElement(name);
}
protected void doWriteBeanFactoryDefinitionAttributes(
BeanFactoryDefinition, ?> def)
throws IOException {
doWriteAttribute("scriptLanguage", def.getScriptLanguage());
doWriteAttribute("name", def.getName());
doWriteAttribute("type", def.getType());
}
protected void doWriteBeanFactoryDefinitionElements(
BeanFactoryDefinition, ?> def)
throws IOException {
doWriteList(null, "property", def.getPropertyDefinitions(), this::doWritePropertyDefinition);
doWriteElement("script", def.getScript(), this::doWriteString);
doWriteElement("properties", new BeanPropertiesAdapter().marshal(def.getProperties()), this::doWriteBeanPropertiesDefinition);
}
protected void doWriteBeanFactoryDefinition(
String name,
BeanFactoryDefinition, ?> def)
throws IOException {
startElement(name);
doWriteBeanFactoryDefinitionAttributes(def);
doWriteBeanFactoryDefinitionElements(def);
endElement(name);
}
protected void doWriteCatchDefinition(
String name,
CatchDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteElement("onWhen", def.getOnWhen(), this::doWriteWhenDefinition);
doWriteList(null, "exception", def.getExceptions(), this::doWriteString);
doWriteList(null, null, def.getOutputs(), this::doWriteProcessorDefinitionRef);
endElement(name);
}
protected void doWriteChoiceDefinition(
String name,
ChoiceDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("precondition", def.getPrecondition());
doWriteList(null, null, def.getWhenClauses(), this::doWriteWhenDefinitionRef);
doWriteElement("otherwise", def.getOtherwise(), this::doWriteOtherwiseDefinition);
endElement(name);
}
protected void doWriteCircuitBreakerDefinition(
String name,
CircuitBreakerDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("configuration", def.getConfiguration());
doWriteElement("faultToleranceConfiguration", def.getFaultToleranceConfiguration(), this::doWriteFaultToleranceConfigurationDefinition);
doWriteElement("resilience4jConfiguration", def.getResilience4jConfiguration(), this::doWriteResilience4jConfigurationDefinition);
doWriteElement("onFallback", def.getOnFallback(), this::doWriteOnFallbackDefinition);
doWriteList(null, null, def.getOutputs(), this::doWriteProcessorDefinitionRef);
endElement(name);
}
protected void doWriteClaimCheckDefinition(
String name,
ClaimCheckDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("filter", def.getFilter());
doWriteAttribute("aggregationStrategy", def.getAggregationStrategy());
doWriteAttribute("aggregationStrategyMethodName", def.getAggregationStrategyMethodName());
doWriteAttribute("operation", def.getOperation());
doWriteAttribute("key", def.getKey());
endElement(name);
}
protected void doWriteContextScanDefinition(
String name,
ContextScanDefinition def)
throws IOException {
startElement(name);
doWriteAttribute("includeNonSingletons", def.getIncludeNonSingletons());
doWriteList(null, "excludes", def.getExcludes(), this::doWriteString);
doWriteList(null, "includes", def.getIncludes(), this::doWriteString);
endElement(name);
}
protected void doWriteConvertBodyDefinition(
String name,
ConvertBodyDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("charset", def.getCharset());
doWriteAttribute("type", def.getType());
doWriteAttribute("mandatory", def.getMandatory());
endElement(name);
}
protected void doWriteConvertHeaderDefinition(
String name,
ConvertHeaderDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("charset", def.getCharset());
doWriteAttribute("toName", def.getToName());
doWriteAttribute("name", def.getName());
doWriteAttribute("type", def.getType());
doWriteAttribute("mandatory", def.getMandatory());
endElement(name);
}
protected void doWriteConvertVariableDefinition(
String name,
ConvertVariableDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("charset", def.getCharset());
doWriteAttribute("toName", def.getToName());
doWriteAttribute("name", def.getName());
doWriteAttribute("type", def.getType());
doWriteAttribute("mandatory", def.getMandatory());
endElement(name);
}
protected void doWriteDataFormatDefinitionAttributes(
DataFormatDefinition def)
throws IOException {
doWriteIdentifiedTypeAttributes(def);
}
protected void doWriteDataFormatDefinition(
String name,
DataFormatDefinition def)
throws IOException {
startElement(name);
doWriteDataFormatDefinitionAttributes(def);
endElement(name);
}
protected void doWriteDelayDefinition(
String name,
DelayDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("executorService", def.getExecutorService());
doWriteAttribute("callerRunsWhenRejected", def.getCallerRunsWhenRejected());
doWriteAttribute("asyncDelayed", def.getAsyncDelayed());
doWriteExpressionNodeElements(def);
endElement(name);
}
protected void doWriteDynamicRouterDefinition(
String name,
DynamicRouterDefinition> def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("uriDelimiter", def.getUriDelimiter());
doWriteAttribute("ignoreInvalidEndpoints", def.getIgnoreInvalidEndpoints());
doWriteAttribute("cacheSize", def.getCacheSize());
doWriteExpressionNodeElements(def);
endElement(name);
}
protected void doWriteEnrichDefinition(
String name,
EnrichDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("variableReceive", def.getVariableReceive());
doWriteAttribute("variableSend", def.getVariableSend());
doWriteAttribute("cacheSize", def.getCacheSize());
doWriteAttribute("aggregationStrategy", def.getAggregationStrategy());
doWriteAttribute("ignoreInvalidEndpoint", def.getIgnoreInvalidEndpoint());
doWriteAttribute("autoStartComponents", def.getAutoStartComponents());
doWriteAttribute("allowOptimisedComponents", def.getAllowOptimisedComponents());
doWriteAttribute("aggregateOnException", def.getAggregateOnException());
doWriteAttribute("aggregationStrategyMethodName", def.getAggregationStrategyMethodName());
doWriteAttribute("shareUnitOfWork", def.getShareUnitOfWork());
doWriteAttribute("aggregationStrategyMethodAllowNull", def.getAggregationStrategyMethodAllowNull());
doWriteExpressionNodeElements(def);
endElement(name);
}
protected void doWriteErrorHandlerDefinition(
String name,
ErrorHandlerDefinition def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteElement(null, def.getErrorHandlerType(), (n, v) -> {
switch (v.getClass().getSimpleName()) {
case "DeadLetterChannelDefinition" -> doWriteDeadLetterChannelDefinition("deadLetterChannel", (DeadLetterChannelDefinition) def.getErrorHandlerType());
case "DefaultErrorHandlerDefinition" -> doWriteDefaultErrorHandlerDefinition("defaultErrorHandler", (DefaultErrorHandlerDefinition) def.getErrorHandlerType());
case "NoErrorHandlerDefinition" -> doWriteNoErrorHandlerDefinition("noErrorHandler", (NoErrorHandlerDefinition) def.getErrorHandlerType());
case "JtaTransactionErrorHandlerDefinition" -> doWriteJtaTransactionErrorHandlerDefinition("jtaTransactionErrorHandler", (JtaTransactionErrorHandlerDefinition) def.getErrorHandlerType());
case "SpringTransactionErrorHandlerDefinition" -> doWriteSpringTransactionErrorHandlerDefinition("springTransactionErrorHandler", (SpringTransactionErrorHandlerDefinition) def.getErrorHandlerType());
}
});
endElement(name);
}
protected void doWriteExpressionNodeAttributes(
ExpressionNode def)
throws IOException {
doWriteProcessorDefinitionAttributes(def);
}
protected void doWriteExpressionNodeElements(
ExpressionNode def)
throws IOException {
doWriteElement(null, def.getExpression(), this::doWriteExpressionDefinitionRef);
}
protected void doWriteExpressionNode(
String name,
ExpressionNode def)
throws IOException {
startElement(name);
doWriteExpressionNodeAttributes(def);
doWriteExpressionNodeElements(def);
endElement(name);
}
protected void doWriteExpressionSubElementDefinition(
String name,
ExpressionSubElementDefinition def)
throws IOException {
startExpressionElement(name);
doWriteElement(null, def.getExpressionType(), this::doWriteExpressionDefinitionRef);
endExpressionElement(name);
}
protected void doWriteFaultToleranceConfigurationCommonAttributes(
FaultToleranceConfigurationCommon def)
throws IOException {
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("failureRatio", def.getFailureRatio());
doWriteAttribute("timeoutScheduledExecutorService", def.getTimeoutScheduledExecutorService());
doWriteAttribute("timeoutDuration", def.getTimeoutDuration());
doWriteAttribute("timeoutEnabled", def.getTimeoutEnabled());
doWriteAttribute("timeoutPoolSize", def.getTimeoutPoolSize());
doWriteAttribute("successThreshold", def.getSuccessThreshold());
doWriteAttribute("requestVolumeThreshold", def.getRequestVolumeThreshold());
doWriteAttribute("bulkheadExecutorService", def.getBulkheadExecutorService());
doWriteAttribute("delay", def.getDelay());
doWriteAttribute("bulkheadWaitingTaskQueue", def.getBulkheadWaitingTaskQueue());
doWriteAttribute("circuitBreaker", def.getCircuitBreaker());
doWriteAttribute("bulkheadMaxConcurrentCalls", def.getBulkheadMaxConcurrentCalls());
doWriteAttribute("bulkheadEnabled", def.getBulkheadEnabled());
}
protected void doWriteFaultToleranceConfigurationCommon(
String name,
FaultToleranceConfigurationCommon def)
throws IOException {
startElement(name);
doWriteFaultToleranceConfigurationCommonAttributes(def);
endElement(name);
}
protected void doWriteFaultToleranceConfigurationDefinition(
String name,
FaultToleranceConfigurationDefinition def)
throws IOException {
startElement(name);
doWriteFaultToleranceConfigurationCommonAttributes(def);
endElement(name);
}
protected void doWriteFilterDefinition(
String name,
FilterDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("statusPropertyName", def.getStatusPropertyName());
doWriteOutputExpressionNodeElements(def);
endElement(name);
}
protected void doWriteFinallyDefinition(
String name,
FinallyDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteList(null, null, def.getOutputs(), this::doWriteProcessorDefinitionRef);
endElement(name);
}
protected void doWriteFromDefinition(
String name,
FromDefinition def)
throws IOException {
startElement(name);
doWriteOptionalIdentifiedDefinitionAttributes(def);
doWriteAttribute("variableReceive", def.getVariableReceive());
doWriteAttribute("uri", def.getUri());
endElement(name);
}
protected void doWriteGlobalOptionDefinition(
String name,
GlobalOptionDefinition def)
throws IOException {
startElement(name);
doWriteAttribute("value", def.getValue());
doWriteAttribute("key", def.getKey());
endElement(name);
}
protected void doWriteGlobalOptionsDefinition(
String name,
GlobalOptionsDefinition def)
throws IOException {
startElement(name);
doWriteList(null, "globalOption", def.getGlobalOptions(), this::doWriteGlobalOptionDefinition);
endElement(name);
}
protected void doWriteIdempotentConsumerDefinition(
String name,
IdempotentConsumerDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("completionEager", def.getCompletionEager());
doWriteAttribute("skipDuplicate", def.getSkipDuplicate());
doWriteAttribute("eager", def.getEager());
doWriteAttribute("idempotentRepository", def.getIdempotentRepository());
doWriteAttribute("removeOnFailure", def.getRemoveOnFailure());
doWriteOutputExpressionNodeElements(def);
endElement(name);
}
protected void doWriteIdentifiedTypeAttributes(
IdentifiedType def)
throws IOException {
doWriteAttribute("id", def.getId());
}
protected void doWriteIdentifiedType(
String name,
IdentifiedType def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
endElement(name);
}
protected void doWriteInputTypeDefinition(
String name,
InputTypeDefinition def)
throws IOException {
startElement(name);
doWriteOptionalIdentifiedDefinitionAttributes(def);
doWriteAttribute("urn", def.getUrn());
doWriteAttribute("validate", def.getValidate());
endElement(name);
}
protected void doWriteInterceptDefinitionAttributes(
InterceptDefinition def)
throws IOException {
doWriteProcessorDefinitionAttributes(def);
}
protected void doWriteInterceptDefinitionElements(
InterceptDefinition def)
throws IOException {
doWriteList(null, null, def.getOutputs(), this::doWriteProcessorDefinitionRef);
}
protected void doWriteInterceptDefinition(
String name,
InterceptDefinition def)
throws IOException {
startElement(name);
doWriteInterceptDefinitionAttributes(def);
doWriteInterceptDefinitionElements(def);
endElement(name);
}
protected void doWriteInterceptFromDefinition(
String name,
InterceptFromDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("uri", def.getUri());
doWriteInterceptDefinitionElements(def);
endElement(name);
}
protected void doWriteInterceptSendToEndpointDefinition(
String name,
InterceptSendToEndpointDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("afterUri", def.getAfterUri());
doWriteAttribute("uri", def.getUri());
doWriteAttribute("skipSendToOriginalEndpoint", def.getSkipSendToOriginalEndpoint());
doWriteList(null, null, def.getOutputs(), this::doWriteProcessorDefinitionRef);
endElement(name);
}
protected void doWriteKameletDefinition(
String name,
KameletDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("name", def.getName());
doWriteList(null, null, def.getOutputs(), this::doWriteProcessorDefinitionRef);
endElement(name);
}
protected void doWriteLoadBalanceDefinition(
String name,
LoadBalanceDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteElement(null, def.getLoadBalancerType(), (n, v) -> {
switch (v.getClass().getSimpleName()) {
case "CustomLoadBalancerDefinition" -> doWriteCustomLoadBalancerDefinition("customLoadBalancer", (CustomLoadBalancerDefinition) def.getLoadBalancerType());
case "FailoverLoadBalancerDefinition" -> doWriteFailoverLoadBalancerDefinition("failover", (FailoverLoadBalancerDefinition) def.getLoadBalancerType());
case "RandomLoadBalancerDefinition" -> doWriteRandomLoadBalancerDefinition("random", (RandomLoadBalancerDefinition) def.getLoadBalancerType());
case "RoundRobinLoadBalancerDefinition" -> doWriteRoundRobinLoadBalancerDefinition("roundRobin", (RoundRobinLoadBalancerDefinition) def.getLoadBalancerType());
case "StickyLoadBalancerDefinition" -> doWriteStickyLoadBalancerDefinition("sticky", (StickyLoadBalancerDefinition) def.getLoadBalancerType());
case "TopicLoadBalancerDefinition" -> doWriteTopicLoadBalancerDefinition("topic", (TopicLoadBalancerDefinition) def.getLoadBalancerType());
case "WeightedLoadBalancerDefinition" -> doWriteWeightedLoadBalancerDefinition("weighted", (WeightedLoadBalancerDefinition) def.getLoadBalancerType());
}
});
doWriteList(null, null, def.getOutputs(), this::doWriteProcessorDefinitionRef);
endElement(name);
}
protected void doWriteLoadBalancerDefinitionAttributes(
LoadBalancerDefinition def)
throws IOException {
doWriteIdentifiedTypeAttributes(def);
}
protected void doWriteLoadBalancerDefinition(
String name,
LoadBalancerDefinition def)
throws IOException {
startElement(name);
doWriteLoadBalancerDefinitionAttributes(def);
endElement(name);
}
protected void doWriteLogDefinition(
String name,
LogDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("logName", def.getLogName());
doWriteAttribute("marker", def.getMarker());
doWriteAttribute("logger", def.getLogger());
doWriteAttribute("message", def.getMessage());
doWriteAttribute("loggingLevel", def.getLoggingLevel());
endElement(name);
}
protected void doWriteLoopDefinition(
String name,
LoopDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("doWhile", def.getDoWhile());
doWriteAttribute("breakOnShutdown", def.getBreakOnShutdown());
doWriteAttribute("copy", def.getCopy());
doWriteOutputExpressionNodeElements(def);
endElement(name);
}
protected void doWriteMarshalDefinition(
String name,
MarshalDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("variableReceive", def.getVariableReceive());
doWriteAttribute("variableSend", def.getVariableSend());
doWriteElement(null, def.getDataFormatType(), (n, v) -> {
switch (v.getClass().getSimpleName()) {
case "ASN1DataFormat" -> doWriteASN1DataFormat("asn1", (ASN1DataFormat) def.getDataFormatType());
case "AvroDataFormat" -> doWriteAvroDataFormat("avro", (AvroDataFormat) def.getDataFormatType());
case "BarcodeDataFormat" -> doWriteBarcodeDataFormat("barcode", (BarcodeDataFormat) def.getDataFormatType());
case "Base64DataFormat" -> doWriteBase64DataFormat("base64", (Base64DataFormat) def.getDataFormatType());
case "BeanioDataFormat" -> doWriteBeanioDataFormat("beanio", (BeanioDataFormat) def.getDataFormatType());
case "BindyDataFormat" -> doWriteBindyDataFormat("bindy", (BindyDataFormat) def.getDataFormatType());
case "CBORDataFormat" -> doWriteCBORDataFormat("cbor", (CBORDataFormat) def.getDataFormatType());
case "CryptoDataFormat" -> doWriteCryptoDataFormat("crypto", (CryptoDataFormat) def.getDataFormatType());
case "CsvDataFormat" -> doWriteCsvDataFormat("csv", (CsvDataFormat) def.getDataFormatType());
case "CustomDataFormat" -> doWriteCustomDataFormat("custom", (CustomDataFormat) def.getDataFormatType());
case "FhirJsonDataFormat" -> doWriteFhirJsonDataFormat("fhirJson", (FhirJsonDataFormat) def.getDataFormatType());
case "FhirXmlDataFormat" -> doWriteFhirXmlDataFormat("fhirXml", (FhirXmlDataFormat) def.getDataFormatType());
case "FlatpackDataFormat" -> doWriteFlatpackDataFormat("flatpack", (FlatpackDataFormat) def.getDataFormatType());
case "GrokDataFormat" -> doWriteGrokDataFormat("grok", (GrokDataFormat) def.getDataFormatType());
case "GzipDeflaterDataFormat" -> doWriteGzipDeflaterDataFormat("gzipDeflater", (GzipDeflaterDataFormat) def.getDataFormatType());
case "HL7DataFormat" -> doWriteHL7DataFormat("hl7", (HL7DataFormat) def.getDataFormatType());
case "IcalDataFormat" -> doWriteIcalDataFormat("ical", (IcalDataFormat) def.getDataFormatType());
case "JacksonXMLDataFormat" -> doWriteJacksonXMLDataFormat("jacksonXml", (JacksonXMLDataFormat) def.getDataFormatType());
case "JaxbDataFormat" -> doWriteJaxbDataFormat("jaxb", (JaxbDataFormat) def.getDataFormatType());
case "JsonDataFormat" -> doWriteJsonDataFormat("json", (JsonDataFormat) def.getDataFormatType());
case "JsonApiDataFormat" -> doWriteJsonApiDataFormat("jsonApi", (JsonApiDataFormat) def.getDataFormatType());
case "LZFDataFormat" -> doWriteLZFDataFormat("lzf", (LZFDataFormat) def.getDataFormatType());
case "MimeMultipartDataFormat" -> doWriteMimeMultipartDataFormat("mimeMultipart", (MimeMultipartDataFormat) def.getDataFormatType());
case "ParquetAvroDataFormat" -> doWriteParquetAvroDataFormat("parquetAvro", (ParquetAvroDataFormat) def.getDataFormatType());
case "ProtobufDataFormat" -> doWriteProtobufDataFormat("protobuf", (ProtobufDataFormat) def.getDataFormatType());
case "RssDataFormat" -> doWriteRssDataFormat("rss", (RssDataFormat) def.getDataFormatType());
case "SoapDataFormat" -> doWriteSoapDataFormat("soap", (SoapDataFormat) def.getDataFormatType());
case "SwiftMtDataFormat" -> doWriteSwiftMtDataFormat("swiftMt", (SwiftMtDataFormat) def.getDataFormatType());
case "SwiftMxDataFormat" -> doWriteSwiftMxDataFormat("swiftMx", (SwiftMxDataFormat) def.getDataFormatType());
case "SyslogDataFormat" -> doWriteSyslogDataFormat("syslog", (SyslogDataFormat) def.getDataFormatType());
case "TarFileDataFormat" -> doWriteTarFileDataFormat("tarFile", (TarFileDataFormat) def.getDataFormatType());
case "ThriftDataFormat" -> doWriteThriftDataFormat("thrift", (ThriftDataFormat) def.getDataFormatType());
case "TidyMarkupDataFormat" -> doWriteTidyMarkupDataFormat("tidyMarkup", (TidyMarkupDataFormat) def.getDataFormatType());
case "UniVocityCsvDataFormat" -> doWriteUniVocityCsvDataFormat("univocityCsv", (UniVocityCsvDataFormat) def.getDataFormatType());
case "UniVocityFixedDataFormat" -> doWriteUniVocityFixedDataFormat("univocityFixed", (UniVocityFixedDataFormat) def.getDataFormatType());
case "UniVocityTsvDataFormat" -> doWriteUniVocityTsvDataFormat("univocityTsv", (UniVocityTsvDataFormat) def.getDataFormatType());
case "XMLSecurityDataFormat" -> doWriteXMLSecurityDataFormat("xmlSecurity", (XMLSecurityDataFormat) def.getDataFormatType());
case "PGPDataFormat" -> doWritePGPDataFormat("pgp", (PGPDataFormat) def.getDataFormatType());
case "YAMLDataFormat" -> doWriteYAMLDataFormat("yaml", (YAMLDataFormat) def.getDataFormatType());
case "ZipDeflaterDataFormat" -> doWriteZipDeflaterDataFormat("zipDeflater", (ZipDeflaterDataFormat) def.getDataFormatType());
case "ZipFileDataFormat" -> doWriteZipFileDataFormat("zipFile", (ZipFileDataFormat) def.getDataFormatType());
}
});
endElement(name);
}
protected void doWriteMulticastDefinition(
String name,
MulticastDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("aggregationStrategy", def.getAggregationStrategy());
doWriteAttribute("onPrepare", def.getOnPrepare());
doWriteAttribute("executorService", def.getExecutorService());
doWriteAttribute("synchronous", def.getSynchronous());
doWriteAttribute("aggregationStrategyMethodName", def.getAggregationStrategyMethodName());
doWriteAttribute("timeout", def.getTimeout());
doWriteAttribute("streaming", def.getStreaming());
doWriteAttribute("stopOnException", def.getStopOnException());
doWriteAttribute("parallelProcessing", def.getParallelProcessing());
doWriteAttribute("parallelAggregate", def.getParallelAggregate());
doWriteAttribute("shareUnitOfWork", def.getShareUnitOfWork());
doWriteAttribute("aggregationStrategyMethodAllowNull", def.getAggregationStrategyMethodAllowNull());
doWriteList(null, null, def.getOutputs(), this::doWriteProcessorDefinitionRef);
endElement(name);
}
protected void doWriteOnCompletionDefinition(
String name,
OnCompletionDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("parallelProcessing", def.getParallelProcessing());
doWriteAttribute("useOriginalMessage", def.getUseOriginalMessage());
doWriteAttribute("mode", def.getMode());
doWriteAttribute("onCompleteOnly", def.getOnCompleteOnly());
doWriteAttribute("executorService", def.getExecutorService());
doWriteAttribute("onFailureOnly", def.getOnFailureOnly());
doWriteElement("onWhen", def.getOnWhen(), this::doWriteWhenDefinition);
doWriteList(null, null, def.getOutputs(), this::doWriteProcessorDefinitionRef);
endElement(name);
}
protected void doWriteOnExceptionDefinition(
String name,
OnExceptionDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("useOriginalMessage", def.getUseOriginalMessage());
doWriteAttribute("onRedeliveryRef", def.getOnRedeliveryRef());
doWriteAttribute("useOriginalBody", def.getUseOriginalBody());
doWriteAttribute("onExceptionOccurredRef", def.getOnExceptionOccurredRef());
doWriteAttribute("redeliveryPolicyRef", def.getRedeliveryPolicyRef());
doWriteElement("continued", def.getContinued(), this::doWriteExpressionSubElementDefinition);
doWriteList(null, "exception", def.getExceptions(), this::doWriteString);
doWriteElement("retryWhile", def.getRetryWhile(), this::doWriteExpressionSubElementDefinition);
doWriteElement("redeliveryPolicy", def.getRedeliveryPolicyType(), this::doWriteRedeliveryPolicyDefinition);
doWriteElement("handled", def.getHandled(), this::doWriteExpressionSubElementDefinition);
doWriteElement("onWhen", def.getOnWhen(), this::doWriteWhenDefinition);
doWriteList(null, null, def.getOutputs(), this::doWriteProcessorDefinitionRef);
endElement(name);
}
protected void doWriteOnFallbackDefinition(
String name,
OnFallbackDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("fallbackViaNetwork", def.getFallbackViaNetwork());
doWriteList(null, null, def.getOutputs(), this::doWriteProcessorDefinitionRef);
endElement(name);
}
protected void doWriteOptimisticLockRetryPolicyDefinition(
String name,
OptimisticLockRetryPolicyDefinition def)
throws IOException {
startElement(name);
doWriteAttribute("retryDelay", def.getRetryDelay());
doWriteAttribute("randomBackOff", def.getRandomBackOff());
doWriteAttribute("maximumRetries", def.getMaximumRetries());
doWriteAttribute("exponentialBackOff", def.getExponentialBackOff());
doWriteAttribute("maximumRetryDelay", def.getMaximumRetryDelay());
endElement(name);
}
protected void doWriteOptionalIdentifiedDefinitionAttributes(
OptionalIdentifiedDefinition> def)
throws IOException {
doWriteAttribute("description", def.getDescription());
doWriteAttribute("id", def.getId());
doWriteAttribute("customId", toString(def.getCustomId()));
}
protected void doWriteOptionalIdentifiedDefinition(
String name,
OptionalIdentifiedDefinition> def)
throws IOException {
startElement(name);
doWriteOptionalIdentifiedDefinitionAttributes(def);
endElement(name);
}
protected void doWriteOtherwiseDefinition(
String name,
OtherwiseDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteList(null, null, def.getOutputs(), this::doWriteProcessorDefinitionRef);
endElement(name);
}
protected void doWriteOutputExpressionNodeAttributes(
OutputExpressionNode def)
throws IOException {
doWriteProcessorDefinitionAttributes(def);
}
protected void doWriteOutputExpressionNodeElements(
OutputExpressionNode def)
throws IOException {
doWriteExpressionNodeElements(def);
doWriteList(null, null, def.getOutputs(), this::doWriteProcessorDefinitionRef);
}
protected void doWriteOutputExpressionNode(
String name,
OutputExpressionNode def)
throws IOException {
startElement(name);
doWriteOutputExpressionNodeAttributes(def);
doWriteOutputExpressionNodeElements(def);
endElement(name);
}
protected void doWriteOutputTypeDefinition(
String name,
OutputTypeDefinition def)
throws IOException {
startElement(name);
doWriteOptionalIdentifiedDefinitionAttributes(def);
doWriteAttribute("urn", def.getUrn());
doWriteAttribute("validate", def.getValidate());
endElement(name);
}
protected void doWritePackageScanDefinition(
String name,
PackageScanDefinition def)
throws IOException {
startElement(name);
doWriteList(null, "excludes", def.getExcludes(), this::doWriteString);
doWriteList(null, "includes", def.getIncludes(), this::doWriteString);
doWriteList(null, "package", def.getPackages(), this::doWriteString);
endElement(name);
}
protected void doWritePausableDefinition(
String name,
PausableDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("untilCheck", def.getUntilCheck());
doWriteAttribute("consumerListener", def.getConsumerListener());
endElement(name);
}
protected void doWritePipelineDefinition(
String name,
PipelineDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteList(null, null, def.getOutputs(), this::doWriteProcessorDefinitionRef);
endElement(name);
}
protected void doWritePolicyDefinition(
String name,
PolicyDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("ref", def.getRef());
doWriteList(null, null, def.getOutputs(), this::doWriteProcessorDefinitionRef);
endElement(name);
}
protected void doWritePollEnrichDefinition(
String name,
PollEnrichDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("variableReceive", def.getVariableReceive());
doWriteAttribute("cacheSize", def.getCacheSize());
doWriteAttribute("aggregationStrategy", def.getAggregationStrategy());
doWriteAttribute("ignoreInvalidEndpoint", def.getIgnoreInvalidEndpoint());
doWriteAttribute("autoStartComponents", def.getAutoStartComponents());
doWriteAttribute("aggregateOnException", def.getAggregateOnException());
doWriteAttribute("aggregationStrategyMethodName", def.getAggregationStrategyMethodName());
doWriteAttribute("timeout", def.getTimeout());
doWriteAttribute("aggregationStrategyMethodAllowNull", def.getAggregationStrategyMethodAllowNull());
doWriteExpressionNodeElements(def);
endElement(name);
}
protected void doWriteProcessDefinition(
String name,
ProcessDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("ref", def.getRef());
endElement(name);
}
protected void doWriteProcessorDefinitionAttributes(
ProcessorDefinition> def)
throws IOException {
doWriteOptionalIdentifiedDefinitionAttributes(def);
doWriteAttribute("inheritErrorHandler", toString(def.isInheritErrorHandler()));
doWriteAttribute("disabled", def.getDisabled());
}
protected void doWriteProcessorDefinition(
String name,
ProcessorDefinition> def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
endElement(name);
}
protected void doWritePropertyDefinition(
String name,
PropertyDefinition def)
throws IOException {
startElement(name);
doWriteAttribute("value", def.getValue());
doWriteAttribute("key", def.getKey());
endElement(name);
}
protected void doWritePropertyDefinitions(
String name,
PropertyDefinitions def)
throws IOException {
startElement(name);
doWriteList(null, "property", def.getProperties(), this::doWritePropertyDefinition);
endElement(name);
}
protected void doWritePropertyExpressionDefinition(
String name,
PropertyExpressionDefinition def)
throws IOException {
startElement(name);
doWriteAttribute("key", def.getKey());
doWriteElement(null, def.getExpression(), this::doWriteExpressionDefinitionRef);
endElement(name);
}
protected void doWriteRecipientListDefinition(
String name,
RecipientListDefinition> def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("cacheSize", def.getCacheSize());
doWriteAttribute("aggregationStrategy", def.getAggregationStrategy());
doWriteAttribute("onPrepare", def.getOnPrepare());
doWriteAttribute("executorService", def.getExecutorService());
doWriteAttribute("synchronous", def.getSynchronous());
doWriteAttribute("aggregationStrategyMethodName", def.getAggregationStrategyMethodName());
doWriteAttribute("timeout", def.getTimeout());
doWriteAttribute("ignoreInvalidEndpoints", def.getIgnoreInvalidEndpoints());
doWriteAttribute("streaming", def.getStreaming());
doWriteAttribute("stopOnException", def.getStopOnException());
doWriteAttribute("delimiter", def.getDelimiter());
doWriteAttribute("parallelProcessing", def.getParallelProcessing());
doWriteAttribute("parallelAggregate", def.getParallelAggregate());
doWriteAttribute("shareUnitOfWork", def.getShareUnitOfWork());
doWriteAttribute("aggregationStrategyMethodAllowNull", def.getAggregationStrategyMethodAllowNull());
doWriteExpressionNodeElements(def);
endElement(name);
}
protected void doWriteRedeliveryPolicyDefinition(
String name,
RedeliveryPolicyDefinition def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("logNewException", def.getLogNewException());
doWriteAttribute("backOffMultiplier", def.getBackOffMultiplier());
doWriteAttribute("exchangeFormatterRef", def.getExchangeFormatterRef());
doWriteAttribute("allowRedeliveryWhileStopping", def.getAllowRedeliveryWhileStopping());
doWriteAttribute("delayPattern", def.getDelayPattern());
doWriteAttribute("retriesExhaustedLogLevel", def.getRetriesExhaustedLogLevel());
doWriteAttribute("logStackTrace", def.getLogStackTrace());
doWriteAttribute("retryAttemptedLogInterval", def.getRetryAttemptedLogInterval());
doWriteAttribute("logRetryAttempted", def.getLogRetryAttempted());
doWriteAttribute("maximumRedeliveryDelay", def.getMaximumRedeliveryDelay());
doWriteAttribute("useExponentialBackOff", def.getUseExponentialBackOff());
doWriteAttribute("logExhaustedMessageHistory", def.getLogExhaustedMessageHistory());
doWriteAttribute("collisionAvoidanceFactor", def.getCollisionAvoidanceFactor());
doWriteAttribute("logRetryStackTrace", def.getLogRetryStackTrace());
doWriteAttribute("asyncDelayedRedelivery", def.getAsyncDelayedRedelivery());
doWriteAttribute("disableRedelivery", def.getDisableRedelivery());
doWriteAttribute("logContinued", def.getLogContinued());
doWriteAttribute("retryAttemptedLogLevel", def.getRetryAttemptedLogLevel());
doWriteAttribute("redeliveryDelay", def.getRedeliveryDelay());
doWriteAttribute("logExhaustedMessageBody", def.getLogExhaustedMessageBody());
doWriteAttribute("logHandled", def.getLogHandled());
doWriteAttribute("maximumRedeliveries", def.getMaximumRedeliveries());
doWriteAttribute("logExhausted", def.getLogExhausted());
doWriteAttribute("useCollisionAvoidance", def.getUseCollisionAvoidance());
endElement(name);
}
protected void doWriteRemoveHeaderDefinition(
String name,
RemoveHeaderDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("name", def.getName());
endElement(name);
}
protected void doWriteRemoveHeadersDefinition(
String name,
RemoveHeadersDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("excludePattern", def.getExcludePattern());
doWriteAttribute("pattern", def.getPattern());
endElement(name);
}
protected void doWriteRemovePropertiesDefinition(
String name,
RemovePropertiesDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("excludePattern", def.getExcludePattern());
doWriteAttribute("pattern", def.getPattern());
endElement(name);
}
protected void doWriteRemovePropertyDefinition(
String name,
RemovePropertyDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("name", def.getName());
endElement(name);
}
protected void doWriteRemoveVariableDefinition(
String name,
RemoveVariableDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("name", def.getName());
endElement(name);
}
protected void doWriteResequenceDefinition(
String name,
ResequenceDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteElement(null, def.getExpression(), this::doWriteExpressionDefinitionRef);
doWriteElement(null, def.getResequencerConfig(), (n, v) -> {
switch (v.getClass().getSimpleName()) {
case "BatchResequencerConfig" -> doWriteBatchResequencerConfig("batchConfig", (BatchResequencerConfig) def.getResequencerConfig());
case "StreamResequencerConfig" -> doWriteStreamResequencerConfig("streamConfig", (StreamResequencerConfig) def.getResequencerConfig());
}
});
doWriteList(null, null, def.getOutputs(), this::doWriteProcessorDefinitionRef);
endElement(name);
}
protected void doWriteResilience4jConfigurationCommonAttributes(
Resilience4jConfigurationCommon def)
throws IOException {
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("failureRateThreshold", def.getFailureRateThreshold());
doWriteAttribute("bulkheadMaxWaitDuration", def.getBulkheadMaxWaitDuration());
doWriteAttribute("slowCallDurationThreshold", def.getSlowCallDurationThreshold());
doWriteAttribute("timeoutCancelRunningFuture", def.getTimeoutCancelRunningFuture());
doWriteAttribute("minimumNumberOfCalls", def.getMinimumNumberOfCalls());
doWriteAttribute("timeoutDuration", def.getTimeoutDuration());
doWriteAttribute("timeoutEnabled", def.getTimeoutEnabled());
doWriteAttribute("timeoutExecutorService", def.getTimeoutExecutorService());
doWriteAttribute("permittedNumberOfCallsInHalfOpenState", def.getPermittedNumberOfCallsInHalfOpenState());
doWriteAttribute("throwExceptionWhenHalfOpenOrOpenState", def.getThrowExceptionWhenHalfOpenOrOpenState());
doWriteAttribute("slowCallRateThreshold", def.getSlowCallRateThreshold());
doWriteAttribute("writableStackTraceEnabled", def.getWritableStackTraceEnabled());
doWriteAttribute("automaticTransitionFromOpenToHalfOpenEnabled", def.getAutomaticTransitionFromOpenToHalfOpenEnabled());
doWriteAttribute("circuitBreaker", def.getCircuitBreaker());
doWriteAttribute("slidingWindowSize", def.getSlidingWindowSize());
doWriteAttribute("config", def.getConfig());
doWriteAttribute("bulkheadMaxConcurrentCalls", def.getBulkheadMaxConcurrentCalls());
doWriteAttribute("slidingWindowType", def.getSlidingWindowType());
doWriteAttribute("bulkheadEnabled", def.getBulkheadEnabled());
doWriteAttribute("waitDurationInOpenState", def.getWaitDurationInOpenState());
}
protected void doWriteResilience4jConfigurationCommon(
String name,
Resilience4jConfigurationCommon def)
throws IOException {
startElement(name);
doWriteResilience4jConfigurationCommonAttributes(def);
endElement(name);
}
protected void doWriteResilience4jConfigurationDefinition(
String name,
Resilience4jConfigurationDefinition def)
throws IOException {
startElement(name);
doWriteResilience4jConfigurationCommonAttributes(def);
endElement(name);
}
protected void doWriteRestContextRefDefinition(
String name,
RestContextRefDefinition def)
throws IOException {
startElement(name);
doWriteAttribute("ref", def.getRef());
endElement(name);
}
protected void doWriteResumableDefinition(
String name,
ResumableDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("resumeStrategy", def.getResumeStrategy());
doWriteAttribute("intermittent", def.getIntermittent());
doWriteAttribute("loggingLevel", def.getLoggingLevel());
endElement(name);
}
protected void doWriteRollbackDefinition(
String name,
RollbackDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("markRollbackOnly", def.getMarkRollbackOnly());
doWriteAttribute("message", def.getMessage());
doWriteAttribute("markRollbackOnlyLast", def.getMarkRollbackOnlyLast());
endElement(name);
}
protected void doWriteRouteBuilderDefinition(
String name,
RouteBuilderDefinition def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("ref", def.getRef());
endElement(name);
}
protected void doWriteRouteConfigurationContextRefDefinition(
String name,
RouteConfigurationContextRefDefinition def)
throws IOException {
startElement(name);
doWriteAttribute("ref", def.getRef());
endElement(name);
}
protected void doWriteRouteConfigurationDefinition(
String name,
RouteConfigurationDefinition def)
throws IOException {
startElement(name);
doWriteOptionalIdentifiedDefinitionAttributes(def);
doWriteAttribute("precondition", def.getPrecondition());
doWriteList(null, "onException", def.getOnExceptions(), this::doWriteOnExceptionDefinition);
doWriteList(null, "onCompletion", def.getOnCompletions(), this::doWriteOnCompletionDefinition);
doWriteList(null, "interceptSendToEndpoint", def.getInterceptSendTos(), this::doWriteInterceptSendToEndpointDefinition);
doWriteElement("errorHandler", def.getErrorHandler(), this::doWriteErrorHandlerDefinition);
doWriteList(null, "interceptFrom", def.getInterceptFroms(), this::doWriteInterceptFromDefinition);
doWriteList(null, "intercept", def.getIntercepts(), this::doWriteInterceptDefinition);
endElement(name);
}
protected void doWriteRouteConfigurationsDefinition(
String name,
RouteConfigurationsDefinition def)
throws IOException {
startElement(name);
doWriteOptionalIdentifiedDefinitionAttributes(def);
doWriteList(null, null, def.getRouteConfigurations(), this::doWriteRouteConfigurationDefinitionRef);
endElement(name);
}
protected void doWriteRouteContextRefDefinition(
String name,
RouteContextRefDefinition def)
throws IOException {
startElement(name);
doWriteAttribute("ref", def.getRef());
endElement(name);
}
protected void doWriteRouteDefinition(
String name,
RouteDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("template", toString(def.isTemplate()));
doWriteAttribute("routeConfigurationId", def.getRouteConfigurationId());
doWriteAttribute("streamCache", def.getStreamCache());
doWriteAttribute("trace", def.getTrace());
doWriteAttribute("rest", toString(def.isRest()));
doWriteAttribute("routePolicyRef", def.getRoutePolicyRef());
doWriteAttribute("precondition", def.getPrecondition());
doWriteAttribute("shutdownRoute", def.getShutdownRoute());
doWriteAttribute("shutdownRunningTask", def.getShutdownRunningTask());
doWriteAttribute("startupOrder", toString(def.getStartupOrder()));
doWriteAttribute("logMask", def.getLogMask());
doWriteAttribute("nodePrefixId", def.getNodePrefixId());
doWriteAttribute("messageHistory", def.getMessageHistory());
doWriteAttribute("autoStartup", def.getAutoStartup());
doWriteAttribute("delayer", def.getDelayer());
doWriteAttribute("group", def.getGroup());
doWriteAttribute("errorHandlerRef", def.getErrorHandlerRef());
doWriteList(null, "routeProperty", def.getRouteProperties(), this::doWritePropertyDefinition);
doWriteElement(null, def.getInput(), this::doWriteFromDefinitionRef);
doWriteElement(null, def.getInputType(), this::doWriteInputTypeDefinitionRef);
doWriteElement(null, def.getOutputType(), this::doWriteOutputTypeDefinitionRef);
doWriteList(null, null, def.getOutputs(), this::doWriteProcessorDefinitionRef);
endElement(name);
}
protected void doWriteRouteTemplateBeanDefinition(
String name,
RouteTemplateBeanDefinition def)
throws IOException {
startElement(name);
doWriteBeanFactoryDefinitionAttributes(def);
doWriteBeanFactoryDefinitionElements(def);
endElement(name);
}
protected void doWriteRouteTemplateContextRefDefinition(
String name,
RouteTemplateContextRefDefinition def)
throws IOException {
startElement(name);
doWriteAttribute("ref", def.getRef());
endElement(name);
}
protected void doWriteRouteTemplateDefinition(
String name,
RouteTemplateDefinition def)
throws IOException {
startElement(name);
doWriteOptionalIdentifiedDefinitionAttributes(def);
doWriteList(null, "templateParameter", def.getTemplateParameters(), this::doWriteRouteTemplateParameterDefinition);
doWriteElement("route", def.getRoute(), this::doWriteRouteDefinition);
doWriteList(null, "templateBean", def.getTemplateBeans(), this::doWriteRouteTemplateBeanDefinition);
endElement(name);
}
protected void doWriteRouteTemplateParameterDefinition(
String name,
RouteTemplateParameterDefinition def)
throws IOException {
startElement(name);
doWriteAttribute("defaultValue", def.getDefaultValue());
doWriteAttribute("name", def.getName());
doWriteAttribute("description", def.getDescription());
doWriteAttribute("required", toString(def.getRequired()));
endElement(name);
}
protected void doWriteRouteTemplatesDefinition(
String name,
RouteTemplatesDefinition def)
throws IOException {
startElement(name);
doWriteOptionalIdentifiedDefinitionAttributes(def);
doWriteList(null, null, def.getRouteTemplates(), this::doWriteRouteTemplateDefinitionRef);
endElement(name);
}
protected void doWriteRoutesDefinition(
String name,
RoutesDefinition def)
throws IOException {
startElement(name);
doWriteOptionalIdentifiedDefinitionAttributes(def);
doWriteList(null, null, def.getRoutes(), this::doWriteRouteDefinitionRef);
endElement(name);
}
protected void doWriteRoutingSlipDefinition(
String name,
RoutingSlipDefinition> def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("uriDelimiter", def.getUriDelimiter());
doWriteAttribute("ignoreInvalidEndpoints", def.getIgnoreInvalidEndpoints());
doWriteAttribute("cacheSize", def.getCacheSize());
doWriteExpressionNodeElements(def);
endElement(name);
}
protected void doWriteSagaActionUriDefinition(
String name,
SagaActionUriDefinition def)
throws IOException {
startElement(name);
doWriteSendDefinitionAttributes(def);
endElement(name);
}
protected void doWriteSagaDefinition(
String name,
SagaDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("propagation", def.getPropagation());
doWriteAttribute("completionMode", def.getCompletionMode());
doWriteAttribute("sagaService", def.getSagaService());
doWriteAttribute("timeout", def.getTimeout());
doWriteElement("completion", def.getCompletion(), this::doWriteSagaActionUriDefinition);
doWriteList(null, "option", def.getOptions(), this::doWritePropertyExpressionDefinition);
doWriteElement("compensation", def.getCompensation(), this::doWriteSagaActionUriDefinition);
doWriteList(null, null, def.getOutputs(), this::doWriteProcessorDefinitionRef);
endElement(name);
}
protected void doWriteSamplingDefinition(
String name,
SamplingDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("messageFrequency", def.getMessageFrequency());
doWriteAttribute("samplePeriod", def.getSamplePeriod());
endElement(name);
}
protected void doWriteScriptDefinition(
String name,
ScriptDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteExpressionNodeElements(def);
endElement(name);
}
protected void doWriteSendDefinitionAttributes(
SendDefinition> def)
throws IOException {
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("uri", def.getUri());
}
protected void doWriteSendDefinition(
String name,
SendDefinition> def)
throws IOException {
startElement(name);
doWriteSendDefinitionAttributes(def);
endElement(name);
}
protected void doWriteSetBodyDefinition(
String name,
SetBodyDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteExpressionNodeElements(def);
endElement(name);
}
protected void doWriteSetExchangePatternDefinition(
String name,
SetExchangePatternDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("pattern", def.getPattern());
endElement(name);
}
protected void doWriteSetHeaderDefinition(
String name,
SetHeaderDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("name", def.getName());
doWriteExpressionNodeElements(def);
endElement(name);
}
protected void doWriteSetHeadersDefinition(
String name,
SetHeadersDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteList(null, null, def.getHeaders(), this::doWriteSetHeaderDefinitionRef);
endElement(name);
}
protected void doWriteSetPropertyDefinition(
String name,
SetPropertyDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("name", def.getName());
doWriteExpressionNodeElements(def);
endElement(name);
}
protected void doWriteSetVariableDefinition(
String name,
SetVariableDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("name", def.getName());
doWriteExpressionNodeElements(def);
endElement(name);
}
protected void doWriteSortDefinition(
String name,
SortDefinition> def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("comparator", def.getComparator());
doWriteExpressionNodeElements(def);
endElement(name);
}
protected void doWriteSplitDefinition(
String name,
SplitDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("aggregationStrategy", def.getAggregationStrategy());
doWriteAttribute("onPrepare", def.getOnPrepare());
doWriteAttribute("executorService", def.getExecutorService());
doWriteAttribute("synchronous", def.getSynchronous());
doWriteAttribute("aggregationStrategyMethodName", def.getAggregationStrategyMethodName());
doWriteAttribute("timeout", def.getTimeout());
doWriteAttribute("streaming", def.getStreaming());
doWriteAttribute("stopOnException", def.getStopOnException());
doWriteAttribute("delimiter", def.getDelimiter());
doWriteAttribute("parallelProcessing", def.getParallelProcessing());
doWriteAttribute("parallelAggregate", def.getParallelAggregate());
doWriteAttribute("shareUnitOfWork", def.getShareUnitOfWork());
doWriteAttribute("aggregationStrategyMethodAllowNull", def.getAggregationStrategyMethodAllowNull());
doWriteOutputExpressionNodeElements(def);
endElement(name);
}
protected void doWriteStepDefinition(
String name,
StepDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteList(null, null, def.getOutputs(), this::doWriteProcessorDefinitionRef);
endElement(name);
}
protected void doWriteStopDefinition(
String name,
StopDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
endElement(name);
}
protected void doWriteTemplatedRouteBeanDefinition(
String name,
TemplatedRouteBeanDefinition def)
throws IOException {
startElement(name);
doWriteBeanFactoryDefinitionAttributes(def);
doWriteBeanFactoryDefinitionElements(def);
endElement(name);
}
protected void doWriteTemplatedRouteDefinition(
String name,
TemplatedRouteDefinition def)
throws IOException {
startElement(name);
doWriteAttribute("routeId", def.getRouteId());
doWriteAttribute("routeTemplateRef", def.getRouteTemplateRef());
doWriteAttribute("prefixId", def.getPrefixId());
doWriteList(null, "bean", def.getBeans(), this::doWriteTemplatedRouteBeanDefinition);
doWriteList(null, "parameter", def.getParameters(), this::doWriteTemplatedRouteParameterDefinition);
endElement(name);
}
protected void doWriteTemplatedRouteParameterDefinition(
String name,
TemplatedRouteParameterDefinition def)
throws IOException {
startElement(name);
doWriteAttribute("name", def.getName());
doWriteAttribute("value", def.getValue());
endElement(name);
}
protected void doWriteTemplatedRoutesDefinition(
String name,
TemplatedRoutesDefinition def)
throws IOException {
startElement(name);
doWriteOptionalIdentifiedDefinitionAttributes(def);
doWriteList(null, null, def.getTemplatedRoutes(), this::doWriteTemplatedRouteDefinitionRef);
endElement(name);
}
protected void doWriteThreadPoolProfileDefinition(
String name,
ThreadPoolProfileDefinition def)
throws IOException {
startElement(name);
doWriteOptionalIdentifiedDefinitionAttributes(def);
doWriteAttribute("keepAliveTime", def.getKeepAliveTime());
doWriteAttribute("maxQueueSize", def.getMaxQueueSize());
doWriteAttribute("allowCoreThreadTimeOut", def.getAllowCoreThreadTimeOut());
doWriteAttribute("poolSize", def.getPoolSize());
doWriteAttribute("defaultProfile", def.getDefaultProfile());
doWriteAttribute("maxPoolSize", def.getMaxPoolSize());
doWriteAttribute("rejectedPolicy", def.getRejectedPolicy());
doWriteAttribute("timeUnit", def.getTimeUnit());
endElement(name);
}
protected void doWriteThreadsDefinition(
String name,
ThreadsDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("keepAliveTime", def.getKeepAliveTime());
doWriteAttribute("callerRunsWhenRejected", def.getCallerRunsWhenRejected());
doWriteAttribute("maxQueueSize", def.getMaxQueueSize());
doWriteAttribute("executorService", def.getExecutorService());
doWriteAttribute("allowCoreThreadTimeOut", def.getAllowCoreThreadTimeOut());
doWriteAttribute("poolSize", def.getPoolSize());
doWriteAttribute("maxPoolSize", def.getMaxPoolSize());
doWriteAttribute("threadName", def.getThreadName());
doWriteAttribute("rejectedPolicy", def.getRejectedPolicy());
doWriteAttribute("timeUnit", def.getTimeUnit());
endElement(name);
}
protected void doWriteThrottleDefinition(
String name,
ThrottleDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("mode", def.getMode());
doWriteAttribute("timePeriodMillis", def.getTimePeriodMillis());
doWriteAttribute("rejectExecution", def.getRejectExecution());
doWriteAttribute("callerRunsWhenRejected", def.getCallerRunsWhenRejected());
doWriteAttribute("executorService", def.getExecutorService());
doWriteAttribute("asyncDelayed", def.getAsyncDelayed());
doWriteExpressionNodeElements(def);
doWriteElement("correlationExpression", def.getCorrelationExpression(), this::doWriteExpressionSubElementDefinition);
endElement(name);
}
protected void doWriteThrowExceptionDefinition(
String name,
ThrowExceptionDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("exceptionType", def.getExceptionType());
doWriteAttribute("ref", def.getRef());
doWriteAttribute("message", def.getMessage());
endElement(name);
}
protected void doWriteToDefinition(
String name,
ToDefinition def)
throws IOException {
startElement(name);
doWriteSendDefinitionAttributes(def);
doWriteAttribute("variableReceive", def.getVariableReceive());
doWriteAttribute("variableSend", def.getVariableSend());
doWriteAttribute("pattern", def.getPattern());
endElement(name);
}
protected void doWriteToDynamicDefinitionAttributes(
ToDynamicDefinition def)
throws IOException {
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("variableReceive", def.getVariableReceive());
doWriteAttribute("variableSend", def.getVariableSend());
doWriteAttribute("cacheSize", def.getCacheSize());
doWriteAttribute("ignoreInvalidEndpoint", def.getIgnoreInvalidEndpoint());
doWriteAttribute("autoStartComponents", def.getAutoStartComponents());
doWriteAttribute("pattern", def.getPattern());
doWriteAttribute("allowOptimisedComponents", def.getAllowOptimisedComponents());
doWriteAttribute("uri", def.getUri());
}
protected void doWriteToDynamicDefinition(
String name,
ToDynamicDefinition def)
throws IOException {
startElement(name);
doWriteToDynamicDefinitionAttributes(def);
endElement(name);
}
protected void doWriteTransactedDefinition(
String name,
TransactedDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("ref", def.getRef());
doWriteList(null, null, def.getOutputs(), this::doWriteProcessorDefinitionRef);
endElement(name);
}
protected void doWriteTransformDefinition(
String name,
TransformDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("toType", def.getToType());
doWriteAttribute("fromType", def.getFromType());
doWriteExpressionNodeElements(def);
endElement(name);
}
protected void doWriteTryDefinition(
String name,
TryDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteList(null, null, def.getOutputs(), this::doWriteProcessorDefinitionRef);
endElement(name);
}
protected void doWriteUnmarshalDefinition(
String name,
UnmarshalDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("variableReceive", def.getVariableReceive());
doWriteAttribute("variableSend", def.getVariableSend());
doWriteAttribute("allowNullBody", def.getAllowNullBody());
doWriteElement(null, def.getDataFormatType(), (n, v) -> {
switch (v.getClass().getSimpleName()) {
case "ASN1DataFormat" -> doWriteASN1DataFormat("asn1", (ASN1DataFormat) def.getDataFormatType());
case "AvroDataFormat" -> doWriteAvroDataFormat("avro", (AvroDataFormat) def.getDataFormatType());
case "BarcodeDataFormat" -> doWriteBarcodeDataFormat("barcode", (BarcodeDataFormat) def.getDataFormatType());
case "Base64DataFormat" -> doWriteBase64DataFormat("base64", (Base64DataFormat) def.getDataFormatType());
case "BeanioDataFormat" -> doWriteBeanioDataFormat("beanio", (BeanioDataFormat) def.getDataFormatType());
case "BindyDataFormat" -> doWriteBindyDataFormat("bindy", (BindyDataFormat) def.getDataFormatType());
case "CBORDataFormat" -> doWriteCBORDataFormat("cbor", (CBORDataFormat) def.getDataFormatType());
case "CryptoDataFormat" -> doWriteCryptoDataFormat("crypto", (CryptoDataFormat) def.getDataFormatType());
case "CsvDataFormat" -> doWriteCsvDataFormat("csv", (CsvDataFormat) def.getDataFormatType());
case "CustomDataFormat" -> doWriteCustomDataFormat("custom", (CustomDataFormat) def.getDataFormatType());
case "FhirJsonDataFormat" -> doWriteFhirJsonDataFormat("fhirJson", (FhirJsonDataFormat) def.getDataFormatType());
case "FhirXmlDataFormat" -> doWriteFhirXmlDataFormat("fhirXml", (FhirXmlDataFormat) def.getDataFormatType());
case "FlatpackDataFormat" -> doWriteFlatpackDataFormat("flatpack", (FlatpackDataFormat) def.getDataFormatType());
case "GrokDataFormat" -> doWriteGrokDataFormat("grok", (GrokDataFormat) def.getDataFormatType());
case "GzipDeflaterDataFormat" -> doWriteGzipDeflaterDataFormat("gzipDeflater", (GzipDeflaterDataFormat) def.getDataFormatType());
case "HL7DataFormat" -> doWriteHL7DataFormat("hl7", (HL7DataFormat) def.getDataFormatType());
case "IcalDataFormat" -> doWriteIcalDataFormat("ical", (IcalDataFormat) def.getDataFormatType());
case "JacksonXMLDataFormat" -> doWriteJacksonXMLDataFormat("jacksonXml", (JacksonXMLDataFormat) def.getDataFormatType());
case "JaxbDataFormat" -> doWriteJaxbDataFormat("jaxb", (JaxbDataFormat) def.getDataFormatType());
case "JsonDataFormat" -> doWriteJsonDataFormat("json", (JsonDataFormat) def.getDataFormatType());
case "JsonApiDataFormat" -> doWriteJsonApiDataFormat("jsonApi", (JsonApiDataFormat) def.getDataFormatType());
case "LZFDataFormat" -> doWriteLZFDataFormat("lzf", (LZFDataFormat) def.getDataFormatType());
case "MimeMultipartDataFormat" -> doWriteMimeMultipartDataFormat("mimeMultipart", (MimeMultipartDataFormat) def.getDataFormatType());
case "ParquetAvroDataFormat" -> doWriteParquetAvroDataFormat("parquetAvro", (ParquetAvroDataFormat) def.getDataFormatType());
case "ProtobufDataFormat" -> doWriteProtobufDataFormat("protobuf", (ProtobufDataFormat) def.getDataFormatType());
case "RssDataFormat" -> doWriteRssDataFormat("rss", (RssDataFormat) def.getDataFormatType());
case "SoapDataFormat" -> doWriteSoapDataFormat("soap", (SoapDataFormat) def.getDataFormatType());
case "SwiftMtDataFormat" -> doWriteSwiftMtDataFormat("swiftMt", (SwiftMtDataFormat) def.getDataFormatType());
case "SwiftMxDataFormat" -> doWriteSwiftMxDataFormat("swiftMx", (SwiftMxDataFormat) def.getDataFormatType());
case "SyslogDataFormat" -> doWriteSyslogDataFormat("syslog", (SyslogDataFormat) def.getDataFormatType());
case "TarFileDataFormat" -> doWriteTarFileDataFormat("tarFile", (TarFileDataFormat) def.getDataFormatType());
case "ThriftDataFormat" -> doWriteThriftDataFormat("thrift", (ThriftDataFormat) def.getDataFormatType());
case "TidyMarkupDataFormat" -> doWriteTidyMarkupDataFormat("tidyMarkup", (TidyMarkupDataFormat) def.getDataFormatType());
case "UniVocityCsvDataFormat" -> doWriteUniVocityCsvDataFormat("univocityCsv", (UniVocityCsvDataFormat) def.getDataFormatType());
case "UniVocityFixedDataFormat" -> doWriteUniVocityFixedDataFormat("univocityFixed", (UniVocityFixedDataFormat) def.getDataFormatType());
case "UniVocityTsvDataFormat" -> doWriteUniVocityTsvDataFormat("univocityTsv", (UniVocityTsvDataFormat) def.getDataFormatType());
case "XMLSecurityDataFormat" -> doWriteXMLSecurityDataFormat("xmlSecurity", (XMLSecurityDataFormat) def.getDataFormatType());
case "PGPDataFormat" -> doWritePGPDataFormat("pgp", (PGPDataFormat) def.getDataFormatType());
case "YAMLDataFormat" -> doWriteYAMLDataFormat("yaml", (YAMLDataFormat) def.getDataFormatType());
case "ZipDeflaterDataFormat" -> doWriteZipDeflaterDataFormat("zipDeflater", (ZipDeflaterDataFormat) def.getDataFormatType());
case "ZipFileDataFormat" -> doWriteZipFileDataFormat("zipFile", (ZipFileDataFormat) def.getDataFormatType());
}
});
endElement(name);
}
protected void doWriteValidateDefinition(
String name,
ValidateDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("predicateExceptionFactory", def.getPredicateExceptionFactory());
doWriteExpressionNodeElements(def);
endElement(name);
}
protected void doWriteValueDefinition(
String name,
ValueDefinition def)
throws IOException {
startElement(name);
doWriteValue(def.getValue());
endElement(name);
}
protected void doWriteWhenDefinition(
String name,
WhenDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteOutputExpressionNodeElements(def);
endElement(name);
}
protected void doWriteWireTapDefinition(
String name,
WireTapDefinition> def)
throws IOException {
startElement(name);
doWriteToDynamicDefinitionAttributes(def);
doWriteAttribute("dynamicUri", def.getDynamicUri());
doWriteAttribute("onPrepare", def.getOnPrepare());
doWriteAttribute("executorService", def.getExecutorService());
doWriteAttribute("copy", def.getCopy());
endElement(name);
}
protected void doWriteApplicationDefinition(
String name,
ApplicationDefinition def)
throws IOException {
startElement(name);
doWriteBeansDefinitionElements(def);
endElement(name);
}
protected void doWriteBeanConstructorDefinition(
String name,
BeanConstructorDefinition def)
throws IOException {
startElement(name);
doWriteAttribute("index", toString(def.getIndex()));
doWriteAttribute("value", def.getValue());
endElement(name);
}
protected void doWriteBeanConstructorsDefinition(
String name,
BeanConstructorsDefinition def)
throws IOException {
startElement(name);
doWriteList(null, "constructor", def.getConstructors(), this::doWriteBeanConstructorDefinition);
endElement(name);
}
protected void doWriteBeanPropertiesDefinition(
String name,
BeanPropertiesDefinition def)
throws IOException {
startElement(name);
doWriteList(null, "property", def.getProperties(), this::doWriteBeanPropertyDefinition);
endElement(name);
}
protected void doWriteBeanPropertyDefinition(
String name,
BeanPropertyDefinition def)
throws IOException {
startElement(name);
doWriteAttribute("value", def.getValue());
doWriteAttribute("key", def.getKey());
doWriteElement("properties", def.getProperties(), this::doWriteBeanPropertiesDefinition);
endElement(name);
}
protected void doWriteBeansDefinitionElements(
BeansDefinition def)
throws IOException {
doWriteList(null, "route", def.getRoutes(), this::doWriteRouteDefinition);
domElements(def.getSpringBeans());
domElements(def.getBlueprintBeans());
doWriteList(null, "component-scan", def.getComponentScanning(), this::doWriteComponentScanDefinition);
doWriteList(null, "bean", def.getBeans(), this::doWriteRegistryBeanDefinition);
doWriteList(null, "restConfiguration", def.getRestConfigurations(), this::doWriteRestConfigurationDefinition);
doWriteList(null, "rest", def.getRests(), this::doWriteRestDefinition);
doWriteList(null, "routeConfiguration", def.getRouteConfigurations(), this::doWriteRouteConfigurationDefinition);
doWriteList(null, "routeTemplate", def.getRouteTemplates(), this::doWriteRouteTemplateDefinition);
doWriteList(null, "templatedRoute", def.getTemplatedRoutes(), this::doWriteTemplatedRouteDefinition);
}
protected void doWriteBeansDefinition(
String name,
BeansDefinition def)
throws IOException {
startElement(name);
doWriteBeansDefinitionElements(def);
endElement(name);
}
protected void doWriteComponentScanDefinition(
String name,
ComponentScanDefinition def)
throws IOException {
startElement(name);
doWriteAttribute("base-package", def.getBasePackage());
endElement(name);
}
protected void doWriteRegistryBeanDefinition(
String name,
RegistryBeanDefinition def)
throws IOException {
startElement(name);
doWriteAttribute("factoryMethod", def.getFactoryMethod());
doWriteAttribute("initMethod", def.getInitMethod());
doWriteAttribute("scriptLanguage", def.getScriptLanguage());
doWriteAttribute("builderClass", def.getBuilderClass());
doWriteAttribute("name", def.getName());
doWriteAttribute("builderMethod", def.getBuilderMethod());
doWriteAttribute("destroyMethod", def.getDestroyMethod());
doWriteAttribute("type", def.getType());
doWriteAttribute("factoryBean", def.getFactoryBean());
doWriteElement("constructors", new BeanConstructorsAdapter().marshal(def.getConstructors()), this::doWriteBeanConstructorsDefinition);
doWriteElement("script", def.getScript(), this::doWriteString);
doWriteElement("properties", new BeanPropertiesAdapter().marshal(def.getProperties()), this::doWriteBeanPropertiesDefinition);
endElement(name);
}
protected void doWriteBlacklistServiceCallServiceFilterConfiguration(
String name,
BlacklistServiceCallServiceFilterConfiguration def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteServiceCallConfigurationElements(def);
doWriteList(null, "servers", def.getServers(), this::doWriteString);
endElement(name);
}
protected void doWriteCachingServiceCallServiceDiscoveryConfiguration(
String name,
CachingServiceCallServiceDiscoveryConfiguration def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("units", def.getUnits());
doWriteAttribute("timeout", def.getTimeout());
doWriteServiceCallConfigurationElements(def);
doWriteElement(null, def.getServiceDiscoveryConfiguration(), (n, v) -> {
switch (v.getClass().getSimpleName()) {
case "ConsulServiceCallServiceDiscoveryConfiguration" -> doWriteConsulServiceCallServiceDiscoveryConfiguration("consulServiceDiscovery", (ConsulServiceCallServiceDiscoveryConfiguration) def.getServiceDiscoveryConfiguration());
case "DnsServiceCallServiceDiscoveryConfiguration" -> doWriteDnsServiceCallServiceDiscoveryConfiguration("dnsServiceDiscovery", (DnsServiceCallServiceDiscoveryConfiguration) def.getServiceDiscoveryConfiguration());
case "KubernetesServiceCallServiceDiscoveryConfiguration" -> doWriteKubernetesServiceCallServiceDiscoveryConfiguration("kubernetesServiceDiscovery", (KubernetesServiceCallServiceDiscoveryConfiguration) def.getServiceDiscoveryConfiguration());
case "CombinedServiceCallServiceDiscoveryConfiguration" -> doWriteCombinedServiceCallServiceDiscoveryConfiguration("combinedServiceDiscovery", (CombinedServiceCallServiceDiscoveryConfiguration) def.getServiceDiscoveryConfiguration());
case "StaticServiceCallServiceDiscoveryConfiguration" -> doWriteStaticServiceCallServiceDiscoveryConfiguration("staticServiceDiscovery", (StaticServiceCallServiceDiscoveryConfiguration) def.getServiceDiscoveryConfiguration());
}
});
endElement(name);
}
protected void doWriteCombinedServiceCallServiceDiscoveryConfiguration(
String name,
CombinedServiceCallServiceDiscoveryConfiguration def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteServiceCallConfigurationElements(def);
doWriteList(null, null, def.getServiceDiscoveryConfigurations(), (n, v) -> {
switch (v.getClass().getSimpleName()) {
case "ConsulServiceCallServiceDiscoveryConfiguration" -> doWriteConsulServiceCallServiceDiscoveryConfiguration("consulServiceDiscovery", (ConsulServiceCallServiceDiscoveryConfiguration) v);
case "DnsServiceCallServiceDiscoveryConfiguration" -> doWriteDnsServiceCallServiceDiscoveryConfiguration("dnsServiceDiscovery", (DnsServiceCallServiceDiscoveryConfiguration) v);
case "KubernetesServiceCallServiceDiscoveryConfiguration" -> doWriteKubernetesServiceCallServiceDiscoveryConfiguration("kubernetesServiceDiscovery", (KubernetesServiceCallServiceDiscoveryConfiguration) v);
case "StaticServiceCallServiceDiscoveryConfiguration" -> doWriteStaticServiceCallServiceDiscoveryConfiguration("staticServiceDiscovery", (StaticServiceCallServiceDiscoveryConfiguration) v);
case "CachingServiceCallServiceDiscoveryConfiguration" -> doWriteCachingServiceCallServiceDiscoveryConfiguration("cachingServiceDiscovery", (CachingServiceCallServiceDiscoveryConfiguration) v);
}
});
endElement(name);
}
protected void doWriteCombinedServiceCallServiceFilterConfiguration(
String name,
CombinedServiceCallServiceFilterConfiguration def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteServiceCallConfigurationElements(def);
doWriteList(null, null, def.getServiceFilterConfigurations(), (n, v) -> {
switch (v.getClass().getSimpleName()) {
case "BlacklistServiceCallServiceFilterConfiguration" -> doWriteBlacklistServiceCallServiceFilterConfiguration("blacklistServiceFilter", (BlacklistServiceCallServiceFilterConfiguration) v);
case "CustomServiceCallServiceFilterConfiguration" -> doWriteCustomServiceCallServiceFilterConfiguration("customServiceFilter", (CustomServiceCallServiceFilterConfiguration) v);
case "HealthyServiceCallServiceFilterConfiguration" -> doWriteHealthyServiceCallServiceFilterConfiguration("healthyServiceFilter", (HealthyServiceCallServiceFilterConfiguration) v);
case "PassThroughServiceCallServiceFilterConfiguration" -> doWritePassThroughServiceCallServiceFilterConfiguration("passThroughServiceFilter", (PassThroughServiceCallServiceFilterConfiguration) v);
}
});
endElement(name);
}
protected void doWriteConsulServiceCallServiceDiscoveryConfiguration(
String name,
ConsulServiceCallServiceDiscoveryConfiguration def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("password", def.getPassword());
doWriteAttribute("readTimeoutMillis", def.getReadTimeoutMillis());
doWriteAttribute("aclToken", def.getAclToken());
doWriteAttribute("connectTimeoutMillis", def.getConnectTimeoutMillis());
doWriteAttribute("writeTimeoutMillis", def.getWriteTimeoutMillis());
doWriteAttribute("datacenter", def.getDatacenter());
doWriteAttribute("userName", def.getUserName());
doWriteAttribute("blockSeconds", def.getBlockSeconds());
doWriteAttribute("url", def.getUrl());
doWriteServiceCallConfigurationElements(def);
endElement(name);
}
protected void doWriteCustomServiceCallServiceFilterConfiguration(
String name,
CustomServiceCallServiceFilterConfiguration def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("ref", def.getServiceFilterRef());
doWriteServiceCallConfigurationElements(def);
endElement(name);
}
protected void doWriteDefaultServiceCallServiceLoadBalancerConfiguration(
String name,
DefaultServiceCallServiceLoadBalancerConfiguration def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteServiceCallConfigurationElements(def);
endElement(name);
}
protected void doWriteDnsServiceCallServiceDiscoveryConfiguration(
String name,
DnsServiceCallServiceDiscoveryConfiguration def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("domain", def.getDomain());
doWriteAttribute("proto", def.getProto());
doWriteServiceCallConfigurationElements(def);
endElement(name);
}
protected void doWriteHealthyServiceCallServiceFilterConfiguration(
String name,
HealthyServiceCallServiceFilterConfiguration def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteServiceCallConfigurationElements(def);
endElement(name);
}
protected void doWriteKubernetesServiceCallServiceDiscoveryConfiguration(
String name,
KubernetesServiceCallServiceDiscoveryConfiguration def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("lookup", def.getLookup());
doWriteAttribute("portProtocol", def.getPortProtocol());
doWriteAttribute("caCertData", def.getCaCertData());
doWriteAttribute("portName", def.getPortName());
doWriteAttribute("oauthToken", def.getOauthToken());
doWriteAttribute("clientKeyAlgo", def.getClientKeyAlgo());
doWriteAttribute("clientCertFile", def.getClientCertFile());
doWriteAttribute("dnsDomain", def.getDnsDomain());
doWriteAttribute("password", def.getPassword());
doWriteAttribute("apiVersion", def.getApiVersion());
doWriteAttribute("clientKeyData", def.getClientKeyData());
doWriteAttribute("masterUrl", def.getMasterUrl());
doWriteAttribute("namespace", def.getNamespace());
doWriteAttribute("clientCertData", def.getClientCertData());
doWriteAttribute("clientKeyFile", def.getClientKeyFile());
doWriteAttribute("caCertFile", def.getCaCertFile());
doWriteAttribute("clientKeyPassphrase", def.getClientKeyPassphrase());
doWriteAttribute("trustCerts", def.getTrustCerts());
doWriteAttribute("username", def.getUsername());
doWriteServiceCallConfigurationElements(def);
endElement(name);
}
protected void doWritePassThroughServiceCallServiceFilterConfiguration(
String name,
PassThroughServiceCallServiceFilterConfiguration def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteServiceCallConfigurationElements(def);
endElement(name);
}
protected void doWriteServiceCallConfigurationAttributes(
ServiceCallConfiguration def)
throws IOException {
doWriteIdentifiedTypeAttributes(def);
}
protected void doWriteServiceCallConfigurationElements(
ServiceCallConfiguration def)
throws IOException {
doWriteList(null, "properties", def.getProperties(), this::doWritePropertyDefinition);
}
protected void doWriteServiceCallConfiguration(
String name,
ServiceCallConfiguration def)
throws IOException {
startElement(name);
doWriteServiceCallConfigurationAttributes(def);
doWriteServiceCallConfigurationElements(def);
endElement(name);
}
protected void doWriteServiceCallConfigurationDefinition(
String name,
ServiceCallConfigurationDefinition def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("serviceDiscoveryRef", def.getServiceDiscoveryRef());
doWriteAttribute("pattern", def.getPattern());
doWriteAttribute("uri", def.getUri());
doWriteAttribute("expressionRef", def.getExpressionRef());
doWriteAttribute("component", def.getComponent());
doWriteAttribute("loadBalancerRef", def.getLoadBalancerRef());
doWriteAttribute("serviceFilterRef", def.getServiceFilterRef());
doWriteAttribute("serviceChooserRef", def.getServiceChooserRef());
doWriteElement(null, def.getServiceFilterConfiguration(), (n, v) -> {
switch (v.getClass().getSimpleName()) {
case "BlacklistServiceCallServiceFilterConfiguration" -> doWriteBlacklistServiceCallServiceFilterConfiguration("blacklistServiceFilter", (BlacklistServiceCallServiceFilterConfiguration) def.getServiceFilterConfiguration());
case "CombinedServiceCallServiceFilterConfiguration" -> doWriteCombinedServiceCallServiceFilterConfiguration("combinedServiceFilter", (CombinedServiceCallServiceFilterConfiguration) def.getServiceFilterConfiguration());
case "CustomServiceCallServiceFilterConfiguration" -> doWriteCustomServiceCallServiceFilterConfiguration("customServiceFilter", (CustomServiceCallServiceFilterConfiguration) def.getServiceFilterConfiguration());
case "HealthyServiceCallServiceFilterConfiguration" -> doWriteHealthyServiceCallServiceFilterConfiguration("healthyServiceFilter", (HealthyServiceCallServiceFilterConfiguration) def.getServiceFilterConfiguration());
case "PassThroughServiceCallServiceFilterConfiguration" -> doWritePassThroughServiceCallServiceFilterConfiguration("passThroughServiceFilter", (PassThroughServiceCallServiceFilterConfiguration) def.getServiceFilterConfiguration());
}
});
doWriteElement(null, def.getLoadBalancerConfiguration(), (n, v) -> {
switch (v.getClass().getSimpleName()) {
case "DefaultServiceCallServiceLoadBalancerConfiguration" -> doWriteDefaultServiceCallServiceLoadBalancerConfiguration("defaultLoadBalancer", (DefaultServiceCallServiceLoadBalancerConfiguration) def.getLoadBalancerConfiguration());
}
});
doWriteElement(null, def.getServiceDiscoveryConfiguration(), (n, v) -> {
switch (v.getClass().getSimpleName()) {
case "CachingServiceCallServiceDiscoveryConfiguration" -> doWriteCachingServiceCallServiceDiscoveryConfiguration("cachingServiceDiscovery", (CachingServiceCallServiceDiscoveryConfiguration) def.getServiceDiscoveryConfiguration());
case "CombinedServiceCallServiceDiscoveryConfiguration" -> doWriteCombinedServiceCallServiceDiscoveryConfiguration("combinedServiceDiscovery", (CombinedServiceCallServiceDiscoveryConfiguration) def.getServiceDiscoveryConfiguration());
case "ConsulServiceCallServiceDiscoveryConfiguration" -> doWriteConsulServiceCallServiceDiscoveryConfiguration("consulServiceDiscovery", (ConsulServiceCallServiceDiscoveryConfiguration) def.getServiceDiscoveryConfiguration());
case "DnsServiceCallServiceDiscoveryConfiguration" -> doWriteDnsServiceCallServiceDiscoveryConfiguration("dnsServiceDiscovery", (DnsServiceCallServiceDiscoveryConfiguration) def.getServiceDiscoveryConfiguration());
case "KubernetesServiceCallServiceDiscoveryConfiguration" -> doWriteKubernetesServiceCallServiceDiscoveryConfiguration("kubernetesServiceDiscovery", (KubernetesServiceCallServiceDiscoveryConfiguration) def.getServiceDiscoveryConfiguration());
case "StaticServiceCallServiceDiscoveryConfiguration" -> doWriteStaticServiceCallServiceDiscoveryConfiguration("staticServiceDiscovery", (StaticServiceCallServiceDiscoveryConfiguration) def.getServiceDiscoveryConfiguration());
case "ZooKeeperServiceCallServiceDiscoveryConfiguration" -> doWriteZooKeeperServiceCallServiceDiscoveryConfiguration("zookeeperServiceDiscovery", (ZooKeeperServiceCallServiceDiscoveryConfiguration) def.getServiceDiscoveryConfiguration());
}
});
doWriteElement("expression", def.getExpressionConfiguration(), this::doWriteServiceCallExpressionConfiguration);
endElement(name);
}
protected void doWriteServiceCallDefinition(
String name,
ServiceCallDefinition def)
throws IOException {
startElement(name);
doWriteProcessorDefinitionAttributes(def);
doWriteAttribute("serviceDiscoveryRef", def.getServiceDiscoveryRef());
doWriteAttribute("configurationRef", def.getConfigurationRef());
doWriteAttribute("pattern", def.getPattern());
doWriteAttribute("uri", def.getUri());
doWriteAttribute("expressionRef", def.getExpressionRef());
doWriteAttribute("component", def.getComponent());
doWriteAttribute("loadBalancerRef", def.getLoadBalancerRef());
doWriteAttribute("serviceFilterRef", def.getServiceFilterRef());
doWriteAttribute("name", def.getName());
doWriteAttribute("serviceChooserRef", def.getServiceChooserRef());
doWriteElement(null, def.getServiceFilterConfiguration(), (n, v) -> {
switch (v.getClass().getSimpleName()) {
case "BlacklistServiceCallServiceFilterConfiguration" -> doWriteBlacklistServiceCallServiceFilterConfiguration("blacklistServiceFilter", (BlacklistServiceCallServiceFilterConfiguration) def.getServiceFilterConfiguration());
case "CombinedServiceCallServiceFilterConfiguration" -> doWriteCombinedServiceCallServiceFilterConfiguration("combinedServiceFilter", (CombinedServiceCallServiceFilterConfiguration) def.getServiceFilterConfiguration());
case "CustomServiceCallServiceFilterConfiguration" -> doWriteCustomServiceCallServiceFilterConfiguration("customServiceFilter", (CustomServiceCallServiceFilterConfiguration) def.getServiceFilterConfiguration());
case "HealthyServiceCallServiceFilterConfiguration" -> doWriteHealthyServiceCallServiceFilterConfiguration("healthyServiceFilter", (HealthyServiceCallServiceFilterConfiguration) def.getServiceFilterConfiguration());
case "PassThroughServiceCallServiceFilterConfiguration" -> doWritePassThroughServiceCallServiceFilterConfiguration("passThroughServiceFilter", (PassThroughServiceCallServiceFilterConfiguration) def.getServiceFilterConfiguration());
}
});
doWriteElement(null, def.getLoadBalancerConfiguration(), (n, v) -> {
switch (v.getClass().getSimpleName()) {
case "DefaultServiceCallServiceLoadBalancerConfiguration" -> doWriteDefaultServiceCallServiceLoadBalancerConfiguration("defaultLoadBalancer", (DefaultServiceCallServiceLoadBalancerConfiguration) def.getLoadBalancerConfiguration());
}
});
doWriteElement(null, def.getServiceDiscoveryConfiguration(), (n, v) -> {
switch (v.getClass().getSimpleName()) {
case "CachingServiceCallServiceDiscoveryConfiguration" -> doWriteCachingServiceCallServiceDiscoveryConfiguration("cachingServiceDiscovery", (CachingServiceCallServiceDiscoveryConfiguration) def.getServiceDiscoveryConfiguration());
case "CombinedServiceCallServiceDiscoveryConfiguration" -> doWriteCombinedServiceCallServiceDiscoveryConfiguration("combinedServiceDiscovery", (CombinedServiceCallServiceDiscoveryConfiguration) def.getServiceDiscoveryConfiguration());
case "ConsulServiceCallServiceDiscoveryConfiguration" -> doWriteConsulServiceCallServiceDiscoveryConfiguration("consulServiceDiscovery", (ConsulServiceCallServiceDiscoveryConfiguration) def.getServiceDiscoveryConfiguration());
case "DnsServiceCallServiceDiscoveryConfiguration" -> doWriteDnsServiceCallServiceDiscoveryConfiguration("dnsServiceDiscovery", (DnsServiceCallServiceDiscoveryConfiguration) def.getServiceDiscoveryConfiguration());
case "KubernetesServiceCallServiceDiscoveryConfiguration" -> doWriteKubernetesServiceCallServiceDiscoveryConfiguration("kubernetesServiceDiscovery", (KubernetesServiceCallServiceDiscoveryConfiguration) def.getServiceDiscoveryConfiguration());
case "StaticServiceCallServiceDiscoveryConfiguration" -> doWriteStaticServiceCallServiceDiscoveryConfiguration("staticServiceDiscovery", (StaticServiceCallServiceDiscoveryConfiguration) def.getServiceDiscoveryConfiguration());
case "ZooKeeperServiceCallServiceDiscoveryConfiguration" -> doWriteZooKeeperServiceCallServiceDiscoveryConfiguration("zookeeperServiceDiscovery", (ZooKeeperServiceCallServiceDiscoveryConfiguration) def.getServiceDiscoveryConfiguration());
}
});
doWriteElement("expression", def.getExpressionConfiguration(), this::doWriteServiceCallExpressionConfiguration);
endElement(name);
}
protected void doWriteServiceCallExpressionConfiguration(
String name,
ServiceCallExpressionConfiguration def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("hostHeader", def.getHostHeader());
doWriteAttribute("portHeader", def.getPortHeader());
doWriteServiceCallConfigurationElements(def);
doWriteElement(null, def.getExpressionType(), this::doWriteExpressionDefinitionRef);
endElement(name);
}
protected void doWriteServiceCallServiceChooserConfiguration(
String name,
ServiceCallServiceChooserConfiguration def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteServiceCallConfigurationElements(def);
endElement(name);
}
protected void doWriteServiceCallServiceDiscoveryConfigurationAttributes(
ServiceCallServiceDiscoveryConfiguration def)
throws IOException {
doWriteIdentifiedTypeAttributes(def);
}
protected void doWriteServiceCallServiceDiscoveryConfigurationElements(
ServiceCallServiceDiscoveryConfiguration def)
throws IOException {
doWriteServiceCallConfigurationElements(def);
}
protected void doWriteServiceCallServiceDiscoveryConfiguration(
String name,
ServiceCallServiceDiscoveryConfiguration def)
throws IOException {
startElement(name);
doWriteServiceCallServiceDiscoveryConfigurationAttributes(def);
doWriteServiceCallServiceDiscoveryConfigurationElements(def);
endElement(name);
}
protected void doWriteServiceCallServiceFilterConfigurationAttributes(
ServiceCallServiceFilterConfiguration def)
throws IOException {
doWriteIdentifiedTypeAttributes(def);
}
protected void doWriteServiceCallServiceFilterConfigurationElements(
ServiceCallServiceFilterConfiguration def)
throws IOException {
doWriteServiceCallConfigurationElements(def);
}
protected void doWriteServiceCallServiceFilterConfiguration(
String name,
ServiceCallServiceFilterConfiguration def)
throws IOException {
startElement(name);
doWriteServiceCallServiceFilterConfigurationAttributes(def);
doWriteServiceCallServiceFilterConfigurationElements(def);
endElement(name);
}
protected void doWriteServiceCallServiceLoadBalancerConfigurationAttributes(
ServiceCallServiceLoadBalancerConfiguration def)
throws IOException {
doWriteIdentifiedTypeAttributes(def);
}
protected void doWriteServiceCallServiceLoadBalancerConfigurationElements(
ServiceCallServiceLoadBalancerConfiguration def)
throws IOException {
doWriteServiceCallConfigurationElements(def);
}
protected void doWriteServiceCallServiceLoadBalancerConfiguration(
String name,
ServiceCallServiceLoadBalancerConfiguration def)
throws IOException {
startElement(name);
doWriteServiceCallServiceLoadBalancerConfigurationAttributes(def);
doWriteServiceCallServiceLoadBalancerConfigurationElements(def);
endElement(name);
}
protected void doWriteStaticServiceCallServiceDiscoveryConfiguration(
String name,
StaticServiceCallServiceDiscoveryConfiguration def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteServiceCallConfigurationElements(def);
doWriteList(null, "servers", def.getServers(), this::doWriteString);
endElement(name);
}
protected void doWriteZooKeeperServiceCallServiceDiscoveryConfiguration(
String name,
ZooKeeperServiceCallServiceDiscoveryConfiguration def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("reconnectMaxRetries", def.getReconnectMaxRetries());
doWriteAttribute("nodes", def.getNodes());
doWriteAttribute("basePath", def.getBasePath());
doWriteAttribute("reconnectBaseSleepTime", def.getReconnectBaseSleepTime());
doWriteAttribute("namespace", def.getNamespace());
doWriteAttribute("reconnectMaxSleepTime", def.getReconnectMaxSleepTime());
doWriteAttribute("sessionTimeout", def.getSessionTimeout());
doWriteAttribute("connectionTimeout", def.getConnectionTimeout());
doWriteServiceCallConfigurationElements(def);
endElement(name);
}
protected void doWriteBatchResequencerConfig(
String name,
BatchResequencerConfig def)
throws IOException {
startElement(name);
doWriteAttribute("reverse", def.getReverse());
doWriteAttribute("batchSize", def.getBatchSize());
doWriteAttribute("allowDuplicates", def.getAllowDuplicates());
doWriteAttribute("batchTimeout", def.getBatchTimeout());
doWriteAttribute("ignoreInvalidExchanges", def.getIgnoreInvalidExchanges());
endElement(name);
}
protected void doWriteResequencerConfig(
String name,
ResequencerConfig def)
throws IOException {
startElement(name);
endElement(name);
}
protected void doWriteStreamResequencerConfig(
String name,
StreamResequencerConfig def)
throws IOException {
startElement(name);
doWriteAttribute("comparator", def.getComparator());
doWriteAttribute("timeout", def.getTimeout());
doWriteAttribute("rejectOld", def.getRejectOld());
doWriteAttribute("ignoreInvalidExchanges", def.getIgnoreInvalidExchanges());
doWriteAttribute("deliveryAttemptInterval", def.getDeliveryAttemptInterval());
doWriteAttribute("capacity", def.getCapacity());
endElement(name);
}
protected void doWriteASN1DataFormat(
String name,
ASN1DataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("unmarshalType", def.getUnmarshalTypeName());
doWriteAttribute("usingIterator", def.getUsingIterator());
endElement(name);
}
protected void doWriteAvroDataFormat(
String name,
AvroDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("allowUnmarshallType", def.getAllowUnmarshallType());
doWriteAttribute("include", def.getInclude());
doWriteAttribute("contentTypeHeader", def.getContentTypeHeader());
doWriteAttribute("unmarshalType", def.getUnmarshalTypeName());
doWriteAttribute("autoDiscoverSchemaResolver", def.getAutoDiscoverSchemaResolver());
doWriteAttribute("timezone", def.getTimezone());
doWriteAttribute("moduleClassNames", def.getModuleClassNames());
doWriteAttribute("instanceClassName", def.getInstanceClassName());
doWriteAttribute("collectionType", def.getCollectionTypeName());
doWriteAttribute("allowJmsType", def.getAllowJmsType());
doWriteAttribute("jsonView", def.getJsonViewTypeName());
doWriteAttribute("enableFeatures", def.getEnableFeatures());
doWriteAttribute("useList", def.getUseList());
doWriteAttribute("disableFeatures", def.getDisableFeatures());
doWriteAttribute("moduleRefs", def.getModuleRefs());
doWriteAttribute("schemaResolver", def.getSchemaResolver());
doWriteAttribute("useDefaultObjectMapper", def.getUseDefaultObjectMapper());
doWriteAttribute("objectMapper", def.getObjectMapper());
doWriteAttribute("library", toString(def.getLibrary()));
doWriteAttribute("autoDiscoverObjectMapper", def.getAutoDiscoverObjectMapper());
endElement(name);
}
protected void doWriteBarcodeDataFormat(
String name,
BarcodeDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("barcodeFormat", def.getBarcodeFormat());
doWriteAttribute("width", def.getWidth());
doWriteAttribute("imageType", def.getImageType());
doWriteAttribute("height", def.getHeight());
endElement(name);
}
protected void doWriteBase64DataFormat(
String name,
Base64DataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("urlSafe", def.getUrlSafe());
doWriteAttribute("lineSeparator", def.getLineSeparator());
doWriteAttribute("lineLength", def.getLineLength());
endElement(name);
}
protected void doWriteBeanioDataFormat(
String name,
BeanioDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("mapping", def.getMapping());
doWriteAttribute("ignoreUnexpectedRecords", def.getIgnoreUnexpectedRecords());
doWriteAttribute("ignoreUnidentifiedRecords", def.getIgnoreUnidentifiedRecords());
doWriteAttribute("beanReaderErrorHandlerType", def.getBeanReaderErrorHandlerType());
doWriteAttribute("unmarshalSingleObject", def.getUnmarshalSingleObject());
doWriteAttribute("encoding", def.getEncoding());
doWriteAttribute("streamName", def.getStreamName());
doWriteAttribute("ignoreInvalidRecords", def.getIgnoreInvalidRecords());
endElement(name);
}
protected void doWriteBindyDataFormat(
String name,
BindyDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("unwrapSingleInstance", def.getUnwrapSingleInstance());
doWriteAttribute("classType", def.getClassTypeAsString());
doWriteAttribute("locale", def.getLocale());
doWriteAttribute("type", def.getType());
doWriteAttribute("allowEmptyStream", def.getAllowEmptyStream());
endElement(name);
}
protected void doWriteCBORDataFormat(
String name,
CBORDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("allowUnmarshallType", def.getAllowUnmarshallType());
doWriteAttribute("useDefaultObjectMapper", def.getUseDefaultObjectMapper());
doWriteAttribute("prettyPrint", def.getPrettyPrint());
doWriteAttribute("objectMapper", def.getObjectMapper());
doWriteAttribute("unmarshalType", def.getUnmarshalTypeName());
doWriteAttribute("collectionType", def.getCollectionTypeName());
doWriteAttribute("allowJmsType", def.getAllowJmsType());
doWriteAttribute("enableFeatures", def.getEnableFeatures());
doWriteAttribute("useList", def.getUseList());
doWriteAttribute("disableFeatures", def.getDisableFeatures());
endElement(name);
}
protected void doWriteCryptoDataFormat(
String name,
CryptoDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("shouldAppendHMAC", def.getShouldAppendHMAC());
doWriteAttribute("inline", def.getInline());
doWriteAttribute("macAlgorithm", def.getMacAlgorithm());
doWriteAttribute("algorithmParameterRef", def.getAlgorithmParameterRef());
doWriteAttribute("initVectorRef", def.getInitVectorRef());
doWriteAttribute("cryptoProvider", def.getCryptoProvider());
doWriteAttribute("keyRef", def.getKeyRef());
doWriteAttribute("bufferSize", def.getBufferSize());
doWriteAttribute("algorithm", def.getAlgorithm());
endElement(name);
}
protected void doWriteCsvDataFormat(
String name,
CsvDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("escapeDisabled", def.getEscapeDisabled());
doWriteAttribute("recordConverterRef", def.getRecordConverterRef());
doWriteAttribute("ignoreEmptyLines", def.getIgnoreEmptyLines());
doWriteAttribute("trailingDelimiter", def.getTrailingDelimiter());
doWriteAttribute("lazyLoad", def.getLazyLoad());
doWriteAttribute("headerDisabled", def.getHeaderDisabled());
doWriteAttribute("commentMarkerDisabled", def.getCommentMarkerDisabled());
doWriteAttribute("skipHeaderRecord", def.getSkipHeaderRecord());
doWriteAttribute("quote", def.getQuote());
doWriteAttribute("useMaps", def.getUseMaps());
doWriteAttribute("trim", def.getTrim());
doWriteAttribute("delimiter", def.getDelimiter());
doWriteAttribute("nullString", def.getNullString());
doWriteAttribute("allowMissingColumnNames", def.getAllowMissingColumnNames());
doWriteAttribute("escape", def.getEscape());
doWriteAttribute("nullStringDisabled", def.getNullStringDisabled());
doWriteAttribute("commentMarker", def.getCommentMarker());
doWriteAttribute("formatRef", def.getFormatRef());
doWriteAttribute("quoteMode", def.getQuoteMode());
doWriteAttribute("formatName", def.getFormatName());
doWriteAttribute("ignoreSurroundingSpaces", def.getIgnoreSurroundingSpaces());
doWriteAttribute("quoteDisabled", def.getQuoteDisabled());
doWriteAttribute("useOrderedMaps", def.getUseOrderedMaps());
doWriteAttribute("ignoreHeaderCase", def.getIgnoreHeaderCase());
doWriteAttribute("recordSeparatorDisabled", def.getRecordSeparatorDisabled());
doWriteAttribute("captureHeaderRecord", def.getCaptureHeaderRecord());
doWriteAttribute("marshallerFactoryRef", def.getMarshallerFactoryRef());
doWriteAttribute("recordSeparator", def.getRecordSeparator());
doWriteList(null, "header", def.getHeader(), this::doWriteString);
endElement(name);
}
protected void doWriteCustomDataFormat(
String name,
CustomDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("ref", def.getRef());
endElement(name);
}
protected void doWriteDataFormatsDefinition(
String name,
DataFormatsDefinition def)
throws IOException {
startElement(name);
doWriteList(null, null, def.getDataFormats(), (n, v) -> {
switch (v.getClass().getSimpleName()) {
case "ASN1DataFormat" -> doWriteASN1DataFormat("asn1", (ASN1DataFormat) v);
case "AvroDataFormat" -> doWriteAvroDataFormat("avro", (AvroDataFormat) v);
case "BarcodeDataFormat" -> doWriteBarcodeDataFormat("barcode", (BarcodeDataFormat) v);
case "Base64DataFormat" -> doWriteBase64DataFormat("base64", (Base64DataFormat) v);
case "BeanioDataFormat" -> doWriteBeanioDataFormat("beanio", (BeanioDataFormat) v);
case "BindyDataFormat" -> doWriteBindyDataFormat("bindy", (BindyDataFormat) v);
case "CBORDataFormat" -> doWriteCBORDataFormat("cbor", (CBORDataFormat) v);
case "CryptoDataFormat" -> doWriteCryptoDataFormat("crypto", (CryptoDataFormat) v);
case "CsvDataFormat" -> doWriteCsvDataFormat("csv", (CsvDataFormat) v);
case "CustomDataFormat" -> doWriteCustomDataFormat("custom", (CustomDataFormat) v);
case "FhirJsonDataFormat" -> doWriteFhirJsonDataFormat("fhirJson", (FhirJsonDataFormat) v);
case "FhirXmlDataFormat" -> doWriteFhirXmlDataFormat("fhirXml", (FhirXmlDataFormat) v);
case "FlatpackDataFormat" -> doWriteFlatpackDataFormat("flatpack", (FlatpackDataFormat) v);
case "GrokDataFormat" -> doWriteGrokDataFormat("grok", (GrokDataFormat) v);
case "GzipDeflaterDataFormat" -> doWriteGzipDeflaterDataFormat("gzipDeflater", (GzipDeflaterDataFormat) v);
case "HL7DataFormat" -> doWriteHL7DataFormat("hl7", (HL7DataFormat) v);
case "IcalDataFormat" -> doWriteIcalDataFormat("ical", (IcalDataFormat) v);
case "JacksonXMLDataFormat" -> doWriteJacksonXMLDataFormat("jacksonXml", (JacksonXMLDataFormat) v);
case "JaxbDataFormat" -> doWriteJaxbDataFormat("jaxb", (JaxbDataFormat) v);
case "JsonDataFormat" -> doWriteJsonDataFormat("json", (JsonDataFormat) v);
case "JsonApiDataFormat" -> doWriteJsonApiDataFormat("jsonApi", (JsonApiDataFormat) v);
case "LZFDataFormat" -> doWriteLZFDataFormat("lzf", (LZFDataFormat) v);
case "MimeMultipartDataFormat" -> doWriteMimeMultipartDataFormat("mimeMultipart", (MimeMultipartDataFormat) v);
case "ParquetAvroDataFormat" -> doWriteParquetAvroDataFormat("parquetAvro", (ParquetAvroDataFormat) v);
case "PGPDataFormat" -> doWritePGPDataFormat("pgp", (PGPDataFormat) v);
case "ProtobufDataFormat" -> doWriteProtobufDataFormat("protobuf", (ProtobufDataFormat) v);
case "RssDataFormat" -> doWriteRssDataFormat("rss", (RssDataFormat) v);
case "SoapDataFormat" -> doWriteSoapDataFormat("soap", (SoapDataFormat) v);
case "SwiftMtDataFormat" -> doWriteSwiftMtDataFormat("swiftMt", (SwiftMtDataFormat) v);
case "SwiftMxDataFormat" -> doWriteSwiftMxDataFormat("swiftMx", (SwiftMxDataFormat) v);
case "SyslogDataFormat" -> doWriteSyslogDataFormat("syslog", (SyslogDataFormat) v);
case "TarFileDataFormat" -> doWriteTarFileDataFormat("tarFile", (TarFileDataFormat) v);
case "ThriftDataFormat" -> doWriteThriftDataFormat("thrift", (ThriftDataFormat) v);
case "TidyMarkupDataFormat" -> doWriteTidyMarkupDataFormat("tidyMarkup", (TidyMarkupDataFormat) v);
case "UniVocityCsvDataFormat" -> doWriteUniVocityCsvDataFormat("univocityCsv", (UniVocityCsvDataFormat) v);
case "UniVocityFixedDataFormat" -> doWriteUniVocityFixedDataFormat("univocityFixed", (UniVocityFixedDataFormat) v);
case "UniVocityTsvDataFormat" -> doWriteUniVocityTsvDataFormat("univocityTsv", (UniVocityTsvDataFormat) v);
case "XMLSecurityDataFormat" -> doWriteXMLSecurityDataFormat("xmlSecurity", (XMLSecurityDataFormat) v);
case "YAMLDataFormat" -> doWriteYAMLDataFormat("yaml", (YAMLDataFormat) v);
case "ZipDeflaterDataFormat" -> doWriteZipDeflaterDataFormat("zipDeflater", (ZipDeflaterDataFormat) v);
case "ZipFileDataFormat" -> doWriteZipFileDataFormat("zipFile", (ZipFileDataFormat) v);
}
});
endElement(name);
}
protected void doWriteFhirDataformatAttributes(
FhirDataformat def)
throws IOException {
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("contentTypeHeader", def.getContentTypeHeader());
doWriteAttribute("dontStripVersionsFromReferencesAtPaths", def.getDontStripVersionsFromReferencesAtPaths());
doWriteAttribute("parserOptions", def.getParserOptions());
doWriteAttribute("prettyPrint", def.getPrettyPrint());
doWriteAttribute("dontEncodeElements", def.getDontEncodeElements());
doWriteAttribute("summaryMode", def.getSummaryMode());
doWriteAttribute("forceResourceId", def.getForceResourceId());
doWriteAttribute("encodeElementsAppliesToChildResourcesOnly", def.getEncodeElementsAppliesToChildResourcesOnly());
doWriteAttribute("parserErrorHandler", def.getParserErrorHandler());
doWriteAttribute("serverBaseUrl", def.getServerBaseUrl());
doWriteAttribute("fhirVersion", def.getFhirVersion());
doWriteAttribute("suppressNarratives", def.getSuppressNarratives());
doWriteAttribute("fhirContext", def.getFhirContext());
doWriteAttribute("stripVersionsFromReferences", def.getStripVersionsFromReferences());
doWriteAttribute("encodeElements", def.getEncodeElements());
doWriteAttribute("preferTypes", def.getPreferTypes());
doWriteAttribute("overrideResourceIdWithBundleEntryFullUrl", def.getOverrideResourceIdWithBundleEntryFullUrl());
doWriteAttribute("omitResourceId", def.getOmitResourceId());
}
protected void doWriteFhirDataformat(
String name,
FhirDataformat def)
throws IOException {
startElement(name);
doWriteFhirDataformatAttributes(def);
endElement(name);
}
protected void doWriteFhirJsonDataFormat(
String name,
FhirJsonDataFormat def)
throws IOException {
startElement(name);
doWriteFhirDataformatAttributes(def);
endElement(name);
}
protected void doWriteFhirXmlDataFormat(
String name,
FhirXmlDataFormat def)
throws IOException {
startElement(name);
doWriteFhirDataformatAttributes(def);
endElement(name);
}
protected void doWriteFlatpackDataFormat(
String name,
FlatpackDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("allowShortLines", def.getAllowShortLines());
doWriteAttribute("delimiter", def.getDelimiter());
doWriteAttribute("fixed", def.getFixed());
doWriteAttribute("definition", def.getDefinition());
doWriteAttribute("ignoreFirstRecord", def.getIgnoreFirstRecord());
doWriteAttribute("parserFactoryRef", def.getParserFactoryRef());
doWriteAttribute("textQualifier", def.getTextQualifier());
doWriteAttribute("ignoreExtraColumns", def.getIgnoreExtraColumns());
endElement(name);
}
protected void doWriteGrokDataFormat(
String name,
GrokDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("flattened", def.getFlattened());
doWriteAttribute("namedOnly", def.getNamedOnly());
doWriteAttribute("pattern", def.getPattern());
doWriteAttribute("allowMultipleMatchesPerLine", def.getAllowMultipleMatchesPerLine());
endElement(name);
}
protected void doWriteGzipDeflaterDataFormat(
String name,
GzipDeflaterDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
endElement(name);
}
protected void doWriteHL7DataFormat(
String name,
HL7DataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("validate", def.getValidate());
endElement(name);
}
protected void doWriteIcalDataFormat(
String name,
IcalDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("validating", def.getValidating());
endElement(name);
}
protected void doWriteJacksonXMLDataFormat(
String name,
JacksonXMLDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("include", def.getInclude());
doWriteAttribute("allowUnmarshallType", def.getAllowUnmarshallType());
doWriteAttribute("contentTypeHeader", def.getContentTypeHeader());
doWriteAttribute("prettyPrint", def.getPrettyPrint());
doWriteAttribute("unmarshalType", def.getUnmarshalTypeName());
doWriteAttribute("timezone", def.getTimezone());
doWriteAttribute("moduleClassNames", def.getModuleClassNames());
doWriteAttribute("collectionType", def.getCollectionTypeName());
doWriteAttribute("allowJmsType", def.getAllowJmsType());
doWriteAttribute("jsonView", def.getJsonViewTypeName());
doWriteAttribute("enableFeatures", def.getEnableFeatures());
doWriteAttribute("useList", def.getUseList());
doWriteAttribute("disableFeatures", def.getDisableFeatures());
doWriteAttribute("moduleRefs", def.getModuleRefs());
doWriteAttribute("enableJaxbAnnotationModule", def.getEnableJaxbAnnotationModule());
doWriteAttribute("xmlMapper", def.getXmlMapper());
endElement(name);
}
protected void doWriteJaxbDataFormat(
String name,
JaxbDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("accessExternalSchemaProtocols", def.getAccessExternalSchemaProtocols());
doWriteAttribute("schema", def.getSchema());
doWriteAttribute("contentTypeHeader", def.getContentTypeHeader());
doWriteAttribute("prettyPrint", def.getPrettyPrint());
doWriteAttribute("schemaLocation", def.getSchemaLocation());
doWriteAttribute("contextPathIsClassName", def.getContextPathIsClassName());
doWriteAttribute("ignoreJAXBElement", def.getIgnoreJAXBElement());
doWriteAttribute("xmlStreamWriterWrapper", def.getXmlStreamWriterWrapper());
doWriteAttribute("schemaSeverityLevel", def.getSchemaSeverityLevel());
doWriteAttribute("contextPath", def.getContextPath());
doWriteAttribute("noNamespaceSchemaLocation", def.getNoNamespaceSchemaLocation());
doWriteAttribute("encoding", def.getEncoding());
doWriteAttribute("fragment", def.getFragment());
doWriteAttribute("filterNonXmlChars", def.getFilterNonXmlChars());
doWriteAttribute("mustBeJAXBElement", def.getMustBeJAXBElement());
doWriteAttribute("objectFactory", def.getObjectFactory());
doWriteAttribute("namespacePrefixRef", def.getNamespacePrefixRef());
doWriteAttribute("partClass", def.getPartClass());
doWriteAttribute("jaxbProviderProperties", def.getJaxbProviderProperties());
doWriteAttribute("partNamespace", def.getPartNamespace());
endElement(name);
}
protected void doWriteJsonApiDataFormat(
String name,
JsonApiDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("dataFormatTypes", def.getDataFormatTypes());
doWriteAttribute("mainFormatType", def.getMainFormatType());
endElement(name);
}
protected void doWriteJsonDataFormat(
String name,
JsonDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("dateFormatPattern", def.getDateFormatPattern());
doWriteAttribute("contentTypeHeader", def.getContentTypeHeader());
doWriteAttribute("timezone", def.getTimezone());
doWriteAttribute("moduleClassNames", def.getModuleClassNames());
doWriteAttribute("collectionType", def.getCollectionTypeName());
doWriteAttribute("allowJmsType", def.getAllowJmsType());
doWriteAttribute("jsonView", def.getJsonViewTypeName());
doWriteAttribute("enableFeatures", def.getEnableFeatures());
doWriteAttribute("library", toString(def.getLibrary()));
doWriteAttribute("autoDiscoverObjectMapper", def.getAutoDiscoverObjectMapper());
doWriteAttribute("allowUnmarshallType", def.getAllowUnmarshallType());
doWriteAttribute("include", def.getInclude());
doWriteAttribute("prettyPrint", def.getPrettyPrint());
doWriteAttribute("unmarshalType", def.getUnmarshalTypeName());
doWriteAttribute("autoDiscoverSchemaResolver", def.getAutoDiscoverSchemaResolver());
doWriteAttribute("useList", def.getUseList());
doWriteAttribute("disableFeatures", def.getDisableFeatures());
doWriteAttribute("moduleRefs", def.getModuleRefs());
doWriteAttribute("schemaResolver", def.getSchemaResolver());
doWriteAttribute("useDefaultObjectMapper", def.getUseDefaultObjectMapper());
doWriteAttribute("objectMapper", def.getObjectMapper());
doWriteAttribute("namingStrategy", def.getNamingStrategy());
endElement(name);
}
protected void doWriteLZFDataFormat(
String name,
LZFDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("usingParallelCompression", def.getUsingParallelCompression());
endElement(name);
}
protected void doWriteMimeMultipartDataFormat(
String name,
MimeMultipartDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("headersInline", def.getHeadersInline());
doWriteAttribute("multipartWithoutAttachment", def.getMultipartWithoutAttachment());
doWriteAttribute("multipartSubType", def.getMultipartSubType());
doWriteAttribute("includeHeaders", def.getIncludeHeaders());
doWriteAttribute("binaryContent", def.getBinaryContent());
endElement(name);
}
protected void doWritePGPDataFormat(
String name,
PGPDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("armored", def.getArmored());
doWriteAttribute("signaturePassword", def.getSignaturePassword());
doWriteAttribute("signatureKeyRing", def.getSignatureKeyRing());
doWriteAttribute("signatureVerificationOption", def.getSignatureVerificationOption());
doWriteAttribute("keyFileName", def.getKeyFileName());
doWriteAttribute("signatureKeyUserid", def.getSignatureKeyUserid());
doWriteAttribute("integrity", def.getIntegrity());
doWriteAttribute("password", def.getPassword());
doWriteAttribute("provider", def.getProvider());
doWriteAttribute("compressionAlgorithm", def.getCompressionAlgorithm());
doWriteAttribute("keyUserid", def.getKeyUserid());
doWriteAttribute("signatureKeyFileName", def.getSignatureKeyFileName());
doWriteAttribute("hashAlgorithm", def.getHashAlgorithm());
doWriteAttribute("algorithm", def.getAlgorithm());
endElement(name);
}
protected void doWriteParquetAvroDataFormat(
String name,
ParquetAvroDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("compressionCodecName", def.getCompressionCodecName());
doWriteAttribute("unmarshalType", def.getUnmarshalTypeName());
doWriteAttribute("lazyLoad", def.getLazyLoad());
endElement(name);
}
protected void doWriteProtobufDataFormat(
String name,
ProtobufDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("contentTypeHeader", def.getContentTypeHeader());
doWriteAttribute("contentTypeFormat", def.getContentTypeFormat());
doWriteAttribute("timezone", def.getTimezone());
doWriteAttribute("moduleClassNames", def.getModuleClassNames());
doWriteAttribute("collectionType", def.getCollectionTypeName());
doWriteAttribute("allowJmsType", def.getAllowJmsType());
doWriteAttribute("jsonView", def.getJsonViewTypeName());
doWriteAttribute("enableFeatures", def.getEnableFeatures());
doWriteAttribute("library", toString(def.getLibrary()));
doWriteAttribute("autoDiscoverObjectMapper", def.getAutoDiscoverObjectMapper());
doWriteAttribute("allowUnmarshallType", def.getAllowUnmarshallType());
doWriteAttribute("include", def.getInclude());
doWriteAttribute("unmarshalType", def.getUnmarshalTypeName());
doWriteAttribute("autoDiscoverSchemaResolver", def.getAutoDiscoverSchemaResolver());
doWriteAttribute("instanceClass", def.getInstanceClass());
doWriteAttribute("useList", def.getUseList());
doWriteAttribute("disableFeatures", def.getDisableFeatures());
doWriteAttribute("moduleRefs", def.getModuleRefs());
doWriteAttribute("schemaResolver", def.getSchemaResolver());
doWriteAttribute("useDefaultObjectMapper", def.getUseDefaultObjectMapper());
doWriteAttribute("objectMapper", def.getObjectMapper());
endElement(name);
}
protected void doWriteRssDataFormat(
String name,
RssDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
endElement(name);
}
protected void doWriteSoapDataFormat(
String name,
SoapDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("schema", def.getSchema());
doWriteAttribute("namespacePrefixRef", def.getNamespacePrefixRef());
doWriteAttribute("elementNameStrategyRef", def.getElementNameStrategyRef());
doWriteAttribute("contextPath", def.getContextPath());
doWriteAttribute("encoding", def.getEncoding());
doWriteAttribute("version", def.getVersion());
endElement(name);
}
protected void doWriteSwiftMtDataFormat(
String name,
SwiftMtDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("writeInJson", def.getWriteInJson());
endElement(name);
}
protected void doWriteSwiftMxDataFormat(
String name,
SwiftMxDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("readMessageId", def.getReadMessageId());
doWriteAttribute("writeInJson", def.getWriteInJson());
doWriteAttribute("writeConfigRef", def.getWriteConfigRef());
doWriteAttribute("readConfigRef", def.getReadConfigRef());
endElement(name);
}
protected void doWriteSyslogDataFormat(
String name,
SyslogDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
endElement(name);
}
protected void doWriteTarFileDataFormat(
String name,
TarFileDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("maxDecompressedSize", def.getMaxDecompressedSize());
doWriteAttribute("usingIterator", def.getUsingIterator());
doWriteAttribute("preservePathElements", def.getPreservePathElements());
doWriteAttribute("allowEmptyDirectory", def.getAllowEmptyDirectory());
endElement(name);
}
protected void doWriteThriftDataFormat(
String name,
ThriftDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("contentTypeHeader", def.getContentTypeHeader());
doWriteAttribute("contentTypeFormat", def.getContentTypeFormat());
doWriteAttribute("instanceClass", def.getInstanceClass());
endElement(name);
}
protected void doWriteTidyMarkupDataFormat(
String name,
TidyMarkupDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("omitXmlDeclaration", def.getOmitXmlDeclaration());
doWriteAttribute("dataObjectType", def.getDataObjectTypeName());
endElement(name);
}
protected void doWriteUniVocityAbstractDataFormatAttributes(
UniVocityAbstractDataFormat def)
throws IOException {
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("headerExtractionEnabled", def.getHeaderExtractionEnabled());
doWriteAttribute("skipEmptyLines", def.getSkipEmptyLines());
doWriteAttribute("asMap", def.getAsMap());
doWriteAttribute("ignoreLeadingWhitespaces", def.getIgnoreLeadingWhitespaces());
doWriteAttribute("lineSeparator", def.getLineSeparator());
doWriteAttribute("ignoreTrailingWhitespaces", def.getIgnoreTrailingWhitespaces());
doWriteAttribute("lazyLoad", def.getLazyLoad());
doWriteAttribute("nullValue", def.getNullValue());
doWriteAttribute("normalizedLineSeparator", def.getNormalizedLineSeparator());
doWriteAttribute("emptyValue", def.getEmptyValue());
doWriteAttribute("headersDisabled", def.getHeadersDisabled());
doWriteAttribute("comment", def.getComment());
doWriteAttribute("numberOfRecordsToRead", def.getNumberOfRecordsToRead());
}
protected void doWriteUniVocityAbstractDataFormatElements(
UniVocityAbstractDataFormat def)
throws IOException {
doWriteList(null, null, def.getHeaders(), this::doWriteUniVocityHeaderRef);
}
protected void doWriteUniVocityAbstractDataFormat(
String name,
UniVocityAbstractDataFormat def)
throws IOException {
startElement(name);
doWriteUniVocityAbstractDataFormatAttributes(def);
doWriteUniVocityAbstractDataFormatElements(def);
endElement(name);
}
protected void doWriteUniVocityCsvDataFormat(
String name,
UniVocityCsvDataFormat def)
throws IOException {
startElement(name);
doWriteUniVocityAbstractDataFormatAttributes(def);
doWriteAttribute("quoteEscape", def.getQuoteEscape());
doWriteAttribute("quote", def.getQuote());
doWriteAttribute("delimiter", def.getDelimiter());
doWriteAttribute("quoteAllFields", def.getQuoteAllFields());
doWriteUniVocityAbstractDataFormatElements(def);
endElement(name);
}
protected void doWriteUniVocityFixedDataFormat(
String name,
UniVocityFixedDataFormat def)
throws IOException {
startElement(name);
doWriteUniVocityAbstractDataFormatAttributes(def);
doWriteAttribute("recordEndsOnNewline", def.getRecordEndsOnNewline());
doWriteAttribute("padding", def.getPadding());
doWriteAttribute("skipTrailingCharsUntilNewline", def.getSkipTrailingCharsUntilNewline());
doWriteUniVocityAbstractDataFormatElements(def);
endElement(name);
}
protected void doWriteUniVocityHeader(
String name,
UniVocityHeader def)
throws IOException {
startElement(name);
doWriteAttribute("length", def.getLength());
doWriteValue(def.getName());
endElement(name);
}
protected void doWriteUniVocityTsvDataFormat(
String name,
UniVocityTsvDataFormat def)
throws IOException {
startElement(name);
doWriteUniVocityAbstractDataFormatAttributes(def);
doWriteAttribute("escapeChar", def.getEscapeChar());
doWriteUniVocityAbstractDataFormatElements(def);
endElement(name);
}
protected void doWriteXMLSecurityDataFormat(
String name,
XMLSecurityDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("addKeyValueForEncryptedKey", def.getAddKeyValueForEncryptedKey());
doWriteAttribute("keyCipherAlgorithm", def.getKeyCipherAlgorithm());
doWriteAttribute("recipientKeyAlias", def.getRecipientKeyAlias());
doWriteAttribute("keyOrTrustStoreParametersRef", def.getKeyOrTrustStoreParametersRef());
doWriteAttribute("digestAlgorithm", def.getDigestAlgorithm());
doWriteAttribute("mgfAlgorithm", def.getMgfAlgorithm());
doWriteAttribute("secureTagContents", def.getSecureTagContents());
doWriteAttribute("passPhraseByte", toString(def.getPassPhraseByte()));
doWriteAttribute("keyPassword", def.getKeyPassword());
doWriteAttribute("secureTag", def.getSecureTag());
doWriteAttribute("xmlCipherAlgorithm", def.getXmlCipherAlgorithm());
doWriteAttribute("passPhrase", def.getPassPhrase());
endElement(name);
}
protected void doWriteYAMLDataFormat(
String name,
YAMLDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("resolver", def.getResolver());
doWriteAttribute("unmarshalType", def.getUnmarshalTypeName());
doWriteAttribute("prettyFlow", def.getPrettyFlow());
doWriteAttribute("allowAnyType", def.getAllowAnyType());
doWriteAttribute("representer", def.getRepresenter());
doWriteAttribute("constructor", def.getConstructor());
doWriteAttribute("library", toString(def.getLibrary()));
doWriteAttribute("maxAliasesForCollections", def.getMaxAliasesForCollections());
doWriteAttribute("dumperOptions", def.getDumperOptions());
doWriteAttribute("useApplicationContextClassLoader", def.getUseApplicationContextClassLoader());
doWriteAttribute("allowRecursiveKeys", def.getAllowRecursiveKeys());
doWriteList(null, "typeFilter", def.getTypeFilters(), this::doWriteYAMLTypeFilterDefinition);
endElement(name);
}
protected void doWriteYAMLTypeFilterDefinition(
String name,
YAMLTypeFilterDefinition def)
throws IOException {
startElement(name);
doWriteAttribute("type", def.getType());
doWriteAttribute("value", def.getValue());
endElement(name);
}
protected void doWriteZipDeflaterDataFormat(
String name,
ZipDeflaterDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("compressionLevel", def.getCompressionLevel());
endElement(name);
}
protected void doWriteZipFileDataFormat(
String name,
ZipFileDataFormat def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("maxDecompressedSize", def.getMaxDecompressedSize());
doWriteAttribute("usingIterator", def.getUsingIterator());
doWriteAttribute("preservePathElements", def.getPreservePathElements());
doWriteAttribute("allowEmptyDirectory", def.getAllowEmptyDirectory());
endElement(name);
}
protected void doWriteDeadLetterChannelDefinition(
String name,
DeadLetterChannelDefinition def)
throws IOException {
startElement(name);
doWriteDefaultErrorHandlerDefinitionAttributes(def);
doWriteAttribute("deadLetterHandleNewException", def.getDeadLetterHandleNewException());
doWriteAttribute("deadLetterUri", def.getDeadLetterUri());
doWriteDefaultErrorHandlerDefinitionElements(def);
endElement(name);
}
protected void doWriteDefaultErrorHandlerDefinitionAttributes(
DefaultErrorHandlerDefinition def)
throws IOException {
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("executorServiceRef", def.getExecutorServiceRef());
doWriteAttribute("level", def.getLevel());
doWriteAttribute("loggerRef", def.getLoggerRef());
doWriteAttribute("useOriginalMessage", def.getUseOriginalMessage());
doWriteAttribute("onRedeliveryRef", def.getOnRedeliveryRef());
doWriteAttribute("retryWhileRef", def.getRetryWhileRef());
doWriteAttribute("logName", def.getLogName());
doWriteAttribute("useOriginalBody", def.getUseOriginalBody());
doWriteAttribute("onPrepareFailureRef", def.getOnPrepareFailureRef());
doWriteAttribute("onExceptionOccurredRef", def.getOnExceptionOccurredRef());
doWriteAttribute("redeliveryPolicyRef", def.getRedeliveryPolicyRef());
}
protected void doWriteDefaultErrorHandlerDefinitionElements(
DefaultErrorHandlerDefinition def)
throws IOException {
doWriteElement("redeliveryPolicy", def.getRedeliveryPolicy(), this::doWriteRedeliveryPolicyDefinition);
}
protected void doWriteDefaultErrorHandlerDefinition(
String name,
DefaultErrorHandlerDefinition def)
throws IOException {
startElement(name);
doWriteDefaultErrorHandlerDefinitionAttributes(def);
doWriteDefaultErrorHandlerDefinitionElements(def);
endElement(name);
}
protected void doWriteJtaTransactionErrorHandlerDefinition(
String name,
JtaTransactionErrorHandlerDefinition def)
throws IOException {
startElement(name);
doWriteTransactionErrorHandlerDefinitionAttributes(def);
doWriteDefaultErrorHandlerDefinitionElements(def);
endElement(name);
}
protected void doWriteNoErrorHandlerDefinition(
String name,
NoErrorHandlerDefinition def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
endElement(name);
}
protected void doWriteRefErrorHandlerDefinition(
String name,
RefErrorHandlerDefinition def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("ref", def.getRef());
endElement(name);
}
protected void doWriteSpringTransactionErrorHandlerDefinition(
String name,
SpringTransactionErrorHandlerDefinition def)
throws IOException {
startElement(name);
doWriteTransactionErrorHandlerDefinitionAttributes(def);
doWriteDefaultErrorHandlerDefinitionElements(def);
endElement(name);
}
protected void doWriteTransactionErrorHandlerDefinitionAttributes(
TransactionErrorHandlerDefinition def)
throws IOException {
doWriteDefaultErrorHandlerDefinitionAttributes(def);
doWriteAttribute("rollbackLoggingLevel", def.getRollbackLoggingLevel());
doWriteAttribute("transactedPolicyRef", def.getTransactedPolicyRef());
}
protected void doWriteTransactionErrorHandlerDefinitionElements(
TransactionErrorHandlerDefinition def)
throws IOException {
doWriteDefaultErrorHandlerDefinitionElements(def);
}
protected void doWriteTransactionErrorHandlerDefinition(
String name,
TransactionErrorHandlerDefinition def)
throws IOException {
startElement(name);
doWriteTransactionErrorHandlerDefinitionAttributes(def);
doWriteTransactionErrorHandlerDefinitionElements(def);
endElement(name);
}
protected void doWriteCSimpleExpression(
String name,
CSimpleExpression def)
throws IOException {
startElement(name);
doWriteTypedExpressionDefinitionAttributes(def);
doWriteValue(def.getExpression());
endElement(name);
}
protected void doWriteConstantExpression(
String name,
ConstantExpression def)
throws IOException {
startElement(name);
doWriteTypedExpressionDefinitionAttributes(def);
doWriteValue(def.getExpression());
endElement(name);
}
protected void doWriteDatasonnetExpression(
String name,
DatasonnetExpression def)
throws IOException {
startElement(name);
doWriteSingleInputTypedExpressionDefinitionAttributes(def);
doWriteAttribute("outputMediaType", def.getOutputMediaType());
doWriteAttribute("bodyMediaType", def.getBodyMediaType());
doWriteValue(def.getExpression());
endElement(name);
}
protected void doWriteExchangePropertyExpression(
String name,
ExchangePropertyExpression def)
throws IOException {
startElement(name);
doWriteExpressionDefinitionAttributes(def);
doWriteValue(def.getExpression());
endElement(name);
}
protected void doWriteExpressionDefinitionAttributes(
ExpressionDefinition def)
throws IOException {
doWriteAttribute("trim", def.getTrim());
doWriteAttribute("id", def.getId());
}
protected void doWriteExpressionDefinition(
String name,
ExpressionDefinition def)
throws IOException {
startElement(name);
doWriteExpressionDefinitionAttributes(def);
doWriteValue(def.getExpression());
endElement(name);
}
protected void doWriteGroovyExpression(
String name,
GroovyExpression def)
throws IOException {
startElement(name);
doWriteTypedExpressionDefinitionAttributes(def);
doWriteValue(def.getExpression());
endElement(name);
}
protected void doWriteHeaderExpression(
String name,
HeaderExpression def)
throws IOException {
startElement(name);
doWriteExpressionDefinitionAttributes(def);
doWriteValue(def.getExpression());
endElement(name);
}
protected void doWriteHl7TerserExpression(
String name,
Hl7TerserExpression def)
throws IOException {
startElement(name);
doWriteSingleInputTypedExpressionDefinitionAttributes(def);
doWriteValue(def.getExpression());
endElement(name);
}
protected void doWriteJavaExpression(
String name,
JavaExpression def)
throws IOException {
startElement(name);
doWriteTypedExpressionDefinitionAttributes(def);
doWriteAttribute("preCompile", def.getPreCompile());
doWriteAttribute("singleQuotes", def.getSingleQuotes());
doWriteValue(def.getExpression());
endElement(name);
}
protected void doWriteJavaScriptExpression(
String name,
JavaScriptExpression def)
throws IOException {
startElement(name);
doWriteTypedExpressionDefinitionAttributes(def);
doWriteValue(def.getExpression());
endElement(name);
}
protected void doWriteJoorExpression(
String name,
JoorExpression def)
throws IOException {
startElement(name);
doWriteTypedExpressionDefinitionAttributes(def);
doWriteAttribute("preCompile", def.getPreCompile());
doWriteAttribute("singleQuotes", def.getSingleQuotes());
doWriteValue(def.getExpression());
endElement(name);
}
protected void doWriteJqExpression(
String name,
JqExpression def)
throws IOException {
startElement(name);
doWriteSingleInputTypedExpressionDefinitionAttributes(def);
doWriteValue(def.getExpression());
endElement(name);
}
protected void doWriteJsonPathExpression(
String name,
JsonPathExpression def)
throws IOException {
startElement(name);
doWriteSingleInputTypedExpressionDefinitionAttributes(def);
doWriteAttribute("unpackArray", def.getUnpackArray());
doWriteAttribute("writeAsString", def.getWriteAsString());
doWriteAttribute("allowSimple", def.getAllowSimple());
doWriteAttribute("suppressExceptions", def.getSuppressExceptions());
doWriteAttribute("allowEasyPredicate", def.getAllowEasyPredicate());
doWriteAttribute("option", def.getOption());
doWriteValue(def.getExpression());
endElement(name);
}
protected void doWriteLanguageExpression(
String name,
LanguageExpression def)
throws IOException {
startElement(name);
doWriteExpressionDefinitionAttributes(def);
doWriteAttribute("language", def.getLanguage());
doWriteValue(def.getExpression());
endElement(name);
}
protected void doWriteMethodCallExpression(
String name,
MethodCallExpression def)
throws IOException {
startElement(name);
doWriteTypedExpressionDefinitionAttributes(def);
doWriteAttribute("ref", def.getRef());
doWriteAttribute("method", def.getMethod());
doWriteAttribute("scope", def.getScope());
doWriteAttribute("beanType", def.getBeanTypeName());
doWriteAttribute("validate", def.getValidate());
doWriteValue(def.getExpression());
endElement(name);
}
protected void doWriteMvelExpression(
String name,
MvelExpression def)
throws IOException {
startElement(name);
doWriteTypedExpressionDefinitionAttributes(def);
doWriteValue(def.getExpression());
endElement(name);
}
protected void doWriteNamespaceAwareExpressionAttributes(
NamespaceAwareExpression def)
throws IOException {
doWriteSingleInputTypedExpressionDefinitionAttributes(def);
}
protected void doWriteNamespaceAwareExpressionElements(
NamespaceAwareExpression def)
throws IOException {
doWriteList(null, "namespace", def.getNamespace(), this::doWritePropertyDefinition);
}
protected void doWriteNamespaceAwareExpression(
String name,
NamespaceAwareExpression def)
throws IOException {
startElement(name);
doWriteNamespaceAwareExpressionAttributes(def);
doWriteValue(def.getExpression());
doWriteNamespaceAwareExpressionElements(def);
endElement(name);
}
protected void doWriteOgnlExpression(
String name,
OgnlExpression def)
throws IOException {
startElement(name);
doWriteTypedExpressionDefinitionAttributes(def);
doWriteValue(def.getExpression());
endElement(name);
}
protected void doWritePythonExpression(
String name,
PythonExpression def)
throws IOException {
startElement(name);
doWriteTypedExpressionDefinitionAttributes(def);
doWriteValue(def.getExpression());
endElement(name);
}
protected void doWriteRefExpression(
String name,
RefExpression def)
throws IOException {
startElement(name);
doWriteTypedExpressionDefinitionAttributes(def);
doWriteValue(def.getExpression());
endElement(name);
}
protected void doWriteSimpleExpression(
String name,
SimpleExpression def)
throws IOException {
startElement(name);
doWriteTypedExpressionDefinitionAttributes(def);
doWriteValue(def.getExpression());
endElement(name);
}
protected void doWriteSingleInputTypedExpressionDefinitionAttributes(
SingleInputTypedExpressionDefinition def)
throws IOException {
doWriteTypedExpressionDefinitionAttributes(def);
doWriteAttribute("source", def.getSource());
}
protected void doWriteSingleInputTypedExpressionDefinition(
String name,
SingleInputTypedExpressionDefinition def)
throws IOException {
startElement(name);
doWriteSingleInputTypedExpressionDefinitionAttributes(def);
doWriteValue(def.getExpression());
endElement(name);
}
protected void doWriteSpELExpression(
String name,
SpELExpression def)
throws IOException {
startElement(name);
doWriteTypedExpressionDefinitionAttributes(def);
doWriteValue(def.getExpression());
endElement(name);
}
protected void doWriteTokenizerExpression(
String name,
TokenizerExpression def)
throws IOException {
startElement(name);
doWriteSingleInputTypedExpressionDefinitionAttributes(def);
doWriteAttribute("regex", def.getRegex());
doWriteAttribute("endToken", def.getEndToken());
doWriteAttribute("includeTokens", def.getIncludeTokens());
doWriteAttribute("skipFirst", def.getSkipFirst());
doWriteAttribute("xml", def.getXml());
doWriteAttribute("inheritNamespaceTagName", def.getInheritNamespaceTagName());
doWriteAttribute("groupDelimiter", def.getGroupDelimiter());
doWriteAttribute("group", def.getGroup());
doWriteAttribute("token", def.getToken());
doWriteValue(def.getExpression());
endElement(name);
}
protected void doWriteTypedExpressionDefinitionAttributes(
TypedExpressionDefinition def)
throws IOException {
doWriteExpressionDefinitionAttributes(def);
doWriteAttribute("resultType", def.getResultTypeName());
}
protected void doWriteTypedExpressionDefinition(
String name,
TypedExpressionDefinition def)
throws IOException {
startElement(name);
doWriteTypedExpressionDefinitionAttributes(def);
doWriteValue(def.getExpression());
endElement(name);
}
protected void doWriteVariableExpression(
String name,
VariableExpression def)
throws IOException {
startElement(name);
doWriteExpressionDefinitionAttributes(def);
doWriteValue(def.getExpression());
endElement(name);
}
protected void doWriteWasmExpression(
String name,
WasmExpression def)
throws IOException {
startElement(name);
doWriteTypedExpressionDefinitionAttributes(def);
doWriteAttribute("module", def.getModule());
doWriteValue(def.getExpression());
endElement(name);
}
protected void doWriteXMLTokenizerExpression(
String name,
XMLTokenizerExpression def)
throws IOException {
startElement(name);
doWriteSingleInputTypedExpressionDefinitionAttributes(def);
doWriteAttribute("mode", def.getMode());
doWriteAttribute("group", def.getGroup());
doWriteNamespaces(def);
doWriteValue(def.getExpression());
endElement(name);
}
protected void doWriteXPathExpression(
String name,
XPathExpression def)
throws IOException {
startElement(name);
doWriteSingleInputTypedExpressionDefinitionAttributes(def);
doWriteAttribute("preCompile", def.getPreCompile());
doWriteAttribute("objectModel", def.getObjectModel());
doWriteAttribute("logNamespaces", def.getLogNamespaces());
doWriteAttribute("threadSafety", def.getThreadSafety());
doWriteAttribute("factoryRef", def.getFactoryRef());
doWriteAttribute("resultQName", def.getResultQName());
doWriteAttribute("saxon", def.getSaxon());
doWriteAttribute("documentType", def.getDocumentTypeName());
doWriteNamespaces(def);
doWriteValue(def.getExpression());
endElement(name);
}
protected void doWriteXQueryExpression(
String name,
XQueryExpression def)
throws IOException {
startElement(name);
doWriteSingleInputTypedExpressionDefinitionAttributes(def);
doWriteAttribute("configurationRef", def.getConfigurationRef());
doWriteNamespaces(def);
doWriteValue(def.getExpression());
endElement(name);
}
protected void doWriteCustomLoadBalancerDefinition(
String name,
CustomLoadBalancerDefinition def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("ref", def.getRef());
endElement(name);
}
protected void doWriteFailoverLoadBalancerDefinition(
String name,
FailoverLoadBalancerDefinition def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("sticky", def.getSticky());
doWriteAttribute("maximumFailoverAttempts", def.getMaximumFailoverAttempts());
doWriteAttribute("roundRobin", def.getRoundRobin());
doWriteList(null, "exception", def.getExceptions(), this::doWriteString);
endElement(name);
}
protected void doWriteRandomLoadBalancerDefinition(
String name,
RandomLoadBalancerDefinition def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
endElement(name);
}
protected void doWriteRoundRobinLoadBalancerDefinition(
String name,
RoundRobinLoadBalancerDefinition def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
endElement(name);
}
protected void doWriteStickyLoadBalancerDefinition(
String name,
StickyLoadBalancerDefinition def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteElement("correlationExpression", def.getCorrelationExpression(), this::doWriteExpressionSubElementDefinition);
endElement(name);
}
protected void doWriteTopicLoadBalancerDefinition(
String name,
TopicLoadBalancerDefinition def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
endElement(name);
}
protected void doWriteWeightedLoadBalancerDefinition(
String name,
WeightedLoadBalancerDefinition def)
throws IOException {
startElement(name);
doWriteIdentifiedTypeAttributes(def);
doWriteAttribute("distributionRatioDelimiter", def.getDistributionRatioDelimiter());
doWriteAttribute("distributionRatio", def.getDistributionRatio());
doWriteAttribute("roundRobin", def.getRoundRobin());
endElement(name);
}
protected void doWriteApiKeyDefinition(
String name,
ApiKeyDefinition def)
throws IOException {
startElement(name);
doWriteRestSecurityDefinitionAttributes(def);
doWriteAttribute("inHeader", def.getInHeader());
doWriteAttribute("inCookie", def.getInCookie());
doWriteAttribute("name", def.getName());
doWriteAttribute("inQuery", def.getInQuery());
endElement(name);
}
protected void doWriteBasicAuthDefinition(
String name,
BasicAuthDefinition def)
throws IOException {
startElement(name);
doWriteRestSecurityDefinitionAttributes(def);
endElement(name);
}
protected void doWriteBearerTokenDefinition(
String name,
BearerTokenDefinition def)
throws IOException {
startElement(name);
doWriteRestSecurityDefinitionAttributes(def);
doWriteAttribute("format", def.getFormat());
endElement(name);
}
protected void doWriteDeleteDefinition(
String name,
DeleteDefinition def)
throws IOException {
startElement(name);
doWriteVerbDefinitionAttributes(def);
doWriteVerbDefinitionElements(def);
endElement(name);
}
protected void doWriteGetDefinition(
String name,
GetDefinition def)
throws IOException {
startElement(name);
doWriteVerbDefinitionAttributes(def);
doWriteVerbDefinitionElements(def);
endElement(name);
}
protected void doWriteHeadDefinition(
String name,
HeadDefinition def)
throws IOException {
startElement(name);
doWriteVerbDefinitionAttributes(def);
doWriteVerbDefinitionElements(def);
endElement(name);
}
protected void doWriteMutualTLSDefinition(
String name,
MutualTLSDefinition def)
throws IOException {
startElement(name);
doWriteRestSecurityDefinitionAttributes(def);
endElement(name);
}
protected void doWriteOAuth2Definition(
String name,
OAuth2Definition def)
throws IOException {
startElement(name);
doWriteRestSecurityDefinitionAttributes(def);
doWriteAttribute("tokenUrl", def.getTokenUrl());
doWriteAttribute("authorizationUrl", def.getAuthorizationUrl());
doWriteAttribute("refreshUrl", def.getRefreshUrl());
doWriteAttribute("flow", def.getFlow());
doWriteList(null, "scopes", def.getScopes(), this::doWriteRestPropertyDefinition);
endElement(name);
}
protected void doWriteOpenIdConnectDefinition(
String name,
OpenIdConnectDefinition def)
throws IOException {
startElement(name);
doWriteRestSecurityDefinitionAttributes(def);
doWriteAttribute("url", def.getUrl());
endElement(name);
}
protected void doWriteParamDefinition(
String name,
ParamDefinition def)
throws IOException {
startElement(name);
doWriteAttribute("arrayType", def.getArrayType());
doWriteAttribute("dataFormat", def.getDataFormat());
doWriteAttribute("defaultValue", def.getDefaultValue());
doWriteAttribute("dataType", def.getDataType());
doWriteAttribute("name", def.getName());
doWriteAttribute("description", def.getDescription());
doWriteAttribute("type", toString(def.getType()));
doWriteAttribute("collectionFormat", toString(def.getCollectionFormat()));
doWriteAttribute("required", toString(def.getRequired()));
doWriteList("allowableValues", "value", def.getAllowableValues(), this::doWriteValueDefinition);
doWriteList(null, "examples", def.getExamples(), this::doWriteRestPropertyDefinition);
endElement(name);
}
protected void doWritePatchDefinition(
String name,
PatchDefinition def)
throws IOException {
startElement(name);
doWriteVerbDefinitionAttributes(def);
doWriteVerbDefinitionElements(def);
endElement(name);
}
protected void doWritePostDefinition(
String name,
PostDefinition def)
throws IOException {
startElement(name);
doWriteVerbDefinitionAttributes(def);
doWriteVerbDefinitionElements(def);
endElement(name);
}
protected void doWritePutDefinition(
String name,
PutDefinition def)
throws IOException {
startElement(name);
doWriteVerbDefinitionAttributes(def);
doWriteVerbDefinitionElements(def);
endElement(name);
}
protected void doWriteResponseHeaderDefinition(
String name,
ResponseHeaderDefinition def)
throws IOException {
startElement(name);
doWriteAttribute("arrayType", def.getArrayType());
doWriteAttribute("dataFormat", def.getDataFormat());
doWriteAttribute("dataType", def.getDataType());
doWriteAttribute("name", def.getName());
doWriteAttribute("description", def.getDescription());
doWriteAttribute("collectionFormat", toString(def.getCollectionFormat()));
doWriteAttribute("example", def.getExample());
doWriteList("allowableValues", "value", def.getAllowableValues(), this::doWriteValueDefinition);
endElement(name);
}
protected void doWriteResponseMessageDefinition(
String name,
ResponseMessageDefinition def)
throws IOException {
startElement(name);
doWriteAttribute("code", def.getCode());
doWriteAttribute("responseModel", def.getResponseModel());
doWriteAttribute("message", def.getMessage());
doWriteList(null, "header", def.getHeaders(), this::doWriteResponseHeaderDefinition);
doWriteList(null, "examples", def.getExamples(), this::doWriteRestPropertyDefinition);
endElement(name);
}
protected void doWriteRestBindingDefinition(
String name,
RestBindingDefinition def)
throws IOException {
startElement(name);
doWriteOptionalIdentifiedDefinitionAttributes(def);
doWriteAttribute("enableCORS", def.getEnableCORS());
doWriteAttribute("type", def.getType());
doWriteAttribute("outType", def.getOutType());
doWriteAttribute("component", def.getComponent());
doWriteAttribute("bindingMode", def.getBindingMode());
doWriteAttribute("enableNoContentResponse", def.getEnableNoContentResponse());
doWriteAttribute("skipBindingOnErrorCode", def.getSkipBindingOnErrorCode());
doWriteAttribute("clientRequestValidation", def.getClientRequestValidation());
doWriteAttribute("produces", def.getProduces());
doWriteAttribute("consumes", def.getConsumes());
endElement(name);
}
protected void doWriteRestConfigurationDefinition(
String name,
RestConfigurationDefinition def)
throws IOException {
startElement(name);
doWriteAttribute("scheme", def.getScheme());
doWriteAttribute("inlineRoutes", def.getInlineRoutes());
doWriteAttribute("apiContextPath", def.getApiContextPath());
doWriteAttribute("hostNameResolver", toString(def.getHostNameResolver()));
doWriteAttribute("skipBindingOnErrorCode", def.getSkipBindingOnErrorCode());
doWriteAttribute("clientRequestValidation", def.getClientRequestValidation());
doWriteAttribute("producerApiDoc", def.getProducerApiDoc());
doWriteAttribute("host", def.getHost());
doWriteAttribute("producerComponent", def.getProducerComponent());
doWriteAttribute("enableCORS", def.getEnableCORS());
doWriteAttribute("useXForwardHeaders", def.getUseXForwardHeaders());
doWriteAttribute("apiHost", def.getApiHost());
doWriteAttribute("contextPath", def.getContextPath());
doWriteAttribute("apiContextRouteId", def.getApiContextRouteId());
doWriteAttribute("component", def.getComponent());
doWriteAttribute("bindingMode", toString(def.getBindingMode()));
doWriteAttribute("port", def.getPort());
doWriteAttribute("enableNoContentResponse", def.getEnableNoContentResponse());
doWriteAttribute("xmlDataFormat", def.getXmlDataFormat());
doWriteAttribute("apiVendorExtension", def.getApiVendorExtension());
doWriteAttribute("apiComponent", def.getApiComponent());
doWriteAttribute("jsonDataFormat", def.getJsonDataFormat());
doWriteList(null, "consumerProperty", def.getConsumerProperties(), this::doWriteRestPropertyDefinition);
doWriteList(null, "corsHeaders", def.getCorsHeaders(), this::doWriteRestPropertyDefinition);
doWriteList(null, "componentProperty", def.getComponentProperties(), this::doWriteRestPropertyDefinition);
doWriteList(null, "apiProperty", def.getApiProperties(), this::doWriteRestPropertyDefinition);
doWriteList(null, "endpointProperty", def.getEndpointProperties(), this::doWriteRestPropertyDefinition);
doWriteList(null, "dataFormatProperty", def.getDataFormatProperties(), this::doWriteRestPropertyDefinition);
endElement(name);
}
protected void doWriteRestDefinition(
String name,
RestDefinition def)
throws IOException {
startElement(name);
doWriteOptionalIdentifiedDefinitionAttributes(def);
doWriteAttribute("enableCORS", def.getEnableCORS());
doWriteAttribute("path", def.getPath());
doWriteAttribute("bindingMode", def.getBindingMode());
doWriteAttribute("apiDocs", def.getApiDocs());
doWriteAttribute("enableNoContentResponse", def.getEnableNoContentResponse());
doWriteAttribute("skipBindingOnErrorCode", def.getSkipBindingOnErrorCode());
doWriteAttribute("clientRequestValidation", def.getClientRequestValidation());
doWriteAttribute("produces", def.getProduces());
doWriteAttribute("disabled", def.getDisabled());
doWriteAttribute("tag", def.getTag());
doWriteAttribute("consumes", def.getConsumes());
doWriteList(null, "securityRequirements", def.getSecurityRequirements(), this::doWriteSecurityDefinition);
doWriteList(null, null, def.getVerbs(), this::doWriteVerbDefinitionRef);
doWriteElement("securityDefinitions", def.getSecurityDefinitions(), this::doWriteRestSecuritiesDefinition);
endElement(name);
}
protected void doWriteRestPropertyDefinition(
String name,
RestPropertyDefinition def)
throws IOException {
startElement(name);
doWriteAttribute("value", def.getValue());
doWriteAttribute("key", def.getKey());
endElement(name);
}
protected void doWriteRestSecuritiesDefinition(
String name,
RestSecuritiesDefinition def)
throws IOException {
startElement(name);
doWriteList(null, null, def.getSecurityDefinitions(), (n, v) -> {
switch (v.getClass().getSimpleName()) {
case "ApiKeyDefinition" -> doWriteApiKeyDefinition("apiKey", (ApiKeyDefinition) v);
case "BasicAuthDefinition" -> doWriteBasicAuthDefinition("basicAuth", (BasicAuthDefinition) v);
case "BearerTokenDefinition" -> doWriteBearerTokenDefinition("bearer", (BearerTokenDefinition) v);
case "OAuth2Definition" -> doWriteOAuth2Definition("oauth2", (OAuth2Definition) v);
case "OpenIdConnectDefinition" -> doWriteOpenIdConnectDefinition("openIdConnect", (OpenIdConnectDefinition) v);
case "MutualTLSDefinition" -> doWriteMutualTLSDefinition("mutualTLS", (MutualTLSDefinition) v);
}
});
endElement(name);
}
protected void doWriteRestSecurityDefinitionAttributes(
RestSecurityDefinition def)
throws IOException {
doWriteAttribute("description", def.getDescription());
doWriteAttribute("key", def.getKey());
}
protected void doWriteRestSecurityDefinition(
String name,
RestSecurityDefinition def)
throws IOException {
startElement(name);
doWriteRestSecurityDefinitionAttributes(def);
endElement(name);
}
protected void doWriteRestsDefinition(
String name,
RestsDefinition def)
throws IOException {
startElement(name);
doWriteOptionalIdentifiedDefinitionAttributes(def);
doWriteList(null, null, def.getRests(), this::doWriteRestDefinitionRef);
endElement(name);
}
protected void doWriteSecurityDefinition(
String name,
SecurityDefinition def)
throws IOException {
startElement(name);
doWriteAttribute("scopes", def.getScopes());
doWriteAttribute("key", def.getKey());
endElement(name);
}
protected void doWriteVerbDefinitionAttributes(
VerbDefinition def)
throws IOException {
doWriteOptionalIdentifiedDefinitionAttributes(def);
doWriteAttribute("enableCORS", def.getEnableCORS());
doWriteAttribute("deprecated", def.getDeprecated());
doWriteAttribute("type", def.getType());
doWriteAttribute("outType", def.getOutType());
doWriteAttribute("path", def.getPath());
doWriteAttribute("routeId", def.getRouteId());
doWriteAttribute("bindingMode", def.getBindingMode());
doWriteAttribute("apiDocs", def.getApiDocs());
doWriteAttribute("enableNoContentResponse", def.getEnableNoContentResponse());
doWriteAttribute("skipBindingOnErrorCode", def.getSkipBindingOnErrorCode());
doWriteAttribute("clientRequestValidation", def.getClientRequestValidation());
doWriteAttribute("produces", def.getProduces());
doWriteAttribute("disabled", def.getDisabled());
doWriteAttribute("consumes", def.getConsumes());
}
protected void doWriteVerbDefinitionElements(
VerbDefinition def)
throws IOException {
doWriteList(null, null, def.getParams(), this::doWriteParamDefinitionRef);
doWriteList(null, null, def.getSecurity(), this::doWriteSecurityDefinitionRef);
doWriteList(null, null, def.getResponseMsgs(), this::doWriteResponseMessageDefinitionRef);
doWriteElement("to", def.getTo(), this::doWriteToDefinition);
}
protected void doWriteVerbDefinition(
String name,
VerbDefinition def)
throws IOException {
startElement(name);
doWriteVerbDefinitionAttributes(def);
doWriteVerbDefinitionElements(def);
endElement(name);
}
protected void doWriteCustomTransformerDefinition(
String name,
CustomTransformerDefinition def)
throws IOException {
startElement(name);
doWriteTransformerDefinitionAttributes(def);
doWriteAttribute("ref", def.getRef());
doWriteAttribute("className", def.getClassName());
endElement(name);
}
protected void doWriteDataFormatTransformerDefinition(
String name,
DataFormatTransformerDefinition def)
throws IOException {
startElement(name);
doWriteTransformerDefinitionAttributes(def);
doWriteElement(null, def.getDataFormatType(), (n, v) -> {
switch (v.getClass().getSimpleName()) {
case "ASN1DataFormat" -> doWriteASN1DataFormat("asn1", (ASN1DataFormat) def.getDataFormatType());
case "AvroDataFormat" -> doWriteAvroDataFormat("avro", (AvroDataFormat) def.getDataFormatType());
case "BarcodeDataFormat" -> doWriteBarcodeDataFormat("barcode", (BarcodeDataFormat) def.getDataFormatType());
case "Base64DataFormat" -> doWriteBase64DataFormat("base64", (Base64DataFormat) def.getDataFormatType());
case "BeanioDataFormat" -> doWriteBeanioDataFormat("beanio", (BeanioDataFormat) def.getDataFormatType());
case "BindyDataFormat" -> doWriteBindyDataFormat("bindy", (BindyDataFormat) def.getDataFormatType());
case "CBORDataFormat" -> doWriteCBORDataFormat("cbor", (CBORDataFormat) def.getDataFormatType());
case "CryptoDataFormat" -> doWriteCryptoDataFormat("crypto", (CryptoDataFormat) def.getDataFormatType());
case "CsvDataFormat" -> doWriteCsvDataFormat("csv", (CsvDataFormat) def.getDataFormatType());
case "CustomDataFormat" -> doWriteCustomDataFormat("custom", (CustomDataFormat) def.getDataFormatType());
case "FhirJsonDataFormat" -> doWriteFhirJsonDataFormat("fhirJson", (FhirJsonDataFormat) def.getDataFormatType());
case "FhirXmlDataFormat" -> doWriteFhirXmlDataFormat("fhirXml", (FhirXmlDataFormat) def.getDataFormatType());
case "FlatpackDataFormat" -> doWriteFlatpackDataFormat("flatpack", (FlatpackDataFormat) def.getDataFormatType());
case "GrokDataFormat" -> doWriteGrokDataFormat("grok", (GrokDataFormat) def.getDataFormatType());
case "GzipDeflaterDataFormat" -> doWriteGzipDeflaterDataFormat("gzipDeflater", (GzipDeflaterDataFormat) def.getDataFormatType());
case "HL7DataFormat" -> doWriteHL7DataFormat("hl7", (HL7DataFormat) def.getDataFormatType());
case "IcalDataFormat" -> doWriteIcalDataFormat("ical", (IcalDataFormat) def.getDataFormatType());
case "JacksonXMLDataFormat" -> doWriteJacksonXMLDataFormat("jacksonXml", (JacksonXMLDataFormat) def.getDataFormatType());
case "JaxbDataFormat" -> doWriteJaxbDataFormat("jaxb", (JaxbDataFormat) def.getDataFormatType());
case "JsonDataFormat" -> doWriteJsonDataFormat("json", (JsonDataFormat) def.getDataFormatType());
case "JsonApiDataFormat" -> doWriteJsonApiDataFormat("jsonApi", (JsonApiDataFormat) def.getDataFormatType());
case "LZFDataFormat" -> doWriteLZFDataFormat("lzf", (LZFDataFormat) def.getDataFormatType());
case "MimeMultipartDataFormat" -> doWriteMimeMultipartDataFormat("mimeMultipart", (MimeMultipartDataFormat) def.getDataFormatType());
case "ParquetAvroDataFormat" -> doWriteParquetAvroDataFormat("parquetAvro", (ParquetAvroDataFormat) def.getDataFormatType());
case "ProtobufDataFormat" -> doWriteProtobufDataFormat("protobuf", (ProtobufDataFormat) def.getDataFormatType());
case "RssDataFormat" -> doWriteRssDataFormat("rss", (RssDataFormat) def.getDataFormatType());
case "SoapDataFormat" -> doWriteSoapDataFormat("soap", (SoapDataFormat) def.getDataFormatType());
case "SwiftMtDataFormat" -> doWriteSwiftMtDataFormat("swiftMt", (SwiftMtDataFormat) def.getDataFormatType());
case "SwiftMxDataFormat" -> doWriteSwiftMxDataFormat("swiftMx", (SwiftMxDataFormat) def.getDataFormatType());
case "SyslogDataFormat" -> doWriteSyslogDataFormat("syslog", (SyslogDataFormat) def.getDataFormatType());
case "TarFileDataFormat" -> doWriteTarFileDataFormat("tarFile", (TarFileDataFormat) def.getDataFormatType());
case "ThriftDataFormat" -> doWriteThriftDataFormat("thrift", (ThriftDataFormat) def.getDataFormatType());
case "TidyMarkupDataFormat" -> doWriteTidyMarkupDataFormat("tidyMarkup", (TidyMarkupDataFormat) def.getDataFormatType());
case "UniVocityCsvDataFormat" -> doWriteUniVocityCsvDataFormat("univocityCsv", (UniVocityCsvDataFormat) def.getDataFormatType());
case "UniVocityFixedDataFormat" -> doWriteUniVocityFixedDataFormat("univocityFixed", (UniVocityFixedDataFormat) def.getDataFormatType());
case "UniVocityTsvDataFormat" -> doWriteUniVocityTsvDataFormat("univocityTsv", (UniVocityTsvDataFormat) def.getDataFormatType());
case "XMLSecurityDataFormat" -> doWriteXMLSecurityDataFormat("xmlSecurity", (XMLSecurityDataFormat) def.getDataFormatType());
case "PGPDataFormat" -> doWritePGPDataFormat("pgp", (PGPDataFormat) def.getDataFormatType());
case "YAMLDataFormat" -> doWriteYAMLDataFormat("yaml", (YAMLDataFormat) def.getDataFormatType());
case "ZipDeflaterDataFormat" -> doWriteZipDeflaterDataFormat("zipDeflater", (ZipDeflaterDataFormat) def.getDataFormatType());
case "ZipFileDataFormat" -> doWriteZipFileDataFormat("zipFile", (ZipFileDataFormat) def.getDataFormatType());
}
});
endElement(name);
}
protected void doWriteEndpointTransformerDefinition(
String name,
EndpointTransformerDefinition def)
throws IOException {
startElement(name);
doWriteTransformerDefinitionAttributes(def);
doWriteAttribute("ref", def.getRef());
doWriteAttribute("uri", def.getUri());
endElement(name);
}
protected void doWriteLoadTransformerDefinition(
String name,
LoadTransformerDefinition def)
throws IOException {
startElement(name);
doWriteTransformerDefinitionAttributes(def);
doWriteAttribute("defaults", def.getDefaults());
doWriteAttribute("packageScan", def.getPackageScan());
endElement(name);
}
protected void doWriteTransformerDefinitionAttributes(
TransformerDefinition def)
throws IOException {
doWriteAttribute("toType", def.getToType());
doWriteAttribute("fromType", def.getFromType());
doWriteAttribute("scheme", def.getScheme());
doWriteAttribute("name", def.getName());
}
protected void doWriteTransformerDefinition(
String name,
TransformerDefinition def)
throws IOException {
startElement(name);
doWriteTransformerDefinitionAttributes(def);
endElement(name);
}
protected void doWriteTransformersDefinition(
String name,
TransformersDefinition def)
throws IOException {
startElement(name);
doWriteList(null, null, def.getTransformers(), (n, v) -> {
switch (v.getClass().getSimpleName()) {
case "DataFormatTransformerDefinition" -> doWriteDataFormatTransformerDefinition("dataFormatTransformer", (DataFormatTransformerDefinition) v);
case "EndpointTransformerDefinition" -> doWriteEndpointTransformerDefinition("endpointTransformer", (EndpointTransformerDefinition) v);
case "LoadTransformerDefinition" -> doWriteLoadTransformerDefinition("loadTransformer", (LoadTransformerDefinition) v);
case "CustomTransformerDefinition" -> doWriteCustomTransformerDefinition("customTransformer", (CustomTransformerDefinition) v);
}
});
endElement(name);
}
protected void doWriteCustomValidatorDefinition(
String name,
CustomValidatorDefinition def)
throws IOException {
startElement(name);
doWriteValidatorDefinitionAttributes(def);
doWriteAttribute("ref", def.getRef());
doWriteAttribute("className", def.getClassName());
endElement(name);
}
protected void doWriteEndpointValidatorDefinition(
String name,
EndpointValidatorDefinition def)
throws IOException {
startElement(name);
doWriteValidatorDefinitionAttributes(def);
doWriteAttribute("ref", def.getRef());
doWriteAttribute("uri", def.getUri());
endElement(name);
}
protected void doWritePredicateValidatorDefinition(
String name,
PredicateValidatorDefinition def)
throws IOException {
startElement(name);
doWriteValidatorDefinitionAttributes(def);
doWriteElement(null, def.getExpression(), this::doWriteExpressionDefinitionRef);
endElement(name);
}
protected void doWriteValidatorDefinitionAttributes(
ValidatorDefinition def)
throws IOException {
doWriteAttribute("type", def.getType());
}
protected void doWriteValidatorDefinition(
String name,
ValidatorDefinition def)
throws IOException {
startElement(name);
doWriteValidatorDefinitionAttributes(def);
endElement(name);
}
protected void doWriteValidatorsDefinition(
String name,
ValidatorsDefinition def)
throws IOException {
startElement(name);
doWriteList(null, null, def.getValidators(), (n, v) -> {
switch (v.getClass().getSimpleName()) {
case "EndpointValidatorDefinition" -> doWriteEndpointValidatorDefinition("endpointValidator", (EndpointValidatorDefinition) v);
case "PredicateValidatorDefinition" -> doWritePredicateValidatorDefinition("predicateValidator", (PredicateValidatorDefinition) v);
case "CustomValidatorDefinition" -> doWriteCustomValidatorDefinition("customValidator", (CustomValidatorDefinition) v);
}
});
endElement(name);
}
protected void doWriteFromDefinitionRef(
String n,
FromDefinition v)
throws IOException {
if (v != null) {
switch (v.getClass().getSimpleName()) {
case "FromDefinition" -> doWriteFromDefinition("from", (FromDefinition) v);
}
}
}
protected void doWriteInputTypeDefinitionRef(
String n,
InputTypeDefinition v)
throws IOException {
if (v != null) {
switch (v.getClass().getSimpleName()) {
case "InputTypeDefinition" -> doWriteInputTypeDefinition("inputType", (InputTypeDefinition) v);
}
}
}
protected void doWriteOptionalIdentifiedDefinitionRef(
String n,
OptionalIdentifiedDefinition> v)
throws IOException {
if (v != null) {
switch (v.getClass().getSimpleName()) {
case "AggregateDefinition" -> doWriteAggregateDefinition("aggregate", (AggregateDefinition) v);
case "BeanDefinition" -> doWriteBeanDefinition("bean", (BeanDefinition) v);
case "CatchDefinition" -> doWriteCatchDefinition("doCatch", (CatchDefinition) v);
case "ChoiceDefinition" -> doWriteChoiceDefinition("choice", (ChoiceDefinition) v);
case "CircuitBreakerDefinition" -> doWriteCircuitBreakerDefinition("circuitBreaker", (CircuitBreakerDefinition) v);
case "ClaimCheckDefinition" -> doWriteClaimCheckDefinition("claimCheck", (ClaimCheckDefinition) v);
case "ConvertBodyDefinition" -> doWriteConvertBodyDefinition("convertBodyTo", (ConvertBodyDefinition) v);
case "ConvertHeaderDefinition" -> doWriteConvertHeaderDefinition("convertHeaderTo", (ConvertHeaderDefinition) v);
case "ConvertVariableDefinition" -> doWriteConvertVariableDefinition("convertVariableTo", (ConvertVariableDefinition) v);
case "DelayDefinition" -> doWriteDelayDefinition("delay", (DelayDefinition) v);
case "DynamicRouterDefinition" -> doWriteDynamicRouterDefinition("dynamicRouter", (DynamicRouterDefinition) v);
case "EnrichDefinition" -> doWriteEnrichDefinition("enrich", (EnrichDefinition) v);
case "FilterDefinition" -> doWriteFilterDefinition("filter", (FilterDefinition) v);
case "FinallyDefinition" -> doWriteFinallyDefinition("doFinally", (FinallyDefinition) v);
case "FromDefinition" -> doWriteFromDefinition("from", (FromDefinition) v);
case "IdempotentConsumerDefinition" -> doWriteIdempotentConsumerDefinition("idempotentConsumer", (IdempotentConsumerDefinition) v);
case "InputTypeDefinition" -> doWriteInputTypeDefinition("inputType", (InputTypeDefinition) v);
case "InterceptDefinition" -> doWriteInterceptDefinition("intercept", (InterceptDefinition) v);
case "InterceptFromDefinition" -> doWriteInterceptFromDefinition("interceptFrom", (InterceptFromDefinition) v);
case "InterceptSendToEndpointDefinition" -> doWriteInterceptSendToEndpointDefinition("interceptSendToEndpoint", (InterceptSendToEndpointDefinition) v);
case "KameletDefinition" -> doWriteKameletDefinition("kamelet", (KameletDefinition) v);
case "LoadBalanceDefinition" -> doWriteLoadBalanceDefinition("loadBalance", (LoadBalanceDefinition) v);
case "LogDefinition" -> doWriteLogDefinition("log", (LogDefinition) v);
case "LoopDefinition" -> doWriteLoopDefinition("loop", (LoopDefinition) v);
case "MarshalDefinition" -> doWriteMarshalDefinition("marshal", (MarshalDefinition) v);
case "MulticastDefinition" -> doWriteMulticastDefinition("multicast", (MulticastDefinition) v);
case "OnCompletionDefinition" -> doWriteOnCompletionDefinition("onCompletion", (OnCompletionDefinition) v);
case "OnExceptionDefinition" -> doWriteOnExceptionDefinition("onException", (OnExceptionDefinition) v);
case "OnFallbackDefinition" -> doWriteOnFallbackDefinition("onFallback", (OnFallbackDefinition) v);
case "OtherwiseDefinition" -> doWriteOtherwiseDefinition("otherwise", (OtherwiseDefinition) v);
case "OutputTypeDefinition" -> doWriteOutputTypeDefinition("outputType", (OutputTypeDefinition) v);
case "PausableDefinition" -> doWritePausableDefinition("pausable", (PausableDefinition) v);
case "PipelineDefinition" -> doWritePipelineDefinition("pipeline", (PipelineDefinition) v);
case "PolicyDefinition" -> doWritePolicyDefinition("policy", (PolicyDefinition) v);
case "PollEnrichDefinition" -> doWritePollEnrichDefinition("pollEnrich", (PollEnrichDefinition) v);
case "ProcessDefinition" -> doWriteProcessDefinition("process", (ProcessDefinition) v);
case "RecipientListDefinition" -> doWriteRecipientListDefinition("recipientList", (RecipientListDefinition) v);
case "RemoveHeaderDefinition" -> doWriteRemoveHeaderDefinition("removeHeader", (RemoveHeaderDefinition) v);
case "RemoveHeadersDefinition" -> doWriteRemoveHeadersDefinition("removeHeaders", (RemoveHeadersDefinition) v);
case "RemovePropertiesDefinition" -> doWriteRemovePropertiesDefinition("removeProperties", (RemovePropertiesDefinition) v);
case "RemovePropertyDefinition" -> doWriteRemovePropertyDefinition("removeProperty", (RemovePropertyDefinition) v);
case "RemoveVariableDefinition" -> doWriteRemoveVariableDefinition("removeVariable", (RemoveVariableDefinition) v);
case "ResequenceDefinition" -> doWriteResequenceDefinition("resequence", (ResequenceDefinition) v);
case "ResumableDefinition" -> doWriteResumableDefinition("resumable", (ResumableDefinition) v);
case "RollbackDefinition" -> doWriteRollbackDefinition("rollback", (RollbackDefinition) v);
case "RouteConfigurationDefinition" -> doWriteRouteConfigurationDefinition("routeConfiguration", (RouteConfigurationDefinition) v);
case "RouteConfigurationsDefinition" -> doWriteRouteConfigurationsDefinition("routeConfigurations", (RouteConfigurationsDefinition) v);
case "RouteDefinition" -> doWriteRouteDefinition("route", (RouteDefinition) v);
case "RouteTemplateDefinition" -> doWriteRouteTemplateDefinition("routeTemplate", (RouteTemplateDefinition) v);
case "RouteTemplatesDefinition" -> doWriteRouteTemplatesDefinition("routeTemplates", (RouteTemplatesDefinition) v);
case "RoutesDefinition" -> doWriteRoutesDefinition("routes", (RoutesDefinition) v);
case "RoutingSlipDefinition" -> doWriteRoutingSlipDefinition("routingSlip", (RoutingSlipDefinition) v);
case "SagaDefinition" -> doWriteSagaDefinition("saga", (SagaDefinition) v);
case "SamplingDefinition" -> doWriteSamplingDefinition("sample", (SamplingDefinition) v);
case "ScriptDefinition" -> doWriteScriptDefinition("script", (ScriptDefinition) v);
case "SetBodyDefinition" -> doWriteSetBodyDefinition("setBody", (SetBodyDefinition) v);
case "SetExchangePatternDefinition" -> doWriteSetExchangePatternDefinition("setExchangePattern", (SetExchangePatternDefinition) v);
case "SetHeaderDefinition" -> doWriteSetHeaderDefinition("setHeader", (SetHeaderDefinition) v);
case "SetHeadersDefinition" -> doWriteSetHeadersDefinition("setHeaders", (SetHeadersDefinition) v);
case "SetPropertyDefinition" -> doWriteSetPropertyDefinition("setProperty", (SetPropertyDefinition) v);
case "SetVariableDefinition" -> doWriteSetVariableDefinition("setVariable", (SetVariableDefinition) v);
case "SortDefinition" -> doWriteSortDefinition("sort", (SortDefinition) v);
case "SplitDefinition" -> doWriteSplitDefinition("split", (SplitDefinition) v);
case "StepDefinition" -> doWriteStepDefinition("step", (StepDefinition) v);
case "StopDefinition" -> doWriteStopDefinition("stop", (StopDefinition) v);
case "TemplatedRoutesDefinition" -> doWriteTemplatedRoutesDefinition("templatedRoutes", (TemplatedRoutesDefinition) v);
case "ThreadPoolProfileDefinition" -> doWriteThreadPoolProfileDefinition("threadPoolProfile", (ThreadPoolProfileDefinition) v);
case "ThreadsDefinition" -> doWriteThreadsDefinition("threads", (ThreadsDefinition) v);
case "ThrottleDefinition" -> doWriteThrottleDefinition("throttle", (ThrottleDefinition) v);
case "ThrowExceptionDefinition" -> doWriteThrowExceptionDefinition("throwException", (ThrowExceptionDefinition) v);
case "ToDefinition" -> doWriteToDefinition("to", (ToDefinition) v);
case "ToDynamicDefinition" -> doWriteToDynamicDefinition("toD", (ToDynamicDefinition) v);
case "TransactedDefinition" -> doWriteTransactedDefinition("transacted", (TransactedDefinition) v);
case "TransformDefinition" -> doWriteTransformDefinition("transform", (TransformDefinition) v);
case "TryDefinition" -> doWriteTryDefinition("doTry", (TryDefinition) v);
case "UnmarshalDefinition" -> doWriteUnmarshalDefinition("unmarshal", (UnmarshalDefinition) v);
case "ValidateDefinition" -> doWriteValidateDefinition("validate", (ValidateDefinition) v);
case "WhenDefinition" -> doWriteWhenDefinition("when", (WhenDefinition) v);
case "WireTapDefinition" -> doWriteWireTapDefinition("wireTap", (WireTapDefinition) v);
case "ServiceCallDefinition" -> doWriteServiceCallDefinition("serviceCall", (ServiceCallDefinition) v);
case "DeleteDefinition" -> doWriteDeleteDefinition("delete", (DeleteDefinition) v);
case "GetDefinition" -> doWriteGetDefinition("get", (GetDefinition) v);
case "HeadDefinition" -> doWriteHeadDefinition("head", (HeadDefinition) v);
case "PatchDefinition" -> doWritePatchDefinition("patch", (PatchDefinition) v);
case "PostDefinition" -> doWritePostDefinition("post", (PostDefinition) v);
case "PutDefinition" -> doWritePutDefinition("put", (PutDefinition) v);
case "RestBindingDefinition" -> doWriteRestBindingDefinition("restBinding", (RestBindingDefinition) v);
case "RestDefinition" -> doWriteRestDefinition("rest", (RestDefinition) v);
case "RestsDefinition" -> doWriteRestsDefinition("rests", (RestsDefinition) v);
}
}
}
protected void doWriteOutputTypeDefinitionRef(
String n,
OutputTypeDefinition v)
throws IOException {
if (v != null) {
switch (v.getClass().getSimpleName()) {
case "OutputTypeDefinition" -> doWriteOutputTypeDefinition("outputType", (OutputTypeDefinition) v);
}
}
}
protected void doWriteProcessorDefinitionRef(
String n,
ProcessorDefinition> v)
throws IOException {
if (v != null) {
switch (v.getClass().getSimpleName()) {
case "AggregateDefinition" -> doWriteAggregateDefinition("aggregate", (AggregateDefinition) v);
case "BeanDefinition" -> doWriteBeanDefinition("bean", (BeanDefinition) v);
case "CatchDefinition" -> doWriteCatchDefinition("doCatch", (CatchDefinition) v);
case "ChoiceDefinition" -> doWriteChoiceDefinition("choice", (ChoiceDefinition) v);
case "CircuitBreakerDefinition" -> doWriteCircuitBreakerDefinition("circuitBreaker", (CircuitBreakerDefinition) v);
case "ClaimCheckDefinition" -> doWriteClaimCheckDefinition("claimCheck", (ClaimCheckDefinition) v);
case "ConvertBodyDefinition" -> doWriteConvertBodyDefinition("convertBodyTo", (ConvertBodyDefinition) v);
case "ConvertHeaderDefinition" -> doWriteConvertHeaderDefinition("convertHeaderTo", (ConvertHeaderDefinition) v);
case "ConvertVariableDefinition" -> doWriteConvertVariableDefinition("convertVariableTo", (ConvertVariableDefinition) v);
case "DelayDefinition" -> doWriteDelayDefinition("delay", (DelayDefinition) v);
case "DynamicRouterDefinition" -> doWriteDynamicRouterDefinition("dynamicRouter", (DynamicRouterDefinition) v);
case "EnrichDefinition" -> doWriteEnrichDefinition("enrich", (EnrichDefinition) v);
case "FilterDefinition" -> doWriteFilterDefinition("filter", (FilterDefinition) v);
case "FinallyDefinition" -> doWriteFinallyDefinition("doFinally", (FinallyDefinition) v);
case "IdempotentConsumerDefinition" -> doWriteIdempotentConsumerDefinition("idempotentConsumer", (IdempotentConsumerDefinition) v);
case "InterceptDefinition" -> doWriteInterceptDefinition("intercept", (InterceptDefinition) v);
case "InterceptFromDefinition" -> doWriteInterceptFromDefinition("interceptFrom", (InterceptFromDefinition) v);
case "InterceptSendToEndpointDefinition" -> doWriteInterceptSendToEndpointDefinition("interceptSendToEndpoint", (InterceptSendToEndpointDefinition) v);
case "KameletDefinition" -> doWriteKameletDefinition("kamelet", (KameletDefinition) v);
case "LoadBalanceDefinition" -> doWriteLoadBalanceDefinition("loadBalance", (LoadBalanceDefinition) v);
case "LogDefinition" -> doWriteLogDefinition("log", (LogDefinition) v);
case "LoopDefinition" -> doWriteLoopDefinition("loop", (LoopDefinition) v);
case "MarshalDefinition" -> doWriteMarshalDefinition("marshal", (MarshalDefinition) v);
case "MulticastDefinition" -> doWriteMulticastDefinition("multicast", (MulticastDefinition) v);
case "OnCompletionDefinition" -> doWriteOnCompletionDefinition("onCompletion", (OnCompletionDefinition) v);
case "OnExceptionDefinition" -> doWriteOnExceptionDefinition("onException", (OnExceptionDefinition) v);
case "OnFallbackDefinition" -> doWriteOnFallbackDefinition("onFallback", (OnFallbackDefinition) v);
case "OtherwiseDefinition" -> doWriteOtherwiseDefinition("otherwise", (OtherwiseDefinition) v);
case "PausableDefinition" -> doWritePausableDefinition("pausable", (PausableDefinition) v);
case "PipelineDefinition" -> doWritePipelineDefinition("pipeline", (PipelineDefinition) v);
case "PolicyDefinition" -> doWritePolicyDefinition("policy", (PolicyDefinition) v);
case "PollEnrichDefinition" -> doWritePollEnrichDefinition("pollEnrich", (PollEnrichDefinition) v);
case "ProcessDefinition" -> doWriteProcessDefinition("process", (ProcessDefinition) v);
case "RecipientListDefinition" -> doWriteRecipientListDefinition("recipientList", (RecipientListDefinition) v);
case "RemoveHeaderDefinition" -> doWriteRemoveHeaderDefinition("removeHeader", (RemoveHeaderDefinition) v);
case "RemoveHeadersDefinition" -> doWriteRemoveHeadersDefinition("removeHeaders", (RemoveHeadersDefinition) v);
case "RemovePropertiesDefinition" -> doWriteRemovePropertiesDefinition("removeProperties", (RemovePropertiesDefinition) v);
case "RemovePropertyDefinition" -> doWriteRemovePropertyDefinition("removeProperty", (RemovePropertyDefinition) v);
case "RemoveVariableDefinition" -> doWriteRemoveVariableDefinition("removeVariable", (RemoveVariableDefinition) v);
case "ResequenceDefinition" -> doWriteResequenceDefinition("resequence", (ResequenceDefinition) v);
case "ResumableDefinition" -> doWriteResumableDefinition("resumable", (ResumableDefinition) v);
case "RollbackDefinition" -> doWriteRollbackDefinition("rollback", (RollbackDefinition) v);
case "RouteDefinition" -> doWriteRouteDefinition("route", (RouteDefinition) v);
case "RoutingSlipDefinition" -> doWriteRoutingSlipDefinition("routingSlip", (RoutingSlipDefinition) v);
case "SagaDefinition" -> doWriteSagaDefinition("saga", (SagaDefinition) v);
case "SamplingDefinition" -> doWriteSamplingDefinition("sample", (SamplingDefinition) v);
case "ScriptDefinition" -> doWriteScriptDefinition("script", (ScriptDefinition) v);
case "SetBodyDefinition" -> doWriteSetBodyDefinition("setBody", (SetBodyDefinition) v);
case "SetExchangePatternDefinition" -> doWriteSetExchangePatternDefinition("setExchangePattern", (SetExchangePatternDefinition) v);
case "SetHeaderDefinition" -> doWriteSetHeaderDefinition("setHeader", (SetHeaderDefinition) v);
case "SetHeadersDefinition" -> doWriteSetHeadersDefinition("setHeaders", (SetHeadersDefinition) v);
case "SetPropertyDefinition" -> doWriteSetPropertyDefinition("setProperty", (SetPropertyDefinition) v);
case "SetVariableDefinition" -> doWriteSetVariableDefinition("setVariable", (SetVariableDefinition) v);
case "SortDefinition" -> doWriteSortDefinition("sort", (SortDefinition) v);
case "SplitDefinition" -> doWriteSplitDefinition("split", (SplitDefinition) v);
case "StepDefinition" -> doWriteStepDefinition("step", (StepDefinition) v);
case "StopDefinition" -> doWriteStopDefinition("stop", (StopDefinition) v);
case "ThreadsDefinition" -> doWriteThreadsDefinition("threads", (ThreadsDefinition) v);
case "ThrottleDefinition" -> doWriteThrottleDefinition("throttle", (ThrottleDefinition) v);
case "ThrowExceptionDefinition" -> doWriteThrowExceptionDefinition("throwException", (ThrowExceptionDefinition) v);
case "ToDefinition" -> doWriteToDefinition("to", (ToDefinition) v);
case "ToDynamicDefinition" -> doWriteToDynamicDefinition("toD", (ToDynamicDefinition) v);
case "TransactedDefinition" -> doWriteTransactedDefinition("transacted", (TransactedDefinition) v);
case "TransformDefinition" -> doWriteTransformDefinition("transform", (TransformDefinition) v);
case "TryDefinition" -> doWriteTryDefinition("doTry", (TryDefinition) v);
case "UnmarshalDefinition" -> doWriteUnmarshalDefinition("unmarshal", (UnmarshalDefinition) v);
case "ValidateDefinition" -> doWriteValidateDefinition("validate", (ValidateDefinition) v);
case "WhenDefinition" -> doWriteWhenDefinition("when", (WhenDefinition) v);
case "WireTapDefinition" -> doWriteWireTapDefinition("wireTap", (WireTapDefinition) v);
case "ServiceCallDefinition" -> doWriteServiceCallDefinition("serviceCall", (ServiceCallDefinition) v);
}
}
}
protected void doWriteRouteConfigurationDefinitionRef(
String n,
RouteConfigurationDefinition v)
throws IOException {
if (v != null) {
switch (v.getClass().getSimpleName()) {
case "RouteConfigurationDefinition" -> doWriteRouteConfigurationDefinition("routeConfiguration", (RouteConfigurationDefinition) v);
}
}
}
protected void doWriteRouteDefinitionRef(
String n,
RouteDefinition v)
throws IOException {
if (v != null) {
switch (v.getClass().getSimpleName()) {
case "RouteDefinition" -> doWriteRouteDefinition("route", (RouteDefinition) v);
}
}
}
protected void doWriteRouteTemplateDefinitionRef(
String n,
RouteTemplateDefinition v)
throws IOException {
if (v != null) {
switch (v.getClass().getSimpleName()) {
case "RouteTemplateDefinition" -> doWriteRouteTemplateDefinition("routeTemplate", (RouteTemplateDefinition) v);
}
}
}
protected void doWriteSetHeaderDefinitionRef(
String n,
SetHeaderDefinition v)
throws IOException {
if (v != null) {
switch (v.getClass().getSimpleName()) {
case "SetHeaderDefinition" -> doWriteSetHeaderDefinition("setHeader", (SetHeaderDefinition) v);
}
}
}
protected void doWriteTemplatedRouteDefinitionRef(
String n,
TemplatedRouteDefinition v)
throws IOException {
if (v != null) {
switch (v.getClass().getSimpleName()) {
case "TemplatedRouteDefinition" -> doWriteTemplatedRouteDefinition("templatedRoute", (TemplatedRouteDefinition) v);
}
}
}
protected void doWriteWhenDefinitionRef(
String n,
WhenDefinition v)
throws IOException {
if (v != null) {
switch (v.getClass().getSimpleName()) {
case "WhenDefinition" -> doWriteWhenDefinition("when", (WhenDefinition) v);
}
}
}
protected void doWriteUniVocityHeaderRef(
String n,
UniVocityHeader v)
throws IOException {
if (v != null) {
switch (v.getClass().getSimpleName()) {
case "UniVocityHeader" -> doWriteUniVocityHeader("univocityHeader", (UniVocityHeader) v);
}
}
}
protected void doWriteExpressionDefinitionRef(
String n,
ExpressionDefinition v)
throws IOException {
if (v != null) {
switch (v.getClass().getSimpleName()) {
case "CSimpleExpression" -> doWriteCSimpleExpression("csimple", (CSimpleExpression) v);
case "ConstantExpression" -> doWriteConstantExpression("constant", (ConstantExpression) v);
case "DatasonnetExpression" -> doWriteDatasonnetExpression("datasonnet", (DatasonnetExpression) v);
case "ExchangePropertyExpression" -> doWriteExchangePropertyExpression("exchangeProperty", (ExchangePropertyExpression) v);
case "ExpressionDefinition" -> doWriteExpressionDefinition("expressionDefinition", (ExpressionDefinition) v);
case "GroovyExpression" -> doWriteGroovyExpression("groovy", (GroovyExpression) v);
case "HeaderExpression" -> doWriteHeaderExpression("header", (HeaderExpression) v);
case "Hl7TerserExpression" -> doWriteHl7TerserExpression("hl7terser", (Hl7TerserExpression) v);
case "JavaExpression" -> doWriteJavaExpression("java", (JavaExpression) v);
case "JavaScriptExpression" -> doWriteJavaScriptExpression("js", (JavaScriptExpression) v);
case "JoorExpression" -> doWriteJoorExpression("joor", (JoorExpression) v);
case "JqExpression" -> doWriteJqExpression("jq", (JqExpression) v);
case "JsonPathExpression" -> doWriteJsonPathExpression("jsonpath", (JsonPathExpression) v);
case "LanguageExpression" -> doWriteLanguageExpression("language", (LanguageExpression) v);
case "MethodCallExpression" -> doWriteMethodCallExpression("method", (MethodCallExpression) v);
case "MvelExpression" -> doWriteMvelExpression("mvel", (MvelExpression) v);
case "OgnlExpression" -> doWriteOgnlExpression("ognl", (OgnlExpression) v);
case "PythonExpression" -> doWritePythonExpression("python", (PythonExpression) v);
case "RefExpression" -> doWriteRefExpression("ref", (RefExpression) v);
case "SimpleExpression" -> doWriteSimpleExpression("simple", (SimpleExpression) v);
case "SpELExpression" -> doWriteSpELExpression("spel", (SpELExpression) v);
case "TokenizerExpression" -> doWriteTokenizerExpression("tokenize", (TokenizerExpression) v);
case "VariableExpression" -> doWriteVariableExpression("variable", (VariableExpression) v);
case "WasmExpression" -> doWriteWasmExpression("wasm", (WasmExpression) v);
case "XMLTokenizerExpression" -> doWriteXMLTokenizerExpression("xtokenize", (XMLTokenizerExpression) v);
case "XPathExpression" -> doWriteXPathExpression("xpath", (XPathExpression) v);
case "XQueryExpression" -> doWriteXQueryExpression("xquery", (XQueryExpression) v);
}
}
}
protected void doWriteParamDefinitionRef(
String n,
ParamDefinition v)
throws IOException {
if (v != null) {
switch (v.getClass().getSimpleName()) {
case "ParamDefinition" -> doWriteParamDefinition("param", (ParamDefinition) v);
}
}
}
protected void doWriteResponseMessageDefinitionRef(
String n,
ResponseMessageDefinition v)
throws IOException {
if (v != null) {
switch (v.getClass().getSimpleName()) {
case "ResponseMessageDefinition" -> doWriteResponseMessageDefinition("responseMessage", (ResponseMessageDefinition) v);
}
}
}
protected void doWriteRestDefinitionRef(
String n,
RestDefinition v)
throws IOException {
if (v != null) {
switch (v.getClass().getSimpleName()) {
case "RestDefinition" -> doWriteRestDefinition("rest", (RestDefinition) v);
}
}
}
protected void doWriteSecurityDefinitionRef(
String n,
SecurityDefinition v)
throws IOException {
if (v != null) {
switch (v.getClass().getSimpleName()) {
case "SecurityDefinition" -> doWriteSecurityDefinition("security", (SecurityDefinition) v);
}
}
}
protected void doWriteVerbDefinitionRef(
String n,
VerbDefinition v)
throws IOException {
if (v != null) {
switch (v.getClass().getSimpleName()) {
case "DeleteDefinition" -> doWriteDeleteDefinition("delete", (DeleteDefinition) v);
case "GetDefinition" -> doWriteGetDefinition("get", (GetDefinition) v);
case "HeadDefinition" -> doWriteHeadDefinition("head", (HeadDefinition) v);
case "PatchDefinition" -> doWritePatchDefinition("patch", (PatchDefinition) v);
case "PostDefinition" -> doWritePostDefinition("post", (PostDefinition) v);
case "PutDefinition" -> doWritePutDefinition("put", (PutDefinition) v);
}
}
}
protected void doWriteAttribute(
String attribute,
Object value)
throws IOException {
if (value != null) {
attribute(attribute, value);
}
}
protected void doWriteValue(String value) throws IOException {
if (value != null) {
value(value);
}
}
protected void doWriteList(String wrapperName, String name, List list, ElementSerializer elementSerializer) throws IOException {
if (list != null) {
if (wrapperName != null) {
startElement(wrapperName);
}
for (T v : list) {
elementSerializer.doWriteElement(name, v);
}
if (wrapperName != null) {
endElement(wrapperName);
}
}
}
protected void doWriteElement(String name, T v, ElementSerializer elementSerializer) throws IOException {
if (v != null) {
elementSerializer.doWriteElement(name, v);
}
}
protected String toString(Boolean b) {
return b != null ? b.toString() : null;
}
protected String toString(Enum> e) {
return e != null ? e.name() : null;
}
protected String toString(Number n) {
return n != null ? n.toString() : null;
}
protected String toString(byte[] b) {
return b != null ? Base64.getEncoder().encodeToString(b) : null;
}
protected void doWriteString(String name, String value) throws IOException {
if (value != null) {
startElement(name);
text(name, value);
endElement(name);
}
}
protected void doWriteNamespaces(
NamespaceAwareExpression def)
throws IOException {
if (def.getNamespaceAsMap() != null) {
for (var e : def.getNamespaceAsMap().entrySet()) {
doWriteAttribute("xmlns:" + e.getKey(), e.getValue());
}
}
}
public interface ElementSerializer {
void doWriteElement(String name, T value) throws IOException;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy