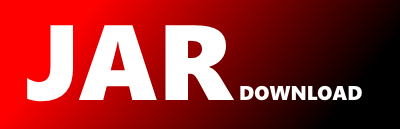
org.apache.cayenne.access.util.DefaultOperationObserver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cayenne-client-nodeps
Show all versions of cayenne-client-nodeps
Cayenne Object Persistence Framework
/*****************************************************************
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
****************************************************************/
package org.apache.cayenne.access.util;
import java.io.PrintWriter;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import org.apache.log4j.Level;
import org.apache.cayenne.CayenneException;
import org.apache.cayenne.access.OperationObserver;
import org.apache.cayenne.access.ResultIterator;
import org.apache.cayenne.query.Query;
import org.apache.cayenne.util.Util;
/**
* Simple implementation of OperationObserver interface. Useful as a superclass of other
* implementations of OperationObserver. This implementation only tracks transaction
* events and exceptions.
*
* This operation observer is unsafe to use in application, since it doesn't rethrow
* the exceptions immediately, and may cause the database to hang.
*
*
* @author Andrei Adamchik
*/
public class DefaultOperationObserver implements OperationObserver {
/**
* @deprecated Unused since 1.2
*/
public static final Level DEFAULT_LOG_LEVEL = Level.INFO;
protected List globalExceptions = new ArrayList();
protected Map queryExceptions = new HashMap();
/**
* Prints the information about query and global exceptions.
*/
public void printExceptions(PrintWriter out) {
if (globalExceptions.size() > 0) {
if (globalExceptions.size() == 1) {
out.println("Global Exception:");
}
else {
out.println("Global Exceptions:");
}
Iterator it = globalExceptions.iterator();
while (it.hasNext()) {
Throwable th = (Throwable) it.next();
th.printStackTrace(out);
}
}
if (queryExceptions.size() > 0) {
if (queryExceptions.size() == 1) {
out.println("Query Exception:");
}
else {
out.println("Query Exceptions:");
}
Iterator it = queryExceptions.keySet().iterator();
while (it.hasNext()) {
Throwable th = (Throwable) queryExceptions.get(it.next());
th.printStackTrace(out);
}
}
}
/** Returns a list of global exceptions that occured during data operation run. */
public List getGlobalExceptions() {
return globalExceptions;
}
/** Returns a list of exceptions that occured during data operation run by query. */
public Map getQueryExceptions() {
return queryExceptions;
}
/**
* Returns true
if at least one exception was registered during query
* execution.
*/
public boolean hasExceptions() {
return globalExceptions.size() > 0 || queryExceptions.size() > 0;
}
/**
* Returns a log level level that should be used when logging query execution.
*
* @deprecated since 1.2
*/
public Level getLoggingLevel() {
return Level.INFO;
}
/**
* Sets log level that should be used for queries. If level
argument is
* null, level is set to DEFAULT_LOG_LEVEL. If level
is equal or higher
* than log level configured for QueryLogger, query SQL statements will be logged.
*
* @deprecated since 1.2
*/
public void setLoggingLevel(Level level) {
// noop
}
public void nextCount(Query query, int resultCount) {
}
public void nextBatchCount(Query query, int[] resultCount) {
}
public void nextDataRows(Query query, List dataRows) {
// noop
}
/**
* Closes ResultIterator without reading its data. If you implement a custom subclass,
* only call super if closing the iterator is what you need.
*/
public void nextDataRows(Query query, ResultIterator it) {
if (it != null) {
try {
it.close();
}
catch (CayenneException ex) {
// don't throw here....
nextQueryException(query, ex);
}
}
}
/**
* Closes ResultIterator without reading its data. If you implement a custom subclass,
* only call super if closing the iterator is what you need.
*
* @since 1.2
*/
public void nextGeneratedDataRows(Query query, ResultIterator keysIterator) {
if (keysIterator != null) {
try {
keysIterator.close();
}
catch (CayenneException ex) {
// don't throw here....
nextQueryException(query, ex);
}
}
}
public void nextQueryException(Query query, Exception ex) {
queryExceptions.put(query, Util.unwindException(ex));
}
public void nextGlobalException(Exception ex) {
globalExceptions.add(Util.unwindException(ex));
}
/**
* Returns false
.
*/
public boolean isIteratedResult() {
return false;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy