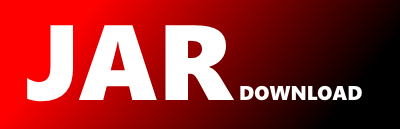
org.apache.cayenne.conf.ConfigLoader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cayenne-client-nodeps
Show all versions of cayenne-client-nodeps
Cayenne Object Persistence Framework
/*****************************************************************
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
****************************************************************/
package org.apache.cayenne.conf;
import java.io.IOException;
import java.io.InputStream;
import java.util.HashMap;
import java.util.Map;
import org.apache.cayenne.util.Util;
import org.xml.sax.Attributes;
import org.xml.sax.ContentHandler;
import org.xml.sax.InputSource;
import org.xml.sax.SAXException;
import org.xml.sax.SAXParseException;
import org.xml.sax.XMLReader;
import org.xml.sax.helpers.DefaultHandler;
/**
* Class that performs runtime loading of Cayenne configuration.
*
* @author Andrei Adamchik
*/
public class ConfigLoader {
protected XMLReader parser;
protected ConfigLoaderDelegate delegate;
/** Creates new ConfigLoader. */
public ConfigLoader(ConfigLoaderDelegate delegate) throws Exception {
if (delegate == null) {
throw new IllegalArgumentException("Delegate must not be null.");
}
this.delegate = delegate;
parser = Util.createXmlReader();
}
/**
* Returns the delegate.
*
* @return ConfigLoaderDelegate
*/
public ConfigLoaderDelegate getDelegate() {
return delegate;
}
/**
* Parses XML input, invoking delegate methods to interpret loaded XML.
*
* @param in
* @return boolean
*/
public boolean loadDomains(InputStream in) {
DefaultHandler handler = new RootHandler();
parser.setContentHandler(handler);
parser.setErrorHandler(handler);
try {
delegate.startedLoading();
parser.parse(new InputSource(in));
delegate.finishedLoading();
}
catch (IOException ioex) {
getDelegate().loadError(ioex);
}
catch (SAXException saxex) {
getDelegate().loadError(saxex);
}
// return true if no failures
return !getDelegate().getStatus().hasFailures();
}
// SAX handlers start below
/**
* Handler for the root element. Its only child must be the "domains" element.
*/
private class RootHandler extends DefaultHandler {
/**
* Handles the start of a datadomains element. A domains handler is created and
* initialised with the element name and attributes.
*
* @exception SAXException if the tag given is not "domains"
*/
public void startElement(
String namespaceURI,
String localName,
String qName,
Attributes attrs) throws SAXException {
if (localName.equals("domains")) {
delegate.shouldLoadProjectVersion(attrs.getValue("", "project-version"));
new DomainsHandler(parser, this);
}
else {
throw new SAXParseException(" should be the root element. <"
+ localName
+ "> is unexpected.", null);
}
}
}
/**
* Handler for the top level "project" element.
*/
private class DomainsHandler extends AbstractHandler {
/**
* Constructor which just delegates to the superconstructor.
*
* @param parentHandler The handler which should be restored to the parser at the
* end of the element. Must not be null
.
*/
public DomainsHandler(XMLReader parser, ContentHandler parentHandler) {
super(parser, parentHandler);
}
/**
* Handles the start of a top-level element within the project. An appropriate
* handler is created and initialised with the details of the element.
*/
public void startElement(
String namespaceURI,
String localName,
String qName,
Attributes atts) throws SAXException {
if (localName.equals("domain")) {
new DomainHandler(getParser(), this).init(localName, atts);
}
else if (localName.equals("view")) {
new ViewHandler(getParser(), this).init(atts);
}
else {
String message = " or are only valid children of . <"
+ localName
+ "> is unexpected.";
throw new SAXParseException(message, null);
}
}
}
private class ViewHandler extends AbstractHandler {
public ViewHandler(XMLReader parser, ContentHandler parentHandler) {
super(parser, parentHandler);
}
public void init(Attributes attrs) {
String name = attrs.getValue("", "name");
String location = attrs.getValue("", "location");
delegate.shouldRegisterDataView(name, location);
}
}
/**
* Handler for the "domain" element.
*/
private class DomainHandler extends AbstractHandler {
private String domainName;
private Map properties;
private Map mapLocations;
public DomainHandler(XMLReader parser, ContentHandler parentHandler) {
super(parser, parentHandler);
}
public void init(String name, Attributes attrs) {
domainName = attrs.getValue("", "name");
mapLocations = new HashMap();
properties = new HashMap();
delegate.shouldLoadDataDomain(domainName);
}
public void startElement(
String namespaceURI,
String localName,
String qName,
Attributes atts) throws SAXException {
if (localName.equals("property")) {
new PropertyHandler(getParser(), this).init(atts, properties);
}
else if (localName.equals("map")) {
// "map" elements go after "property" elements
// must flush properties if there are any
loadProperties();
new MapHandler(getParser(), this).init(
localName,
atts,
domainName,
mapLocations);
}
else if (localName.equals("node")) {
// "node" elements go after "map" elements
// must flush maps if there are any
loadMaps();
new NodeHandler(getParser(), this).init(localName, atts, domainName);
}
else {
String message = " or
© 2015 - 2024 Weber Informatics LLC | Privacy Policy