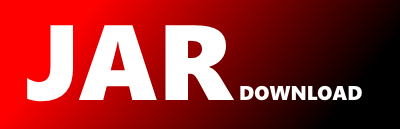
org.apache.cayenne.DataObject Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cayenne-client-nodeps
Show all versions of cayenne-client-nodeps
Cayenne Object Persistence Framework
/*****************************************************************
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
****************************************************************/
package org.apache.cayenne;
import org.apache.cayenne.access.DataContext;
import org.apache.cayenne.validation.ValidationResult;
/**
* Defines basic methods for a persistent object in Cayenne.
*
* @author Andrei Adamchik
*/
public interface DataObject extends Persistent {
public static final long DEFAULT_VERSION = Long.MIN_VALUE;
/**
* Returns a data context this object is registered with, or null if this object has
* no associated DataContext.
*/
public DataContext getDataContext();
/**
* Sets object DataContext.
*/
public void setDataContext(DataContext ctxt);
/**
* Modifies a value of a named property without altering the object state in any way,
* and without triggering any database operations. This method is intended mostly for
* internal use by Cayenne framework, and shouldn't be called from the application
* code.
*/
public void writePropertyDirectly(String propertyName, Object val);
/**
* Returns mapped property value as curently stored in the DataObject. Returned value
* maybe a fault or a real value. This method will not attempt to resolve faults, or
* to read unmapped properties.
*/
public Object readPropertyDirectly(String propertyName);
/**
* Returns a value of the property identified by a property path. Supports reading
* both mapped and unmapped properties. Unmapped properties are accessed in a manner
* consistent with JavaBeans specification.
*
* Property path (or nested property) is a dot-separated path used to traverse object
* relationships until the final object is found. If a null object found while
* traversing path, null is returned. If a list is encountered in the middle of the
* path, CayenneRuntimeException is thrown. Unlike
* {@link #readPropertyDirectly(String)}, this method will resolve an object if it is
* HOLLOW.
*
* Examples:
*
*
* - Read this object property:
* String name = (String)artist.readNestedProperty("name");
*
*
* - Read an object related to this object:
* Gallery g = (Gallery)paintingInfo.readNestedProperty("toPainting.toGallery");
*
*
*
* - Read a property of an object related to this object:
* String name = (String)painting.readNestedProperty("toArtist.artistName");
*
*
*
* - Read to-many relationship list:
* List exhibits = (List)painting.readNestedProperty("toGallery.exhibitArray");
*
*
*
* - Read to-many relationship in the middle of the path (throws exception):
* String name = (String)artist.readNestedProperty("paintingArray.paintingName");
*
*
*
*
*
* @since 1.0.5
*/
public Object readNestedProperty(String path);
/**
* Returns a value of the property identified by propName. Resolves faults if needed.
* This method can safely be used instead of or in addition to the auto-generated
* property accessors in subclasses of CayenneDataObject.
*/
public Object readProperty(String propName);
/**
* Sets the property to the new value. Resolves faults if needed. This method can be
* safely used instead of or in addition to the auto-generated property modifiers to
* set simple properties. Note that to set to-one relationships use
* {@link #setToOneTarget(String, DataObject, boolean)}.
*
* @param propertyName a name of the bean property being modified.
* @param value a new value of the property.
*/
public void writeProperty(String propertyName, Object value);
public void addToManyTarget(String relName, DataObject val, boolean setReverse);
public void removeToManyTarget(String relName, DataObject val, boolean setReverse);
/**
* Sets to-one relationship to a new value. Resolves faults if needed. This method can
* safely be used instead of or in addition to the auto-generated property modifiers
* to set properties that are to-one relationships.
*
* @param relationshipName a name of the bean property being modified - same as the
* name of ObjRelationship.
* @param value a new value of the property.
* @param setReverse whether to update the reverse relationship pointing from the old
* and new values of the property to this object.
*/
public void setToOneTarget(
String relationshipName,
DataObject value,
boolean setReverse);
/**
* Notification method called by DataContext after the object was read from the
* database.
*/
public void fetchFinished();
/**
* Returns a version of a DataRow snapshot that was used to create this object.
*
* @since 1.1
*/
public long getSnapshotVersion();
/**
* @since 1.1
*/
public void setSnapshotVersion(long snapshotVersion);
/**
* Initializes object with data from cache or from the database, if this object is not
* fully resolved.
*
* @since 1.1
* @deprecated since 1.2 use 'getObjectContext().prepareForAccess(object)'
*/
public void resolveFault();
/**
* Performs property validation of the NEW object, appending any validation failures
* to the provided validationResult object. This method is invoked by DataContext
* before committing a NEW object to the database.
*
* @since 1.1
*/
public void validateForInsert(ValidationResult validationResult);
/**
* Performs property validation of the MODIFIED object, appending any validation
* failures to the provided validationResult object. This method is invoked by
* DataContext before committing a MODIFIED object to the database.
*
* @since 1.1
*/
public void validateForUpdate(ValidationResult validationResult);
/**
* Performs property validation of the DELETED object, appending any validation
* failures to the provided validationResult object. This method is invoked by
* DataContext before committing a DELETED object to the database.
*
* @since 1.1
*/
public void validateForDelete(ValidationResult validationResult);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy