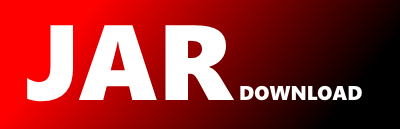
org.apache.cayenne.conf.ConfigSaver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cayenne-client-nodeps
Show all versions of cayenne-client-nodeps
Cayenne Object Persistence Framework
/*****************************************************************
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
****************************************************************/
package org.apache.cayenne.conf;
import java.io.PrintWriter;
import java.util.Iterator;
import org.apache.cayenne.conn.DataSourceInfo;
import org.apache.cayenne.project.Project;
import org.apache.cayenne.util.Util;
/**
* Class that does saving of Cayenne configuration.
*
* @author Andrei Adamchik
*/
public class ConfigSaver {
protected ConfigSaverDelegate delegate;
/**
* Constructor for ConfigSaver.
*/
public ConfigSaver() {
super();
}
/**
* Constructor for ConfigSaver.
*/
public ConfigSaver(ConfigSaverDelegate delegate) {
this.delegate = delegate;
}
/**
* Saves domains into the specified file. Assumes that the maps have already
* been saved.
*/
public void storeDomains(PrintWriter pw) {
pw.println("");
pw.println("");
Iterator it = delegate.domainNames();
while (it.hasNext()) {
storeDomain(pw, (String) it.next());
}
Iterator views = delegate.viewNames();
while (views.hasNext()) {
storeDataView(pw, (String) views.next());
}
pw.println(" ");
}
protected void storeDataView(PrintWriter pw, String dataViewName) {
String location = delegate.viewLocation(dataViewName);
pw.print(" ");
}
protected void storeDomain(PrintWriter pw, String domainName) {
pw.println("");
// store properties
Iterator properties = delegate.propertyNames(domainName);
boolean breakNeeded = properties.hasNext();
while (properties.hasNext()) {
String name = (String) properties.next();
if (name == null) {
continue;
}
String value = delegate.propertyValue(domainName, name);
if (value == null) {
continue;
}
pw.print("\t ");
}
// store maps
Iterator maps = delegate.mapNames(domainName);
if (maps.hasNext()) {
if (breakNeeded) {
pw.println();
}
breakNeeded = true;
}
while (maps.hasNext()) {
String mapName = (String) maps.next();
String mapLocation = delegate.mapLocation(domainName, mapName);
pw.print("\t");
}
// store nodes
Iterator nodes = delegate.nodeNames(domainName);
if (nodes.hasNext() && breakNeeded) {
pw.println();
}
while (nodes.hasNext()) {
String nodeName = (String) nodes.next();
String datasource = delegate.nodeDataSourceName(domainName, nodeName);
String adapter = delegate.nodeAdapterName(domainName, nodeName);
String factory = delegate.nodeFactoryName(domainName, nodeName);
Iterator mapNames = delegate.linkedMapNames(domainName, nodeName);
pw.println("\t");
while (mapNames.hasNext()) {
String mapName = (String) mapNames.next();
pw.println("\t\t\t ");
}
pw.println("\t ");
}
pw.println(" ");
}
/**
* Stores DataSolurceInfo to the specified PrintWriter.
* info
object may contain full or partial information.
*/
public void storeDataNode(PrintWriter out, DataSourceInfo info) {
out.println("");
out.print("");
if (info.getDataSourceUrl() != null) {
String encoded = Util.encodeXmlAttribute(info.getDataSourceUrl());
out.println("\t ");
}
out.println(
"\t ");
if (info.getUserName() != null || info.getPassword() != null) {
out.print("\t ");
}
out.println(" ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy