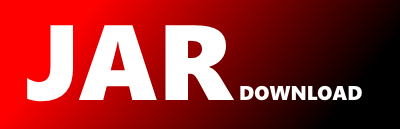
org.apache.cassandra.distributed.api.IIsolatedExecutor Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.cassandra.distributed.api;
import java.io.Serializable;
import java.util.concurrent.Callable;
import java.util.concurrent.Executor;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Future;
import java.util.function.BiConsumer;
import java.util.function.BiFunction;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Supplier;
/**
* Represents a clean way to handoff evaluation of some work to an executor associated
* with a node's lifetime.
*
* There is no transfer of execution to the parallel class hierarchy.
*
* Classes, such as Instance, that are themselves instantiated on the correct ClassLoader, utilise this class
* to ensure the lifetime of any thread evaluating one of its method invocations matches the lifetime of the class itself.
* Since they are instantiated on the correct ClassLoader, sharing only the interface, there is no serialization necessary.
*/
public interface IIsolatedExecutor
{
interface CallableNoExcept extends Callable { O call(); }
interface SerializableRunnable extends Runnable, Serializable {}
interface SerializableSupplier extends Supplier, Serializable {}
interface SerializableCallable extends CallableNoExcept, Serializable {}
interface SerializableConsumer extends Consumer, Serializable {}
interface SerializableBiConsumer extends BiConsumer, Serializable {}
interface TriConsumer { void accept(I1 i1, I2 i2, I3 i3); }
interface SerializableTriConsumer extends TriConsumer, Serializable { }
interface DynamicFunction
{
IO2 apply(IO2 i);
}
interface SerializableDynamicFunction extends DynamicFunction, Serializable {}
interface SerializableFunction extends Function, Serializable {}
interface SerializableBiFunction extends BiFunction, Serializable {}
interface TriFunction { O apply(I1 i1, I2 i2, I3 i3); }
interface SerializableTriFunction extends Serializable, TriFunction {}
interface QuadFunction { O apply(I1 i1, I2 i2, I3 i3, I4 i4); }
interface SerializableQuadFunction extends Serializable, QuadFunction {}
interface QuintFunction { O apply(I1 i1, I2 i2, I3 i3, I4 i4, I5 i5); }
interface SerializableQuintFunction extends Serializable, QuintFunction {}
default IIsolatedExecutor with(ExecutorService executor) { throw new UnsupportedOperationException(); }
default Executor executor() { throw new UnsupportedOperationException(); }
Future shutdown();
/**
* Convert the execution to one performed asynchronously on the IsolatedExecutor, returning a Future of the execution result
*/
CallableNoExcept> async(CallableNoExcept call);
/**
* Convert the execution to one performed synchronously on the IsolatedExecutor
*/
CallableNoExcept sync(CallableNoExcept call);
/**
* Convert the execution to one performed asynchronously on the IsolatedExecutor, returning a Future of the execution result
*/
CallableNoExcept> async(Runnable run);
/**
* Convert the execution to one performed synchronously on the IsolatedExecutor
*/
Runnable sync(Runnable run);
/**
* Convert the execution to one performed asynchronously on the IsolatedExecutor, returning a Future of the execution result
*/
Function> async(Consumer consumer);
/**
* Convert the execution to one performed synchronously on the IsolatedExecutor
*/
Consumer sync(Consumer consumer);
/**
* Convert the execution to one performed asynchronously on the IsolatedExecutor, returning a Future of the execution result
*/
BiFunction> async(BiConsumer consumer);
/**
* Convert the execution to one performed synchronously on the IsolatedExecutor
*/
BiConsumer sync(BiConsumer consumer);
/**
* Convert the execution to one performed synchronously on the IsolatedExecutor
*/
TriFunction> async(TriConsumer consumer);
/**
* Convert the execution to one performed synchronously on the IsolatedExecutor
*/
TriConsumer sync(TriConsumer consumer);
/**
* Convert the execution to one performed asynchronously on the IsolatedExecutor, returning a Future of the execution result
*/
Function> async(Function f);
/**
* Convert the execution to one performed synchronously on the IsolatedExecutor
*/
Function sync(Function f);
/**
* Convert the execution to one performed asynchronously on the IsolatedExecutor, returning a Future of the execution result
*/
BiFunction> async(BiFunction f);
/**
* Convert the execution to one performed synchronously on the IsolatedExecutor
*/
BiFunction sync(BiFunction f);
/**
* Convert the execution to one performed asynchronously on the IsolatedExecutor, returning a Future of the execution result
*/
TriFunction> async(TriFunction f);
/**
* Convert the execution to one performed synchronously on the IsolatedExecutor
*/
TriFunction sync(TriFunction f);
/**
* Convert the execution to one performed asynchronously on the IsolatedExecutor, returning a Future of the execution result
*/
QuadFunction> async(QuadFunction f);
/**
* Convert the execution to one performed synchronously on the IsolatedExecutor
*/
QuadFunction sync(QuadFunction f);
/**
* Convert the execution to one performed asynchronously on the IsolatedExecutor, returning a Future of the execution result
*/
QuintFunction> async(QuintFunction f);
/**
* Convert the execution to one performed synchronously on the IsolatedExecutor
*/
QuintFunction sync(QuintFunction f);
}