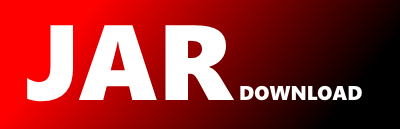
org.apache.cassandra.config.CassandraRelevantProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cassandra-all Show documentation
Show all versions of cassandra-all Show documentation
The Apache Cassandra Project develops a highly scalable second-generation distributed database, bringing together Dynamo's fully distributed design and Bigtable's ColumnFamily-based data model.
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.cassandra.config;
import java.util.concurrent.TimeUnit;
import org.apache.cassandra.exceptions.ConfigurationException;
/** A class that extracts system properties for the cassandra node it runs within. */
public enum CassandraRelevantProperties
{
//base JVM properties
JAVA_HOME("java.home"),
CASSANDRA_PID_FILE ("cassandra-pidfile"),
/**
* Indicates the temporary directory used by the Java Virtual Machine (JVM)
* to create and store temporary files.
*/
JAVA_IO_TMPDIR ("java.io.tmpdir"),
/**
* Path from which to load native libraries.
* Default is absolute path to lib directory.
*/
JAVA_LIBRARY_PATH ("java.library.path"),
JAVA_SECURITY_EGD ("java.security.egd"),
/** Java Runtime Environment version */
JAVA_VERSION ("java.version"),
/** Java Virtual Machine implementation name */
JAVA_VM_NAME ("java.vm.name"),
/** Line separator ("\n" on UNIX). */
LINE_SEPARATOR ("line.separator"),
/** Java class path. */
JAVA_CLASS_PATH ("java.class.path"),
/** Operating system architecture. */
OS_ARCH ("os.arch"),
/** Operating system name. */
OS_NAME ("os.name"),
/** User's home directory. */
USER_HOME ("user.home"),
/** Platform word size sun.arch.data.model. Examples: "32", "64", "unknown"*/
SUN_ARCH_DATA_MODEL ("sun.arch.data.model"),
//JMX properties
/**
* The value of this property represents the host name string
* that should be associated with remote stubs for locally created remote objects,
* in order to allow clients to invoke methods on the remote object.
*/
JAVA_RMI_SERVER_HOSTNAME ("java.rmi.server.hostname"),
/**
* If this value is true, object identifiers for remote objects exported by this VM will be generated by using
* a cryptographically secure random number generator. The default value is false.
*/
JAVA_RMI_SERVER_RANDOM_ID ("java.rmi.server.randomIDs"),
/**
* This property indicates whether password authentication for remote monitoring is
* enabled. By default it is disabled - com.sun.management.jmxremote.authenticate
*/
COM_SUN_MANAGEMENT_JMXREMOTE_AUTHENTICATE ("com.sun.management.jmxremote.authenticate"),
/**
* Controls the JMX server threadpool keap-alive time.
* Should only be set by in-jvm dtests.
*/
SUN_RMI_TRANSPORT_TCP_THREADKEEPALIVETIME("sun.rmi.transport.tcp.threadKeepAliveTime"),
/**
* Controls the distributed garbage collector lease time for JMX objects.
* Should only be set by in-jvm dtests.
*/
JAVA_RMI_DGC_LEASE_VALUE_IN_JVM_DTEST("java.rmi.dgc.leaseValue"),
/**
* The port number to which the RMI connector will be bound - com.sun.management.jmxremote.rmi.port.
* An Integer object that represents the value of the second argument is returned
* if there is no port specified, if the port does not have the correct numeric format,
* or if the specified name is empty or null.
*/
COM_SUN_MANAGEMENT_JMXREMOTE_RMI_PORT ("com.sun.management.jmxremote.rmi.port", "0"),
/** Cassandra jmx remote port */
CASSANDRA_JMX_REMOTE_PORT("cassandra.jmx.remote.port"),
/** This property indicates whether SSL is enabled for monitoring remotely. Default is set to false. */
COM_SUN_MANAGEMENT_JMXREMOTE_SSL ("com.sun.management.jmxremote.ssl"),
/**
* This property indicates whether SSL client authentication is enabled - com.sun.management.jmxremote.ssl.need.client.auth.
* Default is set to false.
*/
COM_SUN_MANAGEMENT_JMXREMOTE_SSL_NEED_CLIENT_AUTH ("com.sun.management.jmxremote.ssl.need.client.auth"),
/**
* This property indicates the location for the access file. If com.sun.management.jmxremote.authenticate is false,
* then this property and the password and access files, are ignored. Otherwise, the access file must exist and
* be in the valid format. If the access file is empty or nonexistent, then no access is allowed.
*/
COM_SUN_MANAGEMENT_JMXREMOTE_ACCESS_FILE ("com.sun.management.jmxremote.access.file"),
/** This property indicates the path to the password file - com.sun.management.jmxremote.password.file */
COM_SUN_MANAGEMENT_JMXREMOTE_PASSWORD_FILE ("com.sun.management.jmxremote.password.file"),
/** Port number to enable JMX RMI connections - com.sun.management.jmxremote.port */
COM_SUN_MANAGEMENT_JMXREMOTE_PORT ("com.sun.management.jmxremote.port"),
/**
* A comma-delimited list of SSL/TLS protocol versions to enable.
* Used in conjunction with com.sun.management.jmxremote.ssl - com.sun.management.jmxremote.ssl.enabled.protocols
*/
COM_SUN_MANAGEMENT_JMXREMOTE_SSL_ENABLED_PROTOCOLS ("com.sun.management.jmxremote.ssl.enabled.protocols"),
/**
* A comma-delimited list of SSL/TLS cipher suites to enable.
* Used in conjunction with com.sun.management.jmxremote.ssl - com.sun.management.jmxremote.ssl.enabled.cipher.suites
*/
COM_SUN_MANAGEMENT_JMXREMOTE_SSL_ENABLED_CIPHER_SUITES ("com.sun.management.jmxremote.ssl.enabled.cipher.suites"),
/** mx4jaddress */
MX4JADDRESS ("mx4jaddress"),
/** mx4jport */
MX4JPORT ("mx4jport"),
RING_DELAY("cassandra.ring_delay_ms"),
FAILED_BOOTSTRAP_TIMEOUT("cassandra.failed_bootstrap_timeout_ms"),
/**
* When bootstraping we wait for all schema versions found in gossip to be seen, and if not seen in time we fail
* the bootstrap; this property will avoid failing and allow bootstrap to continue if set to true.
*/
BOOTSTRAP_SKIP_SCHEMA_CHECK("cassandra.skip_schema_check"),
/**
* When bootstraping how long to wait for schema versions to be seen.
*/
BOOTSTRAP_SCHEMA_DELAY_MS("cassandra.schema_delay_ms"),
/**
* When draining, how long to wait for mutating executors to shutdown.
*/
DRAIN_EXECUTOR_TIMEOUT_MS("cassandra.drain_executor_timeout_ms", String.valueOf(TimeUnit.MINUTES.toMillis(5))),
/**
* Gossip quarantine delay is used while evaluating membership changes and should only be changed with extreme care.
*/
GOSSIPER_QUARANTINE_DELAY("cassandra.gossip_quarantine_delay_ms"),
IGNORED_SCHEMA_CHECK_VERSIONS("cassandra.skip_schema_check_for_versions"),
IGNORED_SCHEMA_CHECK_ENDPOINTS("cassandra.skip_schema_check_for_endpoints"),
/**
* When doing a host replacement its possible that the gossip state is "empty" meaning that the endpoint is known
* but the current state isn't known. If the host replacement is needed to repair this state, this property must
* be true.
*/
REPLACEMENT_ALLOW_EMPTY("cassandra.allow_empty_replace_address", "true"),
/**
* Whether {@link org.apache.cassandra.db.ConsistencyLevel#NODE_LOCAL} should be allowed.
*/
ENABLE_NODELOCAL_QUERIES("cassandra.enable_nodelocal_queries", "false"),
//cassandra properties (without the "cassandra." prefix)
/**
* The cassandra-foreground option will tell CassandraDaemon whether
* to close stdout/stderr, but it's up to us not to background.
* yes/null
*/
CASSANDRA_FOREGROUND ("cassandra-foreground"),
DEFAULT_PROVIDE_OVERLAPPING_TOMBSTONES ("default.provide.overlapping.tombstones"),
ORG_APACHE_CASSANDRA_DISABLE_MBEAN_REGISTRATION ("org.apache.cassandra.disable_mbean_registration"),
//only for testing
ORG_APACHE_CASSANDRA_CONF_CASSANDRA_RELEVANT_PROPERTIES_TEST("org.apache.cassandra.conf.CassandraRelevantPropertiesTest"),
ORG_APACHE_CASSANDRA_DB_VIRTUAL_SYSTEM_PROPERTIES_TABLE_TEST("org.apache.cassandra.db.virtual.SystemPropertiesTableTest"),
/** This property indicates whether disable_mbean_registration is true */
IS_DISABLED_MBEAN_REGISTRATION("org.apache.cassandra.disable_mbean_registration"),
/** what class to use for mbean registeration */
MBEAN_REGISTRATION_CLASS("org.apache.cassandra.mbean_registration_class"),
/** This property indicates if the code is running under the in-jvm dtest framework */
DTEST_IS_IN_JVM_DTEST("org.apache.cassandra.dtest.is_in_jvm_dtest"),
MIGRATION_DELAY("cassandra.migration_delay_ms", "60000"),
/** Defines how often schema definitions are pulled from the other nodes */
SCHEMA_PULL_INTERVAL_MS("cassandra.schema_pull_interval_ms", "60000"),
/**
* Minimum delay after a failed pull request before it is reattempted. It prevents reattempting failed requests
* immediately as it is high chance they will fail anyway. It is better to wait a bit instead of flooding logs
* and wasting resources.
*/
SCHEMA_PULL_BACKOFF_DELAY_MS("cassandra.schema_pull_backoff_delay_ms", "3000"),
/** When enabled, recursive directory deletion will be executed using a unix command `rm -rf` instead of traversing
* and removing individual files. This is now used only tests, but eventually we will make it true by default.*/
USE_NIX_RECURSIVE_DELETE("cassandra.use_nix_recursive_delete"),
/** If set, {@link org.apache.cassandra.net.MessagingService} is shutdown abrtuptly without waiting for anything.
* This is an optimization used in unit tests becuase we never restart a node there. The only node is stopoped
* when the JVM terminates. Therefore, we can use such optimization and not wait unnecessarily. */
NON_GRACEFUL_SHUTDOWN("cassandra.test.messagingService.nonGracefulShutdown"),
/** Flush changes of {@link org.apache.cassandra.schema.SchemaKeyspace} after each schema modification. In production,
* we always do that. However, tests which do not restart nodes may disable this functionality in order to run
* faster. Note that this is disabled for unit tests but if an individual test requires schema to be flushed, it
* can be also done manually for that particular case: {@code flush(SchemaConstants.SCHEMA_KEYSPACE_NAME);}. */
FLUSH_LOCAL_SCHEMA_CHANGES("cassandra.test.flush_local_schema_changes", "true"),
/**
* Delay before checking if gossip is settled.
*/
GOSSIP_SETTLE_MIN_WAIT_MS("cassandra.gossip_settle_min_wait_ms", "5000"),
/**
* Interval delay between checking gossip is settled.
*/
GOSSIP_SETTLE_POLL_INTERVAL_MS("cassandra.gossip_settle_interval_ms", "1000"),
/**
* Number of polls without gossip state change to consider gossip as settled.
*/
GOSSIP_SETTLE_POLL_SUCCESSES_REQUIRED("cassandra.gossip_settle_poll_success_required", "3"),
;
CassandraRelevantProperties(String key, String defaultVal)
{
this.key = key;
this.defaultVal = defaultVal;
}
CassandraRelevantProperties(String key)
{
this.key = key;
this.defaultVal = null;
}
private final String key;
private final String defaultVal;
public String getKey()
{
return key;
}
/**
* Gets the value of the indicated system property.
* @return system property value if it exists, defaultValue otherwise.
*/
public String getString()
{
String value = System.getProperty(key);
return value == null ? defaultVal : STRING_CONVERTER.convert(value);
}
/**
* Sets the property to its default value if a default value was specified. Remove the property otherwise.
*/
public void reset()
{
if (defaultVal != null)
System.setProperty(key, defaultVal);
else
System.getProperties().remove(key);
}
/**
* Gets the value of a system property as a String.
* @return system property String value if it exists, overrideDefaultValue otherwise.
*/
public String getString(String overrideDefaultValue)
{
String value = System.getProperty(key);
if (value == null)
return overrideDefaultValue;
return STRING_CONVERTER.convert(value);
}
/**
* Sets the value into system properties.
* @param value to set
*/
public void setString(String value)
{
System.setProperty(key, value);
}
/**
* Gets the value of a system property as a boolean.
* @return system property boolean value if it exists, false otherwise().
*/
public boolean getBoolean()
{
String value = System.getProperty(key);
return BOOLEAN_CONVERTER.convert(value == null ? defaultVal : value);
}
/**
* Gets the value of a system property as a boolean.
* @return system property boolean value if it exists, overrideDefaultValue otherwise.
*/
public boolean getBoolean(boolean overrideDefaultValue)
{
String value = System.getProperty(key);
if (value == null)
return overrideDefaultValue;
return BOOLEAN_CONVERTER.convert(value);
}
/**
* Sets the value into system properties.
* @param value to set
*/
public void setBoolean(boolean value)
{
System.setProperty(key, Boolean.toString(value));
}
/**
* Gets the value of a system property as a int.
* @return system property int value if it exists, defaultValue otherwise.
*/
public int getInt()
{
String value = System.getProperty(key);
return INTEGER_CONVERTER.convert(value == null ? defaultVal : value);
}
/**
* Gets the value of a system property as a int.
* @return system property int value if it exists, overrideDefaultValue otherwise.
*/
public int getInt(int overrideDefaultValue)
{
String value = System.getProperty(key);
if (value == null)
return overrideDefaultValue;
return INTEGER_CONVERTER.convert(value);
}
/**
* Sets the value into system properties.
* @param value to set
*/
public void setInt(int value)
{
System.setProperty(key, Integer.toString(value));
}
private interface PropertyConverter
{
T convert(String value);
}
private static final PropertyConverter STRING_CONVERTER = value -> value;
private static final PropertyConverter BOOLEAN_CONVERTER = Boolean::parseBoolean;
private static final PropertyConverter INTEGER_CONVERTER = value ->
{
try
{
return Integer.decode(value);
}
catch (NumberFormatException e)
{
throw new ConfigurationException(String.format("Invalid value for system property: " +
"expected integer value but got '%s'", value));
}
};
/**
* @return whether a system property is present or not.
*/
public boolean isPresent()
{
return System.getProperties().containsKey(key);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy